Mybatis-Flex 第一个版本发布,一个优雅的 Mybatis 增强框架
Mybatis-Flex 第一个版本发布,一个优雅的 Mybatis 增强框架。
特征
- 1、很轻量,整个框架只依赖 Mybatis 再无其他第三方依赖
- 2、Entity 类的基本增删改查、以及分页查询
- 3、Row 通用映射支持,可以无需实体类对数据库进行增删改查
- 4、支持多种数据库类型,自由通过方言持续扩展
- 5、支持联合主键,以及不同的主键内容生成策略
- 6、极其友好的 SQL 联动查询,IDE 自动提示不再担心出错
- 7、更多小惊喜
hello world
第一步:编写 Entity 实体类
@Table("tb_account") public class Account { @Id() private Long id; private String userName; private Date birthday; private int sex; //getter setter }
第二步,编写 Mapper 类,并继承 BaseMapper
public interface AccountMapper extends BaseMapper<Account> { //只需定义 Mapper 接口即可,可以无任何内容。 }
第三步:开始查询数据
示例 1:查询 1 条数据
class HelloWorld { public static void main(String... args) { HikariDataSource dataSource = new HikariDataSource(); dataSource.setJdbcUrl("jdbc:mysql://127.0.0.1:3306/mybatis-flex"); dataSource.setUsername("username"); dataSource.setPassword("password"); MybatisFlexBootstrap.getInstance() .setDatasource(dataSource) .addMapper(AccountMapper.class) .start(); //示例1:查询 id=100 条数据 Account account = MybatisFlexBootstrap.getInstance() .execute(AccountMapper.class, mapper -> mapper.selectOneById(100) ); } }
示例2:查询列表
class HelloWorld { public static void main(String... args) { HikariDataSource dataSource = new HikariDataSource(); dataSource.setJdbcUrl("jdbc:mysql://127.0.0.1:3306/mybatis-flex"); dataSource.setUsername("username"); dataSource.setPassword("password"); MybatisFlexBootstrap.getInstance() .setDatasource(dataSource) .addMapper(AccountMapper.class) .start(); //示例2:通过 QueryWrapper 构建条件查询数据列表 QueryWrapper query = QueryWrapper.create() .select() .from(ACCOUNT) .where(ACCOUNT.ID.ge(100)) .and(ACCOUNT.USER_NAME.like("张").or(ACCOUNT.USER_NAME.like("李"))); // 执行 SQL: // ELECT * FROM `tb_account` // WHERE `tb_account`.`id` >= 100 // AND (`tb_account`.`user_name` LIKE '%张%' OR `tb_account`.`user_name` LIKE '%李%' ) List<Account> accounts = MybatisFlexBootstrap.getInstance() .execute(AccountMapper.class, mapper -> mapper.selectListByQuery(query) ); } }
示例3:分页查询
class HelloWorld { public static void main(String... args) { HikariDataSource dataSource = new HikariDataSource(); dataSource.setJdbcUrl("jdbc:mysql://127.0.0.1:3306/mybatis-flex"); dataSource.setUsername("username"); dataSource.setPassword("password"); MybatisFlexBootstrap.getInstance() .setDatasource(dataSource) .addMapper(AccountMapper.class) .start(); // 示例3:分页查询 // 查询第 5 页,每页 10 条数据,通过 QueryWrapper 构建条件查询 QueryWrapper query = QueryWrapper.create() .select() .from(ACCOUNT) .where(ACCOUNT.ID.ge(100)) .and(ACCOUNT.USER_NAME.like("张").or(ACCOUNT.USER_NAME.like("李"))) .orderBy(ACCOUNT.ID.desc()); // 执行 SQL: // ELECT * FROM `tb_account` // WHERE `tb_account`.`id` >= 100 // AND (`tb_account`.`user_name` LIKE '%张%' OR `tb_account`.`user_name` LIKE '%李%' ) // ORDER BY `tb_account`.`id` DESC // LIMIT 40,10 Page<Account> accounts = MybatisFlexBootstrap.getInstance() .execute(AccountMapper.class, mapper -> mapper.paginate(5, 10, query) ); } }
QueryWrapper 示例
select *
QueryWrapper query=new QueryWrapper(); query.select().from(ACCOUNT) // SQL: // SELECT * FROM tb_account
select columns
QueryWrapper query=new QueryWrapper(); query.select(ACCOUNT.ID,ACCOUNT.USER_NAME).from(ACCOUNT) // SQL: // SELECT tb_account.id, tb_account.user_name // FROM tb_account
QueryWrapper query=new QueryWrapper(); query.select(ACCOUNT.ALL_COLUMNS).from(ACCOUNT) // SQL: // SELECT tb_account.id, tb_account.user_name, tb_account.birthday, // tb_account.sex, tb_account.is_normal // FROM tb_account
select functions
QueryWrapper query=new QueryWrapper() .select(ACCOUNT.ID ,ACCOUNT.USER_NAME ,max(ACCOUNT.BIRTHDAY) ,avg(ACCOUNT.SEX).as("sex_avg")) .from(ACCOUNT); // SQL: // SELECT tb_account.id, tb_account.user_name, // MAX(tb_account.birthday), // AVG(tb_account.sex) AS sex_avg // FROM tb_account
where
QueryWrapper queryWrapper=QueryWrapper.create() .select() .from(ACCOUNT) .where(ACCOUNT.ID.ge(100)) .and(ACCOUNT.USER_NAME.like("michael")); // SQL: // SELECT * FROM tb_account // WHERE tb_account.id >= ? // AND tb_account.user_name LIKE ?
exists, not exists
QueryWrapper queryWrapper=QueryWrapper.create() .select() .from(ACCOUNT) .where(ACCOUNT.ID.ge(100)) .and( exist( selectOne().from(ARTICLE).where(ARTICLE.ID.ge(100)) ) ); // SQL: // SELECT * FROM tb_account // WHERE tb_account.id >= ? // AND EXIST ( // SELECT 1 FROM tb_article WHERE tb_article.id >= ? // )
and (...) or (...)
