spring boot整合shiro
一、需要的依赖包
<!--shiro的版本--> <properties> <org.apache.shiro.version>1.3.2</org.apache.shiro.version> </properties> <!-- shiro --> <dependency> <groupId>org.apache.shiro</groupId> <artifactId>shiro-core</artifactId> <version>${org.apache.shiro.version}</version> /dependency> <dependency> <groupId>org.apache.shiro</groupId> <artifactId>shiro-web</artifactId> <version>${org.apache.shiro.version}</version> </dependency> <dependency> <groupId>org.apache.shiro</groupId> <artifactId>shiro-spring</artifactId> <version>${org.apache.shiro.version}</version> </dependency> <dependency> <groupId>org.apache.shiro</groupId> <artifactId>shiro-ehcache</artifactId> <version>${org.apache.shiro.version}</version> </dependency>
二、添加ehcache
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE xml> <ehcache updateCheck="false" name="shiroCache"> <!-- http://ehcache.org/ehcache.xml --> <defaultCache maxElementsInMemory="10000" eternal="false" timeToIdleSeconds="120" timeToLiveSeconds="120" overflowToDisk="false" diskPersistent="false" diskExpiryThreadIntervalSeconds="120" /> <!-- 登录记录缓存 锁定10分钟 --> <cache name="passwordRetryCache" maxEntriesLocalHeap="2000" eternal="false" timeToIdleSeconds="600" timeToLiveSeconds="1800" overflowToDisk="false" statistics="true"> </cache> <cache name="authorizationCache" maxEntriesLocalHeap="2000" eternal="false" timeToIdleSeconds="3600" timeToLiveSeconds="0" overflowToDisk="false" statistics="true"> </cache> <cache name="authenticationCache" maxEntriesLocalHeap="2000" eternal="false" timeToIdleSeconds="3600" timeToLiveSeconds="0" overflowToDisk="false" statistics="true"> </cache> <cache name="shiro-activeSessionCache" maxEntriesLocalHeap="2000" eternal="false" timeToIdleSeconds="3600" timeToLiveSeconds="0" overflowToDisk="false" statistics="true"> </cache> </ehcache>
三、创建一个realm
public class ShiroRealm extends AuthorizingRealm { @Autowired private UserBiz biz; @Override protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principals) { //获取用户权限 SimpleAuthorizationInfo authorizationInfo = new SimpleAuthorizationInfo(); authorizationInfo.setRoles(角色集合); authorizationInfo.setStringPermissions(权限集合); return authorizationInfo; } @Override protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken token) throws AuthenticationException { return new SimpleAuthenticationInfo(获取到的用户账号, 获取到的用户密码, ByteSource.Util.bytes(user.getUserNo() + Constants.token.salt), getName()); } }
四、添加验证器
public class PlatFormCredentialsMatcher extends HashedCredentialsMatcher { @Autowired private UserService service; @Autowired private EhCacheManager shiroEhcacheManager; @Override public boolean doCredentialsMatch(AuthenticationToken token, AuthenticationInfo info) { Cache<String, AtomicInteger> passwordRetryCache = shiroEhcacheManager.getCache("passwordRetryCache"); String userno = (String) token.getPrincipal(); // retry count + 1 AtomicInteger retryCount = passwordRetryCache.get(userno); if (retryCount == null) { retryCount = new AtomicInteger(0); passwordRetryCache.put(userno, retryCount); } if (retryCount.incrementAndGet() > 5) { // if retry count > 5 throw throw new ExcessiveAttemptsException(); } boolean matches = super.doCredentialsMatch(token, info); if (matches) { // clear retry count passwordRetryCache.remove(userno); Result<User> userResult = service.findByUserNo(userno); // 根据登录名查询用户 Subject subject = SecurityUtils.getSubject(); Session session = subject.getSession(); session.setAttribute("user", userResult.getResultData()); } return matches; } }
五、添加shiro配置
