Springboot+ajax传输json数组以及单条数据的方法
Springboot+ajax传输json数组以及单条数据的方法
下面是用ajax传输到后台单条以及多条数据的解析的Demo:
结构图如下:
下面是相关的代码:
pom.xml:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>springboot-ssm</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>springboot-ssm</name> <description>Demo project for Spring Boot</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.9.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> <!--以下两项需要如果不配置,解析themleaft 会有问题--> <thymeleaf.version>3.0.2.RELEASE</thymeleaf.version> <thymeleaf-layout-dialect.version>2.0.5</thymeleaf-layout-dialect.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <!--mybatis与mysql--> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>1.2.0</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> <!--druid依赖--> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid</artifactId> <version>1.0.25</version> </dependency> <!--添加static和templates的依赖--> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <!--解析json--> <dependency> <groupId>net.sf.json-lib</groupId> <artifactId>json-lib</artifactId> <version>2.4</version> <classifier>jdk15</classifier> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
StudentController:
package com.home.controller; import com.home.entity.Student; import com.home.service.StudentService; import net.sf.json.JSONArray; import net.sf.json.JSONObject; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.bind.annotation.ResponseBody; import java.util.List; @Controller public class StudentController { @Autowired private StudentService studentService; @RequestMapping("/list") @ResponseBody public List<Student> list() { List<Student> studentList = studentService.getAllStu(); return studentList; } @RequestMapping("/tableList") public String tableList(Model model) { List<Student> studentList = studentService.getAllStu(); model.addAttribute("studentList", studentList); return "jsonArray"; } @RequestMapping("/goToAdd") public String goToAdd() { return "add"; } @RequestMapping("/add") public String add(Student stu) { studentService.insert(stu); return "result"; } @RequestMapping("/goToAddJson") public String goToAddJson() { return "json"; } @RequestMapping("/jsonArrayAdd") @ResponseBody public String jsonaAdd(@RequestParam("ids") String ids) { System.out.println(ids); JSONArray jsonArray = JSONArray.fromObject(ids); for (int i = 0; i < jsonArray.size(); i++) { JSONObject jsonObject = jsonArray.getJSONObject(i); System.out.println("json数组传递过来的参数为:" + "第" + i + "条:" + "\n" + jsonObject.get("id")); } return "json数组添加成功了"; } //json数组传递 @RequestMapping("/jsonAdd") @ResponseBody public String jsonArrayAdd(@RequestParam("ids") String ids) { JSONObject jsonObject = JSONObject.fromObject(ids); System.out.println("jsonObject==>" + jsonObject); Student stu = (Student) JSONObject.toBean(jsonObject, Student.class); System.out.println("stu==>" + stu); return "成功了!"; } }
Student:
package com.home.entity; import java.io.Serializable; public class Student implements Serializable { private Integer id; private String name; private Integer age; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } @Override public String toString() { return "Student{" + "id=" + id + ", name='" + name + '\'' + ", age=" + age + '}'; } }
StudentMapper:
package com.home.mapper; import com.home.entity.Student; import org.apache.ibatis.annotations.Mapper; import java.util.List; @Mapper public interface StudentMapper { List<Student> getAllStu(); int deleteByPrimaryKey(Integer id); int insert(Student record); int insertSelective(Student record); Student selectByPrimaryKey(Integer id); int updateByPrimaryKeySelective(Student record); int updateByPrimaryKey(Student record); }
