AliOS Things 3.0应用笔记:http client简单应用
简介
AliOS Things 3.0版本于9月27日在云栖大会正式发布,在新版本中带来了全新的应用开发框架,帮助用户快速构建自己的应用。使用户可以更专注于自身应用的开发。
AliOS Things 3.0版本新增加了httpc组件(http 客户端组件),httpc组件支持多种RESTful的API调用,包括GET、POST、PUT、HEAD等,也支持https安全协议。
本示例是基于AliOS Things 3.0应用开发框架做的一个简单的http client应用,通过http API请求获取当前的天气信息。
准备工作
参考 AliOS Things Environment Setup 和 AliOS Things 3.0 应用开发指南指南 搭建好AliOS Things 3.0的应用开发环境。
创建应用工程
参考AliOS Things 3.0 应用开发指南 > AliOS Studio中创建应用工程创建好你的应用工程。
本示例新建的应用工程名称为httpclient_app
,选择的开发板为developerkit
。
编写应用代码
新建好的应用工程文件如下面所示:
.httpclient_app ├── .aos # AliOS Things 3.0 应用工程描述 ├── .vscode # AliOS Studio 配置文件 ├── Config.in # Menuconfig 配置文件 ├── README.md # 应用说明文档 ├── aos.mk # 编译文件 ├── app_main.c # 应用示例代码 └── k_app_config.h # 内核配置
添加http组件
aos-cube会自动根据include的头文件来自动添加组件。
http组件需要用到全局宏:BUILD_AOS
,所以需要在aos.mk
中额外增加一个全局宏定义:
GLOBAL_DEFINES += BUILD_AOS
天气API说明
本示例使用http组件,发送http get请求获取天气数据,天气的API是中国天气网提供的API:http://www.weather.com.cn/data/sk/101210101.html
,其中101210101
代表是杭州。
使用curl
命令可以测试该API接口:
$ curl http://www.weather.com.cn/data/sk/101210101.html {"weatherinfo":{"city":"杭州","cityid":"101210101","temp":"24.8","WD":"东北风","WS":"小于3级","SD":"81%","AP":"1000.3hPa","njd":"暂无实况","WSE":"<3","time":"17:50","sm":"2.1","isRadar":"1","Radar":"JC_RADAR_AZ9571_JB"}}%
或者也可以在浏览器打开该链接测试API接口。
使用http组件
本示例主要使用到了http如下接口,详细的http对外提供的接口和参数说明请参考include/network/http/http.h:
/* httpc 初始化 */ int8_t http_client_initialize(void); /* 创建一个httpc实例 */ httpc_handle_t httpc_init(httpc_connection_t *settings); /* 销毁一个httpc实例 */ int8_t httpc_deinit(httpc_handle_t httpc); /* 发送http请求 */ int8_t httpc_send_request(httpc_handle_t httpc, int8_t method, char *uri, const char *hdr, const char *content_type, const char *param, uint16_t param_len); /* 构建http请求header */ int32_t httpc_construct_header(char *buf, uint16_t buf_size, const char *name, const char *data); /* 等待接口http返回 */ int32_t httpc_recv_response(httpc_handle_t httpc, uint8_t *rsp, uint32_t rsp_size, http_rsp_info_t *info, uint32_t timeout);
完整源码
本示例应用的工程源码点击这里下载。
注意:需要更改app_main.c
中的WIFI_SSID
和 WIFI_PASSWD
为你的路由器信息。
aos.mk:
NAME := httpclient_app $(NAME)_MBINS_TYPE := app $(NAME)_VERSION := 1.0.0 $(NAME)_SUMMARY := httpclient_app $(NAME)_SOURCES += app_main.c GLOBAL_DEFINES += BUILD_AOS GLOBAL_INCLUDES += ./ $(NAME)_COMPONENTS_CUSTOMIZED := http yloop $(NAME)_COMPONENTS += $($(NAME)_COMPONENTS_CUSTOMIZED)
app_main.c:
/* * Copyright (C) 2015-2017 Alibaba Group Holding Limited */ #include <stdio.h> #include <aos/kernel.h> #include <aos/yloop.h> #include <http.h> #include <network/network.h> #define WIFI_SSID "aiot" #define WIFI_PASSWD "12345678" #define REQ_BUF_SIZE 2048 #define RSP_BUF_SIZE 4096 #define HTTP_UP_HDR_SIZE 128 /* weather api hostname */ #define WEATHER_HOSTNAME "http://www.weather.com.cn/" /* weather api uri */ #define WEATHER_URI "data/sk/101210101.html" static httpc_handle_t httpc_handle = 0; static httpc_connection_t settings; /* buffer for send & receive */ static uint8_t rsp_buf[RSP_BUF_SIZE] = {0}; static uint8_t req_buf[REQ_BUF_SIZE] = {0}; /* send http get request */ char *get_weather(void) { int fd; char hdr[HTTP_UP_HDR_SIZE] = {0}; int32_t ret; http_rsp_info_t rsp_info; http_client_initialize(); /* create socket */ if ((fd = socket(AF_INET, SOCK_STREAM, 0)) < 0) { printf("alloc socket fd fail\n"); goto exit; } memset(&settings, 0, sizeof(settings)); settings.socket = fd; settings.server_name = WEATHER_HOSTNAME; settings.req_buf = req_buf; settings.req_buf_size = REQ_BUF_SIZE; /* httpc initialization */ if ((httpc_handle = httpc_init(&settings)) == 0) { printf("http session init fail\n"); close(fd); goto exit; } /* construct httc header: set accept content type */ if ((httpc_construct_header( hdr, HTTP_UP_HDR_SIZE, "Accept", "text/xml,text/javascript,text/html,application/json")) < 0) { printf("http construct header fail\n"); goto exit; } /* send get request */ if ((httpc_send_request(httpc_handle, HTTP_GET, WEATHER_URI, hdr, "application/json", NULL, 0)) != HTTP_SUCCESS) { printf("httpc_send_request fail\n"); goto exit; } /* get response */ if ((httpc_recv_response(httpc_handle, rsp_buf, RSP_BUF_SIZE, &rsp_info, 10000)) < 0) { printf("httpc_recv_response fail\n"); goto exit; } printf("http session %x, buf size %d bytes, recv %d bytes data", httpc_handle, RSP_BUF_SIZE, rsp_info.rsp_len); // if (rsp_info.rsp_len > 0) { // printf("%s", rsp_buf); // } if (rsp_info.message_complete) { // printf("message_complete"); close(settings.socket); httpc_deinit(httpc_handle); return rsp_info.body_start; } exit: close(settings.socket); httpc_deinit(httpc_handle); return NULL; } /* task for get weather */ static void get_weather_task(void *arg) { char *weather_data = NULL; /* get weather data */ if((weather_data = get_weather()) != NULL){ aos_msleep(200); printf("********************** weather data **********************\r\n"); printf("%s\r\n", weather_data); printf("**********************************************************\r\n"); return; } printf("weather request error\r\n"); } /* wifi event */ static void wifi_service_event(input_event_t *event, void *priv_data) { static char ip[16] = {0}; static int get_weather_started = 0; if (event->type != EV_WIFI && event->code != CODE_WIFI_ON_GOT_IP) { return; } netmgr_wifi_get_ip(ip); /* start up only once */ if (get_weather_started == 1) { return; } /* check if ip is available */ if (0 == strcmp(ip, "0.0.0.0")) { printf("ip