Java对象的深度拷贝实现
1. 说明
Java对象复制可分为浅拷贝(shallow copy)和深度拷贝(deep copy)两种。浅拷贝指从源对象中将值复制出来,因此产生的拷贝对象与源对象并不是独立的。如源对象存在引用属性(reference),此时的拷贝对象和源对象的相同引用属性都指向同一个对象,修改引用属性对象的内容,对于拷贝对象和源对象都可见。深度拷贝指将源对象的对象图中所有对象都被拷贝一次,因此产生的拷贝对象与源对象是独立的。即引用属性的拷贝,也是将引用指向的对象拷贝一份。源对象的对象图中任何属性的改变,并不会影响拷贝对象的属性。
理解上面的含义后,我们下面将比较两种拷贝方式,并讨论4种深度拷贝的实现。
2. 准备
2.1 依赖
我们将使用Maven来管理项目依赖,需要引入Gson,JackSon,Apache Commons Lang。
<dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.8.5</version> </dependency> <dependency> <groupId>commons-lang</groupId> <artifactId>commons-lang</artifactId> <version>2.6</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.9.6</version> </dependency>
最新的Gson、JackSon、Apache Commons Lang的版本可以在Maven Central仓库查看。
2.2 模型
我们需要引入2个对象类,来比较两种拷贝方式。
class Address { private String street; private String city; private String country; // standard constructors, getters and setters }
class User { private String name; private Address address; // standard constructors, getters and setters }
3. 浅拷贝
浅拷贝仅从源对象中复制属性的值,如果是引用属性,也只是复制引用的地址值。
import static org.hamcrest.core.IsNot.not; import static org.hamcrest.core.IsSame.sameInstance; import static org.junit.Assert.assertThat; import org.junit.Test; @Test public void shouldNotBeSame_WhenShallowCopy() { Address address = new Address("西湖区丰潭路380号", "杭州", "中国"); User origin = new User("嗨皮的孩子", address); User shallowCopy = new User(origin.getName(), origin.getAddress()); assertThat(shallowCopy, not(sameInstance(origin))); }
在这种情况下origin != shallowCopy,也就是说前拷贝的对象与源对象是不同的。
import static org.hamcrest.core.IsEqual.equalTo; import static org.hamcrest.core.IsNot.not; import static org.junit.Assert.assertThat; import org.junit.Test; @Test public void shouldCopyChange_whenModifyingOriginalObject() { Address address = new Address("西湖区丰潭路380号", "杭州", "中国"); User origin = new User("嗨皮的孩子", address); User shallowCopy = new User(origin.getName(), origin.getAddress()); address.setStreet("西湖区丰潭路381号"); assertThat(shallowCopy.getAddress().getStreet(),equalTo(origin.getAddress().getStreet())); }
在上面的测试中,我们改变源对象的地址属性中的street的值时,拷贝对象的地址属性中的street属性也跟着变化。
4. 深度拷贝
如果我们希望地址对象是不可变(immutable),我们要解决的方法是要在对象树中递归的拷贝每一个可变的对象。下面将讨论不同的方式来实现深度拷贝。
4.1 构造函数
基于复制构造函数实现深度拷贝。
public Address(Address that) { this(that.getStreet(), that.getCity(), that.getCountry()); } public User(User that) { this(that.getName(), new Address(that.getAddress())); }
import static org.junit.Assert.assertNotEquals; import org.junit.Test; @Test public void shouldNotChange_WhenModifyingOriginalObject() { Address address = new Address("西湖区丰潭路380号", "杭州", "中国"); User origin = new User("嗨皮的孩子", address); User shallowCopy = new User(origin); address.setStreet("西湖区丰潭路381号"); assertNotEquals(shallowCopy.getAddress().getStreet(), origin.getAddress().getStreet()); }
基于构造函数实现的深度对象拷贝方法解决“拷贝对象”和“源对象”独立性问题。上述中,我们没有新建String对象,原因String是不可变对象类。
4.2 Cloneable 接口
基于Object#clone方法实现对象复制,但是Object#clone是protected方法,在覆写时,需要改成public修饰。我们需要在类的定义中实现Cloneable接口,标记对象是可以clone。
覆写Address类的clone方法
class Address implements Cloneable { private String street; private String city; private String country; // standard constructors, getters and setters ... ... @Override public Object clone(){ try { return super.clone(); } catch (CloneNotSupportedException e) { return new Address(this.street, this.getCity(), this.getCountry()); } } }
覆写User类的clone方法
class User implements Cloneable { private String name; private Address address; //standard constructors, getters and setters ... ... @Override public Object clone() { User user; try { user = (User) super.clone(); } catch (CloneNotSupportedException e) { user = new User(this.getName(), this.getAddress()); } user.address = (Address) this.address.clone(); return user; } }
