《Kotlin 程序设计》第二章 快速开始:HelloWorld
第二章 快速开始:HelloWorld
正式上架:《Kotlin极简教程》Official on shelves: Kotlin Programming minimalist tutorial
京东JD:https://item.jd.com/12181725.html
天猫Tmall:https://detail.tmall.com/item.htm?id=558540170670
使用云端在线IDE学习Kotlin
我们不需要搭建任何环境,只要有一台能联网的设备,打开浏览器访问:
你就可以尽情享受Kotlin的乐趣了。
使用本地命令行环境编译执行Kotlin代码
当然,我们也可以搭建本地环境,编译执行kotlin代码。
程序的本质就是映射(函数)。比如说kotlinc这个程序,我们知道,Kotlin基于Java虚拟机(JVM),通过Kotlinc编译器生成的JVM字节码与Java编译的字节码基本相同,也因此与Java可以完全兼容,并且语法更加简洁。
Downloading the compiler
Every release ships with a standalone version of the compiler. We can download it from [GitHub Releases]({{ site.data.releases.latest.url }}). The latest release is {{ site.data.releases.latest.version }}.
Manual Install
Unzip the standalone compiler into a directory and optionally add the bin
directory to the system path. The bin
directory contains the scripts needed to compile and run Kotlin on Windows, OS X and Linux.
SDKMAN!
An easier way to install Kotlin on UNIX based systems such as OS X, Linux, Cygwin, FreeBSD and Solaris is by using SDKMAN!.
Simply run the following in a terminal and follow any instructions:
$ curl -s https://get.sdkman.io | bash
Next open a new terminal and install Kotlin with:
$ sdk install kotlin
Homebrew
Alternatively, on OS X you can install the compiler via Homebrew.
$ brew update $ brew install kotlin
MacPorts
If you're a MacPorts user, you can install the compiler with:
$ sudo port install kotlin
Creating and running a first application
-
Create a simple application in Kotlin that displays Hello, World!. Using our favorite editor, we create a new file called hello.kt with the following:
fun main(args: Array<String>) { println("Hello, World!") }
-
Compile the application using the Kotlin compiler
$ kotlinc hello.kt -include-runtime -d hello.jar
The
-d
option indicates what we want the output of the compiler to be called and may be either a directory name for class files or a .jar file name. The-include-runtime
option makes the resulting .jar file self-contained and runnable by including the Kotlin runtime library in it.
If you want to see all available options run$ kotlinc -help
-
Run the application.
$ java -jar hello.jar
Compiling a library
If you're developing a library to be used by other Kotlin applications, you can produce the .jar file without including the Kotlin runtime into it.
$ kotlinc hello.kt -d hello.jar
Since binaries compiled this way depend on the Kotlin runtime you should make sure the latter is present in the classpath whenever your compiled library is used.
You can also use the kotlin
script to run binaries produced by the Kotlin compiler:
$ kotlin -classpath hello.jar HelloKt
HelloKt
is the main class name that the Kotlin compiler generates for the file named hello.kt
.
Running the REPL
We can run the compiler without parameters to have an interactive shell. We can type any valid Kotlin code and see the results.
}})
Using the command line to run scripts
Kotlin can also be used as a scripting language. A script is a Kotlin source file (.kts) with top level executable code.
import java.io.File val folders = File(args[0]).listFiles { file -> file.isDirectory() } folders?.forEach { folder -> println(folder) }
To run a script, we just pass the -script
option to the compiler with the corresponding script file.
