Android中的异步处理技术之IntentService
目录
定义
IntentService为Service的子类,它能够进行耗时任务。
原理
IntentService具有和Service一样的生命周期,同时提供了在后台线程中处理异步任务的机制,与HandlerThread类似,IntentService也是在一个后台线程中顺序执行所有任务,我们通过给Context.startService传递一个Intent类型的参数可以启动IntentService的异步执行,如果此时IntentService正在运行中,那么这个新的Intent将会进入队列进行排队,直到后台线程处理完队列前面的任务;如果此时IntentService没有在运行,那么将会启动一个新的IntentService,当后台线程队列中所有的任务处理完之后,IntentService将会结束它的生命周期,因此IntentService不需要开发者手动结束。
实现
IntentService是一个抽象类因此我们需要继承IntentService并实现它的onHandleIntent方法,并在这个方法中实现后台处理的业务逻辑,同时在子类的构造方法中需要调用super(String name),传入一个名称。
public class MyIntentService extends IntentService { /** * Creates an IntentService. Invoked by your subclass's constructor. * * @param name Used to name the worker thread, important only for debugging. */ public MyIntentService() { super("MyIntentService"); mLocalBroadcastManager=LocalBroadcastManager.getInstance(this); setIntentRedelivery(true); } @Override protected void onHandleIntent(@Nullable Intent intent) { //执行后台任务 } }
上面代码中的setIntentRedelivery方法如果设置为true,那么IntentService的onStartCommand方法将会返回START_REDELIVER_INTENT。这时如果onHandleIntent方法返回之前进程死了,那么进程将会重新启动,intent将会重新投递。同时类似Service还需要在AndroidManifest.xml文件中注册
<service android:name=".MyIntentService"/>
案例
在IntentService中进行进度条数据的更新,然后通过本地广播在来刷新UI界面。
- MyIntentService代码
public class MyIntentService extends IntentService { /** * Creates an IntentService. Invoked by your subclass's constructor. * * @param name Used to name the worker thread, important only for debugging. */ private int count=0; private boolean isRunning=true; private LocalBroadcastManager mLocalBroadcastManager; public MyIntentService() { super("MyIntentService"); mLocalBroadcastManager=LocalBroadcastManager.getInstance(this); setIntentRedelivery(true); } @Override protected void onHandleIntent(@Nullable Intent intent) { count=0; isRunning=true; while (isRunning){ try { count++; if(count>=100){ isRunning=false; } Thread.sleep(100); sendThreadStatus(count); } catch (InterruptedException e) { e.printStackTrace(); } } } private void sendThreadStatus(int progress) { Intent intent = new Intent(MainActivity.ACTION); intent.putExtra("progress", progress); mLocalBroadcastManager.sendBroadcast(intent); } }
- MainActivity代码
public class MainActivity extends AppCompatActivity { public static final String ACTION="com.itfitness.intentservicedemo.myintentserviceaction"; private LocalBroadcastManager mLocalBroadcastManager; private MyBroadcastReceiver mMyBroadcastReceiver; private TextView tv; private ProgressBar pb; private Button mButton; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); initView(); initBroadcastReceiver(); } private void initView() { mButton = findViewById(R.id.bt); tv = (TextView) findViewById(R.id.tv); pb = (ProgressBar) findViewById(R.id.prb); mButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { //启动IntentService Intent intent=new Intent(MainActivity.this,MyIntentService.class); startService(intent); } }); } private void initBroadcastReceiver() { if (mLocalBroadcastManager==null) { mLocalBroadcastManager=LocalBroadcastManager.getInstance(this); } if(mMyBroadcastReceiver==null){ mMyBroadcastReceiver = new MyBroadcastReceiver(); } IntentFilter intentFilter=new IntentFilter(); intentFilter.addAction(ACTION); mLocalBroadcastManager.registerReceiver(mMyBroadcastReceiver,intentFilter); } @Override protected void onDestroy() { super.onDestroy(); if(mLocalBroadcastManager!=null&&mMyBroadcastReceiver!=null){ mLocalBroadcastManager.unregisterReceiver(mMyBroadcastReceiver); } } public class MyBroadcastReceiver extends BroadcastReceiver{ @Override public void onReceive(Context context, Intent intent) { //根据IntentService中的发送的广播来进行UI更新 if(intent.getAction().equals(ACTION)){ int progress = intent.getIntExtra("progress", 0); if(progress>0&&progress<100){ tv.setText("线程进行中。。。。"); }else if(progress>=100){ tv.setText("线程结束"); } pb.setProgress(progress); } } } }
- MainActivity布局代码
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <Button android:layout_width="wrap_content" android:text="开始线程" android:id="@+id/bt" android:layout_gravity="center" android:layout_height="wrap_content" /> <TextView android:layout_width="wrap_content" android:layout_marginTop="20dp" android:layout_gravity="center" android:id="@+id/tv" android:text="线程未开启" android:textSize="15sp" android:layout_height="wrap_content" /> <ProgressBar android:layout_width="match_parent" android:layout_marginTop="10dp" android:max="100" android:id="@+id/prb" style="@style/Widget.AppCompat.ProgressBar.Horizontal" android:layout_height="wrap_content" /> </LinearLayout>
项目源码:https://github.com/myml666/IntentServiceDemo
个人技术博客:https://myml666.github.io
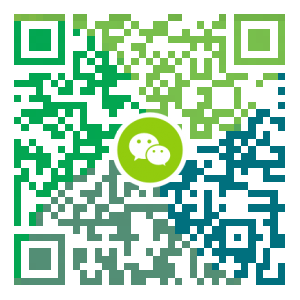
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
CSS-右下角的边框半径 | border-bottom-right-radius
该border-bottom-right-radius属性设置元素右下角的舍入。 /* The corner is a circle */ /* border-bottom-right-radius: radius */ border-bottom-right-radius: 3px; /* Percentage values */ border-bottom-right-radius: 20%; /* corner of a circle if box is a square or else corner of a rectangle */ border-bottom-right-radius: 20% 20%; /* same as above */ /* 20% of horizontal(width) and vertical(height) */ border-bottom-right-radius: 20% 10%; /* 20% of horizontal(width) and 10% of vertical(height) */ /*The corner is an e...
- 下一篇
AndroidStudio笔记(4)编码效率+1 的 File Templates
前言 这一篇是接着 AndroidStudio笔记(3)的,在上一篇中我们使用了 Live Templates的关键字来快速补全和生成代码,那有没有更近一步的骚操作?比如创建文件?答案是肯定的,那就是 File Templates。先看看今天的成果物预览图,如果不感兴趣就可以不看下去。 一键创建 TestViewPagerAdapter 创建TestViewPagerAdapter演示 创建RecyclerViewAdapter 创建RecyclerViewAdapter演示 正文 File Templates 的入口 File Templates 有两个入口。 File -> New -> Edit File Templates Edit File Templates File Templates Settings -> Editor -> File and Code Templates File and Code Templates 简单创建 ViewPagerAdapter File Templates 先来试试水,点击左上角的加号,新建一个模板,名字输入...
相关文章
文章评论
共有0条评论来说两句吧...