原生js——四种对话框
js一共提供了四种对话弹框,即
alert()
、confirm()
、prompt()
、showModalDialog()
。具体使用方法如下:
1.alert()
- alert()向用户显示一条消息并等待用户关闭对话框。
- 在大多数的浏览器里,alert()方法会产生阻塞,并等待用户关闭对话框。
- 也就是说,在用户关掉它们所显示的对话框之前,它们不会返回。这就意味着在弹出一个对话框前,代码就会停止运行。如果当前正在载入文档,也会停止载入,直到用户用要求的输入进行响应为止。
alert("我是一个alert对话框");
2.confirm()
- confirm()也显示一条消息,要求用户单击“确定”或“取消”按钮,并返回一个布尔值。
- 在浏览器中,confirm()会产生阻塞。
var test_value = confirm("你确定要继续吗?"); console.log(test_value); // 如果点击【确定】则返回true,点击【取消】,返回false
3.prompt()
- prompt()同样也显示一条消息,等待用户输入字符串,并返回那个字符串。
- 在浏览器中,prompt()会产生阻塞。
var message = prompt("请输入你的名字:"); console.log(message);
4.showModalDialog()
- 首先要说明的是,之前的三种对话框是通用的,showModalDialog()有一定的兼容性,可以简单概括为:IE、火狐、safari浏览器是支持的。但是Chrome和opera浏览器是不支持的,不过我们可以采用
window.open()
来代替。 - showModalDialog()显示一个包含HTML格式的“模态对话框”,可以给它传入参数,以及从对话框里返回值。
-
showModalDialog()在浏览器当前窗口中显示一个模态窗口,在显示模态窗口的时候,可以传入三个参数:
- 第一个参数用以指定提供对话框HTML内容的URL
- 第二个参数是一个任意值(数组和对象均可),这个值在对话框里的脚本中可以通过window.dialogArguments属性的值访问。
- 第三个参数是一个非标准的列表,包含以分号隔开的name=value对,如果提供了这个参数,可以配置对话框的尺寸或其他属性。具体属性如下:
|属性|说明|
|:---:|:---|
|dialogHeight
|对话框高度,不小于100px|
|dialogWidth
|对话框宽度。|
|dialogLeft
|离屏幕左的距离。|
|dialogTop
|离屏幕上的距离。|
|center
|{ yes | no | 1 | 0 }:是否居中,默认yes,但仍可以指定高度和宽度。|
|help
|{ yes | no | 1 | 0 }:是否显示帮助按钮,默认yes。|
|resizable
|{ yes | no | 1 | 0 } [IE5+]: 是否可被改变大小。默认no。|
|status
|{ yes | no | 1 | 0 } [IE5+]: 是否显示状态栏。默认为yes[ Modeless]或no[Modal]。|
|scroll
|{ yes | no | 1 | 0 | on | off }:是否显示滚动条。默认为yes。|
- 在进行模态弹窗的时候,我们首先应该新建一个弹窗的新页面,并在里面写出我们需要的html、css和js代码。例如我们新建一个modal_html.html页面:
<form action="#"> <label for="user_name">Name:</label> <input type="text" id="user_name"> <br> <label for="user_gender">Gender:</label> <input type="text" id="user_gender"> <br> <label for="user_age">Age:</label> <input type="text" id="user_age"> </form> <button id="btn_ok">ok</button> <button id="btn_cancle">cancle</button> <script> // 获取页面中的三个input元素,并赋值 var user_name = document.getElementById('user_name'); var user_age = document.getElementById('user_age'); var user_gender = document.getElementById('user_gender'); user_name.value = window.dialogArguments[0]; user_age.value = window.dialogArguments[1]; user_gender.value = window.dialogArguments[2]; var btn_ok = document.getElementById('btn_ok'); var btn_cancle = document.getElementById('btn_cancle'); // 点击【ok】按钮,关闭并返回模态窗口中的值 btn_ok.addEventListener("click",function(){ window.returnValue = [] window.returnValue[0] = user_name.value; window.returnValue[1] = user_age.value; window.returnValue[2] = user_gender.value; window.close(); }); // 点击【cancel】,直接关闭模态窗口,不返回值 btn_cancle.addEventListener("click",function(){ window.close(); }); </script>
- 新的页面创建好之后,我们便可以在另外一个页面parent.html进行模态弹窗,按照要求传入三个参数,parent.html页面的代码如下:
var p = window.showModalDialog("modal_html.html", ["Tom", 23, "meal"], "dialogWidth:400px;dialogHeight:200px;resizable:no"); // 上述代码等价于以下代码 var param_1 = "modal_html.html"; var param_2 = ["Tom", 23, "meal"]; var param_3 = "dialogWidth:400px;dialogHeight:200px;resizable:no"; var p = window.showModalDialog(param_1, param_2, param_3);
- 如果想从parent.html页面向模态窗口中传递数据,可以在模态窗口modal_html.html页面中,使用
window.dialogArguments
数组接受,示例代码如下:
var user_name = document.getElementById('user_name'); var user_age = document.getElementById('user_age'); var user_gender = document.getElementById('user_gender'); user_name.value = window.dialogArguments[0]; user_age.value = window.dialogArguments[1]; user_gender.value = window.dialogArguments[2];
- 如果想从模态窗口中向打开模态窗口的parent.html页面返回数据,首先要在parent.html页面设置
window.returnValue
,注意这里不需要使用return
语句显式返回。使用window.close();
语句关闭模态窗口的时候,会自动返回window.returnValue
。事例代码如下:
// 点击【ok】按钮,关闭并返回模态窗口中的值 btn_ok.addEventListener("click",function(){ window.returnValue = [] window.returnValue[0] = user_name.value; window.returnValue[1] = user_age.value; window.returnValue[2] = user_gender.value; window.close(); });
- 在模态窗口页面设置好
window.returnValue
,我们便可以在parent.html页面进行引用,示例代码如下:
var p = window.showModalDialog("modal_html.html", ["Tom", 23, "meal"], "dialogWidth:400px;dialogHeight:200px;resizable:no"); alert(p[0]); // 如果在模态窗口中不修改,则为Tom alert(p[1]); // 如果在模态窗口中不修改,则为 23 alert(p[2]); // 如果在模态窗口中不修改,则为 meal
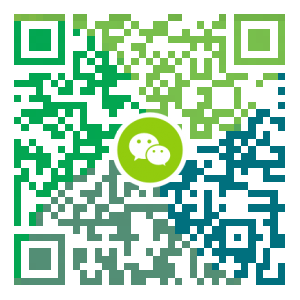
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
关于echarts在默认隐藏的div中不显示的问题解决
关于echarts在默认隐藏的div中不显示的问题解决 echarts在初始默认隐藏的div中显示空白,因为Echarts没有获取到宽高导致初始化失败,很多场景需要的在默认隐藏的div中渲染,折叠面板等等,解决办法是指定div的宽高,根据不同使用场景可以通过控制 class 显示或者隐藏实现。 <div class="chart" id="barChart" style="width:364px;height:200px;"></div> 或者 <style> .chart{ width: 364px;height: 200px;} .hide {display: none;} .show {display: block;} </style> 同样的原因很多插件初始化需要定义内联样式或内部样式、外部样式。
- 下一篇
深入理解Java内存模型(四)——volatile
volatile的特性 当我们声明共享变量为volatile后,对这个变量的读/写将会很特别。理解volatile特性的一个好方法是:把对volatile变量的单个读/写,看成是使用同一个监视器锁对这些单个读/写操作做了同步。下面我们通过具体的示例来说明,请看下面的示例代码: class VolatileFeaturesExample { volatile long vl = 0L; //使用volatile声明64位的long型变量 public void set(long l) { public void getAndIncrement () { vl = l; //单个volatile变量的写 } public long get() { vl++; //复合(多个)volatile变量的读/写 } } return vl; //单个volatile变量的读 } 假设有多个线程分别调用上面程序的三个方法,这个程序在语意上和下面程序等价: class VolatileFeaturesExample { long vl = 0L; // 64位的long型普通变量 public syn...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- MySQL8.0.19开启GTID主从同步CentOS8
- CentOS6,CentOS7官方镜像安装Oracle11G
- Mario游戏-低调大师作品
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- 设置Eclipse缩进为4个空格,增强代码规范
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- CentOS关闭SELinux安全模块
- SpringBoot2更换Tomcat为Jetty,小型站点的福音
- SpringBoot2编写第一个Controller,响应你的http请求并返回结果