Django 搭建CMDB系统完整[10](网络设备、安全设备、存储设备)
cmdb/urls.py
from cmdbapp import machinaroomviews,serverviews,networkdeviceviews,securitydeviceviews,storagedeviceviews
urlpatterns = [
url(r'^static/(?P<path>.*)',machinaroomviews.main_page,name='main_page'),
url(r'^base',machinaroomviews.base,name='base'),
url(r'^search_machinaroom.html',machinaroomviews.add_machinaroom,name='add_machinaroom'),
url(r'^edit_machinaroom.html',machinaroomviews.edit_machinaroom,name='edit_machinaroom'),
url(r'^del_machinaroom.html',machinaroomviews.del_machinaroom,name='del_machinaroom'),
url(r'^batdel_machinaroom.html',machinaroomviews.batdel_machinaroom,name='batdel_machinaroom'),
url(r'^excel_machinaroom.html',machinaroomviews.excel_machinaroom,name='excel_machinaroom'),
url(r'^login/',serverviews.search_server,name='search_server'),
url(r'^add_server.html',serverviews.edit_server,name="edit_server"),
url(r'^del_server.html',serverviews.del_server,name='del_server'),
url(r'^batdel_server.html',serverviews.batdel_server,name='batdel_server'),
url(r'^excel_server.html',serverviews.excel_server,name='excel_server'),
url(r'^search_networkdevice.html',networkdeviceviews.add_networkdevice,name="add_networkdevice"),
url(r'^edit_networkdevice.html',securitydeviceviews.search_securitydevice,name='search_securitydevice'),
url(r'^add_securitydevice.html',securitydeviceviews.edit_securitydevice,name="edit_securitydevice"),
url(r'^del_securitydevice.html',securitydeviceviews.del_securitydevice,name='del_securitydevice'),
url(r'^batdel_securitydevice.html',securitydeviceviews.batdel_securitydevice,name='batdel_securitydevice'),
url(r'^excel_securitydevice.html',securitydeviceviews.excel_securitydevice,name='excel_securitydevice'),
url(r'^search_storagedevice.html',storagedeviceviews.add_storagedevice,name="add_storagedevice"),
url(r'^edit_storagedevice.html$',storagedeviceviews.edit_storagedevice,name="edit_storagedevice"),
url(r'^del_storagedevice.html',storagedeviceviews.del_storagedevice,name='del_storagedevice'),
url(r'^batdel_storagedevice.html',storagedeviceviews.batdel_storagedevice,name='batdel_storagedevice'),
url(r'^excel_storagedevice.html',storagedeviceviews.excel_storagedevice,name='excel_storagedevice'),
]
cmdbapp/networkdeviceviews.py securitydeviceviews.py storagedeviceviews.py
-- coding: utf-8 --
from future import unicode_literals
from django.shortcuts import render,render_to_response
from django.core.paginator import Paginator,InvalidPage,EmptyPage
from cmdbapp.models import *
from django.http import HttpResponse
from django.http import HttpResponseRedirect
from xlwt import *
import StringIO
def search_networkdevice(request):
error = False
each_page = 8
mname=request.GET.get('mname','').strip()
sname=request.GET.get('sname','').strip()
ip=request.GET.get('ip','').strip()
os=request.GET.get('os','').strip()
status=int(request.GET.get('status',100))
if mname=='' and sname=='' and ip=='' and os=='' and status==100:
record_list = Networkdevice.objects.all()
paginator = Paginator(record_list,each_page)
try:
page = int(request.GET.get('page', '1'))
except ValueError:
page = 1
try:
contacts = paginator.page(page)
except (EmptyPage, InvalidPage):
contacts = paginator.page(paginator.num_pages)
return render_to_response('search_networkdevice.html',{'networkdevicelist':contacts,"mname":mname,"sname":sname,"ip":ip,"os":os,"status":status})
else:
q={}
if mname!='' and sname=='' and ip=='' and os=='' and status==100:
a=Machinaroom.objects.filter(name__icontains=mname).values("id")
q['machinaroom__in']=a
if sname!='':
q['hostname__icontains']=sname
if ip!='':
q['ip__icontains']=ip
if os!='':
q['model_icontains']=os
if status!=100:
q['status']=status
record_list = Networkdevice.objects.filter(**q)
paginator = Paginator(record_list,each_page)
try:
page = int(request.GET.get('page', '1'))
except ValueError:
page = 1
try:
contacts = paginator.page(page)
except (EmptyPage, InvalidPage):
contacts = paginator.page(paginator.num_pages)
return render_to_response('search_networkdevice.html',{'networkdevicelist':contacts,"mname":mname,"sname":sname,"ip":ip,"os":os,"status":status})
def add_networkdevice(request):
if request.method=='GET':
objs=Machinaroom.objects.all()
return render_to_response("add_networkdevice.html",{"machinaroomlist":objs})
else:
hostname=request.POST.get("networkdevicehostname","")
model=request.POST.get("networkdevicemodel","")
ip=request.POST.get("networkdeviceip","")
memo=request.POST.get("networkdevicememo","")
port_num=request.POST.get('networkdeviceport_num','')
status=request.POST.get("networkdevicestatus",0)
machinaroomid=request.POST.get("networkdevicemachinaroom")
machinaroom=Machinaroom.objects.get(id=int(machinaroomid))
uu=Networkdevice(hostname=hostname,model=model,ip=ip,memo=memo,port_num=port_num,status=status,machinaroom=machinaroom)
uu.save()
return HttpResponseRedirect("add_networkdevice.html")
def edit_networkdevice(request):
if request.method=='GET':
obj=Networkdevice.objects.get(id=int(request.GET.get('id')))
objs=Machinaroom.objects.all()
return render_to_response('edit_networkdevice.html',{'networkdevice':obj,'machinaroomlist':objs})
else:
id=request.POST.get("id")
hostname=request.POST.get("networkdevicehostname","")
model=request.POST.get("networkdevicemodel","")
ip=request.POST.get("networkdeviceip","")
memo=request.POST.get("networkdevicememo","")
status=request.POST.get("networkdevicestatus",0)
machinaroomid=request.POST.get("networkdevicemachinaroom")
machinaroom=Machinaroom.objects.get(id=int(machinaroomid))