import static org.hamcrest.core.IsEqual.equalTo; import static org.hamcrest.core.IsNot.not; import static org.junit.Assert.assertThat; import org.junit.Test; @Test public void shouldNotChange_WhenModifyingOriginalObjectUsingCloneCopy() { Address address = new Address("西湖区丰潭路380号", "杭州", "中国"); User origin = new User("嗨皮的孩子", address); User shallowCopy = (User) origin.clone(); address.setStreet("西湖区丰潭路381号"); assertThat(shallowCopy.getAddress().getStreet(), not(equalTo(origin.getAddress().getStreet()))); }
super.clone方法实现的是浅拷贝,因此返回的为浅拷贝对象。我们需要对于可变对象的属性,重新设置新的值。
4.3 借助第三方库
前面提到2种深度拷贝方式很直观。但是当你不能添加额外的构造函数或者复写clone方法时,就需要借助第三方库实现深度拷贝。
核心的思想是“我们先系列化对象,然后再反系列化为新的对象”。
4.3.1 Apache Commons Lang
Apache Commons Lang有SerializationUtils#clone方法,使用该方法可以深度复制出对象图中所有实现Serializable接口对象。此方法要求对象图中的类都实现Serializable接口,否则会抛出SerializationException。
class Address implements Serializable, Cloneable { private String street; private String city; private String country; // standard constructors, getters and setters ... ... @Override public Object clone() { try { return super.clone(); } catch (CloneNotSupportedException e) { return new Address(this.street, this.getCity(), this.getCountry()); } } }
class User implements Serializable, Cloneable { private String name; private Address address; //standard constructors, getters and setters ... ... @Override public Object clone() { User user; try { user = (User) super.clone(); } catch (CloneNotSupportedException e) { user = new User(this.getName(), this.getAddress()); } user.address = (Address) this.address.clone(); return user; } }
import static org.hamcrest.core.IsEqual.equalTo; import static org.hamcrest.core.IsNot.not; import static org.junit.Assert.assertThat; import org.apache.commons.lang.SerializationUtils; import org.junit.Test; @Test public void shouldNotChange_WhenModifyingOriginalObjectUsingCommonsClone() { Address address = new Address("西湖区丰潭路380号", "杭州", "中国"); User origin = new User("嗨皮的孩子", address); User shallowCopy = (User) SerializationUtils.clone(origin); address.setStreet("西湖区丰潭路381号"); assertThat(shallowCopy.getAddress().getStreet(), not(equalTo(origin.getAddress().getStreet()))); }
4.3.2 使用Gson的JSON系列化
使用Json系列化方式,也可以的达到深度复制,Gson库可以用来将对象转为JSON,或从JSON转为对象。Gson不要求类实现Serializable接口。
class Address implements Serializable, Cloneable { private String street; private String city; private String country; public Address(String street, String city, String country) { this.street = street; this.city = city; this.country = country; } public Address(Address that) { this(that.getStreet(), that.getCity(), that.getCountry()); } @Override public Object clone(){ try { return super.clone(); } catch (CloneNotSupportedException e) { return new Address(this.street, this.getCity(), this.getCountry()); } } ... ... //getters and setters } class User implements Serializable, Cloneable { private String name; private Address address; public User(String name, Address address) { this.name = name; this.address = address; } public User(User that) { this(that.getName(), new Address(that.getAddress())); } @Override public Object clone() { User user; try { user = (User) super.clone(); } catch (CloneNotSupportedException e) { user = new User(this.getName(), this.getAddress()); } user.address = (Address) this.address.clone(); return user; } ... ... //getters and setters }
import static org.hamcrest.core.IsEqual.equalTo; import static org.hamcrest.core.IsNot.not; import static org.junit.Assert.assertThat; import com.google.gson.Gson; import org.junit.Test; @Test public void shouldNotChange_WhenModifyingOriginalObjectUsingGsonClone() { Address address = new Address("西湖区丰潭路380号", "杭州", "中国"); User origin = new User("嗨皮的孩子", address); Gson gson = new Gson(); User shallowCopy = gson.fromJson(gson.toJson(origin), User.class); address.setStreet("西湖区丰潭路381号"); assertThat(shallowCopy.getAddress().getStreet(), not(equalTo(origin.getAddress().getStreet()))); }
4.3.3 使用Jackson的JSON系列化
Jackson是另一个JSON系列化库,实现上类似于Gson,但是我们需要给类提供默认构造函数。
