React 入门实例 参考阮一峰博客
React 可以在浏览器运行,也可以在服务器运行。服务器的用法与浏览器差别不大。
1 hello world程序
**开发环境**visual studio code和谷歌浏览器(谷歌浏览器翻墙安装React Developer Tools)。
1.1 使用 React 开发新项目
前提安装npm工具,Node版本 >= 6。
在工作空间,执行以下命令:
1. npm install -g create-react-app 2. create-react-app my-app 3. cd my-app 4. npm start
- 1
- 2
- 3
- 4
然后打开http:// localhost:3000 /查看您的应用程序。
创建React App不处理后端逻辑或数据库; 它只是创建一个前端构建管道,所以你可以将它用于任何你想要的后端。
当您准备部署到生产环境时,运行
npm run build
- 1
将在build文件夹中创建应用程序的优化版本。将 src 子目录的 js 文件进行语法转换,转码后的文件全部放在 build 子目录。
结果
在当前文件夹内创建一个目录。my-app
在该目录内,它将生成初始项目结构并安装依赖项:
my-app ├── README.md ├── node_modules ├── package.json ├── .gitignore ├── public │ └── favicon.ico │ └── index.html │ └── manifest.json └── src └── App.css └── App.js └── App.test.js └── index.css └── index.js └── logo.svg └── registerServiceWorker.js
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
没有配置或复杂的文件夹结构,只有构建应用程序所需的文件。
安装完成后,您可以打开项目文件夹,编辑目录、文件开发自己的项目(如下面: 修改APP.js)。
如果您更改了代码,该页面将自动重新加载。您将在控制台中看到构建错误和lint警告。
1) APP.js文件中编写Hello world程序
import React, { Component } from 'react'; class App extends Component { render() { return ( <div className="App"> <h1 className="App-title">Hello World</h1> </div> ); } } export default App;
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
注意:组件类只能包含一个顶层标签,否则也会报错
1.2 运行现有React项目/框架
一般readme文件中有说明,仔细阅读。运行现有源码包的基本步骤
1) 在项目的目录安装依赖
$ npm install
- 1
2) 将src子目录中的所有jsx文件转换为js文件。
$ npm run build
- 1
- 2
3) 启动http server
$ node server.js
- 1
2 render()
render()是 React 的最基本方法,用于将模板转为 HTML 语言,并插入指定的 DOM 节点。
例如:index.js中将App标签,插入 root节点
ReactDOM.render( <App />, document.getElementById('root') );
- 1
- 2
- 3
- 4
3 JSX 语法
HTML 语言直接写在 JavaScript 语言之中,不加任何引号,这就是 JSX 的语法,它允许 HTML 与 JavaScript 的混写。
demo2
(项目index.js中修改import App from ‘./demo1’即可运行对应示例)
import React, { Component } from 'react'; var names = ['Alice', 'Emily', 'Kate']; class App extends Component{ render(){ return( <div> { names.map(function (name, index) { return <div key={index}>Hello, {name}!</div> }) } </div> ) }; } export default App;
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
JSX 的基本语法规则:遇到 HTML 标签(以 < 开头),就用 HTML 规则解析;遇到代码块(以 { 开头),就用 JavaScript 规则解析。
JSX 允许直接在模板插入 JavaScript 变量。如果这个变量是一个数组,则会展开这个数组的所有成员。JSX 会把它的所有成员,添加到模板。
demo03
import React, { Component } from 'react'; var arr = [ <h1>Hello world!</h1>, <h2>React is awesome</h2>, ]; class App extends Component{ render(){ return( <div> <div>{arr}</div> </div> ) }; } export default App;
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
4 组件
React 允许将代码封装成组件(component),然后像插入普通 HTML 标签一样,在网页中插入这个组件。class关键字用于生成一个组件类。
demo04
import React, { Component } from 'react'; class App extends Component{ render(){ return( <div> <HelloMessage name="pengwei" />, </div> ) }; } class HelloMessage extends Component{ render() { return <h1>Hello {this.props.name}</h1>; } } export default App;
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
上面代码中,变量 HelloMessage 就是一个组件类。模板插入 时,会自动生成 HelloMessage 的一个实例(下文的”组件”都指组件类的实例)。所有组件类都必须有自己的 render 方法,用于输出组件。
注意,组件类的第一个字母必须大写,否则会报错,比如HelloMessage不能写成helloMessage。另外,组件类只能包含一个顶层标签,否则也会报错。
组件的用法与原生的 HTML 标签完全一致,可以任意加入属性,比如 ,就是 HelloMessage 组件加入一个 name 属性,值为 John。组件的属性可以在组件类的 this.props 对象上获取,比如 name 属性就可以通过 this.props.name 读取。
注意,添加组件属性, class 属性需要写成 className ,for 属性需要写成 htmlFor ,这是因为 class 和 for 是 JavaScript 的保留字。
5 this.props.children