uu=Networkdevice.objects.filter(id=id).update(hostname=hostname,model=model,ip=ip,memo=memo,status=status,machinaroom=machinaroom)
return HttpResponseRedirect("edit_networkdevice.html?id="+id)
def del_networkdevice(request):
id=request.GET.get('id')
iid=int(id)
Networkdevice.objects.filter(id=iid).delete()
mname=request.GET.get('mname','').strip()
sname=request.GET.get('sname','').strip()
ip=request.GET.get('ip','').strip()
os=request.GET.get('os','').strip()
status=request.GET.get('status',100)
page=request.GET.get('page','1')
return HttpResponseRedirect('search_networkdevice.html?mname='+mname+"&&page="+page+"&&sname="+sname+"&&ip="+ip+"&&os="+os+"&&status="+status)
def batdel_networkdevice(request):
ids=request.GET.get('ids')
b=ids.split(',')
arr = map(int,b)
for aaa in arr:
Networkdevice.objects.filter(id=aaa).delete()
mname=request.GET.get('mname')
sname=request.GET.get('sname','').strip()
ip=request.GET.get('ip','').strip()
os=request.GET.get('os','').strip()
status=request.GET.get('status',100)
return HttpResponseRedirect('search_networkdevice.html?mname='+mname+"&&sname="+sname+"&&ip="+ip+"&&os="+os+"&&status="+status)
def excel_networkdevice(request):
mname=request.GET.get('mname','').strip()
sname=request.GET.get('sname','').strip()
ip=request.GET.get('ip','').strip()
os=request.GET.get('os','').strip()
status=int(request.GET.get('status',100))
if mname=='' and sname=='' and ip=='' and os=='' and status==100:
list_obj = Networkdevice.objects.all()
else:
q={}
if mname!='' and sname=='' and ip=='' and os=='' and status==100:
a=Machinaroom.objects.filter(name__icontains=mname).values("id")
q['machinaroom__in']=a
if sname!='':
q['hostname__icontains']=sname
if ip!='':
q['ip__icontains']=ip
if os!='':
q['model__icontains']=os
if status!=100:
q['status']=status
list_obj = Networkdevice.objects.filter(**q)
if list_obj:
# 创建工作薄
ws = Workbook(encoding='utf-8')
w = ws.add_sheet(u"网络设备清单")
w.write(0, 0, "id")
w.write(0, 1, u"设备名字")
w.write(0, 2, u"制造商/型号")
w.write(0, 3, u"IP")
w.write(0, 4, u"端口数量")
w.write(0,5,u"备注")
w.write(0,6,u"状态")
w.write(0,7,u"机房")
# 写入数据
excel_row = 1
for obj in list_obj:
data_id = obj.id
data_hostname = obj.hostname
data_model = obj.model
data_ip = obj.ip
data_port_num = obj.port_num
data_memo=obj.memo
if obj.status == 0:
data_status='在线'
elif obj.status == 1:
data_status='已下线'
elif obj.status == 2:
data_status='未知'
elif obj.status == 3:
data_status='故障'
else:
data_status='备用'
data_machinaroom=obj.machinaroom.name
w.write(excel_row, 0, data_id)
w.write(excel_row, 1, data_hostname)
w.write(excel_row, 2, data_model)
w.write(excel_row, 3, data_ip)
w.write(excel_row, 4, data_port_num)
w.write(excel_row, 5, data_memo)
w.write(excel_row, 6, data_status)
w.write(excel_row, 7, data_machinaroom)
excel_row += 1
sio = StringIO.StringIO()
ws.save(sio)
sio.seek(0)
response = HttpResponse(sio.getvalue(), content_type='application/vnd.ms-excel')
response['Content-Disposition'] = 'attachment; filename=服务器清单-'+mname+sname+ip+os+'.xls'
response.write(sio.getvalue())
return response
-- coding: utf-8 --
from future import unicode_literals
from django.shortcuts import render,render_to_response
from django.core.paginator import Paginator,InvalidPage,EmptyPage
from cmdbapp.models import *
from django.http import HttpResponse
from django.http import HttpResponseRedirect
from xlwt import *
import StringIO
def search_securitydevice(request):
error = False
each_page = 8
mname=request.GET.get('mname','').strip()
sname=request.GET.get('sname','').strip()
ip=request.GET.get('ip','').strip()
model=request.GET.get('model','').strip()
type=request.GET.get('type','').strip()
status=int(request.GET.get('status',100))
if mname=='' and sname=='' and ip=='' and model=='' and type=='' and status==100:
record_list = Securitydevice.objects.all()
paginator = Paginator(record_list,each_page)
try:
page = int(request.GET.get('page', '1'))
except ValueError:
page = 1
try:
contacts = paginator.page(page)
except (EmptyPage, InvalidPage):
contacts = paginator.page(paginator.num_pages)
return render_to_response('search_securitydevice.html',{'securitydevicelist':contacts,"mname":mname,"sname":sname,"ip":ip,"model":model,"type":type,"status":status})
else:
q={}
if mname!='' and sname=='' and ip=='' and model=='' and type=='' and status==100:
a=Machinaroom.objects.filter(name__icontains=mname).values("id")
q['machinaroom__in']=a
if sname!='':
q['hostname__icontains']=sname
if ip!='':
q['ip__icontains']=ip
if model!='':
q['model_icontains']=model
if type!='':
q['type_icontains']=type
if status!=100:
q['status']=status
record_list = Securitydevice.objects.filter(**q)
paginator = Paginator(record_list,each_page)
try:
page = int(request.GET.get('page', '1'))
except ValueError:
page = 1
try:
contacts = paginator.page(page)
except (EmptyPage, InvalidPage):
contacts = paginator.page(paginator.num_pages)
return render_to_response('search_securitydevice.html',{'securitydevicelist':contacts,"mname":mname,"sname":sname,"ip":ip,"model":model,"type":type,"status":status})
def add_securitydevice(request):
if request.method=='GET':
objs=Machinaroom.objects.all()
return render_to_response("add_securitydevice.html",{"machinaroomlist":objs})
else:
hostname=request.POST.get("securitydevicehostname","")
model=request.POST.get("securitydevicemodel","")
ip=request.POST.get("securitydeviceip","")
memo=request.POST.get("securitydevicememo","")
port_num=request.POST.get('securitydeviceport_num',0)
status=request.POST.get("securitydevicestatus",0)
machinaroomid=request.POST.get("securitydevicemachinaroom")
machinaroom=Machinaroom.objects.get(id=int(machinaroomid))
