Springboot整合redis从安装到FLUSHALL
语言: java+kotlin
windows下安装redis
参考 https://www.cnblogs.com/jaign/articles/7920588.html
安装redis可视化工具 Redis Desktop Manager
参考 https://www.cnblogs.com/zheting/p/7670154.html
依赖
compile('org.springframework.boot:spring-boot-starter-data-redis')
application.yml配置
spring: # redis redis: database: 0 host: localhost port: 6379 password: 12345 jedis: pool: max-active: 10 min-idle: 0 max-idle: 8 timeout: 10000
redis配置类 new RedisConfiguration
package com.futao.springmvcdemo.foundation.configuration import org.springframework.cache.CacheManager import org.springframework.cache.annotation.CachingConfigurerSupport import org.springframework.cache.annotation.EnableCaching import org.springframework.cache.interceptor.KeyGenerator import org.springframework.context.annotation.Bean import org.springframework.context.annotation.Configuration import org.springframework.data.redis.cache.RedisCacheManager import org.springframework.data.redis.connection.RedisConnectionFactory import org.springframework.data.redis.core.RedisTemplate import org.springframework.data.redis.serializer.StringRedisSerializer import javax.annotation.Resource /** * redis配置类 * * @author futao * Created on 2018/10/16. * * redisTemplate.opsForValue();//操作字符串 * redisTemplate.opsForHash();//操作hash * redisTemplate.opsForList();//操作list * redisTemplate.opsForSet();//操作set * redisTemplate.opsForZSet();//操作有序set * */ @Configuration @EnableCaching open class RedisConfiguration : CachingConfigurerSupport() { /** * 自定义redis key的生成规则 */ @Bean override fun keyGenerator(): KeyGenerator { return KeyGenerator { target, method, params -> val builder = StringBuilder() builder.append("${target.javaClass.simpleName}-") .append("${method.name}-") for (param in params) { builder.append("$param-") } builder.toString().toLowerCase() } } /** * 自定义序列化 * 这里的FastJsonRedisSerializer引用的自己定义的 */ @Bean open fun redisTemplate(factory: RedisConnectionFactory): RedisTemplate<String, Any> { val redisTemplate = RedisTemplate<String, Any>() val fastJsonRedisSerializer = FastJsonRedisSerializer(Any::class.java) val stringRedisSerializer = StringRedisSerializer() return redisTemplate.apply { defaultSerializer = fastJsonRedisSerializer keySerializer = stringRedisSerializer hashKeySerializer = stringRedisSerializer valueSerializer = fastJsonRedisSerializer hashValueSerializer = fastJsonRedisSerializer connectionFactory = factory } } @Resource lateinit var redisConnectionFactory: RedisConnectionFactory override fun cacheManager(): CacheManager { return RedisCacheManager.create(redisConnectionFactory) } }
自定义redis中数据的序列化与反序列化 new FastJsonRedisSerializer
package com.futao.springmvcdemo.foundation.configuration import com.alibaba.fastjson.JSON import com.alibaba.fastjson.serializer.SerializerFeature import com.futao.springmvcdemo.model.system.SystemConfig import org.springframework.data.redis.serializer.RedisSerializer import java.nio.charset.Charset /** * 自定义redis中数据的序列化与反序列化 * * @author futao * Created on 2018/10/17. */ class FastJsonRedisSerializer<T>(java: Class<T>) : RedisSerializer<T> { private val clazz: Class<T>? = null /** * Serialize the given object to binary data. * * @param t object to serialize. Can be null. * @return the equivalent binary data. Can be null. */ override fun serialize(t: T?): ByteArray? { return if (t == null) { null } else { JSON.toJSONString(t, SerializerFeature.WriteClassName).toByteArray(Charset.forName(SystemConfig.UTF8_ENCODE)) } } /** * Deserialize an object from the given binary data. * * @param bytes object binary representation. Can be null. * @return the equivalent object instance. Can be null. */ override fun deserialize(bytes: ByteArray?): T? { return if (bytes == null || bytes.isEmpty()) { null } else { val string = String(bytes, Charset.forName(SystemConfig.UTF8_ENCODE)) JSON.parseObject(string, clazz) as T } } }
