Android-来填写一个验证码吧!(一)
熟悉web开发中童鞋们都知道为了防止恶意破解、恶意提交等行为,所以我们在提交表单数据时,都会使用随机验证码功能。在Android应用中我们同样需要这一功能,去有效的避开恶意注册,恶意攻击。那么该如何实现呢?
这里介绍一种,数字英文随机生成的图片验证码,使用很简单,一行代码,调用一个工具类,就能完美的实现图片验证的显示了。
效果如下:
**具体思路如下:
在一块固定宽高的画布上,画上固定个数的随机数字和字母,再画上固定条数的干扰线
随机数和干扰线的颜色随机生成,随机数的样式随机生成。**
理清思路,接下来看下代码吧!
public class CodeUtils { //随机码集 private static final char[] CHARS = { '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z' }; private static CodeUtils mCodeUtils; private int mPaddingLeft, mPaddingTop; private StringBuilder mBuilder = new StringBuilder(); private Random mRandom = new Random(); //Default Settings private static final int DEFAULT_CODE_LENGTH = 4;//验证码的长度 这里是4位 private static final int DEFAULT_FONT_SIZE = 60;//字体大小 private static final int DEFAULT_LINE_NUMBER = 3;//多少条干扰线 private static final int BASE_PADDING_LEFT = 20; //左边距 private static final int RANGE_PADDING_LEFT = 30;//左边距范围值 private static final int BASE_PADDING_TOP = 70;//上边距 private static final int RANGE_PADDING_TOP = 15;//上边距范围值 private static final int DEFAULT_WIDTH = 200;//默认宽度.图片的总宽 private static final int DEFAULT_HEIGHT = 100;//默认高度.图片的总高 private static final int DEFAULT_COLOR = Color.rgb(0xee, 0xee, 0xee);//默认背景颜色值 private String code; public static CodeUtils getInstance() { if (mCodeUtils == null) { mCodeUtils = new CodeUtils(); } return mCodeUtils; } //生成验证码图片 public Bitmap createBitmap() { mPaddingLeft = 0; //每次生成验证码图片时初始化 mPaddingTop = 0; Bitmap bitmap = Bitmap.createBitmap(DEFAULT_WIDTH, DEFAULT_HEIGHT, Bitmap.Config.ARGB_8888); Canvas canvas = new Canvas(bitmap); code = createCode(); canvas.drawARGB(0, 0, 0, 0); canvas.drawColor(DEFAULT_COLOR); Paint paint = new Paint(); paint.setTextSize(DEFAULT_FONT_SIZE); for (int i = 0; i < code.length(); i++) { randomTextStyle(paint); randomPadding(); canvas.drawText(code.charAt(i) + "", mPaddingLeft, mPaddingTop, paint); } //干扰线 for (int i = 0; i < DEFAULT_LINE_NUMBER; i++) { drawLine(canvas, paint); } canvas.save(Canvas.ALL_SAVE_FLAG);//保存 canvas.restore(); return bitmap; } /** * 得到图片中的验证码字符串 * * @return */ public String getCode() { return code; } //生成验证码 public String createCode() { mBuilder.delete(0, mBuilder.length()); //使用之前首先清空内容 for (int i = 0; i < DEFAULT_CODE_LENGTH; i++) { mBuilder.append(CHARS[mRandom.nextInt(CHARS.length)]); } return mBuilder.toString(); } //生成干扰线 private void drawLine(Canvas canvas, Paint paint) { int color = randomColor(); int startX = mRandom.nextInt(DEFAULT_WIDTH); int startY = mRandom.nextInt(DEFAULT_HEIGHT); int stopX = mRandom.nextInt(DEFAULT_WIDTH); int stopY = mRandom.nextInt(DEFAULT_HEIGHT); paint.setStrokeWidth(1); paint.setColor(color); canvas.drawLine(startX, startY, stopX, stopY, paint); } //随机颜色 private int randomColor() { mBuilder.delete(0, mBuilder.length()); //使用之前首先清空内容 String haxString; for (int i = 0; i < 3; i++) { haxString = Integer.toHexString(mRandom.nextInt(0xEE)); if (haxString.length() == 1) { haxString = "0" + haxString; } mBuilder.append(haxString); } return Color.parseColor("#" + mBuilder.toString()); } //随机文本样式 private void randomTextStyle(Paint paint) { int color = randomColor(); paint.setColor(color); paint.setFakeBoldText(mRandom.nextBoolean()); //true为粗体,false为非粗体 float skewX = mRandom.nextInt(11) / 10; skewX = mRandom.nextBoolean() ? skewX : -skewX; paint.setTextSkewX(skewX); //float类型参数,负数表示右斜,整数左斜 paint.setUnderlineText(mRandom.nextBoolean()); //true为下划线,false为非下划线 paint.setStrikeThruText(mRandom.nextBoolean()); //true为删除线,false为非删除线 } //随机间距 private void randomPadding() { mPaddingLeft += BASE_PADDING_LEFT + mRandom.nextInt(RANGE_PADDING_LEFT); mPaddingTop = BASE_PADDING_TOP + mRandom.nextInt(RANGE_PADDING_TOP); } }
至此,我们的工具类就完成了,是不是很简单,那么怎么使用呢?