type=request.POST.get("securitydevicetype","")
uu=Securitydevice(hostname=hostname,model=model,ip=ip,type=type,memo=memo,port_num=port_num,status=status,machinaroom=machinaroom)
uu.save()
return HttpResponseRedirect("add_securitydevice.html")
def edit_securitydevice(request):
if request.method=='GET':
obj=Securitydevice.objects.get(id=int(request.GET.get('id')))
objs=Machinaroom.objects.all()
return render_to_response('edit_securitydevice.html',{'securitydevice':obj,'machinaroomlist':objs})
else:
id=request.POST.get("id")
hostname=request.POST.get("securitydevicehostname","")
model=request.POST.get("securitydevicemodel","")
ip=request.POST.get("securitydeviceip","")
memo=request.POST.get("securitydevicememo","")
status=request.POST.get("securitydevicestatus",0)
port_num=request.POST.get("securitydeviceport_num",0)
machinaroomid=request.POST.get("securitydevicemachinaroom")
machinaroom=Machinaroom.objects.get(id=int(machinaroomid))
type=request.POST.get("securitydevicetype","")
uu=Securitydevice.objects.filter(id=id).update(hostname=hostname,model=model,type=type,ip=ip,port_num=port_num,memo=memo,status=status,machinaroom=machinaroom)
return HttpResponseRedirect("edit_securitydevice.html?id="+id)
def del_securitydevice(request):
id=request.GET.get('id')
iid=int(id)
Securitydevice.objects.filter(id=iid).delete()
mname=request.GET.get('mname','').strip()
sname=request.GET.get('sname','').strip()
ip=request.GET.get('ip','').strip()
model=request.GET.get('model','').strip()
type=request.GET.get('type','').strip()
status=request.GET.get('status',100)
page=request.GET.get('page','1')
return HttpResponseRedirect('search_securitydevice.html?mname='+mname+"&&page="+page+"&&sname="+sname+"&&ip="+ip+"&&model="+model+"&&type="+type+"&&status="+status)
def batdel_securitydevice(request):
ids=request.GET.get('ids')
b=ids.split(',')
arr = map(int,b)
for aaa in arr:
Securitydevice.objects.filter(id=aaa).delete()
mname=request.GET.get('mname')
sname=request.GET.get('sname','').strip()
ip=request.GET.get('ip','').strip()
model=request.GET.get('model','').strip()
type=request.GET.get('type','').strip()
status=request.GET.get('status',100)
return HttpResponseRedirect('search_securitydevice.html?mname='+mname+"&&sname="+sname+"&&ip="+ip+"&&model="+model+"&&type="+type+"&&status="+status)
def excel_securitydevice(request):
mname=request.GET.get('mname','').strip()
sname=request.GET.get('sname','').strip()
ip=request.GET.get('ip','').strip()
model=request.GET.get('model','').strip()
type=request.GET.get('type','').strip()
status=int(request.GET.get('status',100))
if mname=='' and sname=='' and ip=='' and model=='' and type=='' and status==100:
list_obj = Securitydevice.objects.all()
else:
q={}
if mname!='' and sname=='' and ip=='' and model=='' and type=='' and status==100:
a=Machinaroom.objects.filter(name__icontains=mname).values("id")
q['machinaroom__in']=a
if sname!='':
q['hostname__icontains']=sname
if ip!='':
q['ip__icontains']=ip
if model!='':
q['model__icontains']=model
if type!='':
q['type_icontains']=type
if status!=100:
q['status']=status
list_obj = Securitydevice.objects.filter(**q)
if list_obj:
# 创建工作薄
ws = Workbook(encoding='utf-8')
w = ws.add_sheet(u"网络设备清单")
w.write(0, 0, "id")
w.write(0, 1, u"设备名字")
w.write(0, 2, u"制造商/型号")
w.write(0, 3, u"IP")
w.write(0, 4, u"端口数量")
w.write(0,5,u"类型")
w.write(0,6,u"备注")
w.write(0,7,u"状态")
w.write(0,8,u"机房")
# 写入数据
excel_row = 1
for obj in list_obj:
data_id = obj.id
data_hostname = obj.hostname
data_model = obj.model
data_ip = obj.ip
data_port_num = obj.port_num
data_type=obj.type
data_memo=obj.memo
if obj.status == 0:
data_status='在线'
elif obj.status == 1:
data_status='已下线'
elif obj.status == 2:
data_status='未知'
elif obj.status == 3:
data_status='故障'
else:
data_status='备用'
data_machinaroom=obj.machinaroom.name
w.write(excel_row, 0, data_id)
w.write(excel_row, 1, data_hostname)
w.write(excel_row, 2, data_model)
w.write(excel_row, 3, data_ip)
w.write(excel_row, 4, data_port_num)
w.write(excel_row, 5, data_type)
w.write(excel_row, 6, data_memo)
w.write(excel_row, 7, data_status)
w.write(excel_row, 8, data_machinaroom)
excel_row += 1
sio = StringIO.StringIO()
ws.save(sio)
sio.seek(0)
response = HttpResponse(sio.getvalue(), content_type='application/vnd.ms-excel')
response['Content-Disposition'] = 'attachment; filename=服务器清单-'+mname+sname+ip+'.xls'
response.write(sio.getvalue())
return response
-- coding: utf-8 --
from future import unicode_literals
from django.shortcuts import render,render_to_response
from django.core.paginator import Paginator,InvalidPage,EmptyPage
from cmdbapp.models import *
from django.http import HttpResponse
from django.http import HttpResponseRedirect
from xlwt import *
import StringIO
def search_storagedevice(request):
error = False
each_page = 8
mname=request.GET.get('mname','').strip()
sname=request.GET.get('sname','').strip()
ip=request.GET.get('ip','').strip()
model=request.GET.get('model','').strip()
status=int(request.GET.get('status',100))
if mname=='' and sname=='' and ip=='' and model=='' and status==100:
record_list = Storagedevice.objects.all()
paginator = Paginator(record_list,each_page)
try:
page = int(request.GET.get('page', '1'))
except ValueError:
page = 1
try:
contacts = paginator.page(page)
except (EmptyPage, InvalidPage):
contacts = paginator.page(paginator.num_pages)
return render_to_response('search_storagedevice.html',{'storagedevicelist':contacts,"mname":mname,"sname":sname,"ip":ip,"model":model,"status":status})
else:
q={}
if mname!='' and sname=='' and ip=='' and model=='' and type=='' and status==100:
a=Machinaroom.objects.filter(name__icontains=mname).values("id")
q['machinaroom__in']=a
if sname!='':
q['hostname__icontains']=sname
if ip!='':
q['ip__icontains']=ip
if model!='':
q['model_icontains']=mode
if status!=100:
q['status']=status
record_list = Storagedevice.objects.filter(**q)
paginator = Paginator(record_list,each_page)
try:
page = int(request.GET.get('page', '1'))
except ValueError:
page = 1
try:
contacts = paginator.page(page)
except (EmptyPage, InvalidPage):
contacts = paginator.page(paginator.num_pages)
return render_to_response('search_storagedevice.html',{'storagedevicelist':contacts,"mname":mname,"sname":sname,"ip":ip,"model":model,"status":status})
def add_storagedevice(request):
if request.method=='GET':
objs=Machinaroom.objects.all()
return render_to_response("add_storagedevice.html",{"machinaroomlist":objs})
else:
hostname=request.POST.get("storagedevicehostname","")
model=request.POST.get("storagedevicemodel","")
ip=request.POST.get("storagedeviceip","")
memo=request.POST.get("storagedevicememo","")
disk=request.POST.get('storagedevicedisk','')
status=request.POST.get("storagedevicestatus",0)
machinaroomid=request.POST.get("storagedevicemachinaroom")
machinaroom=Machinaroom.objects.get(id=int(machinaroomid))
uu=Storagedevice(hostname=hostname,model=model,ip=ip,memo=memo,disk=disk,status=status,machinaroom=machinaroom)
uu.save()
return HttpResponseRedirect("add_storagedevice.html")
def edit_storagedevice(request):
if request.method=='GET':
obj=Storagedevice.objects.get(id=int(request.GET.get('id')))
objs=Machinaroom.objects.all()
return render_to_response('edit_storagedevice.html',{'storagedevice':obj,'machinaroomlist':objs})
else:
id=request.POST.get("id")
hostname=request.POST.get("storagedevicehostname","")
model=request.POST.get("storagedevicemodel","")
ip=request.POST.get("storagedeviceip","")
memo=request.POST.get("storagedevicememo","")
status=request.POST.get("storagedevicestatus",0)
machinaroomid=request.POST.get("storagedevicemachinaroom")
machinaroom=Machinaroom.objects.get(id=int(machinaroomid))
disk=request.POST.get("storagedevicedisk","")
uu=Storagedevice.objects.filter(id=id).update(hostname=hostname,model=model,disk=disk,ip=ip,memo=memo,status=status,machinaroom=machinaroom)
return HttpResponseRedirect("edit_storagedevice.html?id="+id)
def del_storagedevice(request):
id=request.GET.get('id')
iid=int(id)
Storagedevice.objects.filter(id=iid).delete()
mname=request.GET.get('mname','').strip()
sname=request.GET.get('sname','').strip()
ip=request.GET.get('ip','').strip()
model=request.GET.get('model','').strip()
status=request.GET.get('status',100)
page=request.GET.get('page','1')
return HttpResponseRedirect('search_storagedevice.html?mname='+mname+"&&page="+page+"&&sname="+sname+"&&ip="+ip+"&&model="+model+"&&status="+status)
def batdel_storagedevice(request):
ids=request.GET.get('ids')
b=ids.split(',')
arr = map(int,b)
for aaa in arr:
Storagedevice.objects.filter(id=aaa).delete()
mname=request.GET.get('mname')
sname=request.GET.get('sname','').strip()
ip=request.GET.get('ip','').strip()
model=request.GET.get('model','').strip()
status=request.GET.get('status',100)
return HttpResponseRedirect('search_storagedevice.html?mname='+mname+"&&sname="+sname+"&&ip="+ip+"&&model="+model+"&&status="+status)
def excel_storagedevice(request):
mname=request.GET.get('mname','').strip()
sname=request.GET.get('sname','').strip()
ip=request.GET.get('ip','').strip()
model=request.GET.get('model','').strip()
status=int(request.GET.get('status',100))
if mname=='' and sname=='' and ip=='' and model=='' and status==100:
list_obj = Storagedevice.objects.all()
else:
q={}
if mname!='' and sname=='' and ip=='' and model=='' and status==100:
a=Machinaroom.objects.filter(name__icontains=mname).values("id")
q['machinaroom__in']=a
if sname!='':
q['hostname__icontains']=sname
if ip!='':
q['ip__icontains']=ip
if model!='':
q['model__icontains']=model
if status!=100:
q['status']=status
list_obj = Storagedevice.objects.filter(**q)
if list_obj:
# 创建工作薄
ws = Workbook(encoding='utf-8')
w = ws.add_sheet(u"网络设备清单")
w.write(0, 0, "id")
w.write(0, 1, u"设备名字")
w.write(0, 2, u"制造商/型号")
w.write(0, 3, u"IP")
w.write(0, 4, u"容量信息")
w.write(0,5,u"备注")
w.write(0,6,u"状态")
w.write(0,7,u"机房")
# 写入数据
excel_row = 1
for obj in list_obj:
data_id = obj.id
data_hostname = obj.hostname
data_model = obj.model
data_ip = obj.ip
data_disk = obj.disk
data_memo=obj.memo
if obj.status == 0:
data_status='在线'
elif obj.status == 1:
data_status='已下线'
elif obj.status == 2:
data_status='未知'
elif obj.status == 3:
data_status='故障'
else:
data_status='备用'
data_machinaroom=obj.machinaroom.name
w.write(excel_row, 0, data_id)
w.write(excel_row, 1, data_hostname)
w.write(excel_row, 2, data_model)
w.write(excel_row, 3, data_ip)
w.write(excel_row, 4, data_disk)
w.write(excel_row, 5, data_memo)
w.write(excel_row, 6, data_status)
w.write(excel_row, 7, data_machinaroom)
excel_row += 1
sio = StringIO.StringIO()
ws.save(sio)
sio.seek(0)
response = HttpResponse(sio.getvalue(), content_type='application/vnd.ms-excel')
response['Content-Disposition'] = 'attachment; filename=服务器清单-'+mname+sname+ip+'.xls'
response.write(sio.getvalue())
return response
修改 models.py
-- coding: utf-8 --
from future import unicode_literals
from django.db import models
class Machinaroom(models.Model):
id=models.AutoField(primary_key=True)
name=models.CharField(max_length=200,default=u'机房')
location=models.CharField(max_length=200,default=u'厦门')
vpnurl=models.URLField(default="")
memo = models.CharField(u'备注', max_length=200,default="")
def unicode(self):
