Java高并发秒杀Api-业务分析与DAO层构建3
章节目录
- mybatis与spring整合过程
- spring-dao.xml 配置
- junit4 单元测试
1.mybatis与spring整合过程
1.1 spring-dao.xml 配置
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <!--配置整合mybatis--> <!--1:配置数据库相关参数--> <!-- 1:配置数据库相关参数properties的属性:${url} --> <context:property-placeholder location="classpath:jdbc.properties"/> <!--2.数据库连接池配置--> <bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource"> <!-- 配置连接池属性--> <property name="driverClass" value="${driver}" /> <property name="jdbcUrl" value="${url}" /> <property name="user" value="${username}" /> <property name="password" value="${password}" /> <!-- c3p0连接池的私有属性--> <property name="maxPoolSize" value="30" /> <property name="minPoolSize" value="10" /> <!-- 关闭连接后不自动commit--> <property name="autoCommitOnClose" value="false" /> <!-- 获取连接超时时间 30个连接用完--> <property name="checkoutTimeout" value="1000" /> <!-- 当获取连接失败重试次数--> <property name="acquireRetryAttempts" value="2" /> </bean> <!-- 约定大于配置--> <!-- 3:配置SqlSessionFactory对象--> <bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean"> <!-- 注入数据库连接池--> <property name="dataSource" ref="dataSource" /> <!-- 配置MyBatis全局配置文件:mybatis-config.xml--> <property name="configLocation" value="classpath:mybatis-config.xml" /> <!-- 扫描entity包 使用别名--> <property name="typeAliasesPackage" value="org.seckill.domain" /> <!-- 扫描sql配置文件;mapper需要的xml文件--> <property name="mapperLocations" value="classpath:mapper/*.xml" /> </bean> <!--配置扫描Dao接口包,动态实现Dao接口 mapper 代理,并注入到Spring容器中 MapperScannerConfigurer--> <bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <!--注入sqlSessionFactory,懒加载,用到的时候才加载--> <property name="sqlSessionFactoryBeanName" value="sqlSessionFactory"></property> <!--给出扫描DAO接口包--> <property name="basePackage" value="org.seckill.dao"></property> </bean> </beans>
2.junit 单元测试
SecKillDaoTest.java - 测试秒杀
package org.seckill.dao; import org.junit.Test; import org.junit.runner.RunWith; import org.seckill.domain.SecKill; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; import java.util.Date; import java.util.List; /** * 配置spring 与 junit 启动时加载Spring IOC容器 * spring-test,junit整合 * 告诉junit Spring配置文件位置 */ @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration({"classpath:spring/spring-dao.xml"}) public class SecKillDaoTest { //注入Dao类实现 @Autowired private SecKillDao secKillDao; @Test public void queryById() throws Exception { long id = 1000; SecKill secKill = secKillDao.queryById(id); System.out.println(secKill.getName()); System.out.println(secKill); /** * 1000元秒杀iphone x SecKill{seckillId=1000, name='1000元秒杀iphone x', stock=100, startTime=Fri May 04 00:00:00 CST 2018, endTime=Sat May 05 00:00:00 CST 2018, createTime=Sat May 05 00:05:03 CST 2018 } */ } /** * @throws Exception */ @Test public void queryAll() throws Exception { // queryAll(offset,limit)->queryAll(arg0,arg1) List<SecKill> secKillList = secKillDao.queryAll(0, 100); for (SecKill secKill : secKillList) { System.out.println(secKill); } /** * SecKill{seckillId=1000, name='1000元秒杀iphone x', stock=100, startTime=Fri May 04 00:00:00 CST 2018, endTime=Sat May 05 00:00:00 CST 2018, createTime=Sat May 05 00:05:03 