自制一个 elasticsearch-spring-boot-starter
概 述
Elasticsearch 在企业里落地的场景越来越多了,但是大家在项目里使用 Elasticsearch的姿势也是千奇百怪,这次正好自己需要使用,所以干脆就封装一个 elasticsearch-spring-boot-starter以供复用好了。如果不知道 spring-boot-starter该如何制作,可以参考文章《如何自制一个Spring Boot Starter并推送到远端公服》,下面就来简述一下自制的 elasticsearch-spring-boot-starter该如何使用。
依赖引入
<dependency> <groupId>com.github.hansonwang99</groupId> <artifactId>elasticsearch-spring-boot-starter</artifactId> <version>0.0.8</version> </dependency> <repositories> <repository> <id>jitpack.io</id> <url>https://jitpack.io</url> </repository> </repositories>
配置文件
如果你还没有一个属于自己的 Elasticsearch集群,可以参考文章 《CentOS7 上搭建多节点 Elasticsearch集群》来一步步搭建之,本文实验所用的集群即来源于此。
elasticsearch: host: 192.168.31.75 httpPort: 9200 tcpPort: 9300 clusterName: codesheep docFields: title,filecontent auth: enable: false
各个字段解释如下:
-
host
:Elasticsearch 节点地址 -
httpPort
: Elasticsearch REST端口 -
tcpPort
:Elasticsearch TCP端口 -
clusterName
:集群名 -
docFields
:文档字段,以英文逗号间隔,比如我这里的业务场景是文档包含标题(title)
和内容(filecontent)
字段 -
auth
:是否需要权限认证
由于我这里安装的实验集群并无 x-pack
权限认证的加持,因此无需权限认证,实际使用的集群或者阿里云上的 Elasticsearch集群均有完善的 x-pack
权限认证,此时可以加上用户名/密码的配置:
elasticsearch: host: 192.168.199.75 httpPort: 9200 tcpPort: 9300 clusterName: codesheep docFields: title,filecontent auth: enable: true username: elasticsearch password: xxxxxx
用法例析
- 首先注入相关资源
@Autowired private ISearchService iSearchService; @Autowired private DocModel docModel;
这些都是在 elasticsearch-spring-boot-starter中定义的
- 创建索引
public String createIndex() throws IOException { IndexModel indexModel = new IndexModel(); indexModel.setIndexName("testindex2"); // 注意索引名字必须小写,否则ES抛异常 indexModel.setTypeName("testtype2"); indexModel.setReplicaNumber( 2 ); // 两个节点,因此两个副本 indexModel.setShardNumber( 3 ); XContentBuilder builder = null; builder = XContentFactory.jsonBuilder(); builder.startObject(); { builder.startObject("properties"); { builder.startObject("title"); { builder.field("type", "text"); builder.field("analyzer", "ik_max_word"); } builder.endObject(); builder.startObject("filecontent"); { builder.field("type", "text"); builder.field("analyzer", "ik_max_word"); builder.field("term_vector", "with_positions_offsets"); } builder.endObject(); } builder.endObject(); } builder.endObject(); indexModel.setBuilder( builder ); Boolean res = iSearchService.createIndex(indexModel); if( true==res ) return "创建索引成功"; else return "创建索引失败"; }
- 删除索引
public String deleteIndex() { return (iSearchService.deleteIndex("testindex2")==true) ? "删除索引成功":"删除索引失败"; }
- 判断索引是否存在
if ( existIndex(indexName) ) { ... } else { ... }
- 插入单个文档
public String insertSingleDoc( ) { SingleDoc singleDoc = new SingleDoc(); singleDoc.setIndexName("testindex2"); singleDoc.setTypeName("testtype2"); Map<String,Object> doc = new HashMap<>(); doc.put("title","人工智能标题1"); doc.put("filecontent","人工智能内容1"); singleDoc.setDocMap(doc); return ( true== iSearchService.insertDoc( singleDoc ) ) ? "插入单个文档成功" : "插入单个文档失败"; }
- 批量插入文档
public String insertDocBatch() { BatchDoc batchDoc = new BatchDoc(); batchDoc.setIndexName("testindex2"); batchDoc.setTypeName("testtype2"); Map<String,Object> doc1 = new HashMap<>(); doc1.put("title","人工智能标题1"); doc1.put("filecontent","人工智能内容1"); Map<String,Object> doc2 = new HashMap<>(); doc2.put("title","人工智能标题2"); doc2.put("filecontent","人工智能内容2"); Map<String,Object> doc3 = new HashMap<>(); doc3.put("title","人工智能标题3"); doc3.put("filecontent","人工智能内容3"); Map<String,Object> doc4 = new HashMap<>(); doc4.put("title","人工智能标题4"); doc4.put("filecontent","人工智能内容4"); List<Map<String,Object>> docList = new ArrayList<>(); docList.add( doc1 ); docList.add( doc2 ); docList.add( doc3 ); docList.add( doc4 ); batchDoc.setBatchDocMap( docList ); return ( true== iSearchService.insertDocBatch( batchDoc ) ) ? "批量插入文档成功" : "批量插入文档失败"; }