使用
1. 基于注解的方式
- @Cacheable() redis中的key会根据我们的keyGenerator方法来生成,比如对应下面这个例子,如果曾经以mobile,pageNum,pageSize,orderBy的值执行过list这个方法的话,方法返回的值会存在redis缓存中,下次如果仍然以相同的mobile,pageNum,pageSize,orderBy的值来调用这个方法的话会直接返回缓存中的值
@Service public class UserServiceImpl implements UserService { @Override @Cacheable(value = "user") public List<User> list(String mobile, int pageNum, int pageSize, String orderBy) { PageResultUtils<User> pageResultUtils = new PageResultUtils<>(); final val sql = pageResultUtils.createCriteria(User.class.getSimpleName()) .orderBy(orderBy) .page(pageNum, pageSize) .getSql(); return userDao.list(sql); } }
- 测试
- 第一次请求(可以看到执行了sql,数据是从数据库中读取的)
-
通过redis desktop manager查看redis缓存中已经存储了我们刚才list返回的值
- 后续请求(未执行sql,直接读取的是redis中的值)
- 后续请求(未执行sql,直接读取的是redis中的值)
2. 通过java代码手动set与get
package com.futao.springmvcdemo.controller import com.futao.springmvcdemo.model.entity.User import org.springframework.data.redis.core.RedisTemplate import org.springframework.http.MediaType import org.springframework.web.bind.annotation.GetMapping import org.springframework.web.bind.annotation.RequestMapping import org.springframework.web.bind.annotation.RequestParam import org.springframework.web.bind.annotation.RestController import javax.annotation.Resource /** * @author futao * Created on 2018/10/17. */ @RestController @RequestMapping(path = ["kotlinTest"], produces = [MediaType.APPLICATION_JSON_UTF8_VALUE]) open class KotlinTestController { @Resource private lateinit var redisTemplate: RedisTemplate<Any, Any> /** * 存入缓存 */ @GetMapping(path = ["setCache"]) open fun cache( @RequestParam("name") name: String, @RequestParam("age") age: Int ): User { val user = User().apply { username = name setAge(age.toString()) } redisTemplate.opsForValue().set(name, user) return user } /** * 获取缓存 */ @GetMapping(path = ["getCache"]) open fun getCache( @RequestParam("name") name: String ): User? { return if (redisTemplate.opsForValue().get(name) != null) { redisTemplate.opsForValue().get(name) as User } else null } }
- 测试结果
- 请求(序列化)
-
redis desktop manager中查看
- 读取(反序列化)
- 读取(反序列化)
坑
- 使用注解的方式存入的数据使用redis desktop manager或者
redis-cli --raw
查看显示的是编码之后的,但是使用java代码手动set并不会出现这样的问题(后期需要检查使用注解的方式是不是走了自定义的序列化)
TODO
- redis数据的持久化
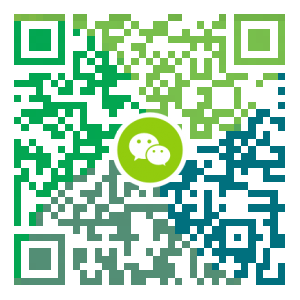
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
Python 新功能:或将允许安全工具查看运行时操作
针对 Python 编程语言的新功能提议之一是希望为运行时添加“透明度”,并让安全和审计工具查看 Python 何时可能运行潜在危险的操作。 在当前的形式下,Python 不允许安全工具查看运行时正在执行的操作。 除非这些操作之一产生可能引起警报的特定错误,否则安全和审计工具就会视而不见,攻击者可能正在使用 Python 在系统上执行恶意操作。 PEP-551 为 Python 提出了两个新的 API 但在 Python Enhancement Proposal 551(PEP-551)中,Python 核心开发人员 Steve Dower 已经提出了两个新的 API,这些 API 将使安全工具能够在 Python 执行潜在危险操作时进行检测。 第一个是 Audit Hook API,它可以引发关于某些类型的 Python 操作的警告消息。 “这些操作通常在 Python 运行时或标准库的深处,比如动态代码编译,模块导入,DNS 解析或使用某些模块,如 ctypes,”Dower 说。 安全或审计工具可能会使用这些消息作为可疑事件的警告标志,并在真正造成危害之前标记或阻止 Python...
- 下一篇
Python字符串颜色输出
\033[1;31;40m # 1是显示方式(可选),31是字体颜色,40m 是字体背景颜色; \033[0m # 恢复终端默认颜色,即取消颜色设置; # cat color.py #!/usr/bin/env python # -*- coding: utf-8 -*- # 字体颜色 print "=====字体颜色======" for i in range(31, 38): print "\033[%s;40mHello world!\033[0m" %i # 背景颜色 print "=====背景颜色======" for i in range(41, 48): print "\033[47;%smHello world!\033[0m" %i # 显示方式 print "=====显示方式======" for i in range(1, 9): print "\033[%s;31;40mHello world!\033[0m" %i # python color.py ----------------------------------------------------...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- SpringBoot2整合MyBatis,连接MySql数据库做增删改查操作
- SpringBoot2整合Redis,开启缓存,提高访问速度
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- Hadoop3单机部署,实现最简伪集群
- CentOS7,CentOS8安装Elasticsearch6.8.6
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- SpringBoot2编写第一个Controller,响应你的http请求并返回结果
- CentOS7安装Docker,走上虚拟化容器引擎之路