this.props 对象的属性与组件的属性一一对应,但是有一个例外,就是 this.props.children 属性,它表示组件的所有子节点。
demo05
import React, { Component } from 'react'; class App extends Component{ render(){ return( <div> <Parent> <span>hello</span> <span>world</span> </Parent> </div> ) }; } class Parent extends Component{ render(){ return( <ol> {React.Children.map(this.props.children,function(child) {return <li>{child}</li>;})} </ol> ) }; } export default App;
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
注意, this.props.children 的值有三种可能:如果当前组件没有子节点,它就是 undefined ;如果有一个子节点,数据类型是 object ;如果有多个子节点,数据类型就是 array 。所以,处理 this.props.children 的时候要小心。
React 提供一个工具方法 React.Children 来处理 this.props.children 。我们可以用 React.Children.map 来遍历子节点,而不用担心 this.props.children 的数据类型
6 PropTypes和DefaultProps
组件的属性可以接受任意值,字符串、对象、函数等等都可以。有时,我们需要一种机制,验证别人使用组件时,提供的参数是否符合要求。
- PropTypes属性,就是用来验证组件实例的属性是否符合要求。
- DefaultProps 方法可以用来设置组件属性的默认值。
demo06
import React, { Component } from 'react'; import PropTypes from 'prop-types'; class App extends Component{ render(){ return( <div> <DefaultMessage /> </div> ); }; } class DefaultMessage extends Component{ render() { return (<h1>Hello {this.props.name}</h1>); } } DefaultMessage.propTypes = { name: PropTypes.string }; DefaultMessage.defaultProps ={ name : 'World' } export default App;
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
7 获取真实的DOM节点
组件并不是真实的 DOM 节点,而是存在于内存之中的一种数据结构,叫做虚拟 DOM (virtual DOM)。只有当它插入文档以后,才会变成真实的 DOM 。根据 React 的设计,所有的 DOM 变动,都先在虚拟 DOM 上发生,然后再将实际发生变动的部分,反映在真实 DOM上,这种算法叫做 DOM diff ,它可以极大提高网页的性能表现。
但是,有时需要从组件获取真实 DOM 的节点,这时就要用到 ref 属性
demo07
import React, { Component } from 'react'; class App extends Component{ render(){ return( <div> <TrueDom /> </div> ); }; } class TrueDom extends Component{ constructor(props) { super(props); // This binding is necessary to make `this` work in the callback this.handleClick = this.handleClick.bind(this); } handleClick() { this.refs.myTextInput.focus(); }; render() { return( <div> <input type="text" ref="myTextInput" /> <input type="button" value="Focus the text input" onClick={this.handleClick} /> </div> ); }; } export default App;
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
需要注意的是,由于 this.refs.[refName] 属性获取的是真实 DOM ,所以必须等到虚拟 DOM 插入文档以后,才能使用这个属性,否则会报错。上面代码中,通过为组件指定 Click 事件的回调函数,确保了只有等到真实 DOM 发生 Click 事件之后,才会读取 this.refs.[refName] 属性。
8 this.state
react将组件看成是一个状态机,一开始有一个初始状态,然后用户互动,导致状态变化,从而触发重新渲染 UI。
由于 this.props 和 this.state 都用于描述组件的特性,可能会产生混淆。一个简单的区分方法是,this.props 表示那些一旦定义,就不再改变的特性,而 this.state 是会随着用户互动而产生变化的特性
demo08
import React, { Component } from 'react'; class App extends Component{ render(){ return( <div> <LikeButton/> </div> ) }; } class LikeButton extends Component{ constructor(props) { super(props); this.state = {liked: false}; // This binding is necessary to make `this` work in the callback this.handleClick = this.handleClick.bind(this); } handleClick(event) { this.setState({liked: !this.state.liked}); }; render() { var text = this.state.liked ? 'like' : 'don\'t liked'; return ( <p onClick={this.handleClick}> You {text} this. Click to toggle. </p> ); }; } export default App;
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
9 表单
用户在表单填入的内容,属于用户跟组件的互动,所以不能用 this.props 读取
demo9
