基于dubbo构建分布式项目与服务模块
Dubbo项目实战视频参考:
http://www.roncoo.com/course/view/f614343765bc4aac8597c6d8b38f06fd
关于分布式服务架构的背景和需求可查阅http://dubbo.io/。不同于传统的单工程项目,本文主要学习如何通过maven和dubbo将构建分布项目以及服务模块,下面直接开始。
创建项目以及模块
创建Maven Project —— mcweb,这个是所有模块的父模块,packaging类型为pom
创建Maven Module —— mcweb-api,这个模块是服务接口层,packaging类型为jar
创建Maven Module —— mcweb-logic,这个是服务实现层或者服务提供者,packaging类型为jar
创建Maven Module —— mcweb-web,这个是web层或者服务消费者,packaging类型为war
我们将以这个简单的样例工程开启分布式开发学习之旅。
配置模块依赖关系
mcweb-service依赖mcweb-api
/mcweb-service/pom.xml
<dependencies>
<dependency>
<groupId>com.edu.mcweb.api</groupId>
<artifactId>mcweb-api</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
mcweb-web 依赖mcweb-api
/mcweb-web/pom.xml
<dependencies>
<dependency>
<groupId>com.edu.mcweb.api</groupId>
<artifactId>mcweb-api</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
<dependency>
<groupId>com.edu.mcweb.service</groupId>
<artifactId>mcweb-service</artifactId>
<version>0.0.1-SNAPSHOT</version>
</dependency>
</dependencies>
模块配置与实现
mcweb-api
mcweb-api层是服务接口层,它的作用是向其他层暴露接口,不需要做其他配置,只需编写我们需要的接口即可,这是面向接口编程的很好例子。
\mcweb\mcweb-api\src\main\java\com\mcweb\api\dao\IUserDao.java
public interface IUserDao {
public User query(Class<User> clazz,Object userId);
}
\mcweb\mcweb-api\src\main\java\com\mcweb\api\service\IUserService.java
public interface IUserService {
public User query(String userId);
}
\mcweb\mcweb-api\src\main\java\com\mcweb\api\entity\User.java
public class User2 implements Serializable{
private String id;
private String userName;
private String userChName;
//省略getter,setter
}
mcweb-logic
模块配置
整个服务模块就是一个web工程,其配置和普通web工程一样,就多了一个dubbo-provider.xml,在applicationContext.xml引入即可(下面将介绍)。这些配置可以根据自己所采用的整合技术进行配置,在此略。
编写代码
\mcweb\mcweb-logic\src\main\java\com\mcweb\logic\dao\UserDao.java
@Repository
public class UserDao implements IUserDao {
@PersistenceContext
protected EntityManager em;
public User query(Class<User> clazz,Object userId) {
//连接数据库的方式
//return em.find(clazz, userId);
User u = new User();
u.setUserName("zhansan");
u.setUserChName("张三");
return u;
}
}
\mcweb\mcweb-logic\src\main\java\com\mcweb\logic\service\UserService.java
@Service
@Transactional
public class UserService implements IUserService {
@Autowired
private IUserDao userDao;
public User query(String userId) {
return userDao.query(User.class, userId);
}
}
部署启动测试
mcweb-logic也是web项目,可以先部署到tomcat运行看是否能正常启动。
mcweb-web
模块配置
同理,整个消费模块也是一个web工程,其配置和普通web工程一样,就多了一个dubbo-consumer.xml,在applicationContext.xml引入即可(下面将介绍)。这些配置可以根据自己所采用的整合技术进行配置,在此略。
编写代码
\mcweb\mcweb-web\src\main\java\com\mcweb\web\controller\UserController.java
@Controller
@RequestMapping(value="/user")
public class UserController {
@Autowired
private IUserService userService;
@RequestMapping(value="/query",method = RequestMethod.GET)
public String query(@RequestParam("userId") String userId,Model model) {
User user = userService.query(userId);
model.addAttribute("user", user);
return "/user.jsp";
}
}
\mcweb\mcweb-web\src\main\webapp\WEB-INF\pages\user.jsp
<body>
${user.userName}
</body>
部署启动测试
Caused by: org.springframework.beans.factory.NoSuchBeanDefinitionException: No qualifying bean of type [com.mcweb.api.service.IUserService] found for dependency: expected at least 1 bean which qualifies as autowire candidate for this dependency.
