iOS开发-UIScreenEdgePanGestureRecognizer实战
UIScreenEdgePanGestureRecognizer名字很长,而且关于其文档也是少的的可怜,苹果官方给的唯一的一个属性是edges,文档中的解释是这样的:
1 | A UIScreenEdgePanGestureRecognizer looks for panning (dragging) gestures that start near an edge of the screen. The system uses screen edge gestures in some cases to initiate view controller transitions. You can use this class to replicate the same gesture behavior for your own actions. |
大概的意思就是UIScreenEdgePanGestureRecognizer跟pan(平移)手势差不多,需要从边缘进行拖动,在控制器转换的时候是有用的,看文档的话我们会发现UIScreenEdgePanGestureRecognizer是UIPanGestureRecognizer的子类,理解会更方便一点。
UIPanGestureRecognizer铺垫
先简单的看下需要实现的视图控制器的效果:
稍微回顾一下UIPanGestureRecognizer,第一个红色的视图我们通过Pan手势进行操作:
1 2 3 4 5 6 7 8 9 | self.panView=[[UIView alloc]initWithFrame:CGRectMake(0, 200, CGRectGetWidth(self.view.bounds), 100)]; [self.panView setBackgroundColor:[UIColor redColor]]; self.panLabel=[[UILabel alloc]initWithFrame:CGRectMake(20, 30, 150, 40)]; [self.panLabel setText: @"博客园-FlyElephant" ]; [self.panLabel setFont:[UIFont systemFontOfSize:14]]; [self.panView addSubview:self.panLabel]; [self.view addSubview:self.panView]; UIPanGestureRecognizer *pangestureRecognizer=[[UIPanGestureRecognizer alloc]initWithTarget:self action:@selector(panGesture:)]; [self.panView addGestureRecognizer:pangestureRecognizer]; |
手势事件:
1 2 3 4 | -( void )panGesture:(UIPanGestureRecognizer *)gesture{ CGPoint translation = [gesture translationInView:gesture.view]; NSLog( @"%@" ,[NSString stringWithFormat: @"(%0.0f, %0.0f)" , translation.x, translation.y]); } |
手势向左滑动的panView的变化:
UIScreenEdgePanGestureRecognizer实战
第二个视图我们可以通过UIScreenEdgePanGestureRecognizer进行设置,跟上面的代码稍微有点重复,如果你有代码洁癖的话可以考虑将以上代码进行惰性初始化,可能感官会更好一点,不过为了方便暂时都写在了一起:
1 2 3 4 5 6 7 8 | self.centerX=CGRectGetWidth(self.view.bounds)/2; self.edgeView=[[UIView alloc]initWithFrame:CGRectMake(0, 320, CGRectGetWidth(self.view.bounds), 100)]; [self.edgeView setBackgroundColor:[UIColor greenColor]]; self.label=[[UILabel alloc]initWithFrame:CGRectMake(10, 30, 320, 40)]; [self.label setText: @"原文地址:http://www.cnblogs.com/xiaofeixiang/" ]; [self.label setFont:[UIFont systemFontOfSize:14]]; [self.edgeView addSubview:self.label]; [self.view addSubview:self.edgeView]; |
注意这个时候手势是加载view不是单独的edgeView上的,手势代码,edges是一个枚举,我们可以设置的是响应边缘右滑事件;
1 2 3 4 5 | UIScreenEdgePanGestureRecognizer *rightEdgeGesture = [[UIScreenEdgePanGestureRecognizer alloc] initWithTarget:self action:@selector(handleRightEdgeGesture:)]; rightEdgeGesture.edges = UIRectEdgeRight; // 右滑显示 [self.view addGestureRecognizer:rightEdgeGesture]; |
