JavaScript对象继承方式
一、对象冒充
其原理如下:构造函数使用 this 关键字给所有属性和方法赋值(即采用类声明的构造函数方式)。因为构造函数只是一个函数,所以可使 Parent 构造函数 成为 Children 的方法,然后调用它。Children 就会收到 Parent 的构造函数中定义的属性和方法。例如,用下面的方式定义 Parent 和 Children:
]// 父类构造函数 var Parent = function(name){ this.name = name; this.sayHi = function(){ console.log("Hi! " + this.name + "."); }//前端全栈学习交流圈:866109386 };//面向1-3年前端人员 //帮助突破技术瓶颈,提升思维能力 // 子类构造函数 var Children = function(name){ this.method = Parent; this.method(name); // 实现继承的关键 delete this.method; this.getName = function(){ console.log(this.name); } }; var p = new Parent("john"); var c = new Children("joe"); p.sayHi(); // 输出: Hi! john. c.sayHi(); // 输出: Hi! joe. c.getName(); // 输出: jo
原理:就是把 Parent 构造函数放到 Children 构造函数里面执行一次。那为什么不直接执行,非要转个弯把 Parent 赋值给 Children 的 method 属性再执行呢? 这跟 this 的指向有关,在函数内 this 是指向 window 的。当将 Parent 赋值给 Children 的 method 时, this 就指向了 Children 类的实例。
二、原型链继承
众所周知,JavaScript 是一门基于原型的语言,在 JavaScript 中 prototype 对象的任何属性和方法都被传递给那个类的所有实例。原型链利用这种功能来实现继承机制:
// 父类构造函数 var Parent = function(){ this.name = "john"; this.sayHi = function(){ console.log("Hi! " + this.name + "."); } }; // 子类构造函数 var Children = function(){}; Children.prototype = new Parent(); // 实现继承的关键 var p = new Parent(); var c = new Children(); p.sayHi(); // 输出: Hi! john. c.sayHi(); // 输出: Hi! john.
注意:调用 Parent 的构造函数,没有给它传递参数。这在原型链中是标准做法。要确保构造函数没有任何参数。
三、使用 call 或 applay 方法
这个方法是与对象冒充方法最相似的方法,因为它也是通过改变了 this 的指向而实现继承:
// 父类构造函数 var Parent = function(name){ this.name = name; this.sayHi = function(){ console.log("Hi! " + this.name + "."); } }; // 子类构造函数 var Children = function(name){ Parent.call(this, name); // 实现继承的关键 this.getName = function(){ console.log(this.name); } }; var p = new Parent("john"); var c = new Children("joe"); p.sayHi(); // 输出: Hi! john. c.sayHi(); // 输出: Hi! john. c.getName(); // 输出: joe
apply 方法本人就不举列了,它和 call 方法的区别在于它的第二个参数必须是数组。
四、混合方式
对象冒充的主要问题是必须使用构造函数方式,这不是最好的选择。不过如果使用原型链,就无法使用带参数的构造函数了。如何选择呢?答案很简单,两者都用。 在 JavaScript 中创建类的最好方式是用构造函数定义属性,用原型定义方法。这种方式同样适用于继承机制:
// 父类构造函数 var Parent = function(name){ this.name = name; }; Parent.prototype.sayHi = function(){ console.log("Hi! " + this.name + "."); }; // 子类构造函数 var Children = function(name, age){ Parent.call(this, name); // 实现继承的关键 this.age = age; }; Children.prototype = new Parent(); // 实现继承的关键 Children.prototype.getAge = function(){ console.log(this.age); };//前端全栈开发交流圈:866109386 //帮助1-3年前端人员突破技术瓶颈,提升思维 var p = new Parent("john"); var c = new Children("joe",30); p.sayHi(); // 输出: Hi! john. c.sayHi(); // 输出: Hi! joe. c.getAge(); // 输出: 30
五、使用Object.create 方法
Object.create 方法会使用指定的原型对象及其属性去创建一个新的对象:
// 父类构造函数 var Parent = function(name){ this.name = name; }; Parent.prototype.sayHi = function(){ console.log("Hi! " + this.name + "."); }; // 子类构造函数 var Children = function(name, age){ Parent.call(this, name); // 实现继承的关键 this.age = age; }; Children.prototype = Object.create(Parent.prototype); // 实现继承的关键 Children.prototype.constructor = children; // @ Children.prototype.getAge = function(){ console.log(this.age); }; var p = new Parent("john"); var c = new Children("joe",30); p.sayHi(); // 输出: Hi! john. c.sayHi(); // 输出: Hi! joe. c.getAge(); // 输出: 30
@ 当执行 Children.prototype = Object.create(Parent.prototype) 这个语句后,Children 的 constructor 就被改变为 Parent ,因此需要将 Children.prototype.constructor 重 新指定为 Children 自身。
六、extends 关键字实现继承
这个是 ES6 的语法糖,下面看下es6实现继承的方法:
class Parent { constructor(name, age) { this.name = name; this.age = age; } } class Children extends Parent { constructor(name, age, job) { this.job = job; // 这里会报错 super(name, age); this.job = job; // 正确 } }
上面代码中,子类的constructor方法没有调用super之前,就使用this关键字,结果报错,而放在super方法之后就是正确的。子类Children的构造函数之中的super(),代表调用父类Parent的构造函数。这是必须的,否则 JavaScript 引擎会报错。
注意,super虽然代表了父类Parent的构造函数,但是返回的是子类Children的实例,即super内部的this指的是Children,因此super()在这里相当于Parent.prototype.constructor.call(this)。
本文来自云栖社区合作伙伴“开源中国”
本文作者:peakedness
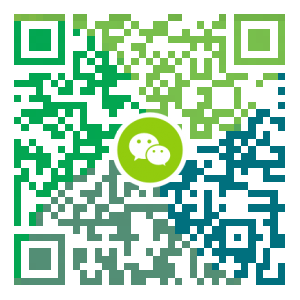
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
Godown — Go 开发的分布式 Key-Value 数据库
Godown 是一个 Go 语言开发的简单、高效分布式 Key-Value 数据库,灵感来源 Redis,基于 Raft 协议,支持 String, Bitmap, Map, List。 演示视频 使用示例 通过 Go 客户端进行连接 package main import ( "fmt" "github.com/namreg/godown/client") func main() { c, err := client.New("127.0.0.1:4000") if err != nil { panic(err) } defer c.Close() res := c.Get("key") if res.Err() != nil { panic(res.Err()) } if res.IsNil() { fmt.Print("key does not exist") } else { fmt.Println(res.Int64()) } } 本文来自云栖社区合作伙伴“开源中国” 本文作者:局长 原文链接
- 下一篇
JavaScript学习(六)
目录 函数 函数的定义 函数的调用 函数的简单调用 在事件响应中调用函数 通过超链接定义函数 函数参数的使用 使用函数的返回值 嵌套函数 递归函数 javascript中的内置函数 (1)parseInt()函数: (2)parseFloat()函数 (3)isNaN()函数 (4)isFinite()函数 (5)encodeURI()函数 (6)decodeURI()函数 Function()构造函数与函数直接量 函数 函数的定义 在javascript中,函数是由关键字function 、函数名加一组参数以及置于大括号中需要执行的一段代码定义的。基本语法如下: function functionName(【parameter 1、parameter 2...】){ statement; [return expression;] } functionName:必选项。用于指定函数名。在同一个页面中,函数名必须是唯一的,并且区分大小写。 parameter:可选性。用于指定参数列表。当使用多个参数时,参数间使用逗号进行分隔。一个函数最多可以有255个参数。 statement:必选项...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- SpringBoot2更换Tomcat为Jetty,小型站点的福音
- SpringBoot2编写第一个Controller,响应你的http请求并返回结果
- CentOS7,8上快速安装Gitea,搭建Git服务器
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- Jdk安装(Linux,MacOS,Windows),包含三大操作系统的最全安装
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- Windows10,CentOS7,CentOS8安装MongoDB4.0.16
- Eclipse初始化配置,告别卡顿、闪退、编译时间过长
- 设置Eclipse缩进为4个空格,增强代码规范