现在,mcweb-logic和mcweb-web已经配置完成,mcweb-logic能正常启动,mcweb-web依赖mcweb-logic中的IUserService服务,如何让mcweb-web能够引用mcweb-logic中的服务将是下面我们要学习的内容。
模块服务化
服务提供者和服务消费者是dubbo架构中两个主要角色,在上面的项目中,mcweb-logic就相当于服务提供者,mcweb-web相当于服务消费者。Dubbo 建议使用 Zookeeper 作为服务的注册中心,所以我们先安装Zookeeper。只要mcweb-logic向Zookeeper注册了服务之后,它就可以向mcweb-web提供依赖服务;同理,mcweb-web需要向Zookeeper申请需要引用mcweb-logic服务。
安装Zookeeper
略
配置服务提供者
引入相关jar
需要引入dubbo和zookeeper相关jar,\mcweb\pom.xml
<!-- dubbo start -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>dubbo</artifactId>
<version>2.5.3</version>
</dependency>
<dependency>
<groupId>org.apache.zookeeper</groupId>
<artifactId>zookeeper</artifactId>
<version>3.4.9</version>
</dependency>
<dependency>
<groupId>com.101tec</groupId>
<artifactId>zkclient</artifactId>
<version>0.7</version>
</dependency>
<dependency>
<groupId>org.jboss.netty</groupId>
<artifactId>netty</artifactId>
<version>3.2.5.Final</version>
</dependency>
<!-- dubbo end -->
\mcweb\mcweb-logic\pom.xml
<dependency>
<groupId>org.jboss.netty</groupId>
<artifactId>netty</artifactId>
<version>3.2.5.Final</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>dubbo</artifactId>
<version>2.5.3</version>
<exclusions>
<exclusion>
<groupId>org.springframework</groupId>
<artifactId>spring</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.apache.zookeeper</groupId>
<artifactId>zookeeper</artifactId>
<version>3.4.9</version>
</dependency>
<dependency>
<groupId>com.101tec</groupId>
<artifactId>zkclient</artifactId>
<version>0.7</version>
</dependency>
新建dubbo-provider.xml
\mcweb\mcweb-logic\src\main\resources\spring\dubbo-provider.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://code.alibabatech.com/schema/dubbo
http://code.alibabatech.com/schema/dubbo/dubbo.xsd">
<!-- 提供方应用信息,用于计算依赖关系 -->
<dubbo:application name="mcweb-logic" />
<!-- zookeeper注册中心地址 -->
<dubbo:registry protocol="zookeeper" address="192.168.2.129:2181" />
<!-- 用dubbo协议在20880端口暴露服务 -->
<dubbo:protocol name="dubbo" port="20880" />
<!-- 服务接口 -->
<dubbo:service interface="com.mcweb.api.service.IUserService" ref="userService" />
</beans>
在\mcweb\mcweb-logic\src\main\resources\spring\applicationContext.xml引入
dubbo-provider.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-3.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.0.xsd" >
<!-- 注解驱动 -->
<context:annotation-config />
<!-- 包扫描 -->
<context:component-scan base-package="com.mcweb.logic" />
<import resource="dubbo-provider.xml" />
</beans>
使用tomcat运行服务提供者
将mcweb-logic部署到tomcat中测试运行,可以看到tomcat控制台输出信息
11:14:06 [localhost-startStop-1-SendThread(192.168.2.129:2181)]
INFO <org.apache.zookeeper.ClientCnxn> Opening socket connection to server
192.168.2.129/192.168.2.129:2181.
