【Java小工匠聊密码学】--消息摘要--HMAC算法
1、什么是HMAC
HMAC是密钥相关的消息认证码,HMAC运算利用哈希算法,以一个密钥和一个消息为输入,生成一个消息摘要作为输出。
2、HMAC用途
我们使用MD和SHA 消息摘要算法,可以保证数据的完整性。但是在网络传输场景下,消息发送者,仅发送原始数据和数据摘要信息是,黑客可以伪装原始数据和数据摘要信息,达到攻击的目的,HMAC算法通过密钥和数据共同生成 消息摘要,黑客在不知道密钥的情况下,伪造数据和消息摘要难度进一步加大。
3、HMAC算法分类
算法种类 摘要长度 HmacMD5 128 HmacSHA1 160 HmacSHA256 256 HmacSHA384 384 HmacSHA512 512
4、HMAC算法实现
4.1 JDK 算法实现
package lzf.cipher.jdk; import java.nio.charset.Charset; import javax.crypto.KeyGenerator; import javax.crypto.Mac; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; /** * @author java小工匠 */ public class JdkHmacUtils { // 获取 HmacMD5 Key public static byte[] getHmacMd5Key() { return getHmacKey("HmacMD5"); } // 获取 HmacSHA256 public static byte[] getHmacSha256Key() { return getHmacKey("HmacSHA256"); } // 获取 HmacSHA512 public static byte[] getHmacSha512Key() { return getHmacKey("HmacSHA512"); } // 获取 HMAC Key public static byte[] getHmacKey(String type) { try { // 1、创建密钥生成器 KeyGenerator keyGenerator = KeyGenerator.getInstance(type); // 2、产生密钥 SecretKey secretKey = keyGenerator.generateKey(); // 3、获取密钥 byte[] key = secretKey.getEncoded(); return key; } catch (Exception e) { throw new RuntimeException(e); } } // HMAC MD5 加密 public static String encryptHmacMD5(byte[] data, byte[] key) { return encryptHmac(data, key, "HmacMD5"); } // HMAC SHA256 加密 public static String encryptHmacSHA256(byte[] data, byte[] key) { return encryptHmac(data, key, "HmacSHA256"); } // HMAC SHA521 加密 public static String encryptHmacSHA512(byte[] data, byte[] key) { return encryptHmac(data, key, "HmacSHA512"); } // 基础MAC 算法 public static String encryptHmac(byte[] data, byte[] key, String type) { try { // 1、还原密钥 SecretKey secretKey = new SecretKeySpec(key, type); // 2、创建MAC对象 Mac mac = Mac.getInstance(type); // 3、设置密钥 mac.init(secretKey); // 4、数据加密 byte[] bytes = mac.doFinal(data); // 5、生成数据 String rs = encodeHex(bytes); return rs; } catch (Exception e) { throw new RuntimeException(e); } } // 数据准16进制编码 public static String encodeHex(final byte[] data) { return encodeHex(data, true); } // 数据转16进制编码 public static String encodeHex(final byte[] data, final boolean toLowerCase) { final char[] DIGITS_LOWER = { '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'a', 'b', 'c', 'd', 'e', 'f' }; final char[] DIGITS_UPPER = { '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'A', 'B', 'C', 'D', 'E', 'F' }; final char[] toDigits = toLowerCase ? DIGITS_LOWER : DIGITS_UPPER; final int l = data.length; final char[] out = new char[l << 1]; // two characters form the hex value. for (int i = 0, j = 0; i < l; i++) { out[j++] = toDigits[(0xF0 & data[i]) >>> 4]; out[j++] = toDigits[0x0F & data[i]]; } return new String(out); } public static void main(String[] args) { byte[] data = "java小工匠".getBytes(Charset.forName("UTF-8")); // MD5 byte[] hmacMd5KeyBytes = getHmacMd5Key(); String hexHamcMd5Key = encodeHex(hmacMd5KeyBytes); System.out.println("HMAC Md5 密钥:" + hexHamcMd5Key); String hmacMd5Encrypt = encryptHmacMD5(data, hmacMd5KeyBytes); System.out.println("HMAC Md5 加密:" + hmacMd5Encrypt); // SHA256 byte[] hmacSha256KeyBytes = getHmacSha256Key(); String hexHamcSha256Key = encodeHex(hmacSha256KeyBytes); System.out.println("HMAC SHA256 密钥:" + hexHamcSha256Key); String hmacSha256Encrypt = encryptHmacSHA256(data, hmacSha256KeyBytes); System.out.println("HMAC SHA256 加密:" + hmacSha256Encrypt); // SHA512 byte[] hmacSha512KeyBytes = getHmacSha512Key(); String hexHamcSha512Key = encodeHex(hmacSha512KeyBytes); System.out.println("HMAC SHA512 密钥:" + hexHamcSha512Key); String hmacSha512Encrypt = encryptHmacSHA512(data, hmacSha512KeyBytes); System.out.println("HMAC SHA512 加密:" + hmacSha512Encrypt); } }
