使用 C++ 类进行封装的 SQLite3
sqlite3pp
该库对SQLite3 API使用C++类进行包装,支持几乎所有SQLite3功能(打开数据库、查询、执行命令、事物等)。
示例代码如下:
database 数据库
sqlite3pp::database db("test.db"); db.execute("INSERT INTO contacts (name, phone) VALUES ('Mike', '555-1234')");
command 命令
sqlite3pp::command cmd( db, "INSERT INTO contacts (name, phone) VALUES (?, ?)"); cmd.binder() << "Mike" << "555-1234"; cmd.execute(); sqlite3pp::command cmd(db, "INSERT INTO contacts (name, phone) VALUES (?, ?)"); cmd.bind(1, "Mike", sqlite3pp::nocopy); cmd.bind(2, "555-1234", sqlite3pp::nocopy); cmd.execute(); sqlite3pp::command cmd( db, "INSERT INTO contacts (name, phone) VALUES (?100, ?101)"); cmd.bind(100, "Mike", sqlite3pp::nocopy); cmd.bind(101, "555-1234", sqlite3pp::nocopy); cmd.execute(); sqlite3pp::command cmd( db, "INSERT INTO contacts (name, phone) VALUES (:user, :phone)"); cmd.bind(":user", "Mike", sqlite3pp::nocopy); cmd.bind(":phone", "555-1234", sqlite3pp::nocopy); cmd.execute(); sqlite3pp::command cmd( db, "INSERT INTO contacts (name, phone) VALUES (:user, '555-0000');" "INSERT INTO contacts (name, phone) VALUES (:user, '555-1111');" "INSERT INTO contacts (name, phone) VALUES (:user, '555-2222')"); cmd.bind(":user", "Mike", sqlite3pp::nocopy); cmd.execute_all();
transaction 事务
sqlite3pp::transaction xct(db); { sqlite3pp::command cmd( db, "INSERT INTO contacts (name, phone) VALUES (:user, :phone)"); cmd.bind(":user", "Mike", sqlite3pp::nocopy); cmd.bind(":phone", "555-1234", sqlite3pp::nocopy); cmd.execute(); } xct.rollback();
query 查询
sqlite3pp::query qry(db, "SELECT id, name, phone FROM contacts");for (int i = 0; i < qry.column_count(); ++i) { cout << qry.column_name(i) << "\t"; } for (sqlite3pp::query::iterator i = qry.begin(); i != qry.end(); ++i) { for (int j = 0; j < qry.column_count(); ++j) { cout << (*i).get<char const*>(j) << "\t"; } cout << endl; } for (sqlite3pp::query::iterator i = qry.begin(); i != qry.end(); ++i) { int id; char const* name, *phone; std::tie(id, name, phone) = (*i).get_columns<int, char const*, char const*>(0, 1, 2); cout << id << "\t" << name << "\t" << phone << endl; } for (sqlite3pp::query::iterator i = qry.begin(); i != qry.end(); ++i) { string name, phone; (*i).getter() >> sqlite3pp::ignore >> name >> phone; cout << "\t" << name << "\t" << phone << endl; } for (auto v : qry) { string name, phone; v.getter() >> sqlite3pp::ignore >> name >> phone; cout << "\t" << name << "\t" << phone << endl; }
attach
sqlite3pp::database db("foods.db"); db.attach("test.db", "test"); sqlite3pp::query qry( db, "SELECT epi.* FROM episodes epi, test.contacts con WHERE epi.id = con.id");
backup 备份
sqlite3pp::database db("test.db"); sqlite3pp::database backupdb("backup.db"); db.backup(backupdb); db.backup( backupdb, [](int pagecount, int remaining, int rc) { cout << pagecount << "/" << remaining << endl;if (rc == SQLITE_OK || rc == SQLITE_BUSY || rc == SQLITE_LOCKED) { // sleep or do nothing.} });
callback 回调
struct rollback_handler{ void operator()() { cout << "handle_rollback" << endl; } }; sqlite3pp::database db("test.db"); db.set_commit_handler([]{ cout << "handle_commit\n"; return 0; }); db.set_rollback_handler(rollback_handler()); int handle_authorize(int evcode, char const* p1, char const* p2, char const* dbname, char const* tvname) { cout << "handle_authorize(" << evcode << ")" << endl; return 0; } db.set_authorize_handler(&handle_authorize); struct handler{ handler() : cnt_(0) {} void handle_update(int opcode, char const* dbname, char const* tablename, int64_t rowid) { cout << "handle_update(" << opcode << ", " << dbname << ", " << tablename << ", " << rowid << ") - " << cnt_++ << endl; } int cnt_; };using namespace std::placeholders;db.set_update_handler(std::bind(&handler::handle_update, &h, _1, _2, _3, _4));