invailable\n"); return; } get_weather_started = 1; printf("wifi connected, ip:%s\n", ip); aos_task_new("get_weather", get_weather_task, NULL, 1024 * 4); } /* task for connect wifi */ static void wifi_connect(void *arg) { /* network init */ netmgr_init(); netmgr_start(false); aos_msleep(100); /* connect to wifi */ printf("\r\nConnecting to wifi: ssid:[%s], passwd:[%s]\r\n", WIFI_SSID, WIFI_PASSWD); netmgr_connect(WIFI_SSID, WIFI_PASSWD, 10 * 1000); } /**********************user code*************************/ int application_start(int argc, char *argv[]) { #ifdef WITH_SAL sal_add_dev(NULL, NULL); sal_init(); #endif /* register wifi event */ aos_register_event_filter(EV_WIFI, wifi_service_event, NULL); aos_task_new("wifi_connect", wifi_connect, NULL, 2048); /* loop for schedule */ aos_loop_run(); }
编译运行
点击编译和烧录。运行后,在串口日志最后面就能看的获取到的天气信息。
运行效果:
[ 0.050]<A>AOS sensor: drv_acc_st_lsm6dsl_init successfully [ 0.060]<A>AOS sensor: drv_gyro_st_lsm6dsl_init gyro do not need reset [ 0.070]<A>AOS sensor: drv_gyro_st_lsm6dsl_init successfully [ 0.080]<A>AOS sensor: drv_als_liteon_ltr553_init successfully [ 0.090]<A>AOS sensor: drv_ps_liteon_ltr553_init successfully [ 0.100]<A>AOS sensor: drv_baro_bosch_bmp280_init successfully [ 0.110]<A>AOS sensor: drv_mag_memsic_mmc3680kj_init successfully [ 0.120]<A>AOS sensor: drv_humi_sensirion_shtc1_init successfully [ 0.130]<A>AOS sensor: drv_temp_sensirion_shtc1_init successfully Welcome to AliOS Things [ 0.150]<E>sal_wifi Uart dev is not configured, use the default cfg uart 1 enter uart_receive_start_dma instance 0x40004800ip invailable Connecting to wifi: ssid:[aiot], passwd:[12345678] wifi connected, ip:192.168.43.111 [[hhttttppcc]][[000088665500]] hhttttppcc__sseenndd__rreeqquueesstt,, sseenndd rreeqquueesstt hheeaaddeerr vGoErTk s/pdaactea//gsikt/h1u0b1/2A1l0i1O0S1-.Thhtimnlg sH/TbToPa/r1d./1d 2Ulsoepre-rAkgietn/ta:o sA/lbioOaSr-dH_TcTlPi-.Ccl i2e1n6t 1 Cache-Control: no-cache Connection: close Host: www.weather.com.cn Accept: text/xml,text/javascript,text/html,application/json Content-Type: application/json socket 0, len 238 [httpc][009130] httpc_send_request, connect 0 [httpc][009160] httpc_send_request, send 238, ret 0 [httpc][019170] on_message_begin, HTTP response (headers), method GET [httpc][019170] on_status, HTTP response status OK [httpc][019180] print_header_field, len 4, Date [httpc][019190] print_header_field, len 29, Wed, 09 Oct 2019 07:32:13 GMT [httpc][019200] print_header_field, len 12, Content-Type [httpc][019200] print_header_field, len 9, text/html [httpc][019210] print_header_field, len 17, Transfer-Encoding [httpc][019220] print_header_field, len 7, chunked [httpc][019220] print_header_field, len 