[雪峰磁针石博客]python GUI作业:使用tkinter的重要控件
题目1:使用tkinter的重要控件 绘制如下菜单:
参考代码:
#!/usr/bin/env python3 # -*- coding: utf-8 -*- # 技术支持:https://www.jianshu.com/u/69f40328d4f0 # 技术支持 https://china-testing.github.io/ # https://github.com/china-testing/python-api-tesing/blob/master/practices/tk/tk3.py # 项目实战讨论QQ群630011153 144081101 # CreateDate: 2018-11-29 import tkinter as tk root = tk.Tk() root.title('tkinter控件') #create a frame widget for placing menu my_menu_bar = tk.Frame(root, relief='raised', bd=2) my_menu_bar.pack(fill=tk.X) # Create Menu Widget 1 and Sub Menu 1 my_menu_button = tk.Menubutton( my_menu_bar, text='菜单1', ) my_menu_button.pack(side=tk.LEFT) #menu widget my_menu = tk.Menu(my_menu_button, tearoff=0) my_menu_button['menu'] = my_menu my_menu.add('command', label='子菜单1') #Add Sub Menu 1 # Create Menu2 and Submenu2 menu_button_2 = tk.Menubutton( my_menu_bar, text='菜单2', ) menu_button_2.pack(side=tk.LEFT) my_menu_2 = tk.Menu(menu_button_2, tearoff=0) menu_button_2['menu'] = my_menu_2 my_menu_2.add('command', label='子菜单2') # Add Sub Menu 2 # # # my_frame_1 and its contents # # creating a frame (my_frame_1) my_frame_1 = tk.Frame(root, bd=2, relief=tk.SUNKEN) my_frame_1.pack(side=tk.LEFT) # add label to to my_frame_1 tk.Label(my_frame_1, text='标签').pack() #add entry widget to my_frame_1 tv = tk.StringVar() #discussed later tk.Entry(my_frame_1, textvariable=tv).pack() tv.set('I am an entry widget') #add button widget to my_frame_1 tk.Button(my_frame_1, text='tk.Button widget').pack() #add check button widget to my_frame_1 tk.Checkbutton(my_frame_1, text='Checktk.Button Widget').pack() #add radio buttons to my_frame_1 tk.Radiobutton(my_frame_1, text='Radio tk.Button Un', value=1).pack() tk.Radiobutton(my_frame_1, text='Radio tk.Button Dos', value=2).pack() tk.Radiobutton(my_frame_1, text='Radio tk.Button Tres', value=3).pack() #tk.OptionMenu Widget tk.Label(my_frame_1, text='Example of tk.OptionMenu Widget:').pack() tk.OptionMenu(my_frame_1, '', "Option A", "Option B", "Option C").pack() #adding my_image image tk.Label(my_frame_1, text='Image Fun with Bitmap Class:').pack() my_image = tk.BitmapImage(file="gir.xbm") my_label = tk.Label(my_frame_1, image=my_image) my_label.image = my_image # keep a reference! my_label.pack() # # # frame2 and widgets it contains. # # #create another frame(my_frame_2) to hold a list box, Spinbox Widget,Scale Widget, : my_frame_2 = tk.Frame(root, bd=2, relief=tk.GROOVE) my_frame_2.pack(side=tk.RIGHT) #add Photimage Class Widget to my_frame_2 tk.Label( my_frame_2, text='Image displayed with \nPhotoImage class widget:').pack() dance_photo = tk.PhotoImage(file='dance.gif') dance_photo_label = tk.Label(my_frame_2, image=dance_photo) dance_photo_label.image = dance_photo dance_photo_label.pack() #add my_listbox widget to my_frame_2 tk.Label(my_frame_2, text='Below is an example of my_listbox widget:').pack() my_listbox = tk.Listbox(my_frame_2, height=4) for line in ['Listbox Choice 1', 'Choice 2', 'Choice 3', 'Choice 4']: my_listbox.insert(tk.END, line) my_listbox.pack() #spinbox widget tk.Label(my_frame_2, text='Below is an example of spinbox widget:').pack() tk.Spinbox(my_frame_2, values=(1, 2, 4, 8, 10)).pack() #scale widget tk.Scale( my_frame_2, from_=0.0, to=100.0, label='Scale widget', orient=tk.HORIZONTAL).pack() #LabelFrame label_frame = tk.LabelFrame( my_frame_2, text="LabelFrame Widget", padx=10, pady=10) label_frame.pack(padx=10, pady=10) tk.Entry(label_frame).pack() #message