StudentService:
package com.home.service; import com.home.entity.Student; import java.util.List; public interface StudentService { List<Student> getAllStu(); void insert(Student stu); }
StudentServiceImpl:
package com.home.service.impl; import com.home.entity.Student; import com.home.mapper.StudentMapper; import com.home.service.StudentService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import javax.annotation.Resource; import java.util.List; @Service public class StudentServiceImpl implements StudentService { @Resource private StudentMapper studentMapper; @Override public List<Student> getAllStu() { return studentMapper.getAllStu(); } @Override public void insert(Student stu) { studentMapper.insert(stu); } }
StudentMapper.xml:
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" > <mapper namespace="com.home.mapper.StudentMapper" > <resultMap id="BaseResultMap" type="com.home.entity.Student" > <id column="id" property="id" jdbcType="INTEGER" /> <result column="name" property="name" jdbcType="VARCHAR" /> <result column="age" property="age" jdbcType="INTEGER" /> </resultMap> <sql id="Base_Column_List" > id, name, age </sql> <select id="selectByPrimaryKey" resultMap="BaseResultMap" parameterType="java.lang.Integer" > select <include refid="Base_Column_List" /> from student where id = #{id,jdbcType=INTEGER} </select> <delete id="deleteByPrimaryKey" parameterType="java.lang.Integer" > delete from student where id = #{id,jdbcType=INTEGER} </delete> <insert id="insert" parameterType="com.home.entity.Student" > insert into student (id, name, age ) values (#{id,jdbcType=INTEGER}, #{name,jdbcType=VARCHAR}, #{age,jdbcType=INTEGER} ) </insert> <insert id="insertSelective" parameterType="com.home.entity.Student" > insert into student <trim prefix="(" suffix=")" suffixOverrides="," > <if test="id != null" > id, </if> <if test="name != null" > name, </if> <if test="age != null" > age, </if> </trim> <trim prefix="values (" suffix=")" suffixOverrides="," > <if test="id != null" > #{id,jdbcType=INTEGER}, </if> <if test="name != null" > #{name,jdbcType=VARCHAR}, </if> <if test="age != null" > #{age,jdbcType=INTEGER}, </if> </trim> </insert> <update id="updateByPrimaryKeySelective" parameterType="com.home.entity.Student" > update student <set > <if test="name != null" > name = #{name,jdbcType=VARCHAR}, </if> <if test="age != null" > age = #{age,jdbcType=INTEGER}, </if> </set> where id = #{id,jdbcType=INTEGER} </update> <update id="updateByPrimaryKey" parameterType="com.home.entity.Student" > update student set name = #{name,jdbcType=VARCHAR}, age = #{age,jdbcType=INTEGER} where id = #{id,jdbcType=INTEGER} </update> <select id="getAllStu" resultType="com.home.entity.Student"> select * from student </select> </mapper>
SqlMapperConfig.xml:
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <!-- <settings> 开启二级缓存 <setting name="cacheEnabled" value="true" /> </settings> --> <!-- 只需要定义个别名,这个应该有 --> <!-- springboot集成pageHelper(Java配置未注入的时候可以用下面配置) --> <!-- <plugins> com.github.pagehelper为PageHelper类所在包名 <plugin interceptor="com.github.pagehelper.PageInterceptor"> 使用下面的方式配置参数,后面会有所有的参数介绍 <property name="helperDialect" value="mysql" /> </plugin> </plugins> --> </configuration>
json.html:
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>Title</title> <!--<script src="${pageContxt.request.contextPath}/jquery-3.3.1/jquery-3.3.1.min.js"></script>--> <script type="text/javascript" th:src="@{../static/jquery-3.3.1/jquery-3.3.1.min.js}"></script> </head> <body> <p>这个页面使用的是json的传输:</p> <form th:action="@{/} " th:method="post"> id:<input id="id" type="text" name="id"><br/> name:<input id="name" type="text" name="name"><br/> age:<input id="age" type="text" name="age"><br/> </form> <button onclick="tijiao()">提交json</button> <script> function tijiao() { var id = $("#id").val().trim(); var name = $("#name").val().trim(); var age = $("#age").val().trim(); console.log("id:" + id + " name:" + name + " age:" + age); var stu = { id:id,name:name,age:age } $.ajax({ type:"post", url:"/jsonAdd", datatype:"json", data:{ids:JSON.stringify(stu)}, success:function(data){ alert(data) ; } }); } </script> </body> </html>
jsonArray.html:
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>Title</title> <script type="text/javascript" th:src="@{../static/jquery-3.3.1/jquery-3.3.1.min.js}"></script> </head> <body> <table border="1" id="stuTable"> <tr> <td>是否选中</td> <td>编号</td> <td>姓名</td> <td>年龄</td> </tr> <tr th:each="stu:${studentList}"> <td><input th:type="checkbox" th:name="id" th:value="${stu.id}"></td> <td th:text="${stu.id}">编号</td> <td th:text="${stu.name}">姓名</td> <td th:text="${stu.age}">年龄</td> </tr> </table> <button id="pltj">批量提交</button> <script> $("#pltj").click( function () { var checkbox = $("#stuTable").find(":checked"); console.log(checkbox); if (checkbox == 0) { return; } else { var str = []; for (var i = 0; i < checkbox.length; i++) { var ck = checkbox[i]; var a = $(ck).val().trim(); str.push({id: a}); console.log("str==>" + str); } $.ajax({ url: "/jsonArrayAdd", datatype: "json", data: {ids: JSON.stringify(str)}, success: function (data) { alert(data); } }); } }); </script> </body> </html>
application.properties:
#server.port=80 logging.level.org.springframework=DEBUG #springboot mybatis #jiazai mybatis peizhiwenjian mybatis.mapper-locations = classpath:mapper/*Mapper.xml mybatis.config-location = classpath:mybatis/sqlMapperConfig.xml #mybatis.type-aliases-package = com.demo.bean #shujuyuan spring.datasource.driver-class-name= com.mysql.jdbc.Driver spring.datasource.url = jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8 spring.datasource.username = ******* spring.datasource.password = ******* spring.thymeleaf.prefix=classpath:/templates/
运行后选择两项,可以得到选中的那一行的id值,进行批量操作了:
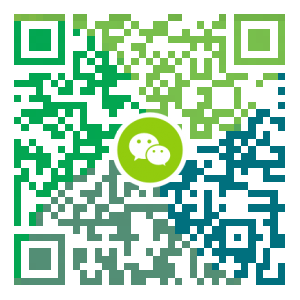
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
java8新特性(四)_Stream详解
之前写过一篇用stream处理map的文章,但是对stream没有一个整体的认识,这次结合并发编程网和ibm中介绍stream的文章进行一个总结,我会着重写对list的处理,毕竟实际工作中大家每天进行使用 Stream简单介绍 定义 A sequence of elements supporting sequential and parallel aggregate operations. 支持顺序并行聚合操作的元素序列 看看大神们怎么解读的 大家可以把Stream当成一个高级版本的Iterator。原始版本的Iterator,用户只能一个一个的遍历元素并对其执行某些操作;高级版本的Stream,用户只要给出需要对其包含的元素执行什么操作,比如“过滤掉长度大于10的字符串”、“获取每个字符串的首字母”等,具体这些操作如何应用到每个元素上,就给Stream就好了 简单demo 写一个过滤null的简单功能 public static void main(String[] args) { List arrys = Arrays.asList(1, null, 3, 4); arrys.fo...
- 下一篇
Java对MongoDB的ObjectId的序列化问题
MongoDB在不特殊指认的情况下,默认的集合主键是“_id”,类型是ObjectId。ObjectId是一个12字节的BSON类型字符串,包含了UNIX时间戳,机器识别码,进程号,计数值信息。机器码用来防止分布式系统生成id时冲突的问题,保证每台机器生成的识别码不同,进程号保证多线程情况下生成的id不同。 ObjectId在java程序中是对象类型,JavaBean中常这样使用: @Document(collection = "c_userinfo") public class UserInfo{ @Id private ObjectId id; private String name; // getter setter略 } 此时,如果直接实体类序列化为json,id将被作为对象处理,前段无法将此对象转为字符串,也无法将此id作为唯一标识调用其他数据。 { id: { "time": 1494233455000, "timestamp": 1494233455, "date": 1494233455000, "new": false, "timeSecond": 149423345...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Windows10,CentOS7,CentOS8安装MongoDB4.0.16
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- Docker安装Oracle12C,快速搭建Oracle学习环境
- 设置Eclipse缩进为4个空格,增强代码规范
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- SpringBoot2全家桶,快速入门学习开发网站教程
- CentOS关闭SELinux安全模块
- CentOS7设置SWAP分区,小内存服务器的救世主
- CentOS7编译安装Gcc9.2.0,解决mysql等软件编译问题
- Linux系统CentOS6、CentOS7手动修改IP地址