Swift 就像 Kotlin?看看 Swift 与 Kotlin 的简单对比
一位国外的程序员认为 Swift 的语法与 Kotlin 相似,并整理了一些 Swift 和 Kotlin 的对比,下面是一些例子,大家不妨也看看。
BASICS Hello World Swift print("Hello, world!") Kotlin println("Hello, world!") 变量和常量 Swift var myVariable = 42 myVariable = 50 let myConstant = 42 Kotlin var myVariable = 42 myVariable = 50 val myConstant = 42 显式类型 Swift let explicitDouble: Double = 70 Kotlin val explicitDouble: Double = 70.0 强制类型转换 Swift let label = "The width is " let width = 94 let widthLabel = label + String(width) Kotlin val label = "The width is " val width = 94 val widthLabel = label + width 字符串插值 Swift let apples = 3 let oranges = 5 let fruitSummary = "I have \(apples + oranges) " + "pieces of fruit." Kotlin val apples = 3 val oranges = 5 val fruitSummary = "I have ${apples + oranges} " + "pieces of fruit." 范围操作符 Swift let names = ["Anna", "Alex", "Brian", "Jack"] let count = names.count for i in 0..<count { print("Person \(i + 1) is called \(names[i])") } // Person 1 is called Anna // Person 2 is called Alex // Person 3 is called Brian // Person 4 is called Jack Kotlin val names = arrayOf("Anna", "Alex", "Brian", "Jack") val count = names.count() for (i in 0..count - 1) { println("Person ${i + 1} is called ${names[i]}") } // Person 1 is called Anna // Person 2 is called Alex // Person 3 is called Brian // Person 4 is called Jack
包罗广泛的范围操作符(Inclusive Range Operator)
Swift for index in 1...5 { print("\(index) times 5 is \(index * 5)") } // 1 times 5 is 5 // 2 times 5 is 10 // 3 times 5 is 15 // 4 times 5 is 20 // 5 times 5 is 25 Kotlin for (index in 1..5) { println("$index times 5 is ${index * 5}") } // 1 times 5 is 5 // 2 times 5 is 10 // 3 times 5 is 15 // 4 times 5 is 20 // 5 times 5 is 25 BASICS 数组 Swift var shoppingList = ["catfish", "water", "tulips", "blue paint"] shoppingList[1] = "bottle of water" Kotlin val shoppingList = arrayOf("catfish", "water", "tulips", "blue paint") shoppingList[1] = "bottle of water" 映射 Swift var occupations = [ "Malcolm": "Captain", "Kaylee": "Mechanic", ] occupations["Jayne"] = "Public Relations" Kotlin val occupations = mutableMapOf( "Malcolm" to "Captain", "Kaylee" to "Mechanic" ) occupations["Jayne"] = "Public Relations" 空集合 Swift let emptyArray = [String]() let emptyDictionary = [String: Float]() Kotlin val emptyArray = arrayOf<String>() val emptyMap = mapOf<String, Float>() FUNCTIONS 函数 Swift func greet(_ name: String,_ day: String) -> String { return "Hello \(name), today is \(day)." } greet("Bob", "Tuesday") Kotlin fun greet(name: String, day: String): String { return "Hello $name, today is $day." } greet("Bob", "Tuesday") 元组返回 Swift func getGasPrices() -> (Double, Double, Double) { return (3.59, 3.69, 3.79) } Kotlin data class GasPrices(val a: Double, val b: Double, val c: Double) fun getGasPrices() = GasPrices(3.59, 3.69, 3.79) 参数的变量数目(Variable Number Of Arguments) Swift func sumOf(_ numbers: Int...) -> Int { var sum = 0 for number in numbers { sum += number } return sum } sumOf(42, 597, 12) Kotlin fun sumOf(vararg numbers: Int): Int { var sum = 0 for (number in numbers) { sum += number } return sum } sumOf(42, 