python爬虫---基本的模块,你一定要懂 ! !
前言
python爬虫,web spider。爬取网站获取网页数据,并进行分析提取。
基本模块使用的是 urllib,urllib2,re,等模块
(一)基本用法,例子
(1)进行基本GET请求,获取网页html
#!coding=utf-8 import urllib import urllib2 url = 'http://www.baidu.com/' # 获取请求 request = urllib2.Request(url) try: # 根据request,得到返回response response = urllib2.urlopen(request) except urllib2.HTTPError, e: if hasattr(e, 'reason'): print e.reason # 读取response的body html = response.read() # 读取response的headers headers = response.info()
(2)表单提交
#!coding=utf-8 import urllib2 import urllib post_url = '' post_data = urllib.urlencode({ 'username': 'username', 'password': 'password', }) post_headers = { 'User-Agent': 'Mozilla/5.0 (X11; Ubuntu; Linux i686; rv:31.0) Gecko/20100101 Firefox/31.0', } request = urllib2.Request( url=post_url, data=post_data, headers=post_headers, ) response = urllib2.urlopen(request) html = response.read()
(3)
小编推荐一个学python的学习qun 491308659 验证码: 南烛
无论你是大牛还是小白,是想转行还是想入行都可以来了解一起进步一起学习!裙内有开发工具,很多干货和技术资料分享!
#!coding=utf-8 import urllib2 import re page_num = 1 url = 'http://tieba.baidu.com/p/3238280985?see_lz=1&pn='+str(page_num) myPage = urllib2.urlopen(url).read().decode('gbk') myRe = re.compile(r'class="d_post_content j_d_post_content ">(.*?)</div>', re.DOTALL) items = myRe.findall(myPage) f = open('baidu.txt', 'a+') import sys reload(sys) sys.setdefaultencoding('utf-8') i = 0 texts = [] for item in items: i += 1 print i text = item.replace('<br>', '') text.replace('\n', '').replace(' ', '') + '\n' print text f.write(text) f.close()
(4)
#coding:utf-8 ''' 模拟登陆163邮箱并下载邮件内容 ''' import urllib import urllib2 import cookielib import re import time import json class Email163: header = {'User-Agent':'Mozilla/5.0 (Windows; U; Windows NT 6.1; en-US; rv:1.9.1.6) Gecko/20091201 Firefox/3.5.6'} user = '' cookie = None sid = None mailBaseUrl='http://twebmail.mail.163.com' def __init__(self): self.cookie = cookielib.CookieJar() cookiePro = urllib2.HTTPCookieProcessor(self.cookie) urllib2.install_opener(urllib2.build_opener(cookiePro)) def login(self,user,pwd): ''' 登录 ''' postdata = urllib.urlencode({ 'username':user, 'password':pwd, 'type':1 }) #注意版本不同,登录URL也不同 req = urllib2.Request( url='https://ssl.mail.163.com/entry/coremail/fcg/ntesdoor2?funcid=loginone&language=-1&passtype=1&iframe=1&product=mail163&from=web&df=email163&race=-2_45_-2_hz&module=&uid='+user+'&style=10&net=t&skinid=null', data=postdata, headers=self.header, ) res = str(urllib2.urlopen(req).read()) #print res patt = re.compile('sid=([^"]+)',re.I) patt = patt.search(res) uname = user.split('@')[0] self.user = user if patt: self.sid = patt.group(1).strip() #print self.sid print '%s Login Successful.....'%(uname) else: print '%s Login failed....'%(uname) def getInBox(self): ''' 获取邮箱列表 ''' print '\nGet mail lists.....\n' sid = self.sid url = self.mailBaseUrl+'/jy3/list/list.do?sid='+sid+'&fid=1&fr=folder' res = urllib2.urlopen(url).read() #获取邮件列表 mailList = [] patt = re.compile('<div\s+class="tdLike Ibx_Td_From"[^>]+>.*?href="([^"]+)"[^>]+>(.*?)<\/a>.*?<div\s+class="tdLike Ibx_Td_Subject"[^>]+>.*?href="[^>]+>(.*?)<\/a>',re.I|re.S) patt = patt.findall(res) if patt==None: return mailList for i in patt: line = { 'from':i[1].decode('utf8'), 'url':self.mailBaseUrl+i[0], 'subject':i[2].decode('utf8') } mailList.append(line) return mailList def getMailMsg(self,url): ''' 下载邮件内容 ''' content='' print '\n Download.....