Android Kotlin/Java 动态设置 shape/drawable 等状态效果
小菜最近遇到一个小需求,程序里面有个别页面,需要动态的调整某个页面的样式,包括一键变灰等效果。
以前页面是用 shape 和 drawable 之类实现的效果。现在需要用 Kotlin/Java 代码实现动态修改。由于小菜技术浅浅,仅整理一下遇到一些坑。
日常应用的样式:
1. 圆角边框
默认 shape.xml 方式:
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android"> <stroke android:width="0.5dp" android:color="@color/colorAccent" /> <corners android:radius="15dp" /> </shape>
现 Kotlin/Java 方式动态修改边框颜色:
var myGrad = tv2!!.getBackground() as GradientDrawable myGrad.setStroke(1, resources.getColor(R.color.colorPrimary))
Tips: GradientDrawable 对象可设置 shape 边框属性(矩形/椭圆等)、stroke 边框宽度和颜色、cornerRadius 圆角角度、color 填充背景色。
2. 圆角边框填充颜色
默认 shape.xml 方式:
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android"> <solid android:color="@color/colorAccent" /> <stroke android:width="0.5dp" android:color="@color/colorAccent" /> <corners android:radius="15dp" /> </shape>
现 Kotlin/Java 方式动态修改边框颜色及填充背景色:
var myGrad = tv3!!.getBackground() as GradientDrawable myGrad.setStroke(1, resources.getColor(R.color.colorPrimary)) myGrad.setColor(resources.getColor(R.color.colorPrimary))
3. 圆角边框填充颜色,点击变更背景色
默认 shape.xml 方式:
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:drawable="@drawable/drawable_test1" android:state_pressed="true" /> <item android:drawable="@drawable/drawable_test2" android:state_focused="false" android:state_pressed="false" /> <item android:drawable="@drawable/drawable_test1" android:state_focused="true" /> <item android:drawable="@drawable/drawable_test2" android:state_focused="false" /> </selector>
现 Kotlin/Java 方式动态修改边框颜色填充背景色,点击变更背景色:
var myGrad1 = GradientDrawable() myGrad1.setStroke(1, resources.getColor(R.color.colorPrimary)) myGrad1.cornerRadius = 80.0f myGrad1.setColor(resources.getColor(R.color.colorPrimary)) var myGrad2 = GradientDrawable() myGrad2.setStroke(1, resources.getColor(R.color.colorPrimary)) myGrad2.cornerRadius = 80.0f myGrad2.setColor(resources.getColor(R.color.white))
tv4!!.background = BitmapUtil.addStateDrawable1(context, myGrad3, myGrad4, myGrad4, myGrad4)
public static StateListDrawable addStateDrawable1(Context context, Drawable idNormal, Drawable idPressed, Drawable idFocused, Drawable idUnable) { StateListDrawable bg = new StateListDrawable(); Drawable normal = idNormal; Drawable pressed = idPressed; Drawable focused = idFocused; Drawable unable = idUnable; // View.PRESSED_ENABLED_STATE_SET bg.addState(new int[] { android.R.attr.state_pressed, android.R.attr.state_enabled }, pressed); // View.ENABLED_FOCUSED_STATE_SET bg.addState(new int[] { android.R.attr.state_enabled, android.R.attr.state_focused }, focused); // View.ENABLED_STATE_SET bg.addState(new int[] { android.R.attr.state_enabled }, normal); // View.FOCUSED_STATE_SET bg.addState(new int[] { android.R.attr.state_focused }, focused); // View.WINDOW_FOCUSED_STATE_SET bg.addState(new int[] { android.R.attr.state_window_focused }, unable); // View.EMPTY_STATE_SET bg.addState(new int[] {}, normal); return bg; }
Tips: StateListDrawable 设置 View 绘制不同状态背景图片,小菜测试中,发现需要设置点击事件或者 Pressed/Focused 状态,小菜认为如果只是设置 StateListDrawable 默认是 normal 样式,不会有点击效果。
4. 圆角边框填充颜色,点击变更背景色及文字颜色
默认 color.xml 方式:
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:color="@color/colorAccent" android:state_selected="true" /> <item android:color="@color/colorAccent" android:state_focused="true" /> <item android:color="@color/colorAccent" android:state_pressed="true" /> <item android:color="@color/white" /> </selector>
现 Kotlin/Java 方式动态修改边框颜色填充背景色,点击变更背景色及文字颜色:
tv5!!.setTextColor(BitmapUtil.createColorStateList(getResources().getColor(R.color.white), getResources().getColor(R.color.colorPrimary), getResources().getColor(R.color.colorPrimary), getResources().getColor(R.color.colorPrimary)));