@Configuration public class ShiroConfiguration { private static Map<String, String> filterChainDefinitionMap = new LinkedHashMap<String, String>(); @Bean(name = "ShiroRealm") public ShiroRealm getShiroRealm(@Qualifier("credentialsMatcher") CredentialsMatcher matcher) { ShiroRealm shiroRealm = new ShiroRealm(); shiroRealm.setCredentialsMatcher(matcher); return shiroRealm; } @Bean(name = "shiroEhcacheManager") public EhCacheManager getEhCacheManager() { EhCacheManager em = new EhCacheManager(); em.setCacheManagerConfigFile("classpath:ehcache/ehcache-shiro.xml"); return em; } @Bean(name="credentialsMatcher") public PlatFormCredentialsMatcher getCredentialsMatcher(){ PlatFormCredentialsMatcher platFormCredentialsMatcher = new PlatFormCredentialsMatcher(); platFormCredentialsMatcher.setHashAlgorithmName("MD5"); platFormCredentialsMatcher.setHashIterations(2); platFormCredentialsMatcher.setStoredCredentialsHexEncoded(true); return platFormCredentialsMatcher; } @Bean(name = "lifecycleBeanPostProcessor") public LifecycleBeanPostProcessor getLifecycleBeanPostProcessor() { return new LifecycleBeanPostProcessor(); } @Bean public DefaultAdvisorAutoProxyCreator getDefaultAdvisorAutoProxyCreator() { DefaultAdvisorAutoProxyCreator daap = new DefaultAdvisorAutoProxyCreator(); daap.setProxyTargetClass(true); return daap; } @Bean(name = "securityManager") public DefaultWebSecurityManager getDefaultWebSecurityManager(@Qualifier("ShiroRealm") ShiroRealm shiroRealm) { DefaultWebSecurityManager dwsm = new DefaultWebSecurityManager(); dwsm.setRealm(shiroRealm); dwsm.setCacheManager(getEhCacheManager()); return dwsm; } @Bean public AuthorizationAttributeSourceAdvisor getAuthorizationAttributeSourceAdvisor(@Qualifier("securityManager")DefaultWebSecurityManager dwsm) { AuthorizationAttributeSourceAdvisor aasa = new AuthorizationAttributeSourceAdvisor(); aasa.setSecurityManager(dwsm); return new AuthorizationAttributeSourceAdvisor(); } @Bean(name = "shiroFilter") public ShiroFilterFactoryBean getShiroFilterFactoryBean(@Qualifier("securityManager")DefaultWebSecurityManager dwsm) { ShiroFilterFactoryBean shiroFilterFactoryBean = new ShiroFilterFactoryBean(); shiroFilterFactoryBean .setSecurityManager(dwsm); shiroFilterFactoryBean.setLoginUrl("/login"); shiroFilterFactoryBean.setSuccessUrl("/admin/index"); shiroFilterFactoryBean.setUnauthorizedUrl("/login"); filterChainDefinitionMap.put("/login", "authc"); filterChainDefinitionMap.put("/BJUI/**", "anon"); filterChainDefinitionMap.put("/platform/**", "anon"); filterChainDefinitionMap.put("/admin/course/category/list", "perms[user:view]"); filterChainDefinitionMap.put("/admin/course/category/edit", "perms[user:update]"); filterChainDefinitionMap.put("/admin/course/category/update", "perms[user:update]"); filterChainDefinitionMap.put("/admin/course/category/add", "perms[user:add]"); filterChainDefinitionMap.put("/admin/**", "anon"); shiroFilterFactoryBean .setFilterChainDefinitionMap(filterChainDefinitionMap); return shiroFilterFactoryBean; } /** * FilterRegistrationBean * @return */ @Bean public FilterRegistrationBean filterRegistrationBean() { FilterRegistrationBean filterRegistration = new FilterRegistrationBean(); filterRegistration.setFilter(new DelegatingFilterProxy("shiroFilter")); filterRegistration.setEnabled(true); filterRegistration.addUrlPatterns("/*"); filterRegistration.setDispatcherTypes(DispatcherType.REQUEST); return filterRegistration; } }
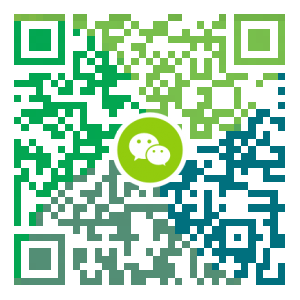
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
nginx解析漏洞允许缓存投毒攻击
许多nginx用户会使用Google 公共 DNS,OpenDNS 或ISP 的解析器解析器等解析程序指令来配置 nginx,但是这当中存在很大的风险,唯一安全的选择是在本地主机上运行一个解析器。我发现,不仅 nginx 的存根 (stub) 解析器会生成非随机的或可预见的 DNS txids,连每个 nginx 的工作进程都会在每个 DNS 查询中使用相同的 UDP 源端口。 只要使用一个简短的脚本就可以在Linux (GNU C 库) 中预测Nginx 的 txids。 这些缺陷允许恶意攻击者发送欺骗性的 DNS 回复去感染解析程序缓存,导致 nginx 代理 HTTP 会向任何攻击者指定的上游服务器发送请求,从而访问到浏览器的恶意内容。 奇怪的是 nginx 开发人员并不认为这存在任何安全问题。在我向 security-@nginx.org 报告之后,他们只修补一部分问题并且拒绝修补其他问题,也没有发布安全咨询。所以我觉得必须要公开我的调查结果,告知nginx 用户他们所面临的潜在风险 。 细节 Nginx 生成 DNS txids所使用的是用于在 Linux/Unix 上ran...
- 下一篇
Spring Boot中使用MongoDB数据库
这一篇介绍Spring Boot中使用MongoDB数据库,需要springboot实战完整视频教程的,点击这里! MongoDB是一个开源的NoSQL文档数据库,它使用一个JSON格式的模式(schema)替换了传统的基于表的关系数据。Spring Boot为使用MongoDB提供了很多便利,包括spring-boot-starter-data-mongodb 'Starter POM'。 引入spring-boot-starter-data-mongodb包,在pom.xml配置文件中增加如下内容(基于之前章节“Spring Boot 构建框架”中的pom.xml文件): <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-mongodb</artifactId></dependency> 注入一个自动配置的org.springframework.data.mongodb....
相关文章
文章评论
共有0条评论来说两句吧...