4.2 CC 算法实现
package lzf.cipher.cc; import java.nio.charset.Charset; import javax.crypto.KeyGenerator; import javax.crypto.Mac; import javax.crypto.SecretKey; import org.apache.commons.codec.binary.Hex; import org.apache.commons.codec.digest.HmacAlgorithms; import org.apache.commons.codec.digest.HmacUtils; /** * @author java小工匠 */ public class CCHmacUtils { // 获取 HmacMD5 Key public static byte[] getHmacMd5Key() { return getHmacKey("HmacMD5"); } // 获取 HmacSHA256 public static byte[] getHmacSha256Key() { return getHmacKey("HmacSHA256"); } // 获取 HmacSHA512 public static byte[] getHmacSha512Key() { return getHmacKey("HmacSHA512"); } // 获取 HMAC Key public static byte[] getHmacKey(String type) { try { // 1、创建密钥生成器 KeyGenerator keyGenerator = KeyGenerator.getInstance(type); // 2、产生密钥 SecretKey secretKey = keyGenerator.generateKey(); // 3、获取密钥 byte[] key = secretKey.getEncoded(); return key; } catch (Exception e) { throw new RuntimeException(e); } } // HMAC MD5 加密 public static String encryptHmacMD5(byte[] data, byte[] key) { Mac mac = HmacUtils.getInitializedMac(HmacAlgorithms.HMAC_MD5, key); return Hex.encodeHexString(mac.doFinal(data)); } // HMAC SHA256 加密 public static String encryptHmacSHA256(byte[] data, byte[] key) { Mac mac = HmacUtils.getInitializedMac(HmacAlgorithms.HMAC_SHA_256, key); return Hex.encodeHexString(mac.doFinal(data)); } // HMAC SHA521 加密 public static String encryptHmacSHA512(byte[] data, byte[] key) { Mac mac = HmacUtils.getInitializedMac(HmacAlgorithms.HMAC_SHA_512, key); return Hex.encodeHexString(mac.doFinal(data)); } public static void main(String[] args) { byte[] data = "java小工匠".getBytes(Charset.forName("UTF-8")); // MD5 byte[] hmacMd5KeyBytes = getHmacMd5Key(); String hexHamcMd5Key = Hex.encodeHexString(hmacMd5KeyBytes); System.out.println("HMAC Md5 密钥:" + hexHamcMd5Key); String hmacMd5Encrypt = encryptHmacMD5(data, hmacMd5KeyBytes); System.out.println("HMAC Md5 加密:" + hmacMd5Encrypt); // SHA256 byte[] hmacSha256KeyBytes = getHmacSha256Key(); String hexHamcSha256Key = Hex.encodeHexString(hmacSha256KeyBytes); System.out.println("HMAC SHA256 密钥:" + hexHamcSha256Key); String hmacSha256Encrypt = encryptHmacSHA256(data, hmacSha256KeyBytes); System.out.println("HMAC SHA256 加密:" + hmacSha256Encrypt); // SHA512 byte[] hmacSha512KeyBytes = getHmacSha512Key(); String hexHamcSha512Key = Hex.encodeHexString(hmacSha512KeyBytes); System.out.println("HMAC SHA512 密钥:" + hexHamcSha512Key); String hmacSha512Encrypt = encryptHmacSHA512(data, hmacSha512KeyBytes); System.out.println("HMAC SHA512 加密:" + hmacSha512Encrypt); } }
4.3 BC 算法实现
package lzf.cipher.bc; import java.nio.charset.Charset; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; import org.bouncycastle.crypto.digests.MD5Digest; import org.bouncycastle.crypto.digests.SHA256Digest; import org.bouncycastle.crypto.digests.SHA512Digest; import org.bouncycastle.crypto.macs.HMac; import org.bouncycastle.util.encoders.Hex; /** * @author java小工匠 */ public class BCHmacUtils { // 获取 HmacMD5 Key public static byte[] getHmacMd5Key() { return getHmacKey("HmacMD5"); } // 获取 HmacSHA256 public static byte[] getHmacSha256Key() { return getHmacKey("HmacSHA256"); } // 获取 HmacSHA512 