python 获取VM物理机信息
内存,CPU
!/usr/bin/python
coding:utf-8
from pyVmomi import vim
from pyVim.connect import SmartConnect, Disconnect, SmartConnectNoSSL
import atexit
import argparse
def get_args():
parser = argparse.ArgumentParser(
description='Arguments for talking to vCenter')
parser.add_argument('-s', '--host', required=True, action='store', help='vSpehre service to connect to') parser.add_argument('-o', '--port', type=int, default=443, action='store', help='Port to connect on') parser.add_argument('-u', '--user', required=True, action='store', help='User name to use') parser.add_argument('-p', '--password', required=True, action='store', help='Password to use') args = parser.parse_args() return args
def get_obj(content, vimtype, name=None):
container = content.viewManager.CreateContainerView(content.rootFolder, vimtype, True)
obj = [ view for view in container.view]
return obj
def main():
esxi_host = {}
args = get_args()
# connect this thing
si = SmartConnectNoSSL(
host=args.host,
user=args.user,
pwd=args.password,
port=args.port)
# disconnect this thing
atexit.register(Disconnect, si)
content = si.RetrieveContent()
esxi_obj = get_obj(content, [vim.HostSystem])
for esxi in esxi_obj:
esxi_host[esxi.name] = {'esxi_info':{},'datastore':{}, 'network': {}, 'vm': {}}
esxi_host[esxi.name]['esxi_info']['厂商'] = esxi.summary.hardware.vendor esxi_host[esxi.name]['esxi_info']['型号'] = esxi.summary.hardware.model for i in esxi.summary.hardware.otherIdentifyingInfo: if isinstance(i, vim.host.SystemIdentificationInfo): esxi_host[esxi.name]['esxi_info']['SN'] = i.identifierValue esxi_host[esxi.name]['esxi_info']['处理器'] = '数量:%s 核数:%s 线程数:%s 频率:%s(%s) ' % (esxi.summary.hardware.numCpuPkgs, esxi.summary.hardware.numCpuCores, esxi.summary.hardware.numCpuThreads, esxi.summary.hardware.cpuMhz, esxi.summary.hardware.cpuModel) esxi_host[esxi.name]['esxi_info']['处理器使用率'] = '%.3f%%' % (float(esxi.summary.quickStats.overallCpuUsage) / (float(esxi.summary.hardware.numCpuPkgs * esxi.summary.hardware.numCpuCores * esxi.summary.hardware.cpuMhz)) * 100) esxi_host[esxi.name]['esxi_info']['内存(MB)'] = esxi.summary.hardware.memorySize/1024/1024 esxi_host[esxi.name]['esxi_info']['可用内存(MB)'] = '%.1f MB' % ((esxi.summary.hardware.memorySize/1024/1024) - esxi.summary.quickStats.overallMemoryUsage) esxi_host[esxi.name]['esxi_info']['内存使用率'] = '%.3f%%' % ((float(esxi.summary.quickStats.overallMemoryUsage)/ (float(esxi.summary.hardware.memorySize/1024/1024))) *100) esxi_host[esxi.name]['esxi_info']['系统'] = esxi.summary.config.product.fullName
for ds in esxi.datastore:
esxi_host[esxi.name]['datastore'][ds.name] = {}
esxi_host[esxi.name]['datastore'][ds.name]['总容量(G)'] = int((ds.summary.capacity)/1024/1024/1024)
esxi_host[esxi.name]['datastore'][ds.name]['空闲容量(G)'] = int((ds.summary.freeSpace)/1024/1024/1024)
esxi_host[esxi.name]['datastore'][ds.name]['类型'] = (ds.summary.type)
for nt in esxi.network:
esxi_host[esxi.name]['network'][nt.name] = {}
