JS常用正则表达式备忘录
摘要: 玩转正则表达式。
- 原文:JS常用正则表达式备忘录
- 作者:前端小智
Fundebug经授权转载,版权归原作者所有。
正则表达式或“regex”用于匹配字符串的各个部分 下面是我创建正则表达式的备忘单。
匹配正则
使用 .test()
方法
let testString = "My test string"; let testRegex = /string/; testRegex.test(testString);
匹配多个模式
使用操作符号 |
const regex = /yes|no|maybe/;
忽略大小写
使用i
标志表示忽略大小写
const caseInsensitiveRegex = /ignore case/i; const testString = 'We use the i flag to iGnOrE CasE'; caseInsensitiveRegex.test(testString); // true
提取变量的第一个匹配项
使用 .match()
方法
const match = "Hello World!".match(/hello/i); // "Hello"
提取数组中的所有匹配项
使用 g
标志
const testString = "Repeat repeat rePeAT"; const regexWithAllMatches = /Repeat/gi; testString.match(regexWithAllMatches); // ["Repeat", "repeat", "rePeAT"]
匹配任意字符
使用通配符.
作为任何字符的占位符
// To match "cat", "BAT", "fAT", "mat" const regexWithWildcard = /.at/gi; const testString = "cat BAT cupcake fAT mat dog"; const allMatchingWords = testString.match(regexWithWildcard); // ["cat", "BAT", "fAT", "mat"]
用多种可能性匹配单个字符
- 使用字符类,你可以使用它来定义要匹配的一组字符
- 把它们放在方括号里
[]
//匹配 "cat" "fat" and "mat" 但不匹配 "bat" const regexWithCharClass = /[cfm]at/g; const testString = "cat fat bat mat"; const allMatchingWords = testString.match(regexWithCharClass); // ["cat", "fat", "mat"]
匹配字母表中的字母
使用字符集内的范围 [a-z]
const regexWidthCharRange = /[a-e]at/; const regexWithCharRange = /[a-e]at/; const catString = "cat"; const batString = "bat"; const fatString = "fat"; regexWithCharRange.test(catString); // true regexWithCharRange.test(batString); // true regexWithCharRange.test(fatString); // false
匹配特定的数字和字母
你还可以使用连字符来匹配数字
const regexWithLetterAndNumberRange = /[a-z0-9]/ig; const testString = "Emma19382"; testString.match(regexWithLetterAndNumberRange) // true
匹配单个未知字符
要匹配您不想拥有的一组字符,使用否定字符集 ^
const allCharsNotVowels = /[^aeiou]/gi; const allCharsNotVowelsOrNumbers = /[^aeiou0-9]/gi;
匹配一行中出现一次或多次的字符
使用 +
标志
const oneOrMoreAsRegex = /a+/gi; const oneOrMoreSsRegex = /s+/gi; const cityInFlorida = "Tallahassee"; cityInFlorida.match(oneOrMoreAsRegex); // ['a', 'a', 'a']; cityInFlorida.match(oneOrMoreSsRegex); // ['ss'];
匹配连续出现零次或多次的字符
使用星号 *
const zeroOrMoreOsRegex = /hi*/gi; const normalHi = "hi"; const happyHi = "hiiiiii"; const twoHis = "hiihii"; const bye = "bye"; normalHi.match(zeroOrMoreOsRegex); // ["hi"] happyHi.match(zeroOrMoreOsRegex); // ["hiiiiii"] twoHis.match(zeroOrMoreOsRegex); // ["hii", "hii"] bye.match(zeroOrMoreOsRegex); // null
惰性匹配
- 字符串中与给定要求匹配的最小部分
- 默认情况下,正则表达式是贪婪的(匹配满足给定要求的字符串的最长部分)
- 使用
?
阻止贪婪模式(惰性匹配 )
const testString = "catastrophe"; const greedyRexex = /c[a-z]*t/gi; const lazyRegex = /c[a-z]*?t/gi; testString.match(greedyRexex); // ["catast"] testString.match(lazyRegex); // ["cat"]
匹配起始字符串模式
要测试字符串开头的字符匹配,请使用插入符号^
,但要放大开头,不要放到字符集中
const emmaAtFrontOfString = "Emma likes cats a lot."; const emmaNotAtFrontOfString = "The cats Emma likes are fluffy."; const startingStringRegex = /^Emma/; startingStringRegex.test(emmaAtFrontOfString); // true startingStringRegex.test(emmaNotAtFrontOfString); // false
代码部署后可能存在的BUG没法实时知道,事后为了解决这些BUG,花了大量的时间进行log 调试,这边顺便给大家推荐一个好用的BUG监控工具 Fundebug。
匹配结束字符串模式
使用 $
来判断字符串是否是以规定的字符结尾
const emmaAtBackOfString = "The cats do not like Emma"; const emmaNotAtBackOfString = "Emma loves the cats"; const startingStringRegex = /Emma$/; startingStringRegex.test(emmaAtBackOfString); // true startingStringRegex.test(emmaNotAtBackOfString); // false
匹配所有字母和数字
使用\word
简写
const longHand = /[A-Za-z0-9_]+/; const shortHand = /\w+/; const numbers = "42"; const myFavoriteColor = "magenta"; longHand.test(numbers); // true shortHand.test(numbers); // true longHand.test(myFavoriteColor); // true shortHand.test(myFavoriteColor); // true