public static byte[] getHmacSha512Key() { return getHmacKey("HmacSHA512"); } // 获取 HMAC Key public static byte[] getHmacKey(String type) { try { // 1、创建密钥生成器 KeyGenerator keyGenerator = KeyGenerator.getInstance(type); // 2、产生密钥 SecretKey secretKey = keyGenerator.generateKey(); // 3、获取密钥 byte[] key = secretKey.getEncoded(); return key; } catch (Exception e) { throw new RuntimeException(e); } } // HMAC MD5 加密 public static String encryptHmacMD5(byte[] data, byte[] key) { HMac hmac = new HMac(new MD5Digest()); hmac.update(data, 0, data.length); byte[] rsData = new byte[hmac.getMacSize()]; hmac.doFinal(rsData, 0); return Hex.toHexString(rsData); } // HMAC SHA256 加密 public static String encryptHmacSHA256(byte[] data, byte[] key) { HMac hmac = new HMac(new SHA256Digest()); hmac.update(data, 0, data.length); byte[] rsData = new byte[hmac.getMacSize()]; hmac.doFinal(rsData, 0); return Hex.toHexString(rsData); } // HMAC SHA521 加密 public static String encryptHmacSHA512(byte[] data, byte[] key) { HMac hmac = new HMac(new SHA512Digest()); hmac.update(data, 0, data.length); byte[] rsData = new byte[hmac.getMacSize()]; hmac.doFinal(rsData, 0); return Hex.toHexString(rsData); } public static void main(String[] args) { byte[] data = "java小工匠".getBytes(Charset.forName("UTF-8")); // MD5 byte[] hmacMd5KeyBytes = getHmacMd5Key(); String hexHamcMd5Key = Hex.toHexString(hmacMd5KeyBytes); System.out.println("HMAC Md5 密钥:" + hexHamcMd5Key); String hmacMd5Encrypt = encryptHmacMD5(data, hmacMd5KeyBytes); System.out.println("HMAC Md5 密钥:" + hmacMd5Encrypt); // SHA256 byte[] hmacSha256KeyBytes = getHmacSha256Key(); String hexHamcSha256Key = Hex.toHexString(hmacSha256KeyBytes); System.out.println("HMAC SHA256 密钥:" + hexHamcSha256Key); String hmacSha256Encrypt = encryptHmacSHA256(data, hmacSha256KeyBytes); System.out.println("HMAC SHA256 密钥:" + hmacSha256Encrypt); // SHA512 byte[] hmacSha512KeyBytes = getHmacSha256Key(); String hexHamcSha512Key = Hex.toHexString(hmacSha512KeyBytes); System.out.println("HMAC SHA512 密钥:" + hexHamcSha512Key); String hmacSha512Encrypt = encryptHmacSHA512(data, hmacSha512KeyBytes); System.out.println("HMAC SHA512 密钥:" + hmacSha512Encrypt); } }
如果读完觉得有收获的话,欢迎点赞、关注、加公众号【小工匠技术圈】
个人公众号,欢迎关注,查阅更多精彩历史!
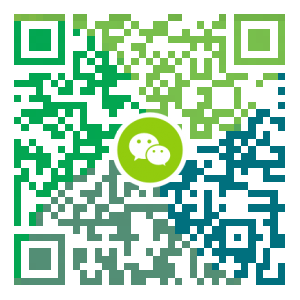
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
Java IO流关闭问题之原理简析
当有嵌套的流时即流被封装,在关闭的时候无论是关闭最外面的还是最里面的都是一样的,因为最外面的包装流的close的方法其实就是调的最里面的流的close方法。所以择其一关闭即可。参考链接:https://blog.csdn.net/congcongsuiyue/article/details/42127787
- 下一篇
Python图像处理之图片验证码识别
在上一篇博客Python图像处理之图片文字识别(OCR)中我们介绍了在Python中如何利用Tesseract软件来识别图片中的英文与中文,本文将具体介绍如何在Python中利用Tesseract软件来识别验证码(数字加字母)。 我们在网上浏览网页或注册账号时,会经常遇到验证码(CAPTCHA),如下图: 本文将具体介绍如何利用Python的图像处理模块pillow和OCR模块pytesseract来识别上述验证码(数字加字母)。 我们识别上述验证码的算法过程如下: 将原图像进行灰度处理,转化为灰度图像; 获取图片中像素点数量最多的像素(此为图片背景),将该像素作为阈值进行二值化处理,将灰度图像转化为黑白图像(用来提高识别的准确率); 去掉黑白图像中的噪声,噪声定义为:以该点为中心的九宫格的黑点的数量小于等于4; 利用pytesseract模块识别,去掉识别结果中的特殊字符,获得识别结果。 我们的图片如下(共66张图片): 完整的Python代码如下: import os import pytesseract from PIL import Image from c...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS7安装Docker,走上虚拟化容器引擎之路
- CentOS7,CentOS8安装Elasticsearch6.8.6
- Docker安装Oracle12C,快速搭建Oracle学习环境
- Linux系统CentOS6、CentOS7手动修改IP地址
- CentOS7设置SWAP分区,小内存服务器的救世主
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- MySQL8.0.19开启GTID主从同步CentOS8
- CentOS6,CentOS7官方镜像安装Oracle11G
- Mario游戏-低调大师作品
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7