Android深度定制化TabLayout:圆角,渐变色,背景边框,圆角渐变下划线,基于Android原生TabLayout
在附录1的基础上丰富自定义的TabLayout,这次增加两个内容:
1, 当选中某一个切换卡时候,文本字体变粗。
2,增加下划线指示器,并且下划线指示器是渐变圆角的。下划线从右往左,从浅蓝变深蓝。
实现效果如图所示:
继承自Android原生TabLayout的MyTabLayout.java:
package zhangphil.test; import android.content.Context; import android.graphics.Color; import android.support.design.widget.TabLayout; import android.text.TextPaint; import android.util.AttributeSet; import android.widget.ImageView; import android.widget.TextView; import java.util.ArrayList; import java.util.List; public class MyTabLayout extends TabLayout { private List<String> titles; public MyTabLayout(Context context) { super(context); init(); } public MyTabLayout(Context context, AttributeSet attrs) { super(context, attrs); init(); } public MyTabLayout(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); init(); } private void init() { titles = new ArrayList<>(); this.addOnTabSelectedListener(new TabLayout.OnTabSelectedListener() { @Override public void onTabSelected(Tab tab) { /** * 设置当前选中的Tab为特殊高亮样式。 */ if (tab != null && tab.getCustomView() != null) { TextView tab_text = tab.getCustomView().findViewById(R.id.tab_text); TextPaint paint = tab_text.getPaint(); paint.setFakeBoldText(true); tab_text.setTextColor(Color.WHITE); tab_text.setBackgroundResource(R.drawable.tablayout_item_pressed); ImageView tab_layout_indicator = tab.getCustomView().findViewById(R.id.tab_indicator); tab_layout_indicator.setBackgroundResource(R.drawable.tablayout_item_indicator); } } @Override public void onTabUnselected(Tab tab) { /** * 重置所有未选中的Tab颜色、字体、背景恢复常态(未选中状态)。 */ if (tab != null && tab.getCustomView() != null) { TextView tab_text = tab.getCustomView().findViewById(R.id.tab_text); tab_text.setTextColor(getResources().getColor(android.R.color.holo_red_light)); TextPaint paint = tab_text.getPaint(); paint.setFakeBoldText(false); tab_text.setBackgroundResource(R.drawable.tablayout_item_normal); ImageView tab_indicator = tab.getCustomView().findViewById(R.id.tab_indicator); tab_indicator.setBackgroundResource(0); } } @Override public void onTabReselected(Tab tab) { } }); } public void setTitle(List<String> titles) { this.titles = titles; /** * 开始添加切换的Tab。 */ for (String title : this.titles) { Tab tab = newTab(); tab.setCustomView(R.layout.tablayout_item); if (tab.getCustomView() != null) { TextView tab_text = tab.getCustomView().findViewById(R.id.tab_text); tab_text.setText(title); } this.addTab(tab); } } }
在onTabSelected中把选中的tab的文本变粗变成白色。同时设置下划线指示器可见。涉及到的下划线res/drawable/tablayout_item_indicator.xml:
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle"> <corners android:radius="50dp" /> <gradient android:angle="180" android:endColor="@android:color/holo_blue_bright" android:startColor="@android:color/holo_blue_dark" android:type="linear" /> </shape>
是一个由右往左渐变的颜色圆角背景shape.
