Spring Boot 整合 elasticsearch
一、简介
我们的应用经常需要添加检索功能,开源的 ElasticSearch 是目前全文搜索引擎的 首选。他可以快速的存储、搜索和分析海量数据。Spring Boot通过整合Spring Data ElasticSearch为我们提供了非常便捷的检索功能支持;
Elasticsearch是一个分布式搜索服务,提供Restful API,底层基于Lucene,采用 多shard(分片)的方式保证数据安全,并且提供自动resharding的功能,github 等大型的站点也是采用了ElasticSearch作为其搜索服务,
二、安装elasticsearch
我们采用 docker镜像安装的方式。
# 下载镜像 docker pull elasticsearch #启动镜像,elasticsearch 启动是会默认分配2G的内存 ,我们启动是设置小一点,防止我们内存不够启动失败 #9200是elasticsearch 默认的web通信接口,9300是分布式情况下,elasticsearch个节点通信的端口 docker run -e ES_JAVA_OPTS="-Xms256m -Xmx256m" -d -p 9200:9200 -p 9300:9300 --name es01 5c1e1ecfe33a
访问 127.0.0.1:9200 如下图,说明安装成功
三、elasticsearch的一些概念
- 以 员工文档 的形式存储为例:一个文档代表一个员工数据。存储数据到 ElasticSearch 的行为叫做索引 ,但在索引一个文档之前,需要确定将文档存 储在哪里。
- 一个 ElasticSearch 集群可以 包含多个索引 ,相应的每个索引可以包含多个类型。这些不同的类型存储着多个文档 ,每个文档又有 多个 属性 。
-
类似关系:
- 索引-数据库
- 类型-表
- 文档-表中的记录 – 属性-列
elasticsearch使用可以参早官方文档,在这里不在讲解。
四、整合 elasticsearch
创建项目 springboot-elasticsearch,引入web支持
SpringBoot 提供了两种方式操作elasticsearch,Jest 和 SpringData。
Jest 操作 elasticsearch
Jest是ElasticSearch的Java HTTP Rest客户端。
ElasticSearch已经有一个Java API,ElasticSearch也在内部使用它,但是Jest填补了空白,它是ElasticSearch Http Rest接口缺少的客户端。
1. pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.gf</groupId> <artifactId>springboot-elasticsearch</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>springboot-elasticsearch</name> <description>Demo project for Spring Boot</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.1.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-elasticsearch</artifactId> </dependency> <dependency> <groupId>io.searchbox</groupId> <artifactId>jest</artifactId> <version>5.3.3</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
2. application.properties
spring.elasticsearch.jest.uris=http://127.0.0.1:9200
3. Article
package com.gf.entity; import io.searchbox.annotations.JestId; public class Article { @JestId private Integer id; private String author; private String title; private String content; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getContent() { return content; } public void setContent(String content) { this.content = content; } @Override public String toString() { final StringBuilder sb = new StringBuilder( "{\"Article\":{" ); sb.append( "\"id\":" ) .append( id ); sb.append( ",\"author\":\"" ) .append( author ).append( '\"' ); sb.append( ",\"title\":\"" ) .append( title ).append( '\"' ); sb.append( ",\"content\":\"" ) .append( content ).append( '\"' ); sb.append( "}}" ); return sb.toString(); } }
4. springboot测试类
package com.gf; import com.gf.entity.Article; import io.searchbox.client.JestClient; import io.searchbox.core.Index; import io.searchbox.core.Search; import io.searchbox.core.SearchResult; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.test.context.junit4.SpringRunner; import java.io.IOException; @RunWith(SpringRunner.class) @SpringBootTest public class SpringbootElasticsearchApplicationTests { @Autowired JestClient jestClient; @Test public void createIndex() { //1. 给ES中索引(保存)一个文档 Article article = new Article(); article.setId( 1 ); article.setTitle( "好消息" ); article.setAuthor( "张三" ); article.setContent( "Hello World" ); //2. 构建一个索引 Index index = new Index.Builder( article ).index( "gf" ).type( "news" ).build(); try { //3. 执行 jestClient.execute( index ); } catch (IOException e) { e.printStackTrace(); } } @Test public void search() { //查询表达式 String query = "{\n" + " \"query\" : {\n" + " \"match\" : {\n" + " \"content\" : \"hello\"\n" + " }\n" + " }\n" + "}"; //构建搜索功能 Search search = new Search.Builder( query ).addIndex( "gf" ).addType( "news" ).build(); try { //执行 SearchResult result = jestClient.execute( search ); System.out.println(result.getJsonString()); } catch (IOException e) { e.printStackTrace(); } } }
