【本人秃顶程序员】使用Spring Cloud Stream和RabbitMQ实现事件驱动的微服务
←←←←←←←←←←←← 快!点关注
让我们展示如何使用Spring Cloud Stream来设计事件驱动的微服务。首先,Spring Cloud Stream首先有什么好处?因为Spring AMPQ提供了访问AMPQ工件所需的一切。如果您不熟悉Spring AMPQ,请查看此repo,其中包含许多有用的示例。那么为什么要使用Spring Cloud Stream ......?
Spring Cloud Stream概念
- Spring Cloud Stream通过Binder概念将使用过的消息代理与Spring Integration消息通道绑定在一起。支持RabbitMQ和Kafka。
- Spring Cloud Stream将基础架构配置从代码中分离为属性文件。这意味着即使您更改了底层代理,您的Spring Integration代码也将是相同的!
示例中的Spring Cloud Stream概念(RabbitMQ)
让我们有一个名为streamInput的交换,它有两个队列streamInput.cities和streamInput.persons。现在让我们将这两个队列插入两个消息通道citiesChannel和personsChannel来消费来自它的传入消息。使用Spring AMPQ,您需要创建SimpleMessageListenerContainer并在代码中连接基础结构。但这有很多样板代码。使用Spring Cloud Stream,您可以将AMPQ配置分离到属性文件:
spring.cloud.stream.bindings.citiesChannel.destination=streamInput spring.cloud.stream.bindings.citiesChannel.group=cities spring.cloud.stream.rabbit.bindings.citiesChannel.consumer.durableSubscription=true spring.cloud.stream.rabbit.bindings.citiesChannel.consumer.bindingRoutingKey=cities spring.cloud.stream.bindings.personsChannel.destination=streamInput spring.cloud.stream.bindings.personsChannel.group=persons spring.cloud.stream.rabbit.bindings.personsChannel.consumer.durableSubscription=true spring.cloud.stream.rabbit.bindings.personsChannel.consumer.bindingRoutingKey=persons
配置详细信息
在类路径上使用RabbitMQ Binder,每个目标都映射到TopicExchange。在示例中,我创建了名为streamInput的TopicExchange, 并将其附加到两个消息通道citiesChannel和personsChannel。
spring.cloud.stream.bindings.citiesChannel.destination = streamInput spring.cloud.stream.bindings.personsChannel.destination = streamInput
现在您需要了解RabbitMQ绑定器的灵感来自Kafka,队列的消费者被分组到消费者组中,其中只有一个消费者将获得消息。这是有道理的,因为您可以轻松扩展消费者。
因此,让我们创建两个队列streamInput.persons和streamInput.cities并将它们附加到streamInput TopicExchange和提到的消息通道
# This will create queue "streamInput.cities" connected to message channel citiesChannel where input messages will land. spring.cloud.stream.bindings.citiesChannel.group=cities # Durable subscription, of course. spring.cloud.stream.rabbit.bindings.citiesChannel.consumer.durableSubscription=true # AMPQ binding to exchange (previous spring.cloud.stream.bindings.<channel name>.destination settings). # Only messages with routingKey = 'cities' will land here. spring.cloud.stream.rabbit.bindings.citiesChannel.consumer.bindingRoutingKey=cities spring.cloud.stream.bindings.personsChannel.group=persons spring.cloud.stream.rabbit.bindings.personsChannel.consumer.durableSubscription=true spring.cloud.stream.rabbit.bindings.personsChannel.consumer.bindingRoutingKey=persons
连接属性到Spring Integration
好的,到目前为止我创建了两个队列。StreamInput.cities绑定到citiesChannel。StreamInput.persons绑定到peopleChannel。
<destination>.<group>是Spring Cloud Stream约定的队列命名,现在让我们将它连接到Spring Integration:
package com.example.spring.cloud.configuration; import org.springframework.cloud.stream.annotation.Input; import org.springframework.messaging.SubscribableChannel; /** \* Created by tomask79 on 30.03.17. */ public interface SinkRabbitAPI { String INPUT_CITIES = "citiesChannel"; String INPUT_PERSONS = "personsChannel"; @Input(SinkRabbitAPI.INPUT_CITIES) SubscribableChannel citiesChannel(); @Input(SinkRabbitAPI.INPUT_PERSONS) SubscribableChannel personsChannel(); }
Spring Boot启动时加载这个属性
package com.example.spring.cloud; import com.example.spring.cloud.configuration.SinkRabbitAPI; import com.example.spring.cloud.configuration.SourceRabbitAPI; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.stream.annotation.EnableBinding; import org.springframework.scheduling.annotation.EnableScheduling; @SpringBootApplication @EnableBinding({SinkRabbitAPI.class}) public class StreamingApplication { public static void main(String\[\] args) { SpringApplication.run(StreamingApplication.class, args); } }
在此之后,我们可以创建消费者从绑定的消息通道中的队列接收消息:
import com.example.spring.cloud.configuration.SinkRabbitAPI; import com.example.spring.cloud.configuration.SourceRabbitAPI; import org.springframework.cloud.stream.annotation.StreamListener; import org.springframework.integration.support.MessageBuilder; import org.springframework.messaging.MessageChannel; import org.springframework.messaging.handler.annotation.SendTo; import org.springframework.stereotype.Service; import javax.annotation.Resource; /** \* Created by tomask79 on 30.03.17. */ @Service public class ProcessingAMPQEndpoint { @StreamListener(SinkRabbitAPI.INPUT_CITIES) public void processCity(final String city) { System.out.println("Trying to process input city: "+city); } @StreamListener(SinkRabbitAPI.INPUT_PERSONS) public void processPersons(final String person) { System.out.println("Trying to process input person: "+person); } }
RabbitMQ绑定器和代理配置
Spring Cloud Stream如何知道在哪里寻找消息中间件?如果在类路径中找到RabbitMQ绑定器,则使用默认RabbitMQ主机(localhost)和端口(5672)连接到RabbitMQ服务器。如果您的消息中间件配置在不同端口,则需要配置属性:
spring: cloud: stream: bindings: ... binders: rabbitbinder: type: rabbit environment: spring: rabbitmq: host: rabbitmq port: 5672 username: XXX password: XXX
测试消息消费
- 安装并运行RabbitMQ代理
- rabbitmq.git
- mvn clean install
- java -jar target / streaming-0.0.1-SNAPSHOT.jar
- 现在使用路由键'cities'或'persons'在streamInput Exchange上发布消息...输出应该是:
Started StreamingApplication in 6.513 seconds (JVM running for 6.92) Trying to process input city: sdjfjljksdflkjsdflkjsdfsfd Trying to process input person: sdjfjljksdflkjsdflkjsdfsfd
使用Spring Cloud Stream重新传递消息
您通常希望在进入DLX交换之前再次尝试接收消息。首先,让我们配置Spring Cloud Stream尝试重新发送失败消息的次数:
spring.cloud.stream.bindings.personsChannel.consumer.maxAttempts = 6
这意味着如果从streamInput.persons队列接收的消息出错,那么Spring Cloud Stream将尝试重新发送六次。让我们试试,首先让我们修改接收端点以模拟接收崩溃:
@StreamListener(SinkRabbitAPI.INPUT_PERSONS) public void processPersons(final String person) { System.out.println("Trying to process input person: "+person); throw new RuntimeException(); }
如果我现在尝试使用人员路由键将某些内容发布到streamInput交换中,那么这应该是输出:
Trying to process input person: sfsdfsdfsd Trying to process input person: sfsdfsdfsd Trying to process input person: sfsdfsdfsd Trying to process input person: sfsdfsdfsd Trying to process input person: sfsdfsdfsd Trying to process input person: sfsdfsdfsd Retry Policy Exhausted at org.springframework.amqp.rabbit.retry.RejectAndDontRequeueRecoverer.recover (RejectAndDontRequeueRecoverer.java:45) ~\[spring-rabbit-1.7.0.RELEASE.jar! /:na\] at org.springframework.amqp.rabbit.config.StatelessRetryOperationsInterc
建议将Spring Cloud Stream 用于事件驱动的MicroServices,因为它可以节省时间,而且您不需要为Java中的AMPQ基础架构编写样板代码。
写在最后:
秃顶程序员的不易,看到这里,点了关注吧!
点关注,不迷路,持续更新!!!
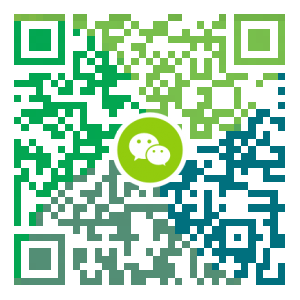
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
Docker镜像细节
前言 只有光头才能变强。 文本已收录至我的GitHub仓库,欢迎Star:https://github.com/ZhongFuCheng3y/3y 回顾前面: 为什么需要Docker? Docker入门为什么可以这么简单? 前面两篇已经讲解了为什么需要Docker这项技术,以及解释了Docker的基本概念/术语,使用Docker成功运行Tomcat~ 在上篇也同样留下一个问题:我们知道Tomcat运行起来需要Java的支持,那么我们在DockerHub拉取下来的Tomcat镜像是不是也有Java环境呢? 所以,这篇主要来讲讲Docker镜像相关的知识点! 一、简单了解Dockerfile Dockerfile是用来构建Docker镜像的文件,是由一系列命令和参数构成的脚本。 简单来说:Dockerfile是镜像的源码。 上一篇我们pull了一份Tomcat的镜像,我们也可以去看看它的Dockerfile长的什么样: 我们随便点进去一个看一下: 我们在Dockerfile的第一行就可以发现FROM openjdk:8-jre,所以可以确定的是:在DockerHub拉取下来的Tomcat镜...
- 下一篇
java异常处理01
java异常处理高级技巧 什么是Java异常? 当Java程序的正常行为被意外行为中断时,会发生故障。这种故障被称为异常。例如,程序尝试打开文件以读取其内容,但该文件不存在将产生异常。Java将异常分为几种类型,所以让我们考虑每一种类型。 检查异常 Java将(例如FileNotFoundException, IOException)引起的异常分类为已检查的异常。Java编译器会检查这些异常,并且在异常发生的位置要求进行捕获处理或者向上抛出(throws)。需要注意的是检查异常属于编译器的行为,要求你必须在代码中捕获或向上抛出(throws)。 运行时(非检查)异常 例如程序进行强制转换(cast),这种可能存在转换失败的异常就是另一种异常。即运行时异常(RuntimeException)。和检查不同,编译器不会检查你在代码中是否进行处理或抛出。运行时异常通常来自编写的不良代码,因此应由程序员修复。 错误(Error) 指一些非常严重,通常无法进行修正必须要重启程序的异常。例如, 尝试从JVM分配内存,但没有足够的可用内存来满足请求(OutOfMemoryError)。运行时尝试调用加...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- Docker安装Oracle12C,快速搭建Oracle学习环境
- CentOS7安装Docker,走上虚拟化容器引擎之路
- Linux系统CentOS6、CentOS7手动修改IP地址
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- CentOS7编译安装Cmake3.16.3,解决mysql等软件编译问题
- CentOS关闭SELinux安全模块
- CentOS7编译安装Gcc9.2.0,解决mysql等软件编译问题
- Hadoop3单机部署,实现最简伪集群
- CentOS6,7,8上安装Nginx,支持https2.0的开启