精通SpringBoot——第三篇:详解WebMvcConfigurer接口
SpringBoot 确实为我们做了很多事情, 但有时候我们想要自己定义一些Handler,Interceptor,ViewResolver,MessageConverter,该怎么做呢。在Spring Boot 1.5版本都是靠重写WebMvcConfigurerAdapter的方法来添加自定义拦截器,消息转换器等。SpringBoot 2.0 后,该类被标记为@Deprecated。因此我们只能靠实现WebMvcConfigurer接口来实现。接下来让我们来看看这个接口类吧!(列举下常用的方法供参考)
package com.developlee.config; import com.alibaba.fastjson.serializer.SerializerFeature; import com.alibaba.fastjson.support.config.FastJsonConfig; import com.alibaba.fastjson.support.spring.FastJsonHttpMessageConverter; import com.developlee.configurer.USLocalDateFormatter; import org.springframework.format.FormatterRegistry; import org.springframework.http.MediaType; import org.springframework.http.converter.HttpMessageConverter; import org.springframework.http.converter.json.JsonbHttpMessageConverter; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.servlet.config.annotation.CorsRegistry; import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry; import org.springframework.web.servlet.config.annotation.WebMvcConfigurer; import org.springframework.web.servlet.config.annotation.WebMvcConfigurerAdapter; import java.time.LocalDate; import java.util.ArrayList; import java.util.List; import java.util.Locale; /** * @TODO // * @Author Lensen * @Date 2018/7/21 * @Description 实现WebMvcConfigurer接口, */ @Configuration public class WebConfig implements WebMvcConfigurer { /** * 添加类型转换器和格式化器 * @param registry */ @Override public void addFormatters(FormatterRegistry registry) { registry.addFormatterForFieldType(LocalDate.class, new USLocalDateFormatter()); } /** * 跨域支持 * @param registry */ @Override public void addCorsMappings(CorsRegistry registry) { registry.addMapping("/**") .allowedOrigins("*") .allowCredentials(true) .allowedMethods("GET", "POST", "DELETE", "PUT") .maxAge(3600 * 24); } /** * 添加静态资源--过滤swagger-api (开源的在线API文档) * @param registry */ @Override public void addResourceHandlers(ResourceHandlerRegistry registry) { //过滤swagger registry.addResourceHandler("swagger-ui.html") .addResourceLocations("classpath:/META-INF/resources/"); registry.addResourceHandler("/webjars/**") .addResourceLocations("classpath:/META-INF/resources/webjars/"); registry.addResourceHandler("/swagger-resources/**") .addResourceLocations("classpath:/META-INF/resources/swagger-resources/"); registry.addResourceHandler("/swagger/**") .addResourceLocations("classpath:/META-INF/resources/swagger*"); registry.addResourceHandler("/v2/api-docs/**") .addResourceLocations("classpath:/META-INF/resources/v2/api-docs/"); } } /** * 配置消息转换器--这里我用的是alibaba 开源的 fastjson * @param converters */ @Override public void configureMessageConverters(List<HttpMessageConverter<?>> converters) { //1.需要定义一个convert转换消息的对象; FastJsonHttpMessageConverter fastJsonHttpMessageConverter = new FastJsonHttpMessageConverter(); //2.添加fastJson的配置信息,比如:是否要格式化返回的json数据; FastJsonConfig fastJsonConfig = new FastJsonConfig(); fastJsonConfig.setSerializerFeatures(SerializerFeature.PrettyFormat, SerializerFeature.WriteMapNullValue, SerializerFeature.WriteNullStringAsEmpty, SerializerFeature.DisableCircularReferenceDetect, SerializerFeature.WriteNullListAsEmpty, SerializerFeature.WriteDateUseDateFormat); //3处理中文乱码问题 List<MediaType> fastMediaTypes = new ArrayList<>(); fastMediaTypes.add(MediaType.APPLICATION_JSON_UTF8); //4.在convert中添加配置信息. fastJsonHttpMessageConverter.setSupportedMediaTypes(fastMediaTypes); fastJsonHttpMessageConverter.setFastJsonConfig(fastJsonConfig); //5.将convert添加到converters当中. converters.add(fastJsonHttpMessageConverter); } @Override public void addInterceptors(InterceptorRegistry registry) { registry.addInterceptor(new ReqInterceptor()).addPathPatterns("/**"); } }
添加一个Interceptor,作为测试
package com.developlee.configurer; import org.springframework.web.servlet.ModelAndView; import org.springframework.web.servlet.handler.HandlerInterceptorAdapter; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; /** * @TODO // * @Author Lensen * @Date 2018/7/21 * @Description 请求Interceptor */ public class ReqInterceptor extends HandlerInterceptorAdapter { @Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { System.out.println("Interceptor preHandler method is running !"); return super.preHandle(request, response, handler); } @Override public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception { System.out.println("Interceptor postHandler method is running !"); super.postHandle(request, response, handler, modelAndView); } @Override public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception { System.out.println("Interceptor afterCompletion method is running !"); super.afterCompletion(request, response, handler, ex); } @Override public void afterConcurrentHandlingStarted(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { System.out.println("Interceptor afterConcurrentHandlingStarted method is running !"); super.afterConcurrentHandlingStarted(request, response, handler); } }
写个controller测试下
@Controller @RequestMapping("/locale") public class LocaleController { @GetMapping("/date") @ResponseBody public String locale(Locale locale){ System.out.println("Controller is running !"); return "Hello" + USLocalDateFormatter.getPattern(locale); } }
请求地址: http://localhost:8080/locale/date ,看看控制台打印信息
//SpringMvc请求处理的执行过程 Interceptor preHandler method is running ! Controller is running ! Interceptor postHandler method is running ! Interceptor afterCompletion method is running !
