使用Python模拟设备接入阿里云物联网的MQTT服务器
前言
由于阿里云物联网套件关于设备认证的文档不够详细,笔者差不多摸索了几天才连接上MQTT。下面是使用Python模拟设备接入阿里云的MQTT。
概述
阿里云物联网套件提供两种接入方式:
- MQTT客户端域名直连(资源受限设备推荐)
- 先HTTPS发送授权后再连接MQTT(一些特殊增值服务,比如设备级别的引流)
本文主要介绍第一种接入方式,TCP直连,并提供Python代码示例。
主要参数
连接域名
<ProductKey>.iot-as-mqtt.cn-shanghai.aliyuncs.com:1883
MQTT Connect报文参数
1、mqttClientId
mqttClientId = "<ClientId>"+"|securemode=3,signmethod=hmacsha1,timestamp=132323232|"
2、mqttUsername
使用&
拼接<DeviceName>
和<ProductKey>
。
mqttUsername = "<DeviceName>&<ProductKey>"
3、mqttPassword
把以下参数按字典键名排序,再把键名都拼接起来(没有分隔符)生成content,然后以DeviceSecret为盐,对content进行hma_sha1加密,最后二进制转为十六进制字符串表示。
- clientId
- deviceName
- productKey
- timestamp
mqttPassword = hmac_sha1(DeviceSecret, content).toHexString();
示例
假设
- clientId = 12345
- deviceName = device
- productKey = pk
- timestamp = 789
- signmethod = hmacsha1
content拼接结果:clientId12345deviceNamedeviceproductKeypktimestamp789
注意:不用拼接signmethod参数。
对content以DeviceSecret为盐进行hmacsha1加签后,再转为十六进制字符串,最后结果:FAFD82A3D602B37FB0FA8B7892F24A477F851A14
注意:不需要base64。
最后总结一下生成的参数:
- mqttHost =
pk.iot-as-mqtt.cn-shanghai.aliyuncs.com
- mqttPort = 1883
- mqttClientId =
12345|securemode=3,signmethod=hmacsha1,timestamp=789|
- mqttUsername =
device&pk
- mqttPassword =
FAFD82A3D602B37FB0FA8B7892F24A477F851A14
参数说明
参数 | 描述 |
---|---|
ProductKey | 产品Key。从iot套件控制台获取 |
DeviceName | 设备名称。从iot套件控制台获取 |
DeviceSecret | 设别密码,从iot套件控制台获取 |
signmethod | 算法类型,hmacmd5或hmacsha1 |
clientId | 客户端自表示id,建议mac或sn |
timestamp | 当前时间毫秒值,可选 |
securemode | 目前安全模式,可选值有2 (TLS直连模式)、3(TCP直连模式) |
示例代码
填写自己的ProductKey
、ClientId
、DeviceName
、DeviceSecret
。
# coding=utf-8 # !/usr/bin/python3 import datetime import time import hmac import hashlib import math TEST = 0 ProductKey = "" ClientId = "12345" # 自定义clientId DeviceName = "" DeviceSecret = "" # signmethod signmethod = "hmacsha1" # signmethod = "hmacmd5" # 当前时间毫秒值 us = math.modf(time.time())[0] ms = int(round(us * 1000)) timestamp = str(ms) data = "".join(("clientId", ClientId, "deviceName", DeviceName, "productKey", ProductKey, "timestamp", timestamp )) # print(round((time.time() * 1000))) print("data:", data) if "hmacsha1" == signmethod: ret = hmac.new(bytes(DeviceSecret, encoding="utf-8"), bytes(data, encoding="utf-8"), hashlib.sha1).hexdigest() elif "hmacmd5" == signmethod: ret = hmac.new(bytes(DeviceSecret, encoding="utf-8"), bytes(data, encoding="utf-8"), hashlib.md5).hexdigest() else: raise ValueError sign = ret print("sign:", sign) # ====================================================== strBroker = ProductKey + ".iot-as-mqtt.cn-shanghai.aliyuncs.com" port = 1883 client_id = "".join((ClientId, "|securemode=3", ",signmethod=", signmethod, ",timestamp=", timestamp, "|")) username = "".join((DeviceName, "&", ProductKey)) password = sign print("="*30) print("client_id:", client_id) print("username:", username) print("password:", password) print("="*30) def secret_test(): DeviceSecret = "secret" data = "clientId12345deviceNamedeviceproductKeypktimestamp789" ret = hmac.new(bytes(DeviceSecret, encoding="utf-8"), bytes(data, encoding="utf-8"), hashlib.sha1).hexdigest() print("test:", ret) # ====================================================== # MQTT Initialize.