Java并发编程之ThreadGroup
ThreadGroup是Java提供的一种对线程进行分组管理的手段,可以对所有线程以组为单位进行操作,如设置优先级、守护线程等。
线程组也有父子的概念,如下图:
线程组的创建
1 public class ThreadGroupCreator { 2 3 public static void main(String[] args) { 4 //获取当前线程的group 5 ThreadGroup currentGroup = Thread.currentThread().getThreadGroup(); 6 //在当前线程执行流中新建一个Group1 7 ThreadGroup group1 = new ThreadGroup("Group1"); 8 //Group1的父线程,就是main线程所在Group 9 System.out.println(group1.getParent() == currentGroup); 10 //定义Group2, 指定group1为其父线程 11 ThreadGroup group2 = new ThreadGroup(group1, "Group2"); 12 System.out.println(group2.getParent() == group1); 13 } 14 }
线程组的基本操作
注意:后添加进线程组的线程,其优先级不能大于线程组的优先级
1 public class ThreadGroupBasic { 2 3 public static void main(String[] args) throws InterruptedException { 4 5 ThreadGroup group = new ThreadGroup("group1"); 6 Thread thread = new Thread(group, () -> { 7 while(true) { 8 try { 9 TimeUnit.SECONDS.sleep(1); 10 } catch (InterruptedException e) { 11 e.printStackTrace(); 12 } 13 } 14 }, "thread"); 15 thread.setDaemon(true); 16 thread.start(); 17 18 TimeUnit.MILLISECONDS.sleep(1); 19 20 ThreadGroup mainGroup = Thread.currentThread().getThreadGroup(); 21 //递归获取mainGroup中活跃线程的估计值 22 System.out.println("activeCount = " + mainGroup.activeCount()); 23 //递归获mainGroup中的活跃子group 24 System.out.println("activeGroupCount = " + mainGroup.activeGroupCount()); 25 //获取group的优先级, 默认为10 26 System.out.println("getMaxPriority = " + mainGroup.getMaxPriority()); 27 //获取group的名字 28 System.out.println("getName = " + mainGroup.getName()); 29 //获取group的父group, 如不存在则返回null 30 System.out.println("getParent = " + mainGroup.getParent()); 31 //活跃线程信息全部输出到控制台 32 mainGroup.list(); 33 System.out.println("----------------------------"); 34 //判断当前group是不是给定group的父线程, 如果两者一样,也会返回true 35 System.out.println("parentOf = " + mainGroup.parentOf(group)); 36 System.out.println("parentOf = " + mainGroup.parentOf(mainGroup)); 37 38 } 39 40 }
线程组的Interrupt
1 ublic class ThreadGroupInterrupt { 2 3 public static void main(String[] args) throws InterruptedException { 4 ThreadGroup group = new ThreadGroup("TestGroup"); 5 new Thread(group, () -> { 6 while(true) { 7 try { 8 TimeUnit.MILLISECONDS.sleep(2); 9 } catch (InterruptedException e) { 10 //received interrupt signal and clear quickly 11 System.out.println(Thread.currentThread().isInterrupted()); 12 break; 13 } 14 } 15 System.out.println("t1 will exit"); 16 }, "t1").start(); 17 new Thread(group, () -> { 18 while(true) { 19 try { 20 TimeUnit.MILLISECONDS.sleep(2); 21 } catch (InterruptedException e) { 22 //received interrupt signal and clear quickly 23 System.out.println(Thread.currentThread().isInterrupted()); 24 break; 25 } 26 } 27 System.out.println("t2 will exit"); 28 }, "t2").start(); 29 //make sure all threads start 30 TimeUnit.MILLISECONDS.sleep(2); 31 32 group.interrupt(); 33 } 34 35 }