除了字母和数字,其他的都要匹配
用\W
表示 \w
的反义
const noAlphaNumericCharRegex = /\W/gi; const weirdCharacters = "!_$!!"; const alphaNumericCharacters = "ab283AD"; noAlphaNumericCharRegex.test(weirdCharacters); // true noAlphaNumericCharRegex.test(alphaNumericCharacters); // false
匹配所有数字
你可以使用字符集[0-9]
,或者使用简写 \d
const digitsRegex = /\d/g; const stringWithDigits = "My cat eats $20.00 worth of food a week."; stringWithDigits.match(digitsRegex); // ["2", "0", "0", "0"]
匹配所有非数字
用\D
表示 \d
的反义
const nonDigitsRegex = /\D/g; const stringWithLetters = "101 degrees"; stringWithLetters.match(nonDigitsRegex); // [" ", "d", "e", "g", "r", "e", "e", "s"]
匹配空格
使用 \s
来匹配空格和回车符
const sentenceWithWhitespace = "I like cats!" var spaceRegex = /\s/g; whiteSpace.match(sentenceWithWhitespace); // [" ", " "]
匹配非空格
用\S
表示 \s
的反义
const sentenceWithWhitespace = "C a t" const nonWhiteSpaceRegex = /\S/g; sentenceWithWhitespace.match(nonWhiteSpaceRegex); // ["C", "a", "t"]
匹配的字符数
你可以使用 {下界,上界}
指定一行中的特定字符数
const regularHi = "hi"; const mediocreHi = "hiii"; const superExcitedHey = "heeeeyyyyy!!!"; const excitedRegex = /hi{1,4}/; excitedRegex.test(regularHi); // true excitedRegex.test(mediocreHi); // true excitedRegex.test(superExcitedHey); //false
匹配最低个数的字符数
使用{下界, }
定义最少数量的字符要求,下面示例表示字母 i
至少要出现2次
const regularHi = "hi"; const mediocreHi = "hiii"; const superExcitedHey = "heeeeyyyyy!!!"; const excitedRegex = /hi{2,}/; excitedRegex.test(regularHi); // false excitedRegex.test(mediocreHi); // true excitedRegex.test(superExcitedHey); //false
匹配精确的字符数
使用{requiredCount}
指定字符要求的确切数量
const regularHi = "hi"; const bestHi = "hii"; const mediocreHi = "hiii"; const excitedRegex = /hi{2}/; excitedRegex.test(regularHi); // false excitedRegex.test(bestHi); // true excitedRegex.test(mediocreHi); //false
匹配0次或1次
使用 ?
匹配字符 0 次或1次
const britishSpelling = "colour"; const americanSpelling = "Color"; const languageRegex = /colou?r/i; languageRegex.test(britishSpelling); // true languageRegex.test(americanSpelling); // true
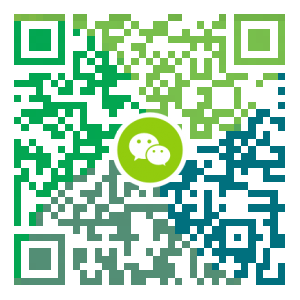
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
阿里云服务器超详细连接
今天发布 更新一下1、远程服务器安装iis+php+mysql环境 iis+php+mysql环境:https://download.csdn.net/download/qq_39161501/10402847 2、解析域名 3、登录服务器绑定域名服务器路径 4、 (1)云服务器ECSA->网络和安全->安全组->创建安全组 https://help.aliyun.com/document_detail/25471.html 5、 (1)云服务器ECS->实例->点击(实例ID/名称) (2)弹出本地实例安全组->加入安全组->找到创建的test安全组 6、访问网址(阿里云服务器搭建成功) 护卫神集成环境下载地址:https://www.huweishen.com/ 护卫神集成环境下载地址:https://www.huweishen.com/优惠详情路径
- 下一篇
如何在java中去除中文文本的停用词
1. 整体思路 第一步:先将中文文本进行分词,这里使用的HanLP-汉语言处理包进行中文文本分词。 第二步:使用停用词表,去除分好的词中的停用词。 2. 中文文本分词环境配置 使用的HanLP-汉语言处理包进行中文文本分词。 ·HanLP-汉语言处理包下载,可以去github上下载 ·HanLP的环境配置有两种方式:方式一、Maven;方式二、下载jar、data、hanlp.properties。 ·官方环境配置步骤也可以在github上查询到。 ·环境配置好后,java使用HanLP进行中文分词文档如下:hanlp.linrunsoft.com/doc.html 3. 下载停用词表 停用词表可以去百度或者其他搜索引擎检索一份,很容易就找到! 4.去除停用词工具类 使用这个工具类的之前,请先完成中文文本分词环境配置,并测试一下。停用词 .txt 文件路径请修改为自己的本地路径。 5. 工具类测试 5.1 测试代码 public class test { public static void main(String args[]) { try { System.out.println...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Eclipse初始化配置,告别卡顿、闪退、编译时间过长
- MySQL8.0.19开启GTID主从同步CentOS8
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- CentOS7编译安装Cmake3.16.3,解决mysql等软件编译问题
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- 设置Eclipse缩进为4个空格,增强代码规范
- CentOS7,CentOS8安装Elasticsearch6.8.6
- CentOS8编译安装MySQL8.0.19
- SpringBoot2整合MyBatis,连接MySql数据库做增删改查操作
- CentOS6,CentOS7官方镜像安装Oracle11G