10, Connection [httpc][019230] print_header_field, len 5, close [httpc][019240] print_header_field, len 6, Server [httpc][019240] print_header_field, len 9, openresty [httpc][019250] print_header_field, len 16, X-Xss-Protection [httpc][019260] print_header_field, len 1, 1 [httpc][019260] print_header_field, len 10, Set-Cookie [httpc][019270] print_header_field, len 8, HttpOnly [httpc][019280] print_header_field, len 3, Age [httpc][019280] print_header_field, len 4, 2068 [httpc][019290] print_header_field, len 5, X-Via [httpc][019300] print_header_field, len 125, 1.1 PSbjsdBGPvu28:10 (Cdn Cache Server V2.0), 1.1 zhdx147:10 (Cdn Cache Server V2.0), 1.1 huadxin69:7 (Cdn Cache Server V2.0) [httpc][019320] on_headers_complete, headers complete [httpc][019320] on_message_complete, HTTP GET response (complete) http session 20002298, buf size 4096 bytes, recv 578 bytes data[ 19.340]<E>sal_wifi HAL_SAL_Close 778 failed ********************** weather data ********************** {"weatherinfo":{"city":"杭州","cityid":"101210101","temp":"24.8","WD":"东北风","WS":"小于3级","SD":"81%","AP":"1000.3hPa","njd":"暂无实况","WSE":"<3","time":"17:50","sm":"2.1","isRadar":"1","Radar":"JC_RADAR_AZ9571_JB"}} **********************************************************
参考文档
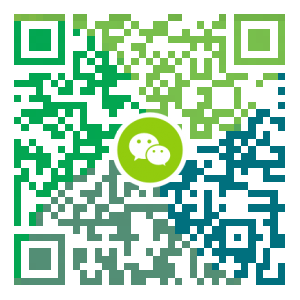
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
大家久等了,改造版阿里巴巴 sentinel 控制台终于开源了
前言 最近几天,好几个小伙伴在后台询问,改造后的 sentinel-dashboard 什么时候开源。讲真,不是不想给大家放出来,是因为一些地方还没有完善好,怕误导了大家,在经过了一个星期业余时间的努力,终于把基础版本搞定了。小伙伴们终于可以进行拉取测试了。 历程 首先回顾一下改造之路: SpringBoot 2.0 + Sentinel 动态限流实战 SpringBoot 2.0 + Nacos + Sentinel 流控规则集中存储 SpringBoot 2.0 + InfluxDB+ Sentinel 实时监控数据存储 阿里巴巴 Sentinel + InfluxDB + Chronograf 实现监控大屏 最终架构 持续学习 sentinel 的学习已经告一段落,后面会持续学InfluxDB,它是目前比较流行的时间序列数据库。 那么什么是时间序列数据库?最简单的定义就是数据格式里包含Timestamp字段的数据,比如某一时间环境的温度、湿度,以及机器的CPU的使用率等等。 随着物联网的发展,作为一名程序员,时序数据库是必不可少的一项必备技能。所以,在开源 sentinel-da...
- 下一篇
云栖大会:企业如何走向双中台的黄金时代?
2019年云栖大会在前些天落下帷幕,历时三天的云栖大会为我们带来了数据、智能、云计算、物联网等领域的精彩分享。 这一次的云栖大会迎来了作为阿里巴巴集团董事局主席、首席执行官的张勇,同时也带来了双中台解决方案。这是继7月阿里云上海峰会之后,阿里云双中台解决方案的又一次隆重亮相。 业务中台+数据中台 利用业务中台,企业将需要复用的业务模块沉淀为能力中心,企业的创新业务部门可利用能力中心,像搭积木一样快速搭建起来各种业务系统;而“数据中台”则打通了不同业务部门之间的烟囱式IT架构,对全域数据进行融合处理,实现了“一切业务数据化”的目标,为企业带来持续的高效创新。 双中台可搭配新零售、会员营销、采购等业务 阿里云双中台解决方案致力于推动企业的数字化转型,面对企业不同的诉求,应对不同的业务场景,可以衍生出双中台全渠道运营解决方案、双中台会员营销解决方
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS关闭SELinux安全模块
- SpringBoot2更换Tomcat为Jetty,小型站点的福音
- CentOS6,CentOS7官方镜像安装Oracle11G
- SpringBoot2编写第一个Controller,响应你的http请求并返回结果
- Red5直播服务器,属于Java语言的直播服务器
- CentOS7编译安装Cmake3.16.3,解决mysql等软件编译问题
- SpringBoot2整合MyBatis,连接MySql数据库做增删改查操作
- SpringBoot2整合Thymeleaf,官方推荐html解决方案
- CentOS8安装Docker,最新的服务器搭配容器使用
- CentOS7,CentOS8安装Elasticsearch6.8.6