java B2B2C Springcloud电子商城系统-断路器(Hystrix)
原理
在微服务架构中,服务之间形成调用链路,链路中的任何一个服务提供者都可能面临着相应超时、宕机等不可用的情况,在高并发的情况下,这种情况会随着并发量的上升恶化,形成“雪崩效应”,而断路器hystrix正是用来解决这一个问题的组件。
断路器基本原理为:
正常情况下,断路器关闭,服务消费者正常请求微服务
一段事件内,失败率达到一定阈值(比如50%失败,或者失败了50次),断路器将断开,此时不再请求服务提供者,而是只是快速失败的方法(断路方法)
断路器打开一段时间,自动进入“半开”状态,此时,断路器可允许一个请求方法服务提供者,如果请求调用成功,则关闭断路器,否则继续保持断路器打开状态。
断路器hystrix是保证了局部发生的错误,不会扩展到整个系统,从而保证系统的即使出现局部问题也不会造成系统雪崩。
配置/使用
下面讲解在restTemplate和feign中断路器的配置和使用步骤
restTemplate+ribbon整合Hystrix
引入hystrix依赖
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-hystrix</artifactId> </dependency>
虽然Eureka依赖了ribbon,ribbon依赖了hystrix-core,但还是要引用了上面的maven依赖,因为下面用到的@HystrixCommand注解用到了hystrix-javanica包
启动类加上@EnableCircuitBreaker注解(@EnableHystrix也可以)
修改HelloWorldController的入口请求方法
@GetMapping("/message") @HystrixCommand(fallbackMethod = "getMessageFallback") public HelloworldMessage getMessage() { HelloMessage hello = getMessageFromHelloService(); WorldMessage world = getMessageFromWorldService(); HelloworldMessage helloworld = new HelloworldMessage(); helloworld.setHello(hello); helloworld.setWord(world); log.debug("Result helloworld message:{}", helloworld); return helloworld; } /** * 断路方法 * @return */ public HelloworldMessage getMessageFallback(){ HelloMessage helloMessage=new HelloMessage(); helloMessage.setName("hello"); helloMessage.setMessage("error occurs"); WorldMessage worldMessage=new WorldMessage(); worldMessage.setMessage("world error occurs"); HelloworldMessage helloworldMessage=new HelloworldMessage(); helloworldMessage.setHello(helloMessage); helloworldMessage.setWord(worldMessage); return helloworldMessage; }
通过@HystrixCommand注解的fallbackMethod指向断路方法,该方法会在调用hello服务或者world服务失败时被调用。
@HystrixCommand 注解还可以配置超时事件等其他属性。
测试
1)依次启动eureka server:discovery/trace/hello/world/helloword项目
2)在浏览器输入地址http:\localhost:8020/message,则返回正确的结果
3)停止hello项目,再次输入上述地址,则执行断路器中的方法。
feign下整合Hystrix
feign禁用Hystrix
在Spring Cloud中,只要Hystrix在项目的classpath中,Feign就会用断路器包裹Feign客户端的所有方法,如果要禁用Hystrix则可以通过自定义feign的配置来解决。
@Configuration public class FeignConfiguration{ @Bean @Scope("prototype") public Feign Builder feignBuilder(){ return Feign.builder(); } }
要禁用Hystrix的接口引用该配置即可
@FeignClient(name="hello",configuration=FeignConfiguration.class) public interface HelloService{ ...... }
feign使用Hystrix
启用Hystrix
默认情况下feign已经整合了Hystrix,在配置文件中开启即可(本人用的的Dalston.SR2版本的Spring Cloud,需要在配置文件开启)
feign: hystrix: enabled: true
接口指定回退类
在HelloService中修改FeignClient类,指定fallback的类
package com.example.helloworldfeign.service; import com.example.helloworldfeign.model.HelloMessage; import org.springframework.cloud.netflix.feign.FeignClient; import org.springframework.web.bind.annotation.GetMapping; /** * @author billjiang 475572229@qq.com * @create 17-8-23 */ @FeignClient(value="hello",fallback = HelloServiceFallback.class) public interface HelloService { @GetMapping("/message") HelloMessage hello(); }
实现了接口fallback的类的实现:
package com.example.helloworldfeign.service; import com.example.helloworldfeign.model.HelloMessage; import org.springframework.stereotype.Component; /** * @author billjiang 475572229@qq.com * @create 17-8-28 */ @Component public class HelloServiceFallback implements HelloService { @Override public HelloMessage hello() { HelloMessage helloMessage=new HelloMessage(); helloMessage.setName("hello"); helloMessage.setMessage("error occurs"); return helloMessage; } }