%s\n'%(url) res = urllib2.urlopen(url).read() patt = re.compile('contentURL:"([^"]+)"',re.I) patt = patt.search(res) if patt==None: return content url = '%s%s'%(self.mailBaseUrl,patt.group(1)) time.sleep(1) res = urllib2.urlopen(url).read() Djson = json.JSONDecoder(encoding='utf8') jsonRes = Djson.decode(res) if 'resultVar' in jsonRes: content = Djson.decode(res)['resultVar'] time.sleep(3) return content ''' Demon ''' #初始化 mail163 = Email163() #登录 mail163.login('lpe234@163.com','944898186') time.sleep(2) #获取收件箱 elist = mail163.getInBox() #获取邮件内容 for i in elist: print '主题:%s 来自:%s 内容:\n%s'%(i['subject'].encode('utf8'),i['from'].encode('utf8')
(5)需要登陆的情况
#1 cookie的处理 import urllib2, cookielib cookie_support= urllib2.HTTPCookieProcessor(cookielib.CookieJar()) opener = urllib2.build_opener(cookie_support, urllib2.HTTPHandler) urllib2.install_opener(opener) content = urllib2.urlopen('http://XXXX').read() #2 用代理和cookie opener = urllib2.build_opener(proxy_support, cookie_support, urllib2.HTTPHandler) #3 表单的处理 import urllib postdata=urllib.urlencode({ 'username':'XXXXX', 'password':'XXXXX', 'continueURI':'http://www.verycd.com/', 'fk':fk, 'login_submit':'登录' }) req = urllib2.Request( url = 'http://secure.verycd.com/signin/*/http://www.verycd.com/', data = postdata ) result = urllib2.urlopen(req).read() #4 伪装成浏览器访问 headers = { 'User-Agent':'Mozilla/5.0 (Windows; U; Windows NT 6.1; en-US; rv:1.9.1.6) Gecko/20091201 Firefox/3.5.6' } req = urllib2.Request( url = 'http://secure.verycd.com/signin/*/http://www.verycd.com/', data = postdata, headers = headers ) #5 反”反盗链” headers = { 'Referer':'http://www.cnbeta.com/articles' }
(6)多线程
from threading import Thread from Queue import Queue from time import sleep #q是任务队列 #NUM是并发线程总数 #JOBS是有多少任务 q = Queue() NUM = 2 JOBS = 10 #具体的处理函数,负责处理单个任务 def do_somthing_using(arguments): print arguments #这个是工作进程,负责不断从队列取数据并处理 def working(): while True: arguments = q.get() do_somthing_using(arguments) sleep(1) q.task_done() #fork NUM个线程等待队列 for i in range(NUM): t = Thread(target=working) t.setDaemon(True) t.start() #把JOBS排入队列 for i in range(JOBS): q.put(i) #等待所有JOBS完成 q.join()
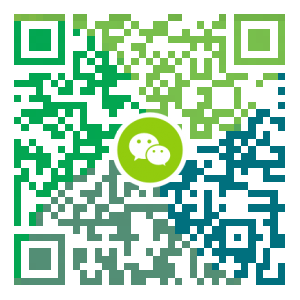
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
Java Spring Boot 2.0 API接口实战Swagger和Spring REST Docs帮助文档
内容摘要:基于Java Spring Boot 2.0可以快速开发REST API,但是如何根据API自动生成 Help Docs是非常重要的问题。本次课程详细介绍几种不同的Rest Help Docs的构建方式,Swagger和Rest Docs、OpenAPI核心原理与区别优缺点,并给出Demo代码。1、API开发、微服务帮助文档解决方法 企业应用接口,目前主要是REST API为主,针对前后端分离架构,或者移动多层架构开发,基于HTTP+JSON方式最为常见。但是API的接口文档一直是个比较棘手的问题,非常重要,几乎每个API开发者都会涉及到接口API文档的编写问题。为接口的调用者提供详细的API文档,成为非常重要的工作内容。 早期Web服务开发者可以使用WSDL,但是REST API无法自动生成文档,目前主流的解决办法,基本是
- 下一篇
var,let和const深入解析(一)
es6有许多特别棒的特性,你可能对该语言的整体非常熟悉,但是你知道它在内部是如何工作的吗?当我们知道它的内部原理以后,我们使用起来也会更加的安心一些。这里我们想逐步的引导你,让你对其有一个更深入,更浅显的认识。让我们就先从es6中的变量开始讲起吧。 let和const 在es6中新引入了两种方式来申明变量,我们仍然可以使用广为传诵的var变量(然而你不应该继续使用它了,继续阅读来了解其中原因),但是现在我们有了两种更牛的工具去使用:let和const。 let let和var非常的相似,在使用方面,你可以使用完全相同的方式来声明变量,例如: let myNewVariable = 2; var myOldVariable = 3; console.log(myNewVariable); // 2 console.log(myOldVariable); // 3 但是实际上,他们之间有几处明显的不同。他们不仅仅是关键字变了,而且实际上它还让会简化我们的一些工作,防止一些奇怪的bug,其中这些不同点是: let是块状作用域(我将会在文章后面着重讲一下作用域相关的东西),而var是函数作用域...
相关文章
文章评论
共有0条评论来说两句吧...