return self.name
class Server(models.Model):
"""
服务器信息
"""
machinaroom= models.ForeignKey(Machinaroom)
hostname = models.CharField(max_length=128, unique=True) model = models.CharField('制造商/型号', max_length=128, default="") ip = models.CharField('IP', max_length=128, default="") os = models.CharField('操作系统', max_length=128, default="") cpu = models.CharField('CPU', max_length=128, default="") memory=models.CharField('memory', max_length=128, default="") disk=models.CharField('硬盘信息',max_length=200, default="") status_choices = ((0, '在线'), (1, '已下线'), (2, '未知'), (3, '故障'), (4, '备用'), ) status = models.SmallIntegerField(choices=status_choices, default=0) memo = models.CharField(u'备注', max_length=200,default="") id =models.AutoField(primary_key=True) class Meta: verbose_name_plural = "服务器表" def __str__(self): return self.hostname
class Networkdevice(models.Model):
status_choices = ((0, '在线'),
(1, '已下线'),
(2, '未知'),
(3, '故障'),
(4, '备用'),
)
status = models.SmallIntegerField(choices=status_choices, default=0)
id =models.AutoField(primary_key=True) machinaroom= models.ForeignKey(Machinaroom) hostname = models.CharField(max_length=128, unique=True) port_num = models.SmallIntegerField(u'端口个数', default=0) model = models.CharField(u'型号', max_length=128, default="") ip = models.CharField(max_length=128, default="") memo = models.CharField(u'备注', max_length=200,default="") class Meta: verbose_name = '网络设备' verbose_name_plural = "网络设备" def __str__(self): return self.hostname
class Securitydevice(models.Model):
status_choices = ((0, '在线'),
(1, '已下线'),
(2, '未知'),
(3, '故障'),
(4, '备用'),
)
status = models.SmallIntegerField(choices=status_choices, default=0)
id =models.AutoField(primary_key=True) machinaroom= models.ForeignKey(Machinaroom) type=models.CharField(u'类型', max_length=128, default="") hostname = models.CharField(max_length=128, unique=True) port_num = models.SmallIntegerField(u'端口个数', default=0) model = models.CharField(u'型号', max_length=128, default="") memo = models.CharField(u'备注', max_length=200,default="") ip = models.CharField(max_length=128, default="") class Meta: verbose_name = '安全设备' verbose_name_plural = "安全设备" def __str__(self): return self.hostname
class Storagedevice(models.Model):
"""存储设备"""
machinaroom= models.ForeignKey(Machinaroom)
model = models.CharField(u'型号', max_length=128, default="")
id =models.AutoField(primary_key=True)
hostname = models.CharField(max_length=128, unique=True,default="")
ip = models.CharField(max_length=128, default="")
status_choices = ((0, '在线'),
(1, '已下线'),
(2, '未知'),
(3, '故障'),
(4, '备用'),
)
status = models.SmallIntegerField(choices=status_choices, default=0)
disk=models.CharField('容量信息',max_length=200, default="")
memo = models.TextField(u'备注', default="")
class Meta:
verbose_name = '存储设备'
verbose_name_plural = "存储设备"
def __str__(self): return self.model
class Software(models.Model):
sub_assset_type_choices = (
(0, 'OS'),
(1, '办公\开发软件'),
(2, '业务软件'),
) sub_asset_type = models.SmallIntegerField(choices=sub_assset_type_choices, verbose_name="服务器类型", default=0) license_num = models.IntegerField(verbose_name="授权数",default=0) version = models.CharField(u'软件/系统版本', max_length=64, help_text=u'eg. CentOS release 6.5 (Final)', unique=True) id =models.AutoField(primary_key=True) def __str__(self): return self.version class Meta: verbose_name = '软件/系统' verbose_name_plural = "软件/系统"
class Manufactory(models.Model):
"""厂商"""
id =models.AutoField(primary_key=True) manufactory = models.CharField(u'厂商名称', max_length=64, unique=True) support_num = models.CharField(u'支持电话', max_length=30, default="") memo = models.CharField(u'备注', max_length=128, default="") def __str__(self): return self.manufactory class Meta: verbose_name = '厂商' verbose_name_plural = "厂商"
templates/add_networkdevice.html add_securitydevice.html add_storagedevice.html edit_networkdevice.html edit_securitydevice.html edit_storagedevice.html search_networkdevice.html search_securitydevice.html search_storagedevice.html
<!DOCTYPE html>
<html>
<head>
<title>CMDB</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.7.1.js"></script>
<link href="/static/style/authority/basic_layout.css" rel="stylesheet" type="text/css">
<link href="/static/style/authority/common_style.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="/static/scripts/authority/commonAll.js"></script>
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.4.4.min.js"></script>
<script src="/static/scripts/My97DatePicker/WdatePicker.js" type="text/javascript" defer="defer"></script>
<script type="text/javascript" src="/static/scripts/artDialog/artDialog.js?skin=default"></script>
</head>
<body>
<form id="addnetworkdeviceform" name="addnetworkdeviceform" action="add_networkdevice.html" method="post">
<div id="container">
<div id="nav_links">
当前位置:服务器资产管理 > <span style="color: #1A5CC6;">新增网络设备</span>
<div id="page_close">
<a href="javascript:parent.$.fancybox.close();">
<img src="/static/images/common/page_close.png" width="20" height="20" style="vertical-align: text-top;"/>
</a>
</div>
</div>
<div class="ui_content">
<table cellspacing="0" cellpadding="0" width="100%" align="left" border="0">
<tr>
<td class="ui_text_rt">设备名字</td>
<td class="ui_text_lt">
<input type="text" id="networkdevicehostname" name="networkdevicehostname" value="" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">制造商/型号</td>
<td class="ui_text_lt">
<input type="text" id="networkdevicemodel" name="networkdevicemodel" value="" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">IP</td>
<td class="ui_text_lt">