597, 12) // sumOf() can also be written in a shorter way: fun sumOf(vararg numbers: Int) = numbers.sum() 函数类型 Swift func makeIncrementer() -> (Int -> Int) { func addOne(number: Int) -> Int { return 1 + number } return addOne } let increment = makeIncrementer() increment(7) Kotlin fun makeIncrementer(): (Int) -> Int { val addOne = fun(number: Int): Int { return 1 + number } return addOne } val increment = makeIncrementer() increment(7) // makeIncrementer can also be written in a shorter way: fun makeIncrementer() = fun(number: Int) = 1 + number 映射 Swift let numbers = [20, 19, 7, 12] numbers.map { 3 * $0 } Kotlin val numbers = listOf(20, 19, 7, 12) numbers.map { 3 * it } 排序 Swift var mutableArray = [1, 5, 3, 12, 2] mutableArray.sort() Kotlin listOf(1, 5, 3, 12, 2).sorted() 命名参数 Swift func area(width: Int, height: Int) -> Int { return width * height } area(width: 2, height: 3) Kotlin fun area(width: Int, height: Int) = width * height area(width = 2, height = 3) // This is also possible with named arguments area(2, height = 2) area(height = 3, width = 2) CLASSES 声明 Swift class Shape { var numberOfSides = 0 func simpleDescription() -> String { return "A shape with \(numberOfSides) sides." } } Kotlin class Shape { var numberOfSides = 0 fun simpleDescription() = "A shape with $numberOfSides sides." } 用法 Swift var shape = Shape() shape.numberOfSides = 7 var shapeDescription = shape.simpleDescription() Kotlin var shape = Shape() shape.numberOfSides = 7 var shapeDescription = shape.simpleDescription() 子类 Swift class NamedShape { var numberOfSides: Int = 0 let name: String init(name: String) { self.name = name } func simpleDescription() -> String { return "A shape with \(numberOfSides) sides." } } class Square: NamedShape { var sideLength: Double init(sideLength: Double, name: String) { self.sideLength = sideLength super.init(name: name) self.numberOfSides = 4 } func area() -> Double { return sideLength * sideLength } override func simpleDescription() -> String { return "A square with sides of length " + sideLength + "." } } let test = Square(sideLength: 5.2, name: "square") test.area() test.simpleDescription() Kotlin open class NamedShape(val name: String) { var numberOfSides = 0 open fun simpleDescription() = "A shape with $numberOfSides sides." } class Square(var sideLength: BigDecimal, name: String) : NamedShape(name) { init { numberOfSides = 4 } fun area() = sideLength.pow(2) override fun simpleDescription() = "A square with sides of length $sideLength." } val test = Square(BigDecimal("5.2"), "square") test.area() test.simpleDescription() 类型检查 Swift var movieCount = 0 var songCount = 0 for item in library { if item is Movie { movieCount += 1 } else if item is Song { songCount += 1 } } Kotlin var movieCount = 0 var songCount = 0 for (item in library) { if (item is Movie) { ++movieCount } else if (item is Song) { ++songCount } } 模式匹配 Swift let nb = 42 switch nb { case 0...7, 8, 9: print("single digit") case 10: print("double digits") case 11...99: print("double digits") case 100...999: print("triple digits") default: print("four or more