public static ColorStateList createColorStateList(int normal, int pressed, int focused, int unable) { int[] colors = new int[] { pressed, focused, normal, focused, unable, normal }; int[][] states = new int[6][]; states[0] = new int[] { android.R.attr.state_pressed, android.R.attr.state_enabled }; states[1] = new int[] { android.R.attr.state_enabled, android.R.attr.state_focused }; states[2] = new int[] { android.R.attr.state_enabled }; states[3] = new int[] { android.R.attr.state_focused }; states[4] = new int[] { android.R.attr.state_window_focused }; states[5] = new int[] {}; ColorStateList colorList = new ColorStateList(states, colors); return colorList; }
Tips: 小菜建议在编辑 color.xml 时,新建在 color 资源文件夹下。ColorStateList 对象设置文字点击时不同状态等文字效果。
5. 部分圆角边框填充颜色
默认 shape.xml 方式:
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android"> <solid android:color="@color/colorAccent" /> <stroke android:width="0.5dp" android:color="@color/colorAccent" /> <corners android:bottomLeftRadius="15dp" android:topLeftRadius="15dp" /> </shape>
现 Kotlin/Java 方式动态修改部分圆角边框:
var myGrad = GradientDrawable() var farr = floatArrayOf(80.0f, 80.0f, 0.0f, 0.0f, 0.0f, 0.0f, 80.0f, 80.0f) myGrad.cornerRadii = farr myGrad.setStroke(1, resources.getColor(R.color.colorPrimary)) myGrad.setColor(resources.getColor(R.color.colorPrimary)) tv6!!.background = myGrad
Tips: GradientDrawable 对象中,若设置四个圆角一致时,可设置 cornerRadius 属性;若设置部分圆角时,可设置 cornerRadii 属性,该属性包括 8 个 float 参数 (左上[X_Radius,Y_Radius],右上[X_Radius,Y_Radius],右下[X_Radius,Y_Radius],左下[X_Radius,Y_Radius]) 且只有设置 [X_Radius,Y_Radius] 两个参数时起作用。
6. 图标绘色
默认设置 tint 属性:
<ImageView android:id="@+id/drawable_iv3" android:layout_width="50dp" android:layout_height="50dp" android:layout_marginTop="20dp" android:src="@mipmap/icon_zan" android:tint="@color/colorAccent" />
现 Kotlin/Java 方式对图标绘色:
var plabit = BitmapUtil.drawableToBitmap3(resources.getDrawable(R.mipmap.icon_zan)) plabit = BitmapUtil.tintBitmap(plabit, resources.getColor(R.color.inactive_bottom_navigation)) iv2!!.setImageDrawable(BitmapDrawable(plabit))
public static Bitmap drawableToBitmap3(Drawable drawable) { Bitmap bitmap = Bitmap.createBitmap(drawable.getIntrinsicWidth(), drawable.getIntrinsicHeight(), drawable.getOpacity() != PixelFormat.OPAQUE ? Bitmap.Config.ARGB_8888 : Bitmap.Config.RGB_565); Canvas canvas = new Canvas(bitmap); drawable.setBounds(0, 0, drawable.getIntrinsicWidth(), drawable.getIntrinsicHeight()); drawable.draw(canvas); return bitmap; }
Tips: 用该方法绘制颜色时,建议不要设置图片的 tint 属性。
7. 图片灰度
设置 ColorMatrix 对象的 Saturation 属性:
val matrixpic = ColorMatrix() matrixpic.setSaturation(0f)//饱和度 0灰色 100过度彩色,50正常 val filter = ColorMatrixColorFilter(matrixpic) iv1!!.setColorFilter(filter)
下面是小菜的公众号,欢迎闲来吐槽~
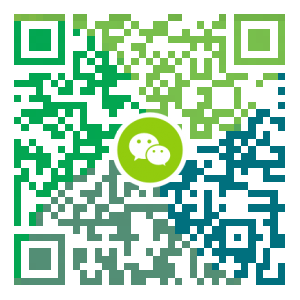
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
JVM笔记8-虚拟机性能监控与故障处理工具
1.JDK命令行工具 Java开发人员肯定都知道JDK的bin目录有“java.exe”,"javac.exe"这两个命令行工具,但并非所有程序员都了解过JDK的bin目录之中其他命令行程序的作用。每次JDK更新,bin目录下命令行工具的数量和功能总会不知不觉地增强。 主要包括用于监控虚拟机和故障处理的工具。这些工具被Sun公司作为礼物附赠给JDK的使用者。如下图: 可以看到这些工具的程序体积都异常小巧。基本都稳定在17K左右。这并非JDK开发团队刻意把他们制作得如此精炼,而是这些命令行工具大多数是JDK/lib/tools.jar类库的一层薄包装而已,它们主要的功能代码是在tools类库中实现的。 之所以这样做,是因为当应用程序部署到生产环境后,无论是直接接触物理服务器还是远程Telnet到服务器上都可能会受到限制。借助tools.jar类库里面的接口,我们可以直接在应用程序中实现功能强大的监控分析功能。 下面列举JDK主要命令行监控工具的用途。 1.jps:虚拟机就进程状况工具 JDK的很多小工具的名字都参考了UNIX命令的命名方式,jps名字像UNIX的ps命令之外,也和ps...
- 下一篇
Java高并发秒杀业务Api-Service层构建过程
章节目录 秒杀Service 接口开发工作 秒杀业务逻辑编写 spring-IOC 管理 service 组件 context:component-scan Spring 声明式事务 junit测试 创建基本的代码包层 1.创建DTO - 数据传输层对象 网络数据到达Controller 层后会使用框架自带的数据绑定 以及反序列化为dto对 象,并作为参数传递至service层进行处理。 2.业务接口实现 注意:业务接口的实现需要站在使用者的角度去设计接口 方法定义粒度-非常明确,参数简练,直接,不要一个大map对象去传递,return 还可以抛出异常。 代码如下:业务逻辑接口声明类 SecKillService.java package org.seckill.service; import org.seckill.domain.SecKill; import org.seckill.dto.Exposer; import org.seckill.dto.SecKillExcution; import org.seckill.exception.RepeatKillException...
相关文章
文章评论
共有0条评论来说两句吧...