QueryWrapper queryWrapper=QueryWrapper.create() .select() .from(ACCOUNT) .where(ACCOUNT.ID.ge(100)) .and(ACCOUNT.SEX.eq(1).or(ACCOUNT.SEX.eq(2))) .or(ACCOUNT.AGE.in(18,19,20).or(ACCOUNT.USER_NAME.like("michael"))); // SQL: // SELECT * FROM tb_account // WHERE tb_account.id >= ? // AND (tb_account.sex = ? OR tb_account.sex = ? ) // OR (tb_account.age IN (?,?,?) OR tb_account.user_name LIKE ? )
group by
QueryWrapper queryWrapper=QueryWrapper.create() .select() .from(ACCOUNT) .groupBy(ACCOUNT.USER_NAME); // SQL: // SELECT * FROM tb_account // GROUP BY tb_account.user_name
having
QueryWrapper queryWrapper=QueryWrapper.create() .select() .from(ACCOUNT) .groupBy(ACCOUNT.USER_NAME) .having(ACCOUNT.AGE.between(18,25)); // SQL: // SELECT * FROM tb_account // GROUP BY tb_account.user_name // HAVING tb_account.age BETWEEN ? AND ?
jion
QueryWrapper queryWrapper = QueryWrapper.create() .select() .from(ACCOUNT) .leftJoin(ARTICLE).on(ACCOUNT.ID.eq(ARTICLE.ACCOUNT_ID)) .where(ACCOUNT.AGE.ge(10)); // SQL: // SELECT * FROM tb_account // LEFT JOIN tb_article // ON tb_account.id = tb_article.account_id // WHERE tb_account.age >= ?
可能存在问题
如何通过实体类 Account.java 生成 QueryWrapper 所需要的 "ACCOUNT" 类 ?
答:通过开发工具构建项目(如下图),或者执行 maven 编译命令: mvn clean package
更多示例
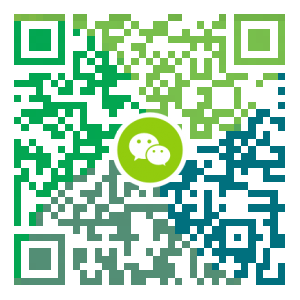
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
首家开源商城 BeikeShop 已免费集成 ChatGPT!!!
前言:跨境电商行业在近几年持续升温,越来越多的商家开始尝试跨境电商的模式 作为一款开源跨境电商系统,BeikeShop正在以强大的功能、易上手的操作方式和良好的用户体验,吸引越来越多的跨境卖家 在最新发布的v1.3.2版本中,BeikeShop集成了ChatGPT聊天插件,让卖家可以轻松使用智能聊天工具,提高服务质量 ✦✦ 一、BeikeShop集成ChatGPT! BeikeShop是一款基于Laravel开发的开源商城系统,主要面向外贸/跨境电商行业提供商品管理、订单管理、会员管理、支付、物流、系统管理等功能 在全新推出的BeikeShop_v1.3.2版本中,我们集成了ChatGPT聊天插件!卖家可以在安装/升级BeikeShop系统后,登录系统后台直接与ChatGPT对话! 未来规划中,BeikeShop的用户可以通过聊天界面进行商品咨询、下单、查询物流等操作,无需繁琐的点击操作,这将会极大提升跨境卖家的运营效率! 我们认为ChatGPT对于使用BeikeShop的卖家中,主要有这4个应用方向: 1. 生成描述 / 快速翻译 在跨境建站过程中,他可以根据你对商品的描述,快捷的生...
- 下一篇
ShopWind 多商户商城更新,Vue 3 前后端分离,页面自定义装修
本次为 V4版本更新,新系统架构(技术栈)vue3+vite(打包编译工具)+CompositionAPI(组合式APIsetup)+ElementPlus+vueRouter(路由) 第三方组件:axios(数据请求)+wangeditor(编辑器),都是通过接口访问数据,页面效果更佳了。。 官网网址:https://www.shopwind.net 开发文档:https://developer.shopwind.net API 接口文档:http://docs.shopwind.net 开发者社区:https://forum.shopwind.net PC 体验 前台体验:https://v4.shopwind.net 后台体验:https://v4.shopwind.net/admin 商家体验:https://v4.shopwind.net/seller/login/index 页面展示 首页 / 用户中心 / 分类页 产品详情 / 购物车 / 下单页 平台后台管理 丰富的功能插件 可视化模板编辑 / DIY 页面布局 更新内容 新增API接口(获取库存预警商品列表) 新增采集...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS7安装Docker,走上虚拟化容器引擎之路
- SpringBoot2编写第一个Controller,响应你的http请求并返回结果
- CentOS关闭SELinux安全模块
- CentOS7,CentOS8安装Elasticsearch6.8.6
- Jdk安装(Linux,MacOS,Windows),包含三大操作系统的最全安装
- Linux系统CentOS6、CentOS7手动修改IP地址
- Windows10,CentOS7,CentOS8安装MongoDB4.0.16
- CentOS8安装Docker,最新的服务器搭配容器使用
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- SpringBoot2配置默认Tomcat设置,开启更多高级功能