Android HTTP2 + Oauth2 + Jwt 接口认证实例
本文节选自《Netkiller Java 手札》
Netkiller Java 手札
Mr. Neo Chan, 陈景峯(BG7NYT)
中国广东省深圳市望海路半岛城邦三期 518067 +86 13113668890 <netkiller@msn.com>
$Id: book.xml 606 2013-05-29 09:52:58Z netkiller $
版权 © 2015-2018 Neo Chan
版权声明
转载请与作者联系,转载时请务必标明文章原始出处和作者信息及本声明。
http://netkiller.github.io |
http://netkiller.sourceforge.net |
我的系列文档
编程语言
Netkiller Architect 手札 | Netkiller Developer 手札 | Netkiller Java 手札 | Netkiller Spring 手札 | Netkiller PHP 手札 | Netkiller Python 手札 |
---|---|---|---|---|---|
Netkiller Testing 手札 | Netkiller Cryptography 手札 | Netkiller Perl 手札 | Netkiller Docbook 手札 | Netkiller Project 手札 | Netkiller Database 手札 |
34.10. Android Oauth2 + Jwt example
Oauth 返回数据,通过 Gson 的 fromJson 存储到下面类中。
package cn.netkiller.okhttp.pojo; public class Oauth { private String access_token; private String token_type; private String refresh_token; private String expires_in; private String scope; private String jti; public String getAccess_token() { return access_token; } public void setAccess_token(String access_token) { this.access_token = access_token; } public String getToken_type() { return token_type; } public void setToken_type(String token_type) { this.token_type = token_type; } public String getRefresh_token() { return refresh_token; } public void setRefresh_token(String refresh_token) { this.refresh_token = refresh_token; } public String getExpires_in() { return expires_in; } public void setExpires_in(String expires_in) { this.expires_in = expires_in; } public String getScope() { return scope; } public void setScope(String scope) { this.scope = scope; } public String getJti() { return jti; } public void setJti(String jti) { this.jti = jti; } @Override public String toString() { return "Oauth{" + "access_token='" + access_token + '\'' + ", token_type='" + token_type + '\'' + ", refresh_token='" + refresh_token + '\'' + ", expires_in='" + expires_in + '\'' + ", scope='" + scope + '\'' + ", jti='" + jti + '\'' + '}'; } }
Activity 文件
package cn.netkiller.okhttp; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.widget.TextView; import com.google.gson.Gson; import java.io.IOException; import cn.netkiller.okhttp.pojo.Oauth; import okhttp3.Authenticator; import okhttp3.Call; import okhttp3.Callback; import okhttp3.Credentials; import okhttp3.FormBody; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.RequestBody; import okhttp3.Response; import okhttp3.Route; public class Oauth2jwtActivity extends AppCompatActivity { private TextView token; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_oauth2jwt); token = (TextView) findViewById(R.id.token); try { get(); } catch (IOException e) { e.printStackTrace(); } } public static Oauth accessToken() throws IOException { OkHttpClient client = new OkHttpClient.Builder().authenticator( new Authenticator() { public Request authenticate(Route route, Response response) { String credential = Credentials.basic("api", "secret"); return response.request().newBuilder().header("Authorization", credential).build(); } } ).build(); String url = "https://api.netkiller.cn/oauth/token"; RequestBody formBody = new FormBody.Builder() .add("grant_type", "password") .add("username", "blockchain") .add("password", "123456") .build(); Request request = new Request.Builder() .url(url) .post(formBody) .build(); Response response = client.newCall(request).execute(); if (!response.isSuccessful()) { throw new IOException("服务器端错误: " + response); } Gson gson = new Gson(); Oauth oauth = gson.fromJson(response.body().string(), Oauth.class); Log.i("oauth", oauth.toString()); return oauth; } public void get() throws IOException { OkHttpClient client = new OkHttpClient.Builder().authenticator( new Authenticator() { public Request authenticate(Route route, Response response) throws IOException { return response.request().newBuilder().header("Authorization", "Bearer " + accessToken().getAccess_token()).build(); } } ).build(); String url = "https://api.netkiller.cn/misc/compatibility"; Request request = new Request.Builder() .url(url) .build(); client.newCall(request).enqueue(new Callback() { @Override public void onFailure(Call call, IOException e) { call.cancel(); } @Override public void onResponse(Call call, Response response) throws IOException { final String myResponse = response.body().string(); runOnUiThread(new Runnable() { @Override public void run() { Log.i("oauth", myResponse); token.setText(myResponse); } }); } }); } public void post() throws IOException { OkHttpClient client = new OkHttpClient.Builder().authenticator( new Authenticator() { public Request authenticate(Route route, Response