在MyTabLayout的setTitle中,为该切换View增加的res/layout/tablayout_item.xml:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="vertical"> <TextView xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/tab_text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="@drawable/tablayout_item_normal" android:gravity="center" android:paddingBottom="5dp" android:paddingLeft="10dp" android:paddingRight="10dp" android:paddingTop="5dp" android:textColor="@android:color/holo_red_light" android:textSize="14dp"></TextView> <ImageView android:id="@+id/tab_indicator" android:layout_width="match_parent" android:layout_height="5dp" android:layout_gravity="center_horizontal" android:layout_marginTop="5dp" /> </LinearLayout>
res/layout/tablayout_item.xml为每一个添加到切换条上的其中一个选项tab。它使用了默认的(未选中)的背景资源作为未选中时候的背景res/drawable/tablayout_item_normal.xml:
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle"> <corners android:radius="50dp" /> <stroke android:width="1px" android:color="@android:color/darker_gray" /> </shape>
res/drawable/tablayout_item_normal.xml其实是一个简单的有圆角边框的shape,没有选中的tab将以此作为背景资源。 当选中了某一个tab时候,把一个具有颜色到的背景资源文件作为tab的背景衬上去res/drawable/tablayout_item_pressed.xml:
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle"> <corners android:radius="50dp" /> <gradient android:angle="180" android:endColor="@android:color/holo_orange_light" android:startColor="@android:color/holo_red_light" android:type="linear" /> </shape>
这其实就是一个具有橙红色从右往左渐变的圆角背景。 具体使用和Android的原生TabLayout一致,写到xml布局里面:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <zhangphil.test.MyTabLayout android:id="@+id/tab_layout" android:layout_width="match_parent" android:layout_height="100dp" app:tabIndicatorHeight="0dp" app:tabMode="scrollable" /> </LinearLayout>
上层Java代码:
package zhangphil.test; import android.os.Bundle; import android.support.annotation.Nullable; import android.support.v7.app.AppCompatActivity; import java.util.Arrays; import java.util.List; public class TabActivity extends AppCompatActivity { @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.tab_avtivity); String[] str = {"zhang", "phil", "zhang phil", "csdn", "zhang phil csdn", "zhang phil @ csdn", "blog.csdn.net/zhangphil", "android"}; List<String> titles = Arrays.asList(str); MyTabLayout tabLayout = findViewById(R.id.tab_layout); tabLayout.setTitle(titles); } }
代码运行结果就是前文的配图。
附录:1,《Android深度定制化TabLayout:圆角,渐变色,背景边框,基于Android原生TabLayout》链接:https://blog.csdn.net/zhangphil/article/details/80489089
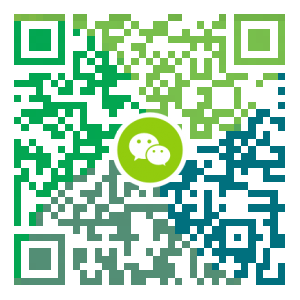
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
Android-解放双手之Gradle自动化打包实战(原创)
本文已独家授权 郭霖 ( guolin_blog) 公众号发布! 前言: 解放双手,双击桌面快捷方式生成apk包,基于Gradle、bat文件让开发人员告别打包烦扰! 前不久有一个Unity3D研发的小仙女在羽毛球场问我关于Android Studio打apk的一些事情,她说他们运营和测试隔三差五的就坐着她旁边要她重复性的打包(估计是那群痴汉打着工作的幌子实际上干着撩妹的活惹火了这位认真敬业的小姐姐),那么有没有省力一点的办法快速打包? 一直以来,对于某些频繁进行打包工作的业务部开发人员来说,打包工作不仅繁琐冗余而且费时费力。如何快速、高效的解决频繁出包以及提高生产效率解放生产力是我们一直在思考的问题。有没有一种办法比如我只想要双击桌面快捷方式然后就打包成APK接着在保存到自定义盘符路径?答案是有的。我们知道Android Studio是基于Gradle来进行快速构建项目,Gradle本质是一个基于Apache Ant和Apache Maven概念的项目自动化构建工具。 由于一些论坛上的文章是基于Android2.X版本来进行解释说明但是笔者通过这几天的实战(Android Studi...
- 下一篇
Android 实现圆角布局,变相实现圆角图片效果(不同位置不同弧度)
小菜最近在处理图片的圆角,不止是四个角全是圆角,还包括单左侧/单右侧/对角线方向的圆角。因为自己太菜只能寻求网上的大神,发现一个自定义圆角布局,这样可以变相的解决我的需求,还可以实现更多的圆角效果,不仅是图片,还包括其他布局。 小菜我作为伸手党,非常感谢大神的分享,参考原文 RoundAngleFrameLayout。 这个布局实现方式很简单,大神只提供了默认的四个圆角,这里我添加了一些方法可以动态的设置圆角的位置与弧度,并说明一下小菜遇到的小问题。小菜根据大神的总结自定义了一个 MyRoundLayout GitHub 布局样式。 Tips: 在设置完角度之后,要添加 invalidate() 刷新 UI,才可以进行动态设置; 自定义的布局样式继承的 FrameLayout,所以设置在需要进行圆角的控件外即可,并不影响其内部控件的样式; 既然 MyRoundLayout 继承的是 FrameLayout,则应遵循 FrameLayout 的特点,内部不能直接设置控件的权重,可在内部添加一层 Layout 布局,在进行权重 weight 的处理; 在使用 MyRoundLayout 时...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- SpringBoot2整合Thymeleaf,官方推荐html解决方案
- CentOS7设置SWAP分区,小内存服务器的救世主
- CentOS8编译安装MySQL8.0.19
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- Docker安装Oracle12C,快速搭建Oracle学习环境
- Linux系统CentOS6、CentOS7手动修改IP地址
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- SpringBoot2初体验,简单认识spring boot2并且搭建基础工程
- Hadoop3单机部署,实现最简伪集群