$ kotlinc -script list_folders.kts <path_to_folder_to_inspect>
使用 Intelli IDEA开发Kotlin程序
Kotlin是JVM上的语言,运行环境需要JDK环境。如果我们学习Kotlin,就不建议使用eclipse了。毕竟Kotlin是JetBrains家族的亲儿子,跟Intelli IDEA是血浓于水啊。
我们使用IDEA新建gradle项目,选择Java,Kotlin(Java)框架支持,如下图:
新建完项目,我们写一个HelloWorld.kt类
package com.easy.kotlin /** * Created by jack on 2017/5/29. */ import java.util.Date import java.text.SimpleDateFormat fun main(args: Array<String>) { println("Hello, world!") println(SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(Date())) }
整体的项目目录结构如下
. ├── README.md ├── build │ ├── classes │ │ └── main │ │ ├── META-INF │ │ │ └── easykotlin_main.kotlin_module │ │ └── com │ │ └── easy │ │ └── kotlin │ │ └── HelloWorldKt.class │ └── kotlin-build │ └── caches │ └── version.txt ├── build.gradle ├── settings.gradle └── src ├── main │ ├── java │ ├── kotlin │ │ └── com │ │ └── easy │ │ └── kotlin │ │ └── HelloWorld.kt │ └── resources └── test ├── java ├── kotlin └── resources 21 directories, 7 files
直接运行HelloWorld.kt,输出结果如下
Hello, world! 2017-05-29 01:15:30
关于工程的编译、构建、运行,是由gradle协同kotlin-gradle-plugin,在kotlin-stdlib-jre8,kotlin-stdlib核心依赖下完成的。build.gradle配置文件如下:
group 'com.easy.kotlin' version '1.0-SNAPSHOT' buildscript { ext.kotlin_version = '1.1.1' repositories { mavenCentral() } dependencies { classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version" } } apply plugin: 'java' apply plugin: 'kotlin' sourceCompatibility = 1.8 repositories { mavenCentral() } dependencies { compile "org.jetbrains.kotlin:kotlin-stdlib-jre8:$kotlin_version" testCompile group: 'junit', name: 'junit', version: '4.12' }
工程源码地址:https://github.com/EasyKotlin/easykotlin/tree/easykotlin_hello_world_20170529
使用Android Studio开发 Kotlin Android应用
2017谷歌I/O大会:宣布 Kotlin 成 Android 开发一级语言。
2017谷歌I/O大会上,谷歌宣布,将Kotlin语言作为安卓开发的一级编程语言。Kotlin由JetBrains公司开发,与Java100%互通,并具备诸多Java尚不支持的新特性。谷歌称还将与JetBrains公司合作,为Kotlin设立一个非盈利基金会。
JetBrains在2010年首次推出Kotlin编程语言,并在次年将之开源。下一版的AndroidStudio(3.0)也将提供支持。
下面我们简要介绍如何在Android上开始一个Kotlin的HelloWorld程序。
对于我们程序员来说,我们正处于一个美好的时代。得益于互联网的发展、工具的进步,我们现在学习一门新技术的成本和难度都比过去低了很多。
假设你之前没有使用过Kotlin,那么从头开始写一个HelloWorld的app也只需要这么几步:
1.首先,你要有一个Android Studio。
本书中,笔者用的是2.2.3版本,其它版本应该也大同小异。
Android Studio 2.3.1 Build #AI-162.3871768, built on April 1, 2017 JRE: 1.8.0_112-release-b06 x86_64 JVM: OpenJDK 64-Bit Server VM by JetBrains s.r.o
2.其次,安装一个Kotlin的插件。
依次打开:Android Studio > Preferences > Plugins,
然后选择『Browse repositories』,在搜索框中搜索Kotlin,结果列表中的『Kotlin』插件,如下图
点击安装,安装完成之后,重启Android Studio。
3.新建一个Android项目
重新打开Android Studio,新建一个Android项目吧,添加一个默认的MainActivity——像以前一样即可。
4.Java to Kotlin
安装完插件的AndroidStudio现在已经拥有开发Kotlin的功能。我们先来尝试它的转换功能:Java -> Kotlin,可以把现有的java文件翻译成Kotlin文件。
打开MainActivity文件,在Code菜单下面可以看到一个新的功能:Convert Java File to Kotlin File。
点击转换,
可以看到转换后的Kotlin文件:MainActivity.kt
package com.kotlin.easy.kotlinandroid import android.support.v7.app.AppCompatActivity import android.os.Bundle class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) } }
这个转换功能,对我们Java程序员在学习Kotlin是十分实用。我们可以基于我们之前的Java编码的经验来迅速学习Kotlin编程。
5.配置gradle文件
MainActivity已经被转换成了Kotlin实现,但是项目目前gradle编译、构建、运行还不能执行,还需要进一步配置一下,让项目支持grade的编译、运行。当然,这一步也不需要我们做太多工作——IDEA都已经帮我们做好了。
在Java代码转换成Kotlin代码之后,打开MainActivity.kt文件,编译器会提示"Kotlin not configured",点击一下Configure按钮,IDEA就会自动帮我们把配置文件写好了。