class Address implements Serializable, Cloneable { private String street; private String city; private String country; public Address() {} public Address(String street, String city, String country) { this.street = street; this.city = city; this.country = country; } public Address(Address that) { this(that.getStreet(), that.getCity(), that.getCountry()); } @Override public Object clone(){ try { return super.clone(); } catch (CloneNotSupportedException e) { return new Address(this.street, this.getCity(), this.getCountry()); } } ... ... //getters and setters } class User implements Serializable, Cloneable { private String name; private Address address; public User() {} public User(String name, Address address) { this.name = name; this.address = address; } public User(User that) { this(that.getName(), new Address(that.getAddress())); } @Override public Object clone() { User user; try { user = (User) super.clone(); } catch (CloneNotSupportedException e) { user = new User(this.getName(), this.getAddress()); } user.address = (Address) this.address.clone(); return user; } ... ... //getters and setters }
import static org.hamcrest.core.IsEqual.equalTo; import static org.hamcrest.core.IsNot.not; import static org.junit.Assert.assertThat; import com.fasterxml.jackson.databind.ObjectMapper; import org.junit.Test; import java.io.IOException; @Test public void shouldNotChange_WhenModifyingOriginalObjectUsingJacksonClone() throws IOException { Address address = new Address("西湖区丰潭路380号", "杭州", "中国"); User origin = new User("嗨皮的孩子", address); ObjectMapper objectMapper = new ObjectMapper(); User shallowCopy = objectMapper.readValue(objectMapper.writeValueAsString(origin), User.class); address.setStreet("西湖区丰潭路381号"); assertThat(shallowCopy.getAddress().getStreet(), not(equalTo(origin.getAddress().getStreet()))); }
5. 总结
当我们需要深度复制对象时,该选择哪一种实现方式呢?依赖于我们需要复制的类,并且是否我们在对象图中拥有这些类。本文来自于java-deep-copy的翻译。
[1] : http://search.maven.org/#search%7Cgav%7C1%7Cg%3A%22com.google.code.gson%22%20AND%20a%3A%22gson%22 "Gson"
[2] : http://search.maven.org/#search%7Cgav%7C1%7Cg%3A%22com.fasterxml.jackson.core%22%20AND%20a%3A%22jackson-databind%22 "JackSon"
[3] : http://search.maven.org/#search%7Cgav%7C1%7Cg%3A%22commons-lang%22%20AND%20a%3A%22commons-lang%22 "Apache Commons Lang"
[4] : http://www.baeldung.com/java-deep-copy "java-deep-copy"
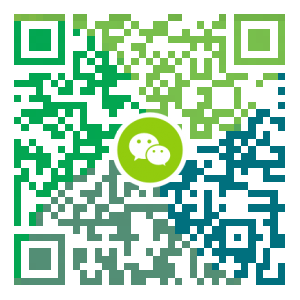
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
[剑指offer] 数组中出现次数超过一半的数字
本文首发于我的个人博客:尾尾部落 题目描述 数组中有一个数字出现的次数超过数组长度的一半,请找出这个数字。例如输入一个长度为9的数组{1,2,3,2,2,2,5,4,2}。由于数字2在数组中出现了5次,超过数组长度的一半,因此输出2。如果不存在则输出0。 解题思路 三种解法: 法1:借助hashmap存储数组中每个数出现的次数,最后看是否有数字出现次数超过数组长度的一半; 法2:排序。数组排序后,如果某个数字出现次数超过数组的长度的一半,则一定会数组中间的位置。所以我们取出排序后中间位置的数,统计一下它的出现次数是否大于数组长度的一半; 法3:某个数字出现的次数大于数组长度的一半,意思就是它出现的次数比其他所有数字出现的次数和还要多。因此我们可以在遍历数组的时候记录两个值:1. 数组中的数字;2. 次数。遍历下一个数字时,若它与之前保存的数字相同,则次数加1,否则次数减1;若次数为0,则保存下一个数字,并将次数置为1。遍历结束后,所保存的数字即为所求。最后再判断它是否符合条件。 参考代码 法1: import java.util.HashMap; import java.util.Ma...
- 下一篇
Java高级编程——MySql采用的算法原理
摘要 本文以MySQL数据库为研究对象,讨论与数据库索引相关的一些话题。特别需要说明的是,MySQL支持诸多存储引擎,而各种存储引擎对索引的支持也各不相同,因此MySQL数据库支持多种索引类型,如BTree索引,哈希索引,全文索引等等。为了避免混乱,本文将只关注于BTree索引,因为这是平常使用MySQL时主要打交道的索引,至于哈希索引和全文索引本文暂不讨论。 文章主要内容分为两个部分。 第一部分主要从数据结构及算法理论层面讨论MySQL数据库索引的数理基础。 第二部分结合MySQL数据库中MyISAM和InnoDB数据存储引擎中索引的架构实现讨论聚集索引、非聚集索引及覆盖索引等话题。 数据结构及算法基础 索引的本质 MySQL官方对索引的定义为:索引(Index)是帮助MySQL高效获取数据的数据结构。提取句子主干,就可以得到索引的本质:索引是数据结构。 我们知道,数据库查询是数据库的最主要功能之一。我们都希望查询数据的速度能尽可能的快,因此数据库系统的设计者会从查询算法的角度进行优化。最基本的查询算法当然是顺序查找(linear search),这种复杂度为O(n)的算法在数据量...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS7编译安装Cmake3.16.3,解决mysql等软件编译问题
- SpringBoot2整合MyBatis,连接MySql数据库做增删改查操作
- SpringBoot2整合Thymeleaf,官方推荐html解决方案
- CentOS关闭SELinux安全模块
- CentOS8安装Docker,最新的服务器搭配容器使用
- CentOS7,CentOS8安装Elasticsearch6.8.6
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- MySQL8.0.19开启GTID主从同步CentOS8
- Red5直播服务器,属于Java语言的直播服务器