widget tk.Message(my_frame_2, text='I am a Message widget').pack() # # # tk.Frame 3 # # my_frame_3 = tk.Frame(root, bd=2, relief=tk.SUNKEN) #text widget and associated tk.Scrollbar widget my_text = tk.Text(my_frame_3, height=10, width=40) file_object = open('textcontent.txt', encoding='utf-8') file_content = file_object.read() file_object.close() my_text.insert(tk.END, file_content) my_text.pack(side=tk.LEFT, fill=tk.X, padx=5) #add scrollbar widget to the text widget my_scrollbar = tk.Scrollbar(my_frame_3, orient=tk.VERTICAL, command=my_text.yview) my_scrollbar.pack(side=tk.RIGHT, fill=tk.Y) my_text.configure(yscrollcommand=my_scrollbar.set) my_frame_3.pack() # # # tk.Frame 4 # # #create another frame(my_frame_4) my_frame_4 = tk.Frame(root) my_frame_4.pack() my_canvas = tk.Canvas(my_frame_4, bg='white', width=340, height=80) my_canvas.pack() my_canvas.create_oval(20, 15, 60, 60, fill='red') my_canvas.create_oval(40, 15, 60, 60, fill='grey') my_canvas.create_text( 130, 38, text='I am a tk.Canvas Widget', font=('arial', 8, 'bold')) # # # A paned window widget # # tk.Label(root, text='Below is an example of Paned window widget:').pack() tk.Label( root, text='Notice you can adjust the size of each pane by dragging it').pack() my_paned_window_1 = tk.PanedWindow() my_paned_window_1.pack(fill=tk.BOTH, expand=2) left_pane_text = tk.Text(my_paned_window_1, height=6, width=15) my_paned_window_1.add(left_pane_text) my_paned_window_2 = tk.PanedWindow(my_paned_window_1, orient=tk.VERTICAL) my_paned_window_1.add(my_paned_window_2) top_pane_text = tk.Text(my_paned_window_2, height=3, width=3) my_paned_window_2.add(top_pane_text) bottom_pane_text = tk.Text(my_paned_window_2, height=3, width=3) my_paned_window_2.add(bottom_pane_text) root.mainloop()
题目2:如何查看tkinter的tk版本?
参考答案:
tkinter._test()
GUI程序设计通常有哪几部分组成?
tkinter的重要控件有哪些?
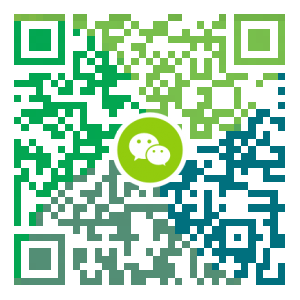
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
[雪峰磁针石博客]python包管理工具:Conda和pip比较
python测试开发项目实战-目录 python工具书籍下载-持续更新 python 3.7极速入门教程 - 目录 Conda和pip通常被认为几乎完全相同。虽然这两个工具的某些功能重叠,但它们设计用于不同的目的。 Pip是Python Packaging Authority推荐的用于从Python Package Index安装包的工具。 Pip安装打包为wheels或源代码分发的Python软件。后者可能要求系统安装兼容的编译器和库。 Conda是跨平台的包和环境管理器,可以安装和管理来自Anaconda repository以 Anaconda Cloud的conda包。 Conda包是二进制文件,徐需要使用编译器来安装它们。另外,conda包不仅限于Python软件。它们还可能包含C或C ++库,R包或任何其他软件。 这是conda和pip之间的关键区别。 Pip安装Python包,而conda安装包可能包含用任何语言编写的软件的包。在使用pip之前,必须通过系统包管理器或下载并运行安装程序来安装Python解释器。而Conda可以直接安装Python包以及Python解释器。...
- 下一篇
[雪峰磁针石博客]python绘图作业:使用pygame库画房子
python测试开发项目实战-目录 python工具书籍下载-持续更新 使用pygame库画如下房子 参考资料 本文最新版本地址 本文涉及的python测试开发库 谢谢点赞! 本文相关海量书籍下载 代码 #!/usr/bin/python3 # -*- coding: utf-8 -*- # 技术支持:https://www.jianshu.com/u/69f40328d4f0 # 技术支持 https://china-testing.github.io/ # https://github.com/china-testing/python-api-tesing/blob/master/practices/pygame_house.py # 项目实战讨论QQ群630011153 144081101 # CreateDate: 2018-12-01 import pygame pygame.init() screen = pygame.display.set_mode((640,480)) #used http://colorpicker.com/ to find RGB colors d...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Eclipse初始化配置,告别卡顿、闪退、编译时间过长
- CentOS8安装Docker,最新的服务器搭配容器使用
- Jdk安装(Linux,MacOS,Windows),包含三大操作系统的最全安装
- Red5直播服务器,属于Java语言的直播服务器
- CentOS6,CentOS7官方镜像安装Oracle11G
- Hadoop3单机部署,实现最简伪集群
- SpringBoot2整合Thymeleaf,官方推荐html解决方案
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- SpringBoot2更换Tomcat为Jetty,小型站点的福音
- SpringBoot2整合Redis,开启缓存,提高访问速度