response) throws IOException { return response.request().newBuilder().header("Authorization", "Bearer " + accessToken().getAccess_token()).build(); } } ).build(); String url = "https://api.netkiller.cn/history/create"; String json = "{\n" + " \"assetsId\": \"5bced71c432c001c6ea31924\",\n" + " \"title\": \"添加信息\",\n" + " \"message\": \"信息内容\",\n" + " \"status\": \"录入\"\n" + "}"; final MediaType JSON = MediaType.parse("application/json; charset=utf-8"); RequestBody body = RequestBody.create(JSON, json); Request request = new Request.Builder() .url(url) .post(body) .build(); client.newCall(request).enqueue(new Callback() { @Override public void onFailure(Call call, IOException e) { call.cancel(); } @Override public void onResponse(Call call, Response response) throws IOException { final String myResponse = response.body().string(); runOnUiThread(new Runnable() { @Override public void run() { // Gson gson = new Gson(); // Oauth oauth = gson.fromJson(myResponse, Oauth.class); // Log.i("oauth", oauth.toString()); token.setText(myResponse); } }); } }); } }
上面的例子演示了,怎样获取 access token 以及 HTTP 基本操作 GET 和 POST,再Restful接口中还可能会用到 PUT, DELETE 等等,原来相同,这里就不在演示。
34.11. HTTP/2
首先确认你的服务器是支持 HTTP2,HTTP2配置方法可以参考 《Netkiller Web 手札》
下面是我的例子仅供参考,Nginx 开启 http2 代理后面的 Spring Cloud 接口。
server { listen 80; listen 443 ssl http2; server_name api.netkiller.cn; ssl_certificate ssl/netkiller.cn.crt; ssl_certificate_key ssl/netkiller.cn.key; ssl_session_cache shared:SSL:20m; ssl_session_timeout 60m; charset utf-8; access_log /var/log/nginx/api.netkiller.cn.access.log; error_page 497 https://$host$uri?$args; if ($scheme = http) { return 301 https://$server_name$request_uri; } location / { proxy_pass http://127.0.0.1:8000; proxy_http_version 1.1; proxy_set_header Host $host; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; } #error_page 404 /404.html; # redirect server error pages to the static page /50x.html # error_page 500 502 503 504 /50x.html; location = /50x.html { root /usr/share/nginx/html; } # deny access to .htaccess files, if Apache's document root # concurs with nginx's one # #location ~ /\.ht { # deny all; #} }
安卓程序如下
String url = "https://api.netkiller.cn/member/json"; OkHttpClient client = new OkHttpClient(); Request request = new Request.Builder().url(url).build(); client.newCall(request).enqueue(new Callback() { @Override public void onFailure(Call call, IOException e) { Log.d("okhttp", "Connect timeout. " + e.getMessage()); } @Override public void onResponse(Call call, Response response) throws IOException { String text = response.body().string(); Log.d("okhttp", "HTTP Code " + response.code() + " TEXT " + text); Log.d("okhttp", "Protocol: " + response.protocol()); } });
运行结果
D/okhttp: HTTP Code 200 TEXT {"status":false,"reason":"","code":0,"data":{"id":null,"username":"12322228888","mobile":"12322228888","password":"123456","wechat":"微信ID","role":"Organization","captcha":null,"createDate":"2018-10-25 09:25:23","updateDate":null}} Protocol: h2
输出 h2 表示当前接口与服务器连接是通过 http2 完成。
Oauth2 + Jwt 的 SpringBoot 实现方法可以参考作者的《Netkiller Spring Cloud 手札》
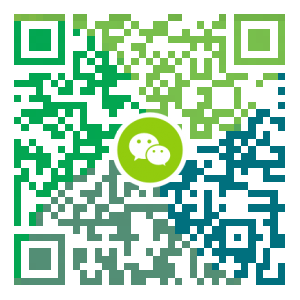
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
简单说说Kafka中的时间轮算法
零、时间轮定义 简单说说时间轮吧,它是一个高效的延时队列,或者说定时器。实际上现在网上对于时间轮算法的解释很多,定义也很全,这里引用一下朱小厮博客里出现的定义: 参考下图,Kafka中的时间轮(TimingWheel)是一个存储定时任务的环形队列,底层采用数组实现,数组中的每个元素可以存放一个定时任务列表(TimerTaskList)。TimerTaskList是一个环形的双向链表,链表中的每一项表示的都是定时任务项(TimerTaskEntry),其中封装了真正的定时任务TimerTask。 如果你理解了上面的定义,那么就不必往下看了。但如果你第一次看到和我一样懵比,并且有不少疑问,那么这篇博文将带你进一步了解时间轮,甚至理解时间轮算法。 如果有兴趣,可以去看看其他的定时器 你真的了解延时队列吗。博主认为,时间轮定时器最大的优点: 是任务的添加与移除,都是O(1)级的复杂度; 不会占用大量的资源; 只需要有一个线程去推进时间轮就可以工作了。 我们将对时间轮做层层推进的解析: 一、为什么使用环形队列 假设我们现在有一个很大的数组,专门用于存放延时任务。它的精度达到了毫秒级!那么我们的延...
- 下一篇
如何构建一个flink sql平台
我们都知道,离线计算有Hive,使用过的知道,需要先定义一个schema,比如针对HDFS这种存储对标mysql定义一个schema,schema的本质是什么?主要描述下面这些信息 1)当前存储的物理位置的描述 2)数据格式的组成形式 然后Hive可以让用户定义一段sql,针对上面定义的schema进行,sql的本质是什么,是业务逻辑的描述。然后Hive内部会将这段sql进行编译转化为原生的底层MapReduce操作,通过这种方式,屏蔽底层技术原理,让业务开发人员集中精力在schema和sql业务逻辑上,flink sql平台也正是做同样的事情。 一开始经过跟上海同事的讨论,选择Uber的Athenax作为技术选型,通过翻阅源码,发现还是有很多不完善的地方,比如配置文件采用yaml,如果做多集群调度,平台代码优化,多存储扩展机制,都没有考虑得很清楚,所以代码拿过来之后基本上可以说按照对yarn和flink的理解重新写了一遍。 大致的工作流程如图所示: 简单解释一下: 1)业务定义job 2)提交到web服务器,存到mysql中 3)------------------- 4)---...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- MySQL8.0.19开启GTID主从同步CentOS8
- Mario游戏-低调大师作品
- Linux系统CentOS6、CentOS7手动修改IP地址
- Docker安装Oracle12C,快速搭建Oracle学习环境
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- CentOS7安装Docker,走上虚拟化容器引擎之路
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- CentOS7编译安装Cmake3.16.3,解决mysql等软件编译问题
- CentOS7编译安装Gcc9.2.0,解决mysql等软件编译问题