响应边缘事件的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | //当前被触摸的view UIView *view = [self.view hitTest:[gesture locationInView:gesture.view] withEvent:nil]; if (UIGestureRecognizerStateBegan == gesture.state || UIGestureRecognizerStateChanged == gesture.state) { CGPoint translation = [gesture translationInView:gesture.view]; [UIView animateWithDuration:0.5 animations:^{ view.center = CGPointMake(self.centerX + translation.x, view.center.y); NSLog( @"%@" ,NSStringFromCGPoint(view.center)); }]; } else //取消,失败,结束的时候返回原处 { [UIView animateWithDuration:0.5 animations:^{ view.center = CGPointMake(self.centerX, view.center.y); }]; } |
具体效果如下:
如果你细心点会发现那个篮球在滑动介结束的时候转动了一下,在处理动画结束的时候加了一个判断,代码如下:
1 2 3 4 5 6 7 8 9 10 | if (gesture.state==UIGestureRecognizerStateEnded) { //旋转360度之后归0 if (self.currentRadius==360.f){ self.currentRadius=0.0f; } [UIView animateWithDuration:1.0 animations:^{ self.currentRadius += 90.0; self.circleView.transform = CGAffineTransformMakeRotation((self.currentRadius * M_PI) / 180.0); }]; } |
如果你想那个篮球一直转动的话通过NSTimer即可实现:
1 | [NSTimer scheduledTimerWithTimeInterval:0.1 target:self selector:@selector(transformRotate) userInfo: nil repeats: YES]; |
转动的代码和上面的差不多,不过每次改变的弧度较小:
1 2 3 4 5 6 7 8 | -( void )transformRotate{ if (self.currentRadius==360.f){ self.currentRadius=0.0f; } else { self.currentRadius += 10.0; self.circleView.transform = CGAffineTransformMakeRotation((self.currentRadius * M_PI) / 180.0); } } |
本文转自Fly_Elephant博客园博客,原文链接:http://www.cnblogs.com/xiaofeixiang/p/4725645.html,如需转载请自行联系原作者
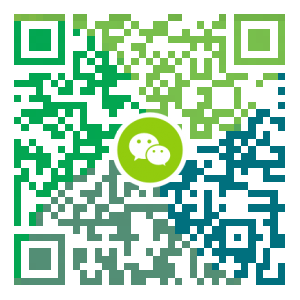
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
在安卓上运行TensorFlow:让深度学习进入移动端
如果你关注我的前一篇帖子,并按照其中的内容实践,你可能已经学会了如何在Linux上安装一个GPU加速的TensorFlow,并构建了你自己的图像分类器。老实讲,在笔记本上对图片进行分类是很花时间的:需要下载分类用的图片,并在终端里输入很多行命令来运行分类。 不过,尽管没有很多的公开资料,好消息是你也可以在有摄像头的手机上运行TensofrFlow的Inception分类器,甚至是你自定义的分类器。然后你只要把摄像头对准你希望做分类的东西,TensorFlow就会告诉你它认为这是什么东西。TensorFlow是可以在iOS和树莓派上面运行,不过在这个教程里,我会介绍如何在安卓设备上运行TensorFlow。 我会一步一步地介绍如何在安卓设备上运行定制化的图片分类器。实现这个功能需要很多步骤,而且其他地方没有这样的介绍,只能通过反复地查看
- 下一篇
iOS开发-UIRefreshControl下拉刷新
下拉刷新一直都是第三库的天下,有的第三库甚至支持上下左右刷新,UIRefreshControl是iOS6之后支持的一个刷新控件,不过由于功能单一,样式不能自定义,因此不能满足大众的需求,用法比较简单在UITableview和UICollectionview上面直接添加子视图即可使用。 代码调用: 1 2 3 4 5 6 7 self .refreshControl = [[UIRefreshControl alloc] init]; [_refreshControl addTarget: self action: @selector (refreshView:) forControlEvents:UIControlEventValueChanged]; [ self .refreshControl setAttributedTitle:[[ NSAttributedString alloc] initWithString:@ "数据加载-FlyElephant" ]]; [ self .refreshControl setTintColor:[UIColor redColor]]...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- SpringBoot2整合Redis,开启缓存,提高访问速度
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- Hadoop3单机部署,实现最简伪集群
- CentOS7,CentOS8安装Elasticsearch6.8.6
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- SpringBoot2编写第一个Controller,响应你的http请求并返回结果
- CentOS7安装Docker,走上虚拟化容器引擎之路
- CentOS8编译安装MySQL8.0.19
- Docker安装Oracle12C,快速搭建Oracle学习环境