world项目同上
测试
1)依次启动eureka server:discovery/trace/hello/world/helloword-feing项目
2)在浏览器输入地址http:\localhost:8030/message,则返回正确的结果
3)停止hello项目,再次输入上述地址,则执行断路器中的方法。
查看断路器错误日志
如果要查看详细的断路器的日志,可以通过注解@FeignClient的fallbackFactory来实现,如下代码所示:
import com.example.helloworldfeign.model.HelloMessage; import org.springframework.cloud.netflix.feign.FeignClient; import org.springframework.web.bind.annotation.GetMapping; /** * @author billjiang 475572229@qq.com * @create 17-8-23 */ @FeignClient(value="hello",fallbackFactory = HelloServiceFallbackFactory.class) public interface HelloService { @GetMapping("/message") HelloMessage hello(); } HelloServiceFallbackFactory类: package com.example.helloworldfeign.service; import com.example.helloworldfeign.model.HelloMessage; import feign.hystrix.FallbackFactory; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.stereotype.Component; /** * @author billjiang 475572229@qq.com * @create 17-8-28 */ @Component public class HelloServiceFallbackFactory implements FallbackFactory<HelloService> { private final static Logger LOGGER= LoggerFactory.getLogger(HelloServiceFallbackFactory.class); @Override public HelloService create(Throwable throwable) { return new HelloService() { @Override public HelloMessage hello() { //print the error LOGGER.error("fallback ,the result is:",throwable); HelloMessage helloMessage=new HelloMessage(); helloMessage.setName("hello"); helloMessage.setMessage("error occurs"); return helloMessage; } }; //需要JAVA Spring Cloud大型企业分布式微服务云构建的B2B2C电子商务平台源码 一零三八七七四六二六 } }
这样会在控制台把具体导致熔断的信息输出,以便跟踪错误。
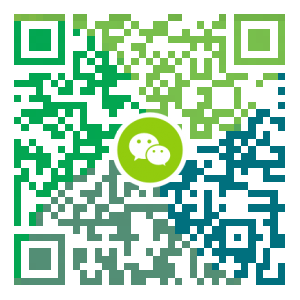
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
陈思淼:阿里6个月重写Lazada,再造“淘宝”的技术总结
2018 年 3 月,一则消息在朋友圈刷屏:阿里巴巴元老彭蕾卸任蚂蚁金服董事长,转而担任东南亚电商网站 Lazada 的 CEO,一时之间舆论纷纷。而在此之前,早在 2017 年 9 月,阿里集团管理层决定,启动 Voyager 项目,开始对 Lazada 的全系统改造,并且要在 2018 年 3 月底之前上线。时间如此紧急,在 6 个月内重造“淘宝”可能吗? 第一次改造 Lazada 的尝试 Lazada 在 2012 年创立于新加坡,业务很快扩展到马来西亚,越南,印度尼西亚,菲律宾,泰国,新加坡六国,阿里巴巴在 2016 年第一次投资 10 亿美元,2017 年第二次追加投资,完成对 Lazada 的系列收购。 在阿里接手之前,Lazada 一直是东南亚六国第一大电商,但是同时也面对非常激烈的市场竞争。包括京东、腾讯都把目光投向了这里,而亚马逊也试图在东南亚扩张。 从业务模式来看,过去 Lazada 更偏自营,更像亚马逊,是一家零售公司而不是互联网公司。Lazada 自建和仓储,同时提供 FBL(Fulfilement By Lazada)服务给商家。Lazada CTO、Voya...
- 下一篇
java B2B2C Springboot电子商城系统-路由网关(zuul)
一、Zuul简介 Zuul的主要功能是路由转发和过滤器。路由功能是微服务的一部分,比如/api/user转发到到user服务,/api/shop转发到到shop服务。zuul默认和Ribbon结合实现了负载均衡的功能。 zuul有以下功能: Authentication Insights Stress Testing Canary Testing Dynamic Routing Service Migration Load Shedding Security Static Response handling Active/Active traffic management 二、创建service-zuul工程 pom.xml文件如下 <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http:/...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- CentOS7设置SWAP分区,小内存服务器的救世主
- Hadoop3单机部署,实现最简伪集群
- SpringBoot2更换Tomcat为Jetty,小型站点的福音
- Windows10,CentOS7,CentOS8安装Nodejs环境
- CentOS7编译安装Cmake3.16.3,解决mysql等软件编译问题
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- 设置Eclipse缩进为4个空格,增强代码规范
- SpringBoot2全家桶,快速入门学习开发网站教程
- CentOS关闭SELinux安全模块