线程组的destroy
1 public class ThreadGroupDestroy { 2 3 public static void main(String[] args) { 4 ThreadGroup group = new ThreadGroup("TestGroup"); 5 ThreadGroup mainGroup = Thread.currentThread().getThreadGroup(); 6 //before destroy 7 System.out.println("group.isDestroyed=" + group.isDestroyed()); 8 mainGroup.list(); 9 10 group.destroy(); 11 //after destroy 12 System.out.println("group.isDestroyed=" + group.isDestroyed()); 13 mainGroup.list(); 14 } 15 16 }
线程组设置守护线程组
线程组设置为守护线程组,并不会影响其线程是否为守护线程,仅仅表示当它内部没有active的线程的时候,会自动destroy
1 public class ThreadGroupDaemon { 2 3 public static void main(String[] args) throws InterruptedException { 4 ThreadGroup group1 = new ThreadGroup("group1"); 5 new Thread(group1, () -> { 6 try { 7 TimeUnit.SECONDS.sleep(1); 8 } catch (InterruptedException e) { 9 e.printStackTrace(); 10 } 11 }, "group1-thread1").start(); 12 ThreadGroup group2 = new ThreadGroup("group2"); 13 new Thread(group2, () -> { 14 try { 15 TimeUnit.SECONDS.sleep(1); 16 } catch (InterruptedException e) { 17 e.printStackTrace(); 18 } 19 }, "group1-thread2").start(); 20 group2.setDaemon(true); 21 22 TimeUnit.SECONDS.sleep(3); 23 System.out.println(group1.isDestroyed()); 24 System.out.println(group2.isDestroyed()); 25 } 26 }
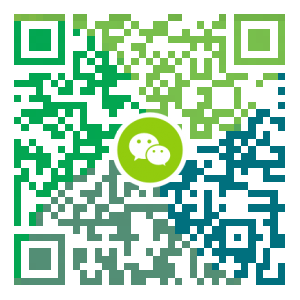
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
《快学 Go 语言》第 4 课 —— 低调的数组
只要掌握了数据结构中的四大法宝,就可以包打天下,他们是:array 、linked list 、hash table、binary tree 。这四大法宝可不是各自为战的,灵活结合才能游刃有余。比如,一个用 hash table 组织的 symbol table,其中个个都是由字符型 array 构成的 linked list 组成的。 --- Go 语言之父 Rob Pike Go 语言里面的数组其实很不常用,这是因为数组是定长的静态的,一旦定义好长度就无法更改,而且不同长度的数组属于不同的类型,之间不能相互转换相互赋值,用起来多有不方便之处。 切片是动态的数组,是可以扩充内容增加长度的数组。当长度不变时,它用起来就和普通数组一样。当长度不同时,它们也属于相同的类型,之间可以相互赋值。这就决定了数组的应用领域都广泛地被切片取代了。 不过也不可以小瞧数组,在切片的底层实现中,数组是切片的基石,是切片的特殊语法隐藏了内部的细节,让用户不能直接看到内部隐藏的数组。切片不过是数组的一个包装,给顽固的数组装上了灵活的翅膀,让石头也可以展翅飞翔。 仅仅是上面纯文字的说明,读者肯定会感觉很懵。下面...
- 下一篇
善用缓存提高你的SPRING工程效率
欢迎查看Java开发之上帝之眼系列教程,如果您正在为Java后端庞大的体系所困扰,如果您正在为各种繁出不穷的技术和各种框架所迷茫,那么本系列文章将带您窥探Java庞大的体系。本系列教程希望您能站在上帝的角度去观察(了解)Java体系。使Java的各种后端技术在你心中模块化;让你在工作中能将Java各个技术了然于心;能够即插即用。本章我们来一起了解Spring工程中缓存的使用。 缓存可以存储常用到的信息,每次需要的时候我们都可以从缓存中获取,本章我们一起来进行了解Spring的缓存抽象.尽管Spring没有提供任何的缓存的实现,但是它对缓存功能提供了声明式的支持,能够与多种流行的缓存进行集成 我们一般在Spring项目中使用缓存功能我们需要对Spring项目进行一些相关的配置,流程如下 启用对缓存的支持 配置缓存管理器 应用缓存 启用对缓存的支持 JavaConfig配置启用 @Configuration @EnableCaching public class SpringCacheConfig { } XML配置启用 <!--启用缓存--> <cache:annota...
相关文章
文章评论
共有0条评论来说两句吧...