function
int test0() { return 100; } sqlite3pp::database db("test.db"); sqlite3pp::ext::function func(db); func.create<int ()>("test0", &test0); void test1(sqlite3pp::ext::context& ctx) { ctx.result(200); }void test2(sqlite3pp::ext::context& ctx) { string args = ctx.get<string>(0); ctx.result(args); }void test3(sqlite3pp::ext::context& ctx) { ctx.result_copy(0); } func.create("test1", &test1); func.create("test2", &test2, 1); func.create("test3", &test3, 1); func.create<int ()>("test4", []{ return 500; }); string test5(string const& value) { return value; } string test6(string const& s1, string const& s2, string const& s3) { return s1 + s2 + s3; } func.create<int (int)>("test5", [](int i){ return i + 10000; }); func.create<string (string, string, string)>("test6", &test6); sqlite3pp::query qry( db, "SELECT test0(), test1(), test2('x'), test3('y'), test4(), test5(10), " "test6('a', 'b', 'c')");
aggregate
void step(sqlite3pp::ext::context& c) { int* sum = (int*) c.aggregate_data(sizeof(int)); *sum += c.get<int>(0); }void finalize(sqlite3pp::ext::context& c) { int* sum = (int*) c.aggregate_data(sizeof(int)); c.result(*sum); } sqlite3pp::database db("foods.db"); sqlite3pp::ext::aggregate aggr(db); aggr.create("aggr0", &step, &finalize); struct mycnt{ void step() { ++n_; } int finish() {return n_; } int n_; }; aggr.create<mycnt>("aggr1"); struct strcnt{ void step(string const& s) { s_ += s; } int finish() {return s_.size(); } string s_; };struct plussum{ void step(int n1, int n2) { n_ += n1 + n2; } int finish() {return n_; } int n_; }; aggr.create<strcnt, string>("aggr2"); aggr.create<plussum, int, int>("aggr3"); sqlite3pp::query qry( db, "SELECT aggr0(id), aggr1(type_id), aggr2(name), aggr3(id, type_id) " "FROM foods");
loadable extension 加载扩展
#define SQLITE3PP_LOADABLE_EXTENSION#include <sqlite3ppext.h>int sqlite3_extension_init( sqlite3 *pdb, char **pzErrMsg, const sqlite3_api_routines *pApi) { SQLITE_EXTENSION_INIT2(pApi); sqlite3pp:database db(sqlite3pp::ext::borrow(pdb)); // pdb is not closed since db just borrows it.}
本文来自云栖社区合作伙伴“开源中国”
本文作者:王练
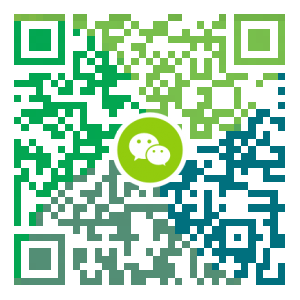
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
Eclipse 4.9 正式发布,支持 Java 11!
来看看 Eclipse 4.9 带来了哪些新特性! 1、外观 新增类似大纲视图的”Minimap” 间主题/暗黑主题,启用方法: Window > Show View > Other,然后在 Show View 对话框中选择General > Minimap 或在Quick Access 搜索框输入“minimap” 改进暗黑主题中的“面包屑导航 (Breadcrumb)” Java 编辑器中的“面包屑导航”在暗黑主题中现在使用了黑色背景,而在日间主题中也使用了扁平化的风格。其实觉得还是别扭,没有 IDEA 中的漂亮,推荐大家阅读Intellij Idea非常6的10个姿势。 2、功能 Project Explorer 可在项目层次结构上显示有问题的标记 使用 Project Explorer 的Projects Presentation > Hierarchical模式时,子项目中的错误标记现在会报告给父节点,包括其他项目中的父项目和文件夹。通过该功能我们可以轻松检测错误,并在项目层次结构折叠时从 Project Explorer 中浏览错误。 创建抽象方法的...
- 下一篇
Java 常用类库 之 Random 随机数类
http://www.verejava.com/?id=169931089810102 import java.util.Random; public class TestRandom { public static void main(String[] args) { //实例化随机数对象 Random rn = new Random(); for (int i = 0; i < 10; i++) { System.out.println(rn.nextInt(10)); } } } //随机数产生验证码 public class Test { public static void main(String[] args) { // char[] chars={'A','B','C','D','E','F','G','H','I','J','K','L','M','N','O','P','Q','R','S','T','U','V','W','X','Y','Z'}; Random rn = new Random(); for (int i = 0; i < 10; i++...
相关文章
文章评论
共有0条评论来说两句吧...