Swift 无限轮播图
ICycleView
ICycleView是一个基于UICollectionView实现的轻量级无限轮播图
Content
- [Features]()
- [Requirements]()
- [CocoaPods]()
-
[Usage]()
- [默认滚动视图]()
- [自定义图片宽度和指示器的位置和颜色]()
- [自定义Cell-纯代码和Xib创建都支持]()
-
[Implementation]()
- [实现原理]()
-
[主要代码]()
- [UICollectionView代理方法]()
- [循环轮播实现]()
- [Contact]()
- [Github]()
Features
- 支持单张图片
- 支持滚动图片宽度设置
- 支持本地图片显示,网路图显示,本地图片和网路图混合显示
- 支持自定义图片展示Cell(纯代码和Xib创建都支持)
- 支持UIPageControl具体位置设置
- 支持UIPageControl显示颜色设置
- 支持图片点击回调
- 支持图片滚动回调
Requirements
- iOS 8.0+
- Swift 4.0+
CocoaPods
pod 'ICycleView', '~> 1.0.0'
在终端 pod search 'ICycleView'
时若出现 Unable to find a pod with name, author, summary, or description matching 'ICycleView'
错误
请在终端运行
1:pod setup
2:$rm ~/Library/Caches/CocoaPods/search_index.json
Usage
默认滚动视图
// 惰性初始化滚动视图 lazy var defaultCycleView: ICycleView = { let cycleView = ICycleView(frame: CGRect(x: 0, y: 50, width: UIScreen.main.bounds.width, height: 130*scaleForPlus)) view.addSubview(cycleView) return cycleView }() // 图片赋值 defaultCycleView.pictures = pictures
自定义图片宽度和指示器的位置和颜色
// 惰性初始化滚动视图 lazy var customPagetrolPositionnCycleView: ICycleView = { let cycleView = ICycleView(frame: CGRect(x: 0, y: 190, width: UIScreen.main.bounds.width, height: 130*scaleForPlus)) cycleView.imgViewWidth = 374*scaleForPlus cycleView.pageIndicatorTintColor = .green view.addSubview(cycleView) return cycleView }() // 图片赋值 customPagetrolPositionnCycleView.pictures = pictures // pageControlStyle属性必须在设置 pictures 后赋值,因为指示器是根据 numberOfPages 计算Size的 customPagetrolPositionnCycleView.pageControlStyle = .bottom(bottom: -20) customPagetrolPositionnCycleView.pageControlStyle = .right(trailing: 30*scaleForPlus)
自定义Cell-纯代码和Xib创建都支持
// 惰性初始化滚动视图 lazy var customPictureCellCycleView: ICycleView = { let cycleView = ICycleView(frame: CGRect(x: 0, y: 345, width: UIScreen.main.bounds.width, height: 130*scaleForPlus)) cycleView.register([UINib.init(nibName: "CustomCycleViewCell", bundle: nil)], identifiers: ["CustomCell"]) cycleView.delegate = self view.addSubview(cycleView) return cycleView }() // 图片赋值 customPictureCellCycleView.pictures = pictures // 代理方法 /** - 协议方法都是可选方法,根据需要实现即可 */ // MARK: ICycleViewDelegate extension ViewController: ICycleViewDelegate { // 图片点击 func iCycleView(cycleView: ICycleView, didSelectItemAt index: Int) { print("你点击了第 \(index) 张图片") } // 图片自动滚动 func iCycleView(cycleView: ICycleView, autoScrollingItemAt index: Int) { print("当前滚动的图片是第 \(index) 张") } // 自定义Cell func iCycleView(cycleView: ICycleView, collectionView: UICollectionView, cellForItemAt indexPath: IndexPath, picture: String) -> UICollectionViewCell { let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "CustomCell", for: indexPath) as! CustomCycleViewCell cell.imgView.kf.setImage(with: URL(string: picture)) cell.titleLab.text = "自定义Cell\n第 \(indexPath.item) 张图片" return cell } }
Implementation
实现原理
- collectionView的cell显示两倍数量的图片,展示图片分为两组,默认显示第二组的第一张
- 左滑collectionView到第二组最后一张,即最后一个cell时,设置scrollView的contentOffset显示第一组的最后一张,继续左滑,实现了无限左滑
- 右滑collectionView到第一组第一张,即第一cell时,设置scrollView的contentOffset显示第二组的第一张,继续右滑,实现了无限右滑
- 由2,3实现无限循环
主要代码
UICollectionView代理方法
// MARK: - UICollectionViewDataSource, UICollectionViewDelegate extension ICycleView: UICollectionViewDataSource, UICollectionViewDelegate { public func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int { return pictures.count * 2 } public func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell { if isCustomCell { // 自定义Cell return delegate?.iCycleView?(cycleView: self, collectionView: collectionView, cellForItemAt: IndexPath(item: indexPath.item % pictures.count, section: 0), picture: pictures[indexPath.item % pictures.count]) ?? UICollectionViewCell() } else { // 默认Cell let cell = collectionView.dequeueReusableCell(withReuseIdentifier: ICycleViewConst.cellIdentifier, for: indexPath) as! ICycleViewCell cell.configureCell(picture: pictures[indexPath.item % pictures.count], placeholderImage: placeholderImage, imgViewWidth: imgViewWidth) return cell } } public func collectionView(_ collectionView: UICollectionView, didSelectItemAt indexPath: IndexPath) { delegate?.iCycleView?(cycleView: self, didSelectItemAt: indexPath.item % pictures.count) } }