- 搜索文档
public List<Map<String,Object>> searchDoc() { SearchModel searchModel = new SearchModel(); searchModel.setIndexName( "testindex2" ); List<String> fields = new ArrayList<>(); fields.add("title"); fields.add("filecontent"); fields.add("id"); searchModel.setFields( fields ); searchModel.setKeyword( "人工" ); searchModel.setPageNum( 1 ); searchModel.setPageSize( 5 ); return iSearchService.queryDocs( searchModel ); }
- 删除文档
public String deleteDoc() { SingleDoc singleDoc = new SingleDoc(); singleDoc.setIndexName("testindex2"); singleDoc.setTypeName("testtype2"); singleDoc.setId("vPHMY2cBcGZ3je_1EgIM"); return (true== iSearchService.deleteDoc(singleDoc)) ? "删除文档成功" : "删除文档失败"; }
- 批量删除文档
public String deleteDocBatch() { BatchDoc batchDoc = new BatchDoc(); batchDoc.setIndexName("testindex2"); batchDoc.setTypeName("testtype2"); List<String> ids = new ArrayList<>(); ids.add("vfHMY2cBcGZ3je_1EgIM"); ids.add("vvHMY2cBcGZ3je_1EgIM"); batchDoc.setDocIds( ids ); return ( true== iSearchService.deleteDocBatch(batchDoc) ) ? "批量删除文档成功" : "批量删除文档失败"; }
- 更新文档
public String updateDoc( @RequestBody SingleDoc singleDoc ) { SingleDoc singleDoc = new SingleDoc(); singleDoc.setId("wPH6Y2cBcGZ3je_1OwI7"); singleDoc.setIndexName("testindex2"); singleDoc.setTypeName("testtype2"); Map<String,Object> doc = new HashMap<>(); doc.put("title","人工智能标题(更新后)"); doc.put("filecontent","人工智能内容(更新后)"); singleDoc.setUpdateDocMap(doc); return (true== iSearchService.updateDoc(singleDoc)) ? "更新文档成功" : "更新文档失败"; }
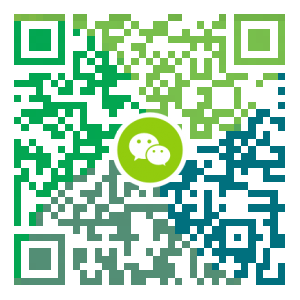
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
Spark2.1.0——内置Web框架详解
Spark2.1.0——内置Web框架详解 任何系统都需要提供监控功能,否则在运行期间发生一些异常时,我们将会束手无策。也许有人说,可以增加日志来解决这个问题。日志只能解决你的程序逻辑在运行期的监控,进而发现Bug,以及提供对业务有帮助的调试信息。当你的JVM进程奔溃或者程序响应速度很慢时,这些日志将毫无用处。好在JVM提供了jstat、jstack、jinfo、jmap、jhat等工具帮助我们分析,更有VisualVM的可视化界面以更加直观的方式对JVM运行期的状况进行监控。此外,像Tomcat、Hadoop等服务都提供了基于Web的监控页面,用浏览器能访问具有样式及布局,并提供丰富监控数据的页面无疑是一种简单、高效的方式。 Spark自然也提供了Web页面来浏览监控数据,而且Master、Worker、Driver根据自身功能提供了不同内容的Web监控页面。无论是Master、Worker,还是Driver,它们都使用了统一的Web框架WebUI。Master、Worker及Driver分别使用MasterWebUI、WorkerWebUI及SparkUI提供的Web界面服务,后三...
- 下一篇
Apache Hadoop 2.7如何支持读写OSS
背景 2017.12.13日Apache Hadoop 3.0.0正式版本发布,默认支持阿里云OSS对象存储系统,作为Hadoop兼容的文件系统,后续版本号大于等于Hadoop 2.9.x系列也支持OSS。然而,低版本的Apache Hadoop官方不再支持OSS,本文将描述如何通过支持包来使Hadoop 2.7.2能够读写OSS。 如何使用 下面的步骤需要在所有的Hadoop节点执行 下载支持包 http://gosspublic.alicdn.com/hadoop-spark/hadoop-oss-2.7.2.tar.gz 解压这个支持包,里面的文件是: [root@apache hadoop-oss-2.7.2]# ls -lh 总用量 3.1M -rw-r--r-- 1 root root 3.1M 2月 28 17:01 hadoo
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Windows10,CentOS7,CentOS8安装MongoDB4.0.16
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- CentOS8安装MyCat,轻松搞定数据库的读写分离、垂直分库、水平分库
- Windows10,CentOS7,CentOS8安装Nodejs环境
- CentOS7设置SWAP分区,小内存服务器的救世主
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- MySQL8.0.19开启GTID主从同步CentOS8
- Jdk安装(Linux,MacOS,Windows),包含三大操作系统的最全安装
- CentOS8编译安装MySQL8.0.19
- Docker安装Oracle12C,快速搭建Oracle学习环境