Will not attempt to authenticate using SASL (unknown error)
11:14:06 [localhost-startStop-1-SendThread(192.168.2.129:2181)]
INFO <org.apache.zookeeper.ClientCnxn>
Socket connection established to 192.168.2.129/192.168.2.129:2181, initiating session
11:14:06 [localhost-startStop-1-SendThread(192.168.2.129:2181)]
INFO <org.apache.zookeeper.ClientCnxn>
Session establishment complete on server 192.168.2.129/192.168.2.129:2181, sessionid =
0x1572b87bcd30000, negotiated timeout = 30000
11:14:06 [localhost-startStop-1-EventThread]
INFO <org.I0Itec.zkclient.ZkClient> zookeeper state changed (SyncConnected)
而在zookeeper的后台日志信息中可以看到
[myid:] - INFO [ProcessThread(sid:0 cport:2181)::PrepRequestProcessor@649]
- Got user-level KeeperException when processing sessionid:0x1572b87bcd30000
type:create cxid:0x6 zxid:0x7 txntype:-1 reqpath:n/a Error
Path:/dubbo/com.mcweb.api.service.IUserService Error:KeeperErrorCode =
NodeExists for /dubbo/com.mcweb.api.service.IUserService
可见,mcweb-logic的IUserService服务已经注册到了zookeeper中。
配置服务消费者
引入相关jar
mcweb\mcweb-web\pom.xml 和mcweb-logic引入的一样
新建dubbo-consumer.xml
\mcweb\mcweb-web\src\main\resources\spring\dubbo-consumer.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://code.alibabatech.com/schema/dubbo
http://code.alibabatech.com/schema/dubbo/dubbo.xsd">
<!-- 消费者应用名,不要与提供者一样 -->
<dubbo:application name="mcweb-web" />
<!-- 注册中心地址 -->
<dubbo:registry protocol="zookeeper" address="192.168.2.129:2181" />
<!-- 用户服务接口,check=false表示服务不启动消费者照样能启动 -->
<dubbo:reference interface="com.mcweb.api.service.IUserService" id="userService"
check="false" />
</beans>
在\mcweb\mcweb-web\src\main\resources\spring\applicationContext.xml
中引入dubbo-consumer.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-3.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.0.xsd" >
<!-- 注解驱动 -->
<context:annotation-config />
<!-- 包扫描 -->
<context:component-scan base-package="com.mcweb.web.controller" />
<import resource="dubbo-consumer.xml" />
</beans>
使用tomcat运行服务消费者
再次将mcweb-web部署到tomcat中测试运行,可以看到tomcat控制台输出信息
INFO <org.apache.zookeeper.ClientCnxn>
Opening socket connection to server 192.168.2.129/192.168.2.129:2181.
Will not attempt to authenticate using SASL (unknown error)
INFO <org.apache.zookeeper.ClientCnxn>
Socket connection established to 192.168.2.129/192.168.2.129:2181, initiating session
INFO <org.apache.zookeeper.ClientCnxn>
Session establishment complete on server 192.168.2.129/192.168.2.129:2181,
sessionid = 0x1572b87bcd30001, negotiated timeout = 30000
INFO <org.I0Itec.zkclient.ZkClient> zookeeper state changed (SyncConnected)
可见mcweb-web能正常引用IUserService服务了。