循环轮播实现
// MARK: - 循环轮播实现 extension ICycleView { // 定时器方法,更新Cell位置 @objc private func updateCollectionViewAutoScrolling() { if let indexPath = collectionView.indexPathsForVisibleItems.last { let nextPath = IndexPath(item: indexPath.item + 1, section: indexPath.section) collectionView.scrollToItem(at: nextPath, at: .centeredHorizontally, animated: true) } } // 开始拖拽时,停止定时器 public func scrollViewWillBeginDragging(_ scrollView: UIScrollView) { timer.fireDate = Date.distantFuture } // 结束拖拽时,恢复定时器 public func scrollViewDidEndDragging(_ scrollView: UIScrollView, willDecelerate decelerate: Bool) { timer.fireDate = Date(timeIntervalSinceNow: autoScrollDelay) } /** - 监听手动减速完成(停止滚动) - 1.collectionView的cell显示两倍数量的图片,展示图片分为两组,默认显示第二组的第一张 - 2.左滑collectionView到第二组最后一张,即最后一个cell时,设置scrollView的contentOffset显示第一组的最后一张,继续左滑,实现了无限左滑 - 3.右滑collectionView到第一组第一张,即第一cell时,设置scrollView的contentOffset显示第二组的第一张,继续右滑,实现了无限右滑 - 4.由2,3实现无限循环 */ public func scrollViewDidEndDecelerating(_ scrollView: UIScrollView) { let offsetX = scrollView.contentOffset.x let page = Int(offsetX / bounds.size.width) let itemsCount = collectionView.numberOfItems(inSection: 0) if page == 0 { // 第一页 collectionView.contentOffset = CGPoint(x: offsetX + CGFloat(pictures.count) * bounds.size.width, y: 0) } else if page == itemsCount - 1 { // 最后一页 collectionView.contentOffset = CGPoint(x: offsetX - CGFloat(pictures.count) * bounds.size.width, y: 0) } } // - 滚动动画结束的时候调用 public func scrollViewDidEndScrollingAnimation(_ scrollView: UIScrollView) { scrollViewDidEndDecelerating(collectionView) } /** - 正在滚动 - 设置分页,算出滚动位置,更新指示器 */ public func scrollViewDidScroll(_ scrollView: UIScrollView) { let offsetX = scrollView.contentOffset.x var page = Int(offsetX / bounds.size.width+0.5) page = page % pictures.count if pageControl.currentPage != page { pageControl.currentPage = page delegate?.iCycleView?(cycleView: self, autoScrollingItemAt: page) } } }
Contact
QQ: 2256472253
Email: ixialuo@126.com
Github
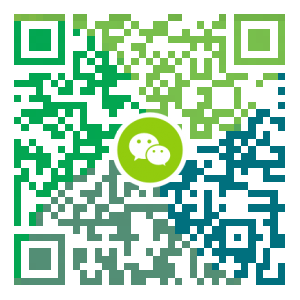
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
Android WebView打开网页一片空白
本文首发于公众号“AntDream”,欢迎微信搜索“AntDream”或扫描文章底部二维码关注,和我一起每天进步一点点 问题描述: 网页链接是Https链接 网页链接在电脑的Chrome浏览器中打开正常 网页链接在手机的Chrome浏览器中打开正常 网页链接在IOS的App上打开正常 网页链接在Android App中打开一片空白,没有任何提示 问题原因 试了很多方法,最后发现是Https的证书有问题,由于网页链接是客户提供的,不知道证书是如何生成的,导致不被Android系统信任 问题的发现之旅 网页链接在手机和电脑都能打开,所以想着网页链接本身应该没有问题。 debug网页加载的过程也没有发现问题 但是后来用UC浏览器打开以后发现加载的过程中会弹出提示: 根据弹出的提示知道很可能是证书的问题,于是重新开始debug项目中的WebView 一般我们在初始化一个WebView时都有几个固定的步骤,其中最重要的一步就是设置WebViewClient enWebview.setWebChromeClient(new WebChromeClient()); enWebview.setWebV...
- 下一篇
Android 7.0相机适配及FileProvider重复那些坑
本文首发于公众号“AntDream”,欢迎微信搜索“AntDream”或扫描文章底部二维码关注,和我一起每天进步一点点 Android 7.0相机拍照适配 (1)首先必须获取拍照的权限 简单一点的可以直接用ActivityCompat的requestPermissions方法 ActivityCompat.requestPermissions(context, new String[]{permission}, requestCode); 权限请求的结果会在onRequestPermissionsResult中回调 @Override public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) { super.onRequestPermissionsResult(requestCode, permissions, grantResults); switch (requestCode) { case 1://对应req...
相关文章
文章评论
共有0条评论来说两句吧...