题外话:从WebMvcConfigurer这个接口中,可以看到JDK8的一些新特性——在接口中新增了default方法和static方法,这两种方法可以有方法体。大家可以业余时间去了解下。这两种新特性为我们编写代码带来了更多改变,让代码变得更加简洁。
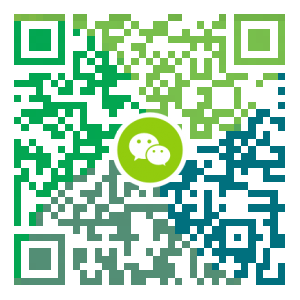
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
Java 学习(20)--异常 / IO 流
异常(Exception) (1)程序出现的不正常的情况。 (2)异常的体系 Throwable(接口,将异常类对象交给 JVM 来处理) |--Error 严重问题,我们不处理。(jvm 错误,程序无法处理) |--Exception异常 (程序可以处理的异常) |--RuntimeException (运行期异常,可以通过逻辑判断进行控制,不需要捕获 处理方法:1.try...catch 2.throws) |--其他(受查)异常 (继承自Exception,且不是RuntimeException,必须要捕获) (3)异常的处理: A:JVM 的默认处理 当系统发生运行异常时,Jvm 会创建一个异常类的对象;查看是否对这个异常进行捕获和处理,若没有,则把异常的名称 ,原因,位置等信息输出在控制台,但是程序不能继续执行了。 B:自己处理 a:try...catch...finally 自己编写处理代码 ,后面的程序可以继续执行 b:throws 把自己处理不了的,在方法上声明,告诉调用者,这里有问题 (4)面试题 A:编译期异常和运行期异常的区别 ? 编译期异常必须要处理的,否则...
- 下一篇
Python里面几种排序算法的比较,sorted的底层实现,虽然我们知道sorted的实现方式,但是却写不出这样的速度
算法与数据结构基础 原文链接:http://note.youdao.com/noteshare?id=7b9757930ce3cc9e0a5e61e4d0aa9ea2⊂=2726FFA02ADE4E74A302D8DA7646FB46 查找算法: 二分查找法: 简介:二分查找法又被称为折半查找法,用于预排序的查找问题 过程: 如果在列表a中查找元素t,先将列表a中间位置的项与查找关键字t比较,如果两者相等,则成功。 否则,将表分为前后两个子表 如果中间位置大于t,则进一步查找前一子表,否则,查找后一子表 重复上述过程 优劣: 时间复杂度为O(log~2~N),比较快 缺点就是必须是有序列表 排序算法: 冒泡排序 简介:两两比较大小,如果不满足升序关系,则交换 过程:略 优劣:: 时间复杂度为O(N^2^),速度较慢 稳定 选择排序 简介:找出最小值,然后放入一个新的列
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Linux系统CentOS6、CentOS7手动修改IP地址
- CentOS8安装Docker,最新的服务器搭配容器使用
- CentOS7安装Docker,走上虚拟化容器引擎之路
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- Docker安装Oracle12C,快速搭建Oracle学习环境
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- CentOS7,8上快速安装Gitea,搭建Git服务器
- MySQL8.0.19开启GTID主从同步CentOS8
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- CentOS8编译安装MySQL8.0.19