我们可以看出,主要的依赖项是:
kotlin-gradle-plugin plugin: 'kotlin-android' kotlin-stdlib-jre7
完整的配置文件如下:
Project build.gradle
// Top-level build file where you can add configuration options common to all sub-projects/modules. buildscript { ext.kotlin_version = '1.1.2-4' repositories { jcenter() } dependencies { classpath 'com.android.tools.build:gradle:2.3.1' classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version" // NOTE: Do not place your application dependencies here; they belong // in the individual module build.gradle files } } allprojects { repositories { jcenter() } } task clean(type: Delete) { delete rootProject.buildDir }
Module build.gradle
apply plugin: 'com.android.application' apply plugin: 'kotlin-android' android { compileSdkVersion 25 buildToolsVersion "25.0.2" defaultConfig { applicationId "com.kotlin.easy.kotlinandroid" minSdkVersion 14 targetSdkVersion 25 versionCode 1 versionName "1.0" testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' } } } dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) androidTestCompile('com.android.support.test.espresso:espresso-core:2.2.2', { exclude group: 'com.android.support', module: 'support-annotations' }) compile 'com.android.support:appcompat-v7:25.3.1' compile 'com.android.support.constraint:constraint-layout:1.0.2' testCompile 'junit:junit:4.12' compile "org.jetbrains.kotlin:kotlin-stdlib-jre7:$kotlin_version" } repositories { mavenCentral() }
所以说使用IDEA来写Kotlin代码,这工具的完美集成会让你用起来如丝般润滑。毕竟Kotlin的亲爸爸JetBrains是专门做工具的,而且Intelli IDEA又是那么敏捷、智能。
配置之后,等Gradle Sync完成,即可运行。
6.运行
运行结果如下
工程源码:https://github.com/EasyKotlin/KotlinAndroid
参考资料
1.https://www.kotliner.cn/2017/03/13/%E5%BF%AB%E9%80%9F%E4%B8%8A%E6%89%8B%20Kotlin%2011%E6%8B%9B/
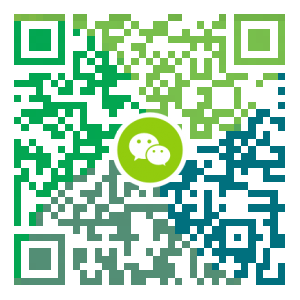
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
《Kotlin 程序设计》第一章 Kotlin简介
第一章 Kotlin简介 正式上架:《Kotlin极简教程》Official on shelves: Kotlin Programming minimalist tutorial 京东JD:https://item.jd.com/12181725.html 天猫Tmall:https://detail.tmall.com/item.htm?id=558540170670 科特林岛(Котлин)是一座俄罗斯的岛屿,位于圣彼得堡以西约30公里处,形状狭长,东西长度约14公里,南北宽度约2公里,面积有16平方公里,扼守俄国进入芬兰湾的水道。科特林岛上建有喀琅施塔得市,为圣彼得堡下辖的城市。[7] 此开篇第一章也。 我们这里讲的Kotlin,就是一门以这个Котлин岛命名的现代程序设计语言。其主要设计者是来自 Saint Petersburg, Russia JetBrains团队的布雷斯拉夫,( Andrey Breslav , 更多信息可以参考:https://www.linkedin.com/in/abreslav/ ),源码实现主要是JetBrains团队以及开源贡献者。 1. K...
- 下一篇
iOS中设置视图的背景图片
在iOS开发中为了美化界面,可能需要给视图加个背景图片。这个过程可以在didViewLoad里面添加。但是view没有类似backgroundImage这样的方法属性来设置背景图片,但是他有已经方法setBackgroundColor:来设置背景颜色。 另外还可以通过UIColor的相关方法colorWithPatternImage根据一个图片来获取对于的颜色,这样绕一下可以达到设置背景图片的效果: 代码如下: - (void)viewDidLoad{ [superviewDidLoad]; [[selfview]setBackgroundColor:[UIColorcolorWithPatternImage:[UIImageimageNamed:@"bg_sand.png"]]] ; } 作者: 峻祁连 邮箱:junqilian@163.com 出处: http://junqilian.cnblogs.com 转载请保留此信息。 本文转自峻祁连. Moving to Cloud/Mobile博客园博客,原文链接:http://www.cnblogs.com/junqil...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- SpringBoot2全家桶,快速入门学习开发网站教程
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- SpringBoot2整合Thymeleaf,官方推荐html解决方案
- 设置Eclipse缩进为4个空格,增强代码规范
- MySQL8.0.19开启GTID主从同步CentOS8
- SpringBoot2编写第一个Controller,响应你的http请求并返回结果
- CentOS6,CentOS7官方镜像安装Oracle11G
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- Hadoop3单机部署,实现最简伪集群