Harmony Codelab样例—弹窗基本使用
一、介绍
本篇Codelab主要基于dialog和button组件,实现弹窗的几种自定义效果,具体效果有:
1. 警告弹窗,点击确认按钮弹窗关闭。
2. 确认弹窗,点击取消按钮或确认按钮,触发对应操作。
3. 加载弹窗,展示加载中效果。
4. 提示弹窗,支持用户输入内容,点击取消和确认按钮,触发对应操作。
5. 进度条弹窗,展示进度条以及百分比。
相关概念
dialog组件:自定义弹窗容器组件。
button组件:按钮组件。
完整示例
源码下载
二、环境搭建
我们首先需要完成HarmonyOS开发环境搭建,可参照如下步骤进行。
软件要求
DevEco Studio版本:DevEco Studio 3.1 Release。
HarmonyOS SDK版本:API version 9。
硬件要求
设备类型:华为手机或运行在DevEco Studio上的华为手机设备模拟器。
HarmonyOS系统:3.1.0 Developer Release。
环境搭建
1. 安装DevEco Studio,详情请参考下载和安装软件。
2. 设置DevEco Studio开发环境,DevEco Studio开发环境需要依赖于网络环境,需要连接上网络才能确保工具的正常使用,可以根据如下两种情况来配置开发环境:
● 如果可以直接访问Internet,只需进行下载HarmonyOS SDK操作。
● 如果网络不能直接访问Internet,需要通过代理服务器才可以访问,请参考配置开发环境。
3. 开发者可以参考以下链接,完成设备调试的相关配置:
● 使用真机进行调试
● 使用模拟器进行调试
三、代码结构解读
本篇Codelab只对核心代码进行讲解,对于完整代码,我们会在源码下载或gitee中提供。
├──entry/src/main/js // 代码区 │ └──MainAbility │ ├──common │ │ └──images // 图片资源 │ ├──i18n // 国际化中英文 │ │ ├──en-US.json │ │ └──zh-CN.json │ ├──pages │ │ └──index │ │ ├──index.css // 页面整体布局以及弹窗样式 │ │ ├──index.hml // 自定义弹窗展示页面 │ │ └──index.js // 弹窗显示关闭逻辑以及动画逻辑 │ └──app.js // 程序入口 └──entry/src/main/resources // 应用资源目录
四、构建应用页面
界面主要包括按钮列表页和自定义弹窗两部分,我们可以通过在dialog标签中添加自定义组件设置弹窗,具体效果如图所示:
首先搭建index.hml中的按钮页,主要包括5种常见的弹窗,分别为AlertDialog、ConfirmDialog、LoadingDialog、PromptDialog以及ProgressDialog。
<!--index.hml--> <div class="btn-div"> <button type="capsule" value="AlertDialog" class="btn" onclick="showAlert"></button> <button type="capsule" value="ConfirmDialog" class="btn" onclick="showConfirm"></button> <button type="capsule" value="LoadingDialog" class="btn" onclick="showLoading"></button> <button type="capsule" value="PromptDialog" class="btn" onclick="showPrompt"></button> <button type="capsule" value="ProgressDialog" class="btn" onclick="showProgress"></button> </div>
然后在index.hml中创建AlertDialog自定义弹窗,效果如图所示:
<!-- index.hml --> <!-- AlertDialog自定义弹窗 --> <dialog id="alertDialog" class="alert-dialog"> <div class="dialog-div"> <div class="alert-inner-txt"> <text class="txt">AlertDialog</text> </div> <div class="alert-inner-btn"> <button class="btn-single" type="capsule" value="Confirm" onclick="confirmClick('alertDialog')"></button> </div> </div> </dialog>
创建ConfirmDialog自定义弹窗,效果如图所示:
<!-- index.hml --> <!-- ConfirmDialog自定义弹窗 --> <dialog id="confirmDialog" class="dialog-main"> <div class="dialog-div"> <div class="inner-txt"> <text class="txt">ConfirmDialog</text> </div> <div class="inner-btn"> <button type="capsule" value="Cancel" class="btn-txt-left" onclick="cancelClick('confirmDialog')"></button> <button type="capsule" value="Confirm" class="btn-txt-right" onclick="confirmClick('confirmDialog')"></button> </div> </div> </dialog>
创建LoadingDialog自定义弹窗,效果如图所示:
<!-- index.hml --> <!-- LoadingDialog自定义弹窗 --> <dialog id="loadingDialog" class="low-height-dialog"> <div class="dialog-loading"> <text>Loading...</text> <image class="loading-img img-rotate" id="loading-img" src="/common/images/ic_loading.svg"></image> </div> </dialog>
