Maven工程配置代码覆盖工具Jacoco
本篇博文我们将给出示例理解如何在Maven工程中配置Jacoco和如何使用Jacoco查看代码覆盖报告~
Jacoco是一个开源的Java代码覆盖率工具,Jacoco可以嵌入到Ant 、Maven中,并提供了EclEmma Eclipse插件,也可以使用JavaAgent技术监控Java程序。很多第三方的工具提供了对Jacoco的集成,如sonar、Jenkins等。
Maven工程
创建Maven工程
打开Eclipse,File->New->Project->Maven Project,新建一个Maven工程~
点击“Next”按钮,然后填写groupId和artifactId信息后点击"Finish"按钮即可~
groupId --> com.xxx.tutorial
artifactId --> jacoco-demo
配置Jacoco
添加maven-complier-plugin
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.6.1</version> <configuration> <skipMain>true</skipMain> <skip>true</skip> <source>1.7</source> <target>1.7</target> </configuration> </plugin>
添加jacoco-maven-plugin
<plugin> <groupId>org.jacoco</groupId> <artifactId>jacoco-maven-plugin</artifactId> <version>${jacoco.version}</version> <executions> <execution> <id>prepare-agent</id> <goals> <goal>prepare-agent</goal> </goals> </execution> <execution> <id>report</id> <phase>prepare-package</phase> <goals> <goal>report</goal> </goals> </execution> <execution> <id>post-unit-test</id> <phase>test</phase> <goals> <goal>report</goal> </goals> <configuration> <dataFile>target/jacoco.exec</dataFile> <outputDirectory>target/jacoco-ut</outputDirectory> </configuration> </execution> </executions> <configuration> <systemPropertyVariables> <jacoco-agent.destfile>target/jacoco.exec</jacoco-agent.destfile> </systemPropertyVariables> </configuration> </plugin>
在这里,我们将单元测试结果的输出目录确定为target/jacoco-ut目录下~
完整的pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.xxx.tutorial</groupId> <artifactId>jacoco-demo</artifactId> <version>0.0.1-SNAPSHOT</version> <properties> <jacoco.version>0.7.5.201505241946</jacoco.version> <junit.version>4.12</junit.version> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>${junit.version}</version> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.6.1</version> <configuration> <skipMain>true</skipMain> <skip>true</skip> <source>1.7</source> <target>1.7</target> </configuration> </plugin> <plugin> <groupId>org.jacoco</groupId> <artifactId>jacoco-maven-plugin</artifactId> <version>${jacoco.version}</version> <executions> <execution> <id>prepare-agent</id> <goals> <goal>prepare-agent</goal> </goals> </execution> <execution> <id>report</id> <phase>prepare-package</phase> <goals> <goal>report</goal> </goals> </execution> <execution> <id>post-unit-test</id> <phase>test</phase> <goals> <goal>report</goal> </goals> <configuration> <dataFile>target/jacoco.exec</dataFile> <outputDirectory>target/jacoco-ut</outputDirectory> </configuration> </execution> </executions> <configuration> <systemPropertyVariables> <jacoco-agent.destfile>target/jacoco.exec</jacoco-agent.destfile> </systemPropertyVariables> </configuration> </plugin> </plugins> </build> </project>
编写代码
Calculator.java
package com.xxx.tutorial; /** * * @author wangmengjun * */ public class Calculator { public int add(int a, int b) { return a + b; } public int sub(int a, int b) { return a - b; } }
Calculator_Test.java
package com.xxx.tutorial; import org.junit.Assert; import org.junit.Test; /** * * @author wangmengjun * */ public class Calculator_Test { private Calculator instance = new Calculator(); @Test public void testAdd() { int a = 10; int b = 20; int expected = 30; Assert.assertEquals(expected, instance.add(a, b)); } @Test public void testSub() { int a = 10; int b = 20; int expected = -10; Assert.assertEquals(expected, instance.sub(a, b)); } }
代码结构如下:
运行并查看Jacoco报告
运行Maven test
执行Maven test, 控制台输出如下结果:
[INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building jacoco-demo 0.0.1-SNAPSHOT [INFO] ------------------------------------------------------------------------ [INFO] [INFO] --- jacoco-maven-plugin:0.7.5.201505241946:prepare-agent (prepare-agent) @ jacoco-demo --- [INFO] argLine set to -javaagent:D:\\java_tools\\Reponsitories\\Maven\\org\\jacoco\\org.jacoco.agent\\0.7.5.201505241946\\org.jacoco.agent-0.7.5.201505241946-runtime.jar=destfile=F:\\JavaDeveloper\\workspaces\\SpringMVCDubboExample\\jacoco-demo\\target\\jacoco.exec [INFO] [INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ jacoco-demo --- [WARNING] Using platform encoding (GBK actually) to copy filtered resources, i.e. build is platform dependent! [INFO] Copying 0 resource [INFO] [INFO] --- maven-compiler-plugin:3.6.1:compile (default-compile) @ jacoco-demo --- [INFO] Not compiling main sources [INFO] [INFO] --- maven-resources-plugin:2.6:testResources (default-testResources) @ jacoco-demo --- [WARNING] Using platform encoding (GBK actually) to copy filtered resources, i.e. build is platform dependent! [INFO] Copying 0 resource [INFO] [INFO] --- maven-compiler-plugin:3.6.1:testCompile (default-testCompile) @ jacoco-demo --- [INFO] Not compiling test sources [INFO] [INFO] --- maven-surefire-plugin:2.12.4:test (default-test) @ jacoco-demo --- [INFO] Surefire report directory: F:\JavaDeveloper\workspaces\SpringMVCDubboExample\jacoco-demo\target\surefire-reports ------------------------------------------------------- T E S T S ------------------------------------------------------- Running com.xxx.tutorial.Calculator_Test Tests run: 2, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.134 sec Results : Tests run: 2, Failures: 0, Errors: 0, Skipped: 0 [INFO] [INFO] --- jacoco-maven-plugin:0.7.5.201505241946:report (post-unit-test) @ jacoco-demo --- [INFO] Analyzed bundle 'jacoco-demo' with 1 classes [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 4.972 s [INFO] Finished at: 2017-06-16T21:02:30+08:00 [INFO] Final Memory: 15M/244M [INFO] ------------------------------------------------------------------------
我们可以看到target目录下,已经生成了Jacoco的单元测试结果报告~
查看Jacoco报告
打开浏览器,在URL栏输入
点击"com.xxx.tutorial"链接,查看这个com.xxx.tutorial包下的类。
再点击"Calculator"链接,展示Calculator类的方法信息~
再点击任何方法的连接,将会出现该类代码覆盖的情况:
绿色的表示覆盖到的,如果没有覆盖则会用红色背景表示
至此,
在Maven工程中配置Jacoco插件,运行并查看执行报告结果的示例就完成了~
另外,如果Eclipse工程中安装了EclEmma插件,执行测试类,
也能得到相应的结果,如:
文章转自:https://my.oschina.net/wangmengjun/blog/974067
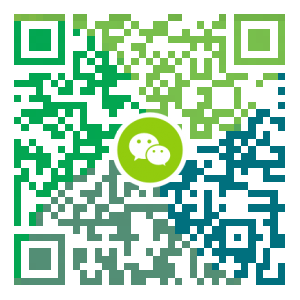
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
深入理解 Java 动态代理机制
Java 有两种代理方式,一种是静态代理,另一种是动态代理。对于静态代理,其实就是通过依赖注入,对对象进行封装,不让外部知道实现的细节。很多 API 就是通过这种形式来封装的。 代理模式结构图(图片来自《大话设计模式》) 下面看下两者在概念上的解释: 静态代理 静态代理类:由程序员创建或者由第三方工具生成,再进行编译;在程序运行之前,代理类的.class文件已经存在了。 静态代理类通常只代理一个类。 静态代理事先知道要代理的是什么。 动态代理 动态代理类:在程序运行时,通过反射机制动态生成。 动态代理类通常代理接口下的所有类。 动态代理事先不知道要代理的是什么,只有在运行的时候才能确定。 动态代理的调用处理程序必须事先InvocationHandler接口,及使用Proxy类中的newProxyInstance方法动态的创建代理类。 Java动态代理只能代理接口,要代理类需要使用第三方的CLIGB等类库。 动态代理的好处 Java动态代理的优势是实现无侵入式的代码扩展,也就是方法的增强;让你可以在不用修改源码的情况下,增强一些方法;在方法的前后你可以做你任何想做的事情(甚至不去执行这个...
- 下一篇
Rabbitmq集群高可用测试
Rabbitmq集群高可用 RabbitMQ是用erlang开发的,集群非常方便,因为erlang天生就是一门分布式语言,但其本身并不支持负载均衡。 Rabbit模式大概分为以下三种:单一模式、普通模式、镜像模式 单一模式:最简单的情况,非集群模式。 没什么好说的。 普通模式:默认的集群模式。 对于Queue来说,消息实体只存在于其中一个节点,A、B两个节点仅有相同的元数据,即队列结构。 当消息进入A节点的Queue中后,consumer从B节点拉取时,RabbitMQ会临时在A、B间进行消息传输,把A中的消息实体取出并经过B发送给consumer。 所以consumer应尽量连接每一个节点,从中取消息。即对于同一个逻辑队列,要在多个节点建立物理Queue。否则无论consumer连A或B,出口总在A,会产生瓶颈。 该模式存在一个问题就是当A节点故障后,B节点无法取到A节点中还未消费的消息实体。 如果做了消息持久化,那么得等A节点恢复,然后才可被消费;如果没有持久化的话,然后就没有然后了…… 镜像模式:把需要的队列做成镜像队列,存在于多个节点,属于RabbitMQ的HA方案。 该模式解...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- SpringBoot2整合Thymeleaf,官方推荐html解决方案
- CentOS7设置SWAP分区,小内存服务器的救世主
- CentOS8编译安装MySQL8.0.19
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- Docker安装Oracle12C,快速搭建Oracle学习环境
- Linux系统CentOS6、CentOS7手动修改IP地址
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- SpringBoot2初体验,简单认识spring boot2并且搭建基础工程
- Hadoop3单机部署,实现最简伪集群