<input type="text" id="networkdeviceip" name="networkdeviceip" value="" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">端口数量</td>
<td class="ui_text_lt">
<input type="text" id="networkdeviceport_num" name="networkdeviceport_num" value="" class="ui_input_txt02" />
</td>
</tr>
<tr> <td class="ui_text_rt">备注</td> <td class="ui_text_lt"> <input type="text" id="networkdevicememo" name="networkdevicememo" value="" class="ui_input_txt02" /> </td> </tr> <tr>
</tr>
<tr>
<td class="ui_text_rt">状态</td>
<td class="ui_text_lt">
<select name="networkdevicestatus" id="networkdevicestatus" class="ui_select01" >
<option value=0>在线</option>
<option value=1>已下线</option>
<option value=2>未知</option>
<option value=3>故障</option>
<option value=4>备用</option>
</select>
</td>
</tr>
<tr>
<td class="ui_text_rt">所属机房</td>
<td class="ui_text_lt">
<select name="networkdevicemachinaroom" id="networkdevicemachinaroom" class="ui_select01">
{% for mr in machinaroomlist %}
<option value={{ mr.id }}>{{ mr.name }}</option>
{% endfor %}
</select>
</td>
</tr>
<tr>
<td> </td>
<td class="ui_text_lt">
<input id="submitbutton" type="submit" value="提交" class="ui_input_btn01"/>
<input id="cancelbutton" type="cancel" value="取消" class="ui_input_btn01"/>
</td>
</tr>
</table>
</div>
</div>
</form>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>CMDB</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.7.1.js"></script>
<link href="/static/style/authority/basic_layout.css" rel="stylesheet" type="text/css">
<link href="/static/style/authority/common_style.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="/static/scripts/authority/commonAll.js"></script>
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.4.4.min.js"></script>
<script src="/static/scripts/My97DatePicker/WdatePicker.js" type="text/javascript" defer="defer"></script>
<script type="text/javascript" src="/static/scripts/artDialog/artDialog.js?skin=default"></script>
</head>
<body>
<form id="addsecuritydeviceform" name="addsecuritydeviceform" action="add_securitydevice.html" method="post">
<div id="container">
<div id="nav_links">
当前位置:服务器资产管理 > <span style="color: #1A5CC6;">新增安全设备</span>
<div id="page_close">
<a href="javascript:parent.$.fancybox.close();">
<img src="/static/images/common/page_close.png" width="20" height="20" style="vertical-align: text-top;"/>
</a>
</div>
</div>
<div class="ui_content">
<table cellspacing="0" cellpadding="0" width="100%" align="left" border="0">
<tr>
<td class="ui_text_rt">设备名字</td>
<td class="ui_text_lt">
<input type="text" id="securitydevicehostname" name="securitydevicehostname" value="" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">制造商/型号</td>
<td class="ui_text_lt">
<input type="text" id="securitydevicemodel" name="securitydevicemodel" value="" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">IP</td>
<td class="ui_text_lt">
<input type="text" id="securitydeviceip" name="securitydeviceip" value="" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">端口数量</td>
<td class="ui_text_lt">
<input type="text" id="securitydeviceport_num" name="securitydeviceport_num" value="" class="ui_input_txt02" />
</td>
</tr>
<tr> <td class="ui_text_rt">类型</td> <td class="ui_text_lt"> <input type="text" id="securitydevicetype" name="securitydevicetype" value="" class="ui_input_txt02" /> </td> </tr> <tr> <td class="ui_text_rt">备注</td> <td class="ui_text_lt"> <input type="text" id="securitydevicememo" name="securitydevicememo" value="" class="ui_input_txt02" /> </td> </tr> <tr>
</tr>
<tr>
<td class="ui_text_rt">状态</td>
<td class="ui_text_lt">
<select name="securitydevicestatus" id="securitydevicestatus" class="ui_select01" >
<option value=0>在线</option>
<option value=1>已下线</option>
<option value=2>未知</option>
<option value=3>故障</option>
<option value=4>备用</option>
</select>
</td>
</tr>
<tr>
<td class="ui_text_rt">所属机房</td>
<td class="ui_text_lt">
<select name="securitydevicemachinaroom" id="securitydevicemachinaroom" class="ui_select01">
{% for mr in machinaroomlist %}
<option value={{ mr.id }}>{{ mr.name }}</option>
{% endfor %}
</select>
</td>
</tr>
<tr>
<td> </td>
<td class="ui_text_lt">
<input id="submitbutton" type="submit" value="提交" class="ui_input_btn01"/>
<input id="cancelbutton" type="cancel" value="取消" class="ui_input_btn01"/>
</td>
</tr>
</table>
</div>
</div>
</form>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>CMDB</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.7.1.js"></script>
<link href="/static/style/authority/basic_layout.css" rel="stylesheet" type="text/css">
<link href="/static/style/authority/common_style.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="/static/scripts/authority/commonAll.js"></script>
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.4.4.min.js"></script>
<script src="/static/scripts/My97DatePicker/WdatePicker.js" type="text/javascript" defer="defer"></script>
<script type="text/javascript" src="/static/scripts/artDialog/artDialog.js?skin=default"></script>
</head>
<body>
<form id="addstoragedeviceform" name="addstoragedeviceform" action="add_storagedevice.html" method="post">
<div id="container">
<div id="nav_links">
当前位置:服务器资产管理 > <span style="color: #1A5CC6;">新增存储设备</span>
<div id="page_close">
<a href="javascript:parent.$.fancybox.close();">
<img src="/static/images/common/page_close.png" width="20" height="20" style="vertical-align: text-top;"/>
</a>
</div>
</div>
<div class="ui_content">
<table cellspacing="0" cellpadding="0" width="100%" align="left" border="0">
<tr>
<td class="ui_text_rt">设备名字</td>
<td class="ui_text_lt">