-------------------------------------- try: import paho.mqtt.client as mqtt except ImportError: print("MQTT client not find. Please install as follow:") print("git clone http://git.eclipse.org/gitroot/paho/org.eclipse.paho.mqtt.python.git") print("cd org.eclipse.paho.mqtt.python") print("sudo python setup.py install") # ====================================================== def on_connect(mqttc, obj, rc): print("OnConnetc, rc: " + str(rc)) mqttc.subscribe("test", 0) def on_publish(mqttc, obj, mid): print("OnPublish, mid: " + str(mid)) def on_subscribe(mqttc, obj, mid, granted_qos): print("Subscribed: " + str(mid) + " " + str(granted_qos)) def on_log(mqttc, obj, level, string): print("Log:" + string) def on_message(mqttc, obj, msg): curtime = datetime.datetime.now() strcurtime = curtime.strftime("%Y-%m-%d %H:%M:%S") print(strcurtime + ": " + msg.topic + " " + str(msg.qos) + " " + str(msg.payload)) on_exec(str(msg.payload)) def on_exec(strcmd): print("Exec:", strcmd) strExec = strcmd # ===================================================== if __name__ == '__main__': if TEST: secret_test() exit(0) mqttc = mqtt.Client(client_id) mqttc.username_pw_set(username, password) mqttc.on_message = on_message mqttc.on_connect = on_connect mqttc.on_publish = on_publish mqttc.on_subscribe = on_subscribe mqttc.on_log = on_log mqttc.connect(strBroker, port, 120) mqttc.loop_forever()
参考资料
终于知道之前为什么总是连接不上了!!!之前文档对password加密的字段是多了「signmethodhmacsha1」字符串!
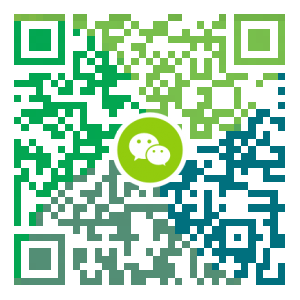
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
IDC:大数据——数字化转型时代的大商机
ZDNET至顶网CIO与应用频道 05月27日 北京消息:2015年5月27日–IDC中国在贵阳举办了年度中国ICT市场趋势论坛,论坛的主题是:“加速创新实现数字化转型”。 在本次论坛上,贵阳市副市长毛有碧女士致开幕词,她重点介绍了贵阳举办本次数博会暨大数据峰会的战略意义。 在一个经济发展水平与发达地区仍有较大差距的西部城市,举办这样一场以大数据为主题的全球性峰会,其所展现出的超前意识显而易见。本次大会的目标就是要为国际大数据产业的发展建言献计,凝聚共识与智慧。毛市长也重点谈到了与IDC的合作,她认为,IDC是全球领先的ICT市场研究与咨询服务商,具有全球视野、良好的本土经验、广泛的行业影响力,未来对贵阳发展大数据产业必将起到重要推动作用”。 这是最坏的阶段:经济增长乏力、实体经济不振、传统行业在被颠覆与重构、IT市场总体增长进入个位数区间、IT第2平台的领导厂商仍在困境中。这是最好的时代:经济结构在调整、改革进程在加快、新兴产业发展迅猛、IT第3平台市场保持高速增长、新兴厂商不断涌现。 IDC中国区总裁霍锦洁认为:“中国的人口红利将非常有助于大数据产业的发展,中国期望在未来技术创新的...
- 下一篇
涨价不停歇 Gartner预测存储涨到2019年
4月18日,目前存储产品的价格上涨持续已经接近一年,而且这种完全出自于市场行为的状况依然没有要停歇的势头。Garter最新的一份报告中称,他们认为想要等到存储设备降价只能到2019年了。 Garter方面表示,他们就掌握的资料进行了分析,整体来看供应商在2017和2018年的产出量依然难以满足市场需求,而2019年薪的供应商加入则有可能会解决这一问题,也就是紫光的长江存储器项目被予以了厚望。 报告显示,除了只能手机和PC以外,物联网设备、自动驾驶等都对存储芯片有着巨大的需求,缺口也很大。而部分存储芯片可能会在今年年底时涨势有所下滑,但是前提是价格已经涨到一定程度。 所以对于有刚需的朋友来讲这并不是一个好消息,能早入手就入手吧,要不然可能真的要错过等两年了。 作者:佚名 来源:51CTO
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS7,CentOS8安装Elasticsearch6.8.6
- CentOS7安装Docker,走上虚拟化容器引擎之路
- Docker安装Oracle12C,快速搭建Oracle学习环境
- Linux系统CentOS6、CentOS7手动修改IP地址
- CentOS7设置SWAP分区,小内存服务器的救世主
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- MySQL8.0.19开启GTID主从同步CentOS8
- CentOS6,CentOS7官方镜像安装Oracle11G
- Mario游戏-低调大师作品
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7