CST 2018} SecKill{seckillId=1001, name='500元秒杀ipad x', stock=200, startTime=Fri May 04 00:00:00 CST 2018, endTime=Sat May 05 00:00:00 CST 2018, createTime=Sat May 05 00:05:03 CST 2018} SecKill{seckillId=1002, name='300元秒杀小米4', stock=300, startTime=Fri May 04 00:00:00 CST 2018, endTime=Sat May 05 00:00:00 CST 2018, createTime=Sat May 05 00:05:03 CST 2018} SecKill{seckillId=1003, name='200元秒杀小米note', stock=400, startTime=Fri May 04 00:00:00 CST 2018, endTime=Sat May 05 00:00:00 CST 2018, createTime=Sat May 05 00:05:03 CST 2018} */ } @Test public void reduceStock() throws Exception { Date killTime = new Date(); int updateCount = secKillDao.reduceStock(1000L, killTime); System.out.println(updateCount); } }
测试秒杀明细
package org.seckill.dao; import org.junit.Test; import org.junit.runner.RunWith; import org.seckill.domain.SuccessKilled; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; @RunWith(SpringJUnit4ClassRunner.class) @ContextConfiguration("classpath:spring/spring-dao.xml") public class SuccessKilledDaoTest { @Autowired private SuccessKilledDao successKilledDao; @Test public void insertSuccessKilled() throws Exception { int successCount = successKilledDao.insertSuccessKilled(1001L, "15300815999"); System.out.println("insertCount=" + successCount); } @Test public void queryByIdWithSecKill() throws Exception { //1个用户只能查询到属于自己的一个秒杀明细 long id = 1001L; String phone = "15300815999"; SuccessKilled successKilled = successKilledDao.queryByIdWithSecKill(id, phone); System.out.println(successKilled); } }
接下来是service 层的构建
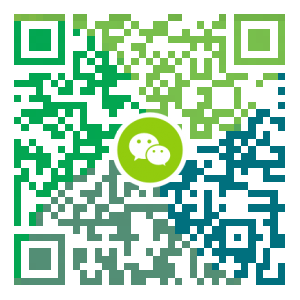
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
c# IPC实现本机进程之间的通信
原文: c# IPC实现本机进程之间的通信 IPC可以实现本地进程之间通信。这种用法不是太常见,常见的替代方案是使用wcf,remoting,web service,socket(tcp/pipe/...)等其他分布式部署方案来替代进程之间的通信。虽然不常见但也避免不了一些场景会使用该方案。 应用包含: 1)使用IPC技术实现多client与一个sever通信(不过是本机,感觉意义不大,但如果想实现本机上运行确实是一个不错的方案); 2)使用IPC技术实现订阅者和生产者分离时,一个server接收并消费消息,客户端是生产消息的。 1 1:新建一个MessageObject类库 2 3 代码如下: 4 5 using System; 6 using System.Collections.Generic; 7 8 namespace MessageObject 9 { 10 //MarshalByRefObject 允许在支持远程处理的应用程序中跨应用程序域边界访问对象。 11 public class RemoteObject : MarshalByRefObject 12 { 13 p...
- 下一篇
JVM笔记8-虚拟机性能监控与故障处理工具
1.JDK命令行工具 Java开发人员肯定都知道JDK的bin目录有“java.exe”,"javac.exe"这两个命令行工具,但并非所有程序员都了解过JDK的bin目录之中其他命令行程序的作用。每次JDK更新,bin目录下命令行工具的数量和功能总会不知不觉地增强。 主要包括用于监控虚拟机和故障处理的工具。这些工具被Sun公司作为礼物附赠给JDK的使用者。如下图: 可以看到这些工具的程序体积都异常小巧。基本都稳定在17K左右。这并非JDK开发团队刻意把他们制作得如此精炼,而是这些命令行工具大多数是JDK/lib/tools.jar类库的一层薄包装而已,它们主要的功能代码是在tools类库中实现的。 之所以这样做,是因为当应用程序部署到生产环境后,无论是直接接触物理服务器还是远程Telnet到服务器上都可能会受到限制。借助tools.jar类库里面的接口,我们可以直接在应用程序中实现功能强大的监控分析功能。 下面列举JDK主要命令行监控工具的用途。 1.jps:虚拟机就进程状况工具 JDK的很多小工具的名字都参考了UNIX命令的命名方式,jps名字像UNIX的ps命令之外,也和ps...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Eclipse初始化配置,告别卡顿、闪退、编译时间过长
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- Windows10,CentOS7,CentOS8安装Nodejs环境
- 设置Eclipse缩进为4个空格,增强代码规范
- CentOS7编译安装Cmake3.16.3,解决mysql等软件编译问题
- CentOS7设置SWAP分区,小内存服务器的救世主
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- Linux系统CentOS6、CentOS7手动修改IP地址
- Docker安装Oracle12C,快速搭建Oracle学习环境
- Windows10,CentOS7,CentOS8安装MongoDB4.0.16