创建PromptDialog自定义弹窗,效果如图所示:
<!-- index.hml --> <!-- PromptDialog自定义弹窗 --> <dialog id="promptDialog" class="dialog-prompt"> <div class="dialog-div-prompt"> <div class="inner-txt-prompt"> <text class="txt">PromptDialog</text> </div> <input class="prompt-input" type="password" placeholder="please enter password"></input> <div class="inner-btn"> <button type="capsule" value="Cancel" class="btn-txt-left" onclick="cancelClick('promptDialog')"></button> <button type="capsule" value="Confirm" class="btn-txt-right" onclick="confirmClick('promptDialog')"></button> </div> </div> </dialog>
创建ProgressDialog自定义弹窗,效果如图所示:
<!-- index.hml --> <!-- ProgressDialog自定义弹窗 --> <dialog id="progressDialog" class="low-height-dialog" oncancel="onCancel"> <div class="dialog-progress-div"> <div class="inner-txt-progress"> <text class="download-txt">Downloading...</text> <text>{{ percent + '%' }}</text> </div> <div class="progress-div"> <progress class="min-progress" type="horizontal" percent="{{ percent }}" secondarypercent="50"></progress> </div> </div> </dialog>
然后在index.js文件中实现不同button的点击事件,展示对应自定义弹窗:
// index.js export default { data: {...}, // 展示AlertDialog showAlert() { this.$element('alertDialog').show(); }, // 展示ConfirmDialog showConfirm() { this.$element('confirmDialog').show(); }, // 展示LoadingDialog showLoading() { ... this.animation = this.$element('loading-img').animate(frames, options); this.animation.play(); this.$element('loadingDialog').show(); }, // 展示PromptDialog showPrompt() { this.$element('promptDialog').show(); }, // 展示ProgressDialog showProgress() { ... } }
五、总结
您已经完成了本次Codelab的学习,并了解到以下知识点:
1. dialog自定义弹窗容器组件的使用。
2. button按钮组件的使用。
本文由博客一文多发平台 OpenWrite 发布!
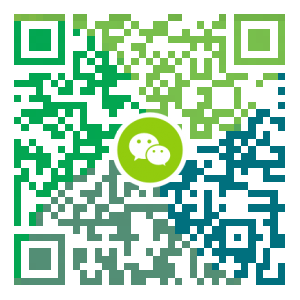
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
Text2Cypher:大语言模型驱动的图查询生成
话接上文《图技术在 LLM 下的应用:知识图谱驱动的大语言模型 Llama Index》 同大家简单介绍过 LLM 和图、知识图谱相关的结合,现在我来和大家分享下最新的成果。毕竟,从 GPT-3 开始展现出超出预期的“理解能力“开始,我一直在做 Graph + LLM 技术组合、互补的研究、探索和分享,截止到现在 NebulaGraph 已经在 LlamaIndex 与 Langchain 项目做出了不少领先的贡献。 是时候,来给你展示展示我的劳动成果了。本文的主题是我们认为这个 LLM+ 领域最唾手可得、最容易摘取的果实,Text2Cypher:自然语言生成图查询。 Text2Cypher 顾名思义,Text2Cypher 做的就是把自然语言的文本转换成 Cypher 查询语句的这件事儿。和另一个大家可能已经比较熟悉的场景 Text2SQL:文本转换 SQL 在形式上没有什么区别。而本质上,大多数知识图谱、图数据库的应用都是在图上按照人类意愿进行查询,我们在图数据库上构造方便的可视化工具、封装方便的 API 的工作都是为这个目标服务的。 一直以来,阻碍图数据库、知识图谱被更广泛应用的...
- 下一篇
HarmonyOS 管理页面跳转及浏览记录导航
历史记录导航 使用者在前端页面点击网页中的链接时,Web组件默认会自动打开并加载目标网址。当前端页面替换为新的加载链接时,会自动记录已经访问的网页地址。可以通过forward()和backward()接口向前/向后浏览上一个/下一个历史记录。 在下面的示例中,点击应用的按钮来触发前端页面的后退操作。 // xxx.ets import web_webview from '@ohos.web.webview'; @Entry @Component struct WebComponent { webviewController: web_webview.WebviewController = new web_webview.WebviewController(); build() { Column() { Button('loadData') .onClick(() => { if (this.webviewController.accessBackward()) { this.webviewController.backward(); return true; } }) Web({...
相关文章
文章评论
共有0条评论来说两句吧...