<input type="text" id="storagedevicehostname" name="storagedevicehostname" value="" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">制造商/型号</td>
<td class="ui_text_lt">
<input type="text" id="storagedevicemodel" name="storagedevicemodel" value="" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">IP</td>
<td class="ui_text_lt">
<input type="text" id="storagedeviceip" name="storagedeviceip" value="" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">容量信息</td>
<td class="ui_text_lt">
<input type="text" id="storagedevicedisk" name="storagedevicedisk" value="" class="ui_input_txt02" />
</td>
</tr>
<tr> <td class="ui_text_rt">备注</td> <td class="ui_text_lt"> <input type="text" id="storagedevicememo" name="storagedevicememo" value="" class="ui_input_txt02" /> </td> </tr> <tr>
</tr>
<tr>
<td class="ui_text_rt">状态</td>
<td class="ui_text_lt">
<select name="storagedevicestatus" id="storagedevicestatus" class="ui_select01" >
<option value=0>在线</option>
<option value=1>已下线</option>
<option value=2>未知</option>
<option value=3>故障</option>
<option value=4>备用</option>
</select>
</td>
</tr>
<tr>
<td class="ui_text_rt">所属机房</td>
<td class="ui_text_lt">
<select name="storagedevicemachinaroom" id="storagedevicemachinaroom" class="ui_select01">
{% for mr in machinaroomlist %}
<option value={{ mr.id }}>{{ mr.name }}</option>
{% endfor %}
</select>
</td>
</tr>
<tr>
<td> </td>
<td class="ui_text_lt">
<input id="submitbutton" type="submit" value="提交" class="ui_input_btn01"/>
<input id="cancelbutton" type="cancel" value="取消" class="ui_input_btn01"/>
</td>
</tr>
</table>
</div>
</div>
</form>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>CMDB</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.7.1.js"></script>
<link href="/static/style/authority/basic_layout.css" rel="stylesheet" type="text/css">
<link href="/static/style/authority/common_style.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="/static/scripts/authority/commonAll.js"></script>
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.4.4.min.js"></script>
<script src="/static/scripts/My97DatePicker/WdatePicker.js" type="text/javascript" defer="defer"></script>
<script type="text/javascript" src="/static/scripts/artDialog/artDialog.js?skin=default"></script>
</head>
<body>
<form id="editnetworkdeviceform" name="editnetworkdeviceform" action="edit_networkdevice.html" method="post">
<div id="container">
<div id="nav_links">
当前位置:服务器资产管理 > <span style="color: #1A5CC6;">编辑网络设备</span>
<div id="page_close">
<a href="javascript:parent.$.fancybox.close();">
<img src="/static/images/common/page_close.png" width="20" height="20" style="vertical-align: text-top;"/>
</a>
</div>
</div>
<div class="ui_content"><input style="visibility:hidden" value={{ networkdevice.id }} type="text" name="id" id="id" />
<table cellspacing="0" cellpadding="0" width="100%" align="left" border="0">
<tr>
<td class="ui_text_rt">设备名字</td>
<td class="ui_text_lt">
<input type="text" id="networkdevicehostname" name="networkdevicehostname" value="{{ networkdevice.hostname }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">制造商/型号</td>
<td class="ui_text_lt">
<input type="text" id="networkdevicemodel" name="networkdevicemodel" value="{{ networkdevice.model }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">IP</td>
<td class="ui_text_lt">
<input type="text" id="networkdeviceip" name="networkdeviceip" value="{{ networkdevice.ip }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">端口数量</td>
<td class="ui_text_lt">
<input type="text" id="networkdeviceport_num" name="networkdeviceport_num" value="{{ networkdevice.port_num }}" class="ui_input_txt02" />
</td>
</tr>
<tr> <td class="ui_text_rt">备注</td> <td class="ui_text_lt"> <input type="text" id="networkdevicememo" name="networkdevicememo" value="{{ networkdevice.memo }}" class="ui_input_txt02" /> </td> </tr> <tr>
</tr>
<tr> <td class="ui_text_rt">状态</td> <td class="ui_text_lt"> <select name="networkdevicestatus" id="networkdevicestatus" class="ui_select01" > <option value=0>在线</option> <option value=1>已下线</option> <option value=2>未知</option> <option value=3>故障</option> <option value=4>备用</option> </select> </td> </tr> <tr> <td class="ui_text_rt">所属机房</td> <td class="ui_text_lt"> <select name="networkdevicemachinaroom" id="networkdevicemachinaroom" class="ui_select01"> {% for mr in machinaroomlist %} <option value={{ mr.id }}>{{ mr.name }}</option> {% endfor %} </select> </td> </tr> <tr> <td> </td> <td class="ui_text_lt"> <input id="submitbutton" type="submit" value="提交" class="ui_input_btn01"/> <input id="cancelbutton" type="cancel" value="取消" class="ui_input_btn01"/> </td> </tr> </table> </div> </div>
</form>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>CMDB</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.7.1.js"></script>
<link href="/static/style/authority/basic_layout.css" rel="stylesheet" type="text/css">
<link href="/static/style/authority/common_style.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="/static/scripts/authority/commonAll.js"></script>
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.4.4.min.js"></script>
<script src="/static/scripts/My97DatePicker/WdatePicker.js" type="text/javascript" defer="defer"></script>
<script type="text/javascript" src="/static/scripts/artDialog/artDialog.js?skin=default"></script>
</head>
<body>
<form id="editsecuritydeviceform" name="editsecuritydeviceform" action="edit_securitydevice.html" method="post">
<div id="container">
<div id="nav_links">
当前位置:服务器资产管理 > <span style="color: #1A5CC6;">编辑安全设备</span>
<div id="page_close">
<a href="javascript:parent.$.fancybox.close();">