digits") } Kotlin val nb = 42 when (nb) { in 0..7, 8, 9 -> println("single digit") 10 -> println("double digits") in 11..99 -> println("double digits") in 100..999 -> println("triple digits") else -> println("four or more digits") } 类型向下转换 Swift for current in someObjects { if let movie = current as? Movie { print("Movie: '\(movie.name)', " + "dir. \(movie.director)") } } Kotlin for (current in someObjects) { if (current is Movie) { println("Movie: '${current.name}', " + "dir. ${current.director}") } } 协议 Swift protocol Nameable { func name() -> String } func f<T: Nameable>(x: T) { print("Name is " + x.name()) } Kotlin interface Nameable { fun name(): String } fun f<T: Nameable>(x: T) { println("Name is " + x.name()) } 扩展 Swift extension Double { var km: Double { return self * 1_000.0 } var m: Double { return self } var cm: Double { return self / 100.0 } var mm: Double { return self / 1_000.0 } var ft: Double { return self / 3.28084 } } let oneInch = 25.4.mm print("One inch is \(oneInch) meters") // prints "One inch is 0.0254 meters" let threeFeet = 3.ft print("Three feet is \(threeFeet) meters") // prints "Three feet is 0.914399970739201 meters" Kotlin val Double.km: Double get() = this * 1000 val Double.m: Double get() = this val Double.cm: Double get() = this / 100 val Double.mm: Double get() = this / 1000 val Double.ft: Double get() = this / 3.28084 val oneInch = 25.4.mm println("One inch is $oneInch meters") // prints "One inch is 0.0254 meters" val threeFeet = 3.0.ft println("Three feet is $threeFeet meters") // prints "Three feet is 0.914399970739201 meters"
本文来自开源中国社区 [http://www.oschina.net]
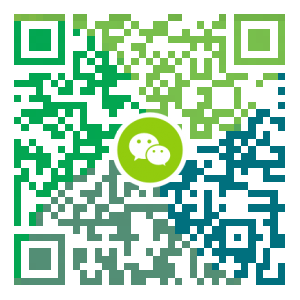
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
App Store 六周年 开发者遭遇更大挑战
7月11日消息,据TechCrunch报道,首先让我们向App Store说声“生日快乐!”今天是苹果App Store的六岁生日,据该公司公布的最新数据显示,目前App Store上有120万个应用供用户下载,累计下载量已经超过了750亿次。 但是根据两家不同的分析公司的报告显示,一些开发者在这项业务上处境艰难。一家公司指出有超过21%曾经亮相过的应用现在已经“死亡”;另一家 公司则表示,App Store上出现了一种“应用倦怠”(app burnout)的新趋势。后一种趋势表明,现在许多应用被用户视为“一次性”应用——我们会把它们玩到无聊为止,但是只要完成了任务或是出现了下一个好 玩的东西,我们就会抛弃它们。 “死亡”和倦怠的应用 与之前开发应用不同,现在开发者要做的不仅仅只是销售推广。他们必须找到并留住忠实用户,提高用户参与度,思考包括移动广告和应用内付费等在内的各种盈利模式,并努力在算法复杂、不断变化的App Store榜单上维持一个高排名。 据Adjust数据显示,截止目前开发者累计在App Store上提交了1601413款应用,但是只有1252777款应用通过了审核。 在...
- 下一篇
桌面版 Android 系统来了
一直使用Windows系统,大家是不是都已经面对它的桌面有点厌倦了呢?最近几位谷歌的工程师打造的Remix OS可能会有耳目一新的感觉。Remix OS for PC基于Android-x86项目,由安卓5.1 Lollipop深度定制而来,不但兼容所有安卓应用和游戏,还针对桌面应用环境增加了各种使用功能。 本次放出的是预览版的内容,版本号为2016011201,体积约692.15MB,主要面向开发者和尝鲜者。需要注意的是,安装需要通过U盘,而且必须支持USB 3.0接口,而且写入速度不低于20MB/s,容量不低于8GB。Remix OS官方下载地址。安装包里自带了U盘启动盘制作工具Remix OS USB Tool,根据向导制作即可。 ====================================分割线================================文章转载自 开源中国社区[http://www.oschina.net]
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS7,CentOS8安装Elasticsearch6.8.6
- CentOS7安装Docker,走上虚拟化容器引擎之路
- Docker安装Oracle12C,快速搭建Oracle学习环境
- Linux系统CentOS6、CentOS7手动修改IP地址
- CentOS7设置SWAP分区,小内存服务器的救世主
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- MySQL8.0.19开启GTID主从同步CentOS8
- CentOS6,CentOS7官方镜像安装Oracle11G
- Mario游戏-低调大师作品
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7