Java描述设计模式(04):抽象工厂模式
GitHub地址:https://github.com/cicadasmile/model-arithmetic-parent
一、抽象工厂模式
1、生活场景
汽车生产根据用户选择的汽车类型,指定不同的工厂进行生产,选择红旗轿车,就要使用中国工厂,选择奥迪轿车,就要使用德国工厂。
2、抽象工厂模式
1) 抽象工厂模式:定义了一个interface用于创建相关对象或相互依赖的对象,而无需指明具体的类;
2) 抽象工厂模式可以将简单工厂模式和工厂方法模式进行整合;
3) 从设计层面看,抽象工厂模式就是对简单工厂模式的改进(或者称为进一步的抽象)。
4) 将工厂抽象成两层,AbstractFactory(抽象工厂) 和 具体实现的工厂子类,方便程序扩展。
3、代码UML图
4、源代码实现
/** * 抽象工厂模式 */ public class C01_AbstractFactory { public static void main(String[] args) { CarProductFactory factory = new ChinaCarFactory() ; factory.getCar("hq") ; factory = new GermanyCarFactory () ; factory.getCar("ad") ; } } // 汽车生产抽象工厂 interface CarProductFactory { CarProduct getCar (String type) ; } // 中国汽车工厂 class ChinaCarFactory implements CarProductFactory { @Override public CarProduct getCar(String type) { CarProduct product = null ; if ("hq".equals(type)){ product = new HQCar() ; product.name="红旗一号" ; product.date="1999-09-19" ; product.material(); product.origin(); } else if ("df".equals(type)){ product = new DFCar() ; product.name="东风一号" ; product.date="2019-09-19" ; product.material(); product.origin(); } return product ; } } // 德国汽车工厂 class GermanyCarFactory implements CarProductFactory { @Override public CarProduct getCar(String type) { CarProduct product = null ; if ("ad".equals(type)){ product = new ADCar() ; product.name="奥迪A8" ; product.date="2017-09-19" ; product.material(); product.origin(); } else if ("bm".equals(type)){ product = new BMCar() ; product.name="宝马X8" ; product.date="2018-09-19" ; product.material(); product.origin(); } return product ; } } // 汽车生产抽象类 abstract class CarProduct { /** * 汽车名称 */ protected String name ; /** * 生产日期 */ protected String date ; /** * 材料 */ abstract void material () ; /** * 产地 */ abstract void origin () ; } // 红旗车 class HQCar extends CarProduct { @Override void material() { System.out.println(super.name+"材料..."); } @Override void origin() { System.out.println(super.date+":"+super.name+"在中国北京生产"); } } // 东风车 class DFCar extends CarProduct { @Override void material() { System.out.println(super.name+"材料..."); } @Override void origin() { System.out.println(super.date+":"+super.name+"在中国南京生产"); } } // 奥迪车 class ADCar extends CarProduct { @Override void material() { System.out.println(super.name+"材料..."); } @Override void origin() { System.out.println(super.date+":"+super.name+"在德国柏林生产"); } } // 宝马车 class BMCar extends CarProduct { @Override void material() { System.out.println(super.name+"材料..."); } @Override void origin() { System.out.println(super.date+":"+super.name+"在德国慕尼黑生产"); } }
二、Spring框架应用
1、场景描述
Spring框架中获取配置文件中Bean的多种方式。
2、核心配置
<bean id="carBean" class="com.model.design.spring.node04.abstractFactory.CarBean"> <property name="name" value="中国红旗" /> </bean> <bean id="carBean1" class="com.model.design.spring.node04.abstractFactory.CarBean"> <property name="name" value="德国奥迪" /> </bean>
3、测试文件
这里使用了两种方式获取。
@RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration(locations = {"classpath:/spring/spring-abstract-factory.xml"}) public class SpringTest { @Resource private BeanFactory beanFactory ; @Test public void test01 (){ CarBean carBean = (CarBean)beanFactory.getBean("carBean") ; System.out.println(carBean.getName()); } @Test public void test02 (){ ApplicationContext context01 = new ClassPathXmlApplicationContext( "/spring/spring-abstract-factory.xml"); CarBean carBean = (CarBean)context01.getBean("carBean1") ; System.out.println(carBean.getName()); } }
4、结构分析
抽象工厂封装对象的创建。在Spring中,通过实现BeanFactory。可以从Spring的各种容器获取bean。根据Bean的配置,getBean方法可以返回不同类型的对象(单例作用域)或初始化新的对象(原型作用域)。在BeanFactory的实现中,我们可以区分:ClassPathXmlApplicationContext,XmlWebApplicationContext等。
三、工厂模式小结
三种工厂模式 (简单工厂模式、工厂方法模式、抽象工厂模式),工厂模式的核心用意将实例化对象的代码封装起来,放到工厂类中统一管理和维护,完成代码依赖关系的解耦。从而提高程序的可扩展性和维护性。
四、源代码地址
GitHub地址:知了一笑 https://github.com/cicadasmile/model-arithmetic-parent 码云地址:知了一笑 https://gitee.com/cicadasmile/model-arithmetic-parent
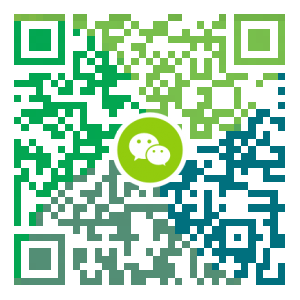
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
java.io.File实现批量创建,递归目录查询、删除操作
今天使用java的File类实现了一个简单的创建多层级多维度的文件目录结构结构如下同时使用递归方法实现了文件的遍历查询和全部删除。(核心思想)判断文件是不是目录,如果是目录就进行递归调用,否则直接进行处理。下面看一下代码吧! import java.io.File; import java.io.IOException; /** * * @author jjking * @version v1.0 * * 创建时间: 2019年8月28日 下午3:10:27 * */ public class ClassWork01 { static final int NUM = 10; /** * 创建文件夹 * @param path 要创建的路径 */ public static void createFilesAndDirs(String path) { File file = new File(path); file.mkdir(); for (int i = 0; i < NUM; i++) { File file0 = new File(path +"\\"+ i); File ...
- 下一篇
Sharding-Jdbc分库分表的导读
前言 Sharding-JDBC是一个开源的分布式数据库中间件,它无需额外部署和依赖,完全兼容JDBC和各种ORM框架。Sharding-JDBC作为面向开发的微服务云原生基础类库,完整的实现了分库分表、读写分离和分布式主键功能,并初步实现了柔性事务。 以2.0.3为例maven包依赖如下 <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.dongpeng</groupId> <artifactId>sharding-jdbc</artifactId> &...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- SpringBoot2全家桶,快速入门学习开发网站教程
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- SpringBoot2整合Thymeleaf,官方推荐html解决方案
- 设置Eclipse缩进为4个空格,增强代码规范
- MySQL8.0.19开启GTID主从同步CentOS8
- SpringBoot2编写第一个Controller,响应你的http请求并返回结果
- CentOS6,CentOS7官方镜像安装Oracle11G
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- Hadoop3单机部署,实现最简伪集群