Jest的更多api ,可以参照github的文档:https://github.com/searchbox-io/Jest
SpringData 操作 elasticsearch
1. application.properties
spring.data.elasticsearch.cluster-name=elasticsearch spring.data.elasticsearch.cluster-nodes=127.0.0.1:9300
2. Book
package com.gf.entity; @Document( indexName = "gf" , type = "book") public class Book { private Integer id; private String bookName; private String author; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getBookName() { return bookName; } public void setBookName(String bookName) { this.bookName = bookName; } public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } @Override public String toString() { final StringBuilder sb = new StringBuilder( "{\"Book\":{" ); sb.append( "\"id\":" ) .append( id ); sb.append( ",\"bookName\":\"" ) .append( bookName ).append( '\"' ); sb.append( ",\"author\":\"" ) .append( author ).append( '\"' ); sb.append( "}}" ); return sb.toString(); } }
3. BookRepository
package com.gf.repository; import com.gf.entity.Book; import org.springframework.data.elasticsearch.repository.ElasticsearchRepository; import java.util.List; public interface BookRepository extends ElasticsearchRepository<Book, Integer>{ List<Book> findByBookNameLike(String bookName); }
4. springboot 测试类
package com.gf; import com.gf.entity.Article; import com.gf.entity.Book; import com.gf.repository.BookRepository; import io.searchbox.client.JestClient; import io.searchbox.core.Index; import io.searchbox.core.Search; import io.searchbox.core.SearchResult; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.test.context.junit4.SpringRunner; import java.io.IOException; import java.util.List; @RunWith(SpringRunner.class) @SpringBootTest public class SpringbootElasticsearchApplicationTests { @Autowired BookRepository bookRepository; @Test public void createIndex2(){ Book book = new Book(); book.setId(1); book.setBookName("西游记"); book.setAuthor( "吴承恩" ); bookRepository.index( book ); } @Test public void useFind() { List<Book> list = bookRepository.findByBookNameLike( "游" ); for (Book book : list) { System.out.println(book); } } }
我们启动测试 ,发现报错 。
这个报错的原因是springData的版本与我elasticsearch的版本有冲突,下午是springData官方文档给出的适配表。
我们使用的springdata elasticsearch的 版本是3.1.3 ,对应的版本应该是6.2.2版本,而我们是的 elasticsearch 是 5.6.9,所以目前我们需要更换elasticsearch的版本为6.X
docker pull elasticsearch:6.5.1 docker run -e ES_JAVA_OPTS="-Xms256m -Xmx256m" -d -p 9200:9200 -p 9300:9300 --name es02 镜像ID
访问127.0.0.1:9200
集群名为docker-cluster,所以我们要修改application.properties的配置了
spring.data.elasticsearch.cluster-name=docker-cluster spring.data.elasticsearch.cluster-nodes=127.0.0.1:9300
我们再次进行测试,测试可以通过了 。我们访问http://127.0.0.1:9200/gf/book/1,可以得到我们存入的索引信息。
源码
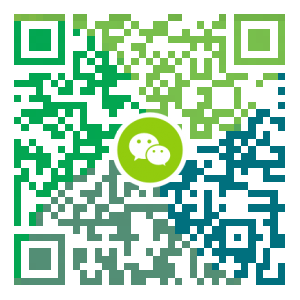
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
CentOS7下安装CDH,clouderamanager,hadoop
一、实验背景 我们是四台电脑一起搭建Hadoop集群 A,B,C,D四个人个有一台电脑,分别在各自的电脑上安装虚拟机,虚拟机装centOS7。(我们建议主机namenode内存大一点8g,硬盘最好100g以上,其余节点2g,50g即可,下文会阐述原因) 系统:VMware下的centOS7 jdk:1.8.0_171 jdk-8u171-linux-x64.tar.gz(Oracle) MySQL: mysql-5.7.18-1.el7.x86_64.rpm-bundle.tar以及mysql-connector-java-5.1.42-bin.jar(老师云盘给的) CDH: CDH-5.11.1-1.cdh5.11.1.p0.4-el7.parcel.sha1; CDH-5.11.1-1.cdh5.11.1.p0.4-el7.parcel; manifest.json;(手动新建的文件) ClouderaManger:cloudera-manager-centos7-cm5.11.1_x86_64.tar.gz 所需要的资源下载地址: parcels:http://archive....
- 下一篇
【直播资料下载】HBase多语言访问
内容概要: 非JAVA语言需要通过Thrift访问HBase,了解Thrift整体架构和基本原理,详细介绍HBase Thrift接口最佳实践,快速入门 讲师介绍:修者——阿里巴巴-云hbase内核开发 高级工程师 点击链接入群 https://dwz.cn/Fvqv066s 或扫码进群 直播链接:https://yq.aliyun.com/live/1089PPT下载:https://yq.aliyun.com/download/3550
相关文章
文章评论
共有0条评论来说两句吧...