也很简单,我们可以看到通过CodeUtils.getInstance().createBitmap();
方法,就可以获取生成的由工具类生成的图片验证码的bitmap
,那么我们只需要将这个bitmap
,设置到需要展示图片验证码的ImageView
上即可。
以下是完整代码:
布局界面:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <RelativeLayout android:layout_width="match_parent" android:layout_height="50dp" android:background="@android:color/holo_red_light"> <TextView android:id="@+id/tv_title" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:text="设置登录密码" android:textColor="#fff" android:textSize="20sp" /> </RelativeLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="45dp" android:layout_marginLeft="15dp" android:layout_marginRight="15dp" android:layout_marginTop="30dp" android:background="@drawable/bg" android:orientation="vertical"> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center_vertical" android:orientation="horizontal"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="20dp" android:layout_marginRight="20dp" android:text="中国+86" android:textColor="#A2CD5A" android:textSize="16sp" /> <View android:layout_width="0.1dp" android:layout_height="match_parent" android:background="#FF7F00" /> <EditText android:id="@+id/et_forgetPass_PhoneNum" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginLeft="20dp" android:background="@null" android:digits="0123456789" android:hint="请填入您的手机号" android:inputType="number" android:maxLength="11" android:textSize="16sp" /> </LinearLayout> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginLeft="15dp" android:layout_marginRight="15dp" android:layout_marginTop="20dp" android:orientation="horizontal"> <LinearLayout android:layout_width="wrap_content" android:layout_height="45dp" android:background="@drawable/bg"> <EditText android:id="@+id/et_phoneCodes" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_marginLeft="10dp" android:layout_marginRight="10dp" android:background="@null" android:hint="请输入右侧验证码" /> </LinearLayout> <ImageView android:id="@+id/image" android:layout_width="100dp" android:layout_height="match_parent" android:layout_marginLeft="10dp" /> </LinearLayout> <Button android:id="@+id/but_forgetpass_toSetCodes" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_margin="35dp" android:background="#ff5e00" android:text="获取验证码" android:textColor="#fff" /> </LinearLayout>
Activity界面:
public class MainActivity extends AppCompatActivity { private Bitmap bitmap; private String code; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //获取需要展示图片验证码的ImageView final ImageView image = (ImageView) findViewById(R.id.image); //获取工具类生成的图片验证码对象 bitmap = CodeUtils.getInstance().createBitmap(); //获取当前图片验证码的对应内容用于校验 code = CodeUtils.getInstance().getCode(); image.setImageBitmap(bitmap); image.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { bitmap = CodeUtils.getInstance().createBitmap(); code = CodeUtils.getInstance().getCode(); image.setImageBitmap(bitmap); Toast.makeText(MainActivity.this, code, Toast.LENGTH_SHORT).show(); } }); } }
综上;我们就完成了一个常见的图片验证码工具的封装和使用
**当然一定还有更多方法和更优化的逻辑,还请大家提出,共同完善,
有了需求才有了功能,有了想法才有了创作,你的反馈会是使我进步的最大动力。**
觉得还不够方便?还想要什么功能?告诉我!欢迎反馈,欢迎Star。
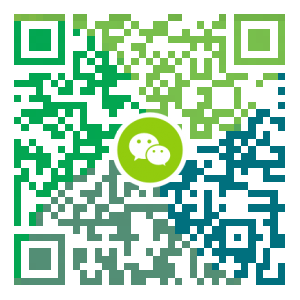
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
《Netkiller Android 手札》之 EventBus 使用详解
本文节选自电子书《Netkiller Android 手札》 Netkiller Android 手札 http://www.netkiller.cn/android/index.html Mr.NeoChan,陈景峯(BG7NYT) 中国广东省深圳市望海路半岛城邦三期 518067 +8613113668890<netkiller@msn.com> $Id: book.xml 606 2013-05-29 09:52:58Z netkiller $ 版权 © 2018 Neo Chan 版权声明 转载请与作者联系,转载时请务必标明文章原始出处和作者信息及本声明。 http://www.netkiller.cn http://netkiller.github.io http://netkiller.sourceforge.net 微信订阅号 netkiller-ebook (微信扫描二维码) QQ:13721218 请注明“读者” QQ群:128659835 请注明“读者” 2018-10 我的系列文档 编程语言 Netkiller Architect 手札 Netkill...
- 下一篇
一句话搞定高仿ios底部弹出提示框(Android)
最近项目里面用到了底部的弹出提示框,UI小姐姐本着设计样式还是ios的好看原则。设计了一个ios样式的底部弹出提示框。OK OK anyway,类似样式并不少见,实现方式有很多,网上随便找一个吧,还不满大街都是。嗯哼,确实不少。但是 !!! 不是讲代码就是讲布局,或者使用方法挺麻烦。 用的时候还要自己手写这部分代码,麻烦不麻烦?作为一名注定要改变世界的程序猿,你让我天天写这个?这是不能忍的。就没有简单的,快捷的,高效的,一句话就能搞定的吗? 有需求就有产品,所以,这个BottomMenu产生了。GitHub项目地址 先来看下效果: How to use: Step 1. Add the JitPack repository to your build fileAdd it in your root build.gradle at the end of repositories: allprojects { repositories { ... maven { url 'https://jitpack.io' } } } Step 2. Add the dependency depend...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Eclipse初始化配置,告别卡顿、闪退、编译时间过长
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- Windows10,CentOS7,CentOS8安装Nodejs环境
- 设置Eclipse缩进为4个空格,增强代码规范
- CentOS7编译安装Cmake3.16.3,解决mysql等软件编译问题
- CentOS7设置SWAP分区,小内存服务器的救世主
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- Linux系统CentOS6、CentOS7手动修改IP地址
- Docker安装Oracle12C,快速搭建Oracle学习环境
- Windows10,CentOS7,CentOS8安装MongoDB4.0.16