部署测试
http://localhost:8090/mcweb-web/user/query.html?userId=aaaa
可见访问成功,至此,服务提供者和服务消费者已经配置成功。
dubbo管控台的安装
略
服务管理
dubbo 管控台可以对注册到 zookeeper 注册中心的服务或服务消费者进行管理,但 管控台是否正常对 Dubbo 服务没有影响。在dubbo的“首页 > 服务治理 > 服务”中可以看到已经注册到 zookeeper 注册中心的服务的相关情况。
小结
(1)通过maven构建分布式应用的基础模块并配置模块之间的依赖关系,大型的分布式应用通常根据业务需求划分很多模块,每一个模块就是一个工程。这样划分好处很多,比如,抽象出系统的基础服务模块,基础配置模块,基础服务模块,基础配置模为其他模块提供支撑;模块与模块之间独立开发测试,上线维护等等。可见,分布式架构给系统带来了很多灵活性。
(2)通过引入dubbo,将模块服务化。模块服务化通常将模块配置成服务提供者服务消费者,需要注意的是,服务提供者也可以作为服务消费者,因为服务提供者还有可能依赖于其他服务。记住下面这几个容易混淆服务化的标签
<!--将模块配置成服务提供者,通过ref属性将spring容器中的userService bean暴露成dubbo服务-->
<dubbo:service interface="com.mcweb.api.service.IUserService" ref="userService" />
<!--将模块配置成服务消费者,生成远程服务代理,通过id属性,可以和本地bean一样使用userService
可以check="false" 表示不检查服务是否启动-->
<dubbo:reference interface="com.mcweb.api.service.IUserService" id="userService"
check="false" />
<!--服务提供者缺省值配置-->
<dubbo:provider>
<!--服务消费者缺省值配置-->
<dubbo:consumer>
(3)zookeeper作为第三方服务协调中心,服务提供者向其注册服务,服务消费者向其申请引用服务。
(4)本文中采用tomcat容器运行dubbo服务,实际上运行与部署服务有多种方式,后面我们会进一步学习。
更多学习信息请关注:
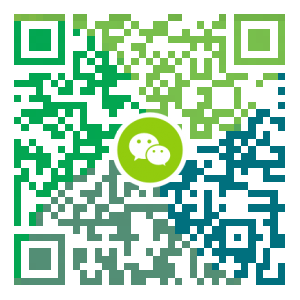
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
基于zabbix 内置key的应用
龙果运维平台开源地址:https://github.com/roncoo/roncoo-cmdb 一、内置key说明: Zabbix 内置了很多丰富的key,使得咱们再添加linux os模板的时候,已经帮我们把key给定义好,这样我们就能够直接链接模板就可以使用了。 我们这边的话列举一些内置key,然后进行一些简单的说明:当我们内置key可以采集到数据的时候我们最好是不用去写自定义key再去采集的:(我见过一篇51 CTO的写监控用户登录数,还用w去监控,没有直接取调用内置key): 二、详情可以查看官方文档: https://www.zabbix.com/documentation/3.0/manual/config/items/itemtypes/zabbix_agent#supported_item_keys 三、内置监控项key列表: agent.hostname 返回被监控端名称(字符串) 使用方式列举:后面使用的方式是一样的: [root@BJ-monitor-h-01 bin]# ./zabbix_get -s 192.168.10.100 -k agent.ho...
- 下一篇
MySQL 事务没有提交导致 锁等待 Lock wait timeout exceeded
java.lang.Exception:### Error updating database. Cause: java.sql.SQLException: Lock wait timeout exceeded; try restarting transaction### The error may involve defaultParameterMap### The error occurred while setting parameters### Cause: java.sql.SQLException: Lock wait timeout exceeded; try restarting transaction; SQL []; Lock wait timeout exceeded; try restarting transaction; nested exception is java.sql.SQLException: Lock wait timeout exceeded; try restarting transaction select * from informati...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS7设置SWAP分区,小内存服务器的救世主
- CentOS7,8上快速安装Gitea,搭建Git服务器
- CentOS7编译安装Gcc9.2.0,解决mysql等软件编译问题
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- CentOS8安装MyCat,轻松搞定数据库的读写分离、垂直分库、水平分库
- Windows10,CentOS7,CentOS8安装Nodejs环境
- CentOS7,CentOS8安装Elasticsearch6.8.6
- CentOS8安装Docker,最新的服务器搭配容器使用
- 设置Eclipse缩进为4个空格,增强代码规范
- Docker快速安装Oracle11G,搭建oracle11g学习环境