esxi_host[esxi.name]['network'][nt.name]['标签ID'] = nt.name
for vm in esxi.vm:
esxi_host[esxi.name]['vm'][vm.name] = {}
esxi_host[esxi.name]['vm'][vm.name]['电源状态'] = vm.runtime.powerState
esxi_host[esxi.name]['vm'][vm.name]['CPU(内核总数)'] = vm.config.hardware.numCPU
esxi_host[esxi.name]['vm'][vm.name]['内存(总数MB)'] = vm.config.hardware.memoryMB
esxi_host[esxi.name]['vm'][vm.name]['系统信息'] = vm.config.guestFullName
if vm.guest.ipAddress:
esxi_host[esxi.name]['vm'][vm.name]['IP'] = vm.guest.ipAddress
else:
esxi_host[esxi.name]['vm'][vm.name]['IP'] = '服务器需要开机后才可以获取'
for d in vm.config.hardware.device:
if isinstance(d, vim.vm.device.VirtualDisk):
esxi_host[esxi.name]['vm'][vm.name][d.deviceInfo.label] = str((d.capacityInKB)/1024/1024) + ' GB'
f = open(args.host + '.txt', 'w') for host in esxi_host: print('ESXI IP:', host) f.write('ESXI IP: %s \n' % host) for hd in esxi_host[host]['esxi_info']: print(' %s: %s' % (hd, esxi_host[host]['esxi_info'][hd])) f.write(' %s: %s' % (hd, esxi_host[host]['esxi_info'][hd]))
for ds in esxi_host[host]['datastore']:
print(' 存储名称:', ds)
f.write(' 存储名称: %s \n' % ds)
for k in esxi_host[host]['datastore'][ds]:
print(' %s: %s' % (k, esxi_host[host]['datastore'][ds][k]))
f.write(' %s: %s \n' % (k, esxi_host[host]['datastore'][ds][k]))
for nt in esxi_host[host]['network']:
print(' 网络名称:', nt)
f.write(' 网络名称:%s \n' % nt)
for k in esxi_host[host]['network'][nt]:
print(' %s: %s' % (k, esxi_host[host]['network'][nt][k]))
f.write(' %s: %s \n' % (k, esxi_host[host]['network'][nt][k]))
for vmachine in esxi_host[host]['vm']:
print(' 虚拟机名称:', vmachine)
f.write(' 虚拟机名称:%s \n' % vmachine)
for k in esxi_host[host]['vm'][vmachine]:
print(' %s: %s' % (k, esxi_host[host]['vm'][vmachine][k]))
f.write(' %s: %s \n' % (k, esxi_host[host]['vm'][vmachine][k]))
f.close()
if name == 'main':
main()
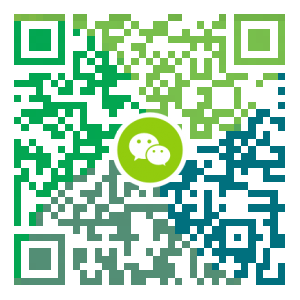
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
python 处理浮点数
a = 1 b = 3 print(a/b) 方法一: print(round(a/b,2)) 方法二: print(format(float(a)/float(b),'.2f')) 方法三: print ('%.2f' %(a/b))
- 下一篇
Java8及新版本许可证及收费?
问题:许可类型及如何收费? JDK 8 Oracle 将继续提供JDK 8 免费的公共更新和自动更新,支持个人用户到 2020 年 12 月底,支持商业用户到 2019 年 1 月;换句话说如果想 Oracle 后续继续为你提供 JDK 8 的支持,那么则需要付费。Oracle目前只提供了部分平台的JDK 8的openJDK构建,多数平台只有第三方的openjdk可以用。 按照 Oracle 公布的支持路线图: java支持路线图 如上图绿色的部分为免费支持的openJDK版本。棕红色部分为Oracke JDK Java SE Advanced, 该系列的产品是收费的,只有在「通用计算」使用范围内是完全免费的。显然通用计算不包括商业使用。 对于最新的java 11 Oracle JDK:支持 BCL 协议,开发人员可以免费使用,但不能用于生产,生产需要商业许可,如果全额付费的话,可支持到 2026 年 9 月。 OpenJDK:支持 GPL V2+CPE协议「一般只支持到发布以后的 6 个月」,可以用于公司使用,因此,对于JDK 11 来说,应该有JDK 11.0.0,然后是两个安全补...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- Hadoop3单机部署,实现最简伪集群
- CentOS7,CentOS8安装Elasticsearch6.8.6
- Eclipse初始化配置,告别卡顿、闪退、编译时间过长
- SpringBoot2更换Tomcat为Jetty,小型站点的福音
- SpringBoot2编写第一个Controller,响应你的http请求并返回结果
- SpringBoot2全家桶,快速入门学习开发网站教程
- SpringBoot2初体验,简单认识spring boot2并且搭建基础工程
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- SpringBoot2整合Thymeleaf,官方推荐html解决方案