import React, { Component } from 'react'; class App extends Component{ render(){ return( <div> <Input/> </div> ) }; } class Input extends Component{ constructor(props) { super(props); this.state = {value: 'Hello!'}; // This binding is necessary to make `this` work in the callback this.handleChange = this.handleChange.bind(this); }; handleChange(event) { this.setState({value: event.target.value}); }; render() { var value = this.state.value; return ( <div> <input type="text" value={value} onChange={this.handleChange} /> <p>{value}</p> </div> ); }; } export default App;
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
上面代码中,文本输入框的值,不能用 this.props.value 读取,而要定义一个 onChange 事件的回调函数,通过 event.target.value 读取用户输入的值。
10 组件的生命周期
组件的生命周期分成三个状态:
- Mounting:已插入真实 DOM
- Updating:正在被重新渲染
- Unmounting:已移出真实 DOM
React 为每个状态都提供了两种处理函数,will 函数在进入状态之前调用,did 函数在进入状态之后调用,三种状态共计五种处理函数:
- componentWillMount()
- componentDidMount()
- componentWillUpdate(object nextProps, object nextState)
- componentDidUpdate(object prevProps, object prevState)
- componentWillUnmount()
此外,React 还提供两种特殊状态的处理函数:
- componentWillReceiveProps(object nextProps):已加载组件收到新的参数时调用
- shouldComponentUpdate(object nextProps, object nextState):组件判断是否重新渲染时调用
demo10
import React, { Component } from 'react'; class App extends Component{ render(){ return( <div> <ComponentLifestyle name = "pengwei"/> </div> ) }; } class ComponentLifestyle extends Component{ constructor(props) { super(props); this.state = {opacity: 1.0}; }; componentWillMount() { this.timer = setInterval(function () { var opacity = this.state.opacity; var flag = this.state.flag; if(opacity >= 1||opacity<=0) {flag = !flag;} if (flag){ opacity -= 0.02; } else{ opacity += 0.02; } this.setState({ opacity: opacity, flag: flag }); }.bind(this), 100); }; render() { return ( <div style={{opacity: this.state.opacity}} flag = {true}> Hello {this.props.name} </div> ); }; } export default App;
原文发布时间:2018年03月25日
本文来源CSDN博客如需转载请紧急联系作者
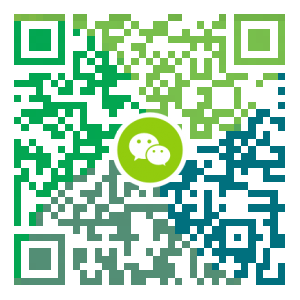
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
JavaScript的初步探索(JS的入坑笔录)
Java script简称js,是web学习的一个重要组成部分。 首先,Js是什么?JS简介: 这门语言可用于 HTML 和 web,更可广泛用于服务器、PC、笔记本电脑、平板电脑和智能手机等设备。 它是一种浏览器脚本语言,它由由若干语言组成,语句是基本单位。语句是由函数、数据、表达式组成。它严格区分大小写,当然事件响应句柄不区分,因为事件响应句柄属于DOM,也属于html。它是也是与HTML一样是一种解释性语言。 注:下文中很多类似的图片,您可以点击一下图片,图片自动放大便于查看。 js的历史: • JavaScript语言最初称为LiveScript语言,是由Netscape(网景)公司开发的脚本语言。希望借助流行的Java使LiveScript流行起来,因此改名为JavaScript。 • Microsoft在IE3.0中引入了JavaScript。因为Microsoft没有被授权使用JavaScript商标,因此将其改名为Jscript。 • 1997年,JavaScript 1.1被提交到ECMA(欧洲计算机制造商协会)。并在1997.6ECMA制定了第一个正式语言规范ECM...
- 下一篇
狼叔:如何正确的学习Node.js
1. 【知乎Live】狼叔:如何正确的学习Node.js 预览地址 i5ting.github.io/How-to-lear… Live 简介 你好,我是 i5ting ,江湖人称「狼叔」,目前是阿里巴巴技术专家,斯达克学院( StuQ )明星讲师, Node.js 技术布道者。曾就职于去哪儿、新浪、网秦,做过前端、后端、数据分析,是一名全栈技术的实践者。 现在,越来越多的科技公司和开发者开始使用 Node.js 开发各种应用。Node.js除了能够辅助大前端开发外,还可以编写Web应用,封装Api,组装RPC服务等,甚至是开发VSCode编辑器一样的PC客户端。和其它技术相比, Node.js 简单易学,性能好、部署容易,能够轻松处理高并发场景下的大量服务器请求。Node.js 周边的生态也非常强大,NPM(Node包管理)上有超过60万个模块,日下载量超过3亿次。但编写 Node.js 代码对新人和其它语言背景的开发者来说,不是一件容易的事,在入门之前需要弄懂不少复杂的概念。 我身边也有很多人问我:如何学习 Node.js ?作为一名 Node.js 布道者,我做过很多 Node....
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS7编译安装Gcc9.2.0,解决mysql等软件编译问题
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- CentOS7,8上快速安装Gitea,搭建Git服务器
- CentOS8安装MyCat,轻松搞定数据库的读写分离、垂直分库、水平分库
- CentOS7编译安装Cmake3.16.3,解决mysql等软件编译问题
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- SpringBoot2全家桶,快速入门学习开发网站教程
- Jdk安装(Linux,MacOS,Windows),包含三大操作系统的最全安装
- MySQL8.0.19开启GTID主从同步CentOS8
- CentOS8编译安装MySQL8.0.19