<img src="/static/images/common/page_close.png" width="20" height="20" style="vertical-align: text-top;"/>
</a>
</div>
</div>
<div class="ui_content"><input style="visibility:hidden" value={{ securitydevice.id }} type="text" name="id" id="id" />
<table cellspacing="0" cellpadding="0" width="100%" align="left" border="0">
<tr>
<td class="ui_text_rt">设备名字</td>
<td class="ui_text_lt">
<input type="text" id="securitydevicehostname" name="securitydevicehostname" value="{{ securitydevice.hostname }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">制造商/型号</td>
<td class="ui_text_lt">
<input type="text" id="securitydevicemodel" name="securitydevicemodel" value="{{ securitydevice.model }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">IP</td>
<td class="ui_text_lt">
<input type="text" id="securitydeviceip" name="securitydeviceip" value="{{ securitydevice.ip }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">端口数量</td>
<td class="ui_text_lt">
<input type="text" id="securitydeviceport_num" name="securitydeviceport_num" value="{{ securitydevice.port_num }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">类型</td>
<td class="ui_text_lt">
<input type="text" id="securitydevicetype" name="securitydevicetype" value="{{ securitydevice.type }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">备注</td>
<td class="ui_text_lt">
<input type="text" id="securitydevicememo" name="securitydevicememo" value="{{ securitydevice.memo }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
</tr>
<tr> <td class="ui_text_rt">状态</td> <td class="ui_text_lt"> <select name="securitydevicestatus" id="securitydevicestatus" class="ui_select01" > <option value=0>在线</option> <option value=1>已下线</option> <option value=2>未知</option> <option value=3>故障</option> <option value=4>备用</option> </select> </td> </tr> <tr> <td class="ui_text_rt">所属机房</td> <td class="ui_text_lt"> <select name="securitydevicemachinaroom" id="securitydevicemachinaroom" class="ui_select01"> {% for mr in machinaroomlist %} <option value={{ mr.id }}>{{ mr.name }}</option> {% endfor %} </select> </td> </tr> <tr> <td> </td> <td class="ui_text_lt"> <input id="submitbutton" type="submit" value="提交" class="ui_input_btn01"/> <input id="cancelbutton" type="cancel" value="取消" class="ui_input_btn01"/> </td> </tr> </table> </div> </div>
</form>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>CMDB</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.7.1.js"></script>
<link href="/static/style/authority/basic_layout.css" rel="stylesheet" type="text/css">
<link href="/static/style/authority/common_style.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="/static/scripts/authority/commonAll.js"></script>
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.4.4.min.js"></script>
<script src="/static/scripts/My97DatePicker/WdatePicker.js" type="text/javascript" defer="defer"></script>
<script type="text/javascript" src="/static/scripts/artDialog/artDialog.js?skin=default"></script>
</head>
<body>
<form id="editstoragedeviceform" name="editstoragedeviceform" action="edit_storagedevice.html" method="post">
<div id="container">
<div id="nav_links">
当前位置:服务器资产管理 > <span style="color: #1A5CC6;">编辑存储设备</span>
<div id="page_close">
<a href="javascript:parent.$.fancybox.close();">
<img src="/static/images/common/page_close.png" width="20" height="20" style="vertical-align: text-top;"/>
</a>
</div>
</div>
<div class="ui_content"><input style="visibility:hidden" value={{ storagedevice.id }} type="text" name="id" id="id" />
<table cellspacing="0" cellpadding="0" width="100%" align="left" border="0">
<tr>
<td class="ui_text_rt">设备名字</td>
<td class="ui_text_lt">
<input type="text" id="storagedevicehostname" name="storagedevicehostname" value="{{ storagedevice.hostname }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">制造商/型号</td>
<td class="ui_text_lt">
<input type="text" id="storagedevicemodel" name="storagedevicemodel" value="{{ storagedevice.model }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">IP</td>
<td class="ui_text_lt">
<input type="text" id="storagedeviceip" name="storagedeviceip" value="{{ storagedevice.ip }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">硬盘信息</td>
<td class="ui_text_lt">
<input type="text" id="storagedevicedisk" name="storagedevicedisk" value="{{ storagedevice.disk }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
<td class="ui_text_rt">备注</td>
<td class="ui_text_lt">
<input type="text" id="storagedevicememo" name="storagedevicememo" value="{{ storagedevice.memo }}" class="ui_input_txt02" />
</td>
</tr>
<tr>
</tr>
<tr> <td class="ui_text_rt">状态</td> <td class="ui_text_lt"> <select name="storagedevicestatus" id="storagedevicestatus" class="ui_select01" > <option value=0>在线</option> <option value=1>已下线</option> <option value=2>未知</option> <option value=3>故障</option> <option value=4>备用</option> </select> </td> </tr> <tr> <td class="ui_text_rt">所属机房</td> <td class="ui_text_lt"> <select name="storagedevicemachinaroom" id="storagedevicemachinaroom" class="ui_select01"> {% for mr in machinaroomlist %} <option value={{ mr.id }}>{{ mr.name }}</option> {% endfor %} </select> </td> </tr> <tr> <td> </td> <td class="ui_text_lt"> <input id="submitbutton" type="submit" value="提交" class="ui_input_btn01"/> <input id="cancelbutton" type="cancel" value="取消" class="ui_input_btn01"/> </td> </tr> </table> </div> </div>
</form>
</body>
</html>
{% extends 'base.html' %}
{% block title %}
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.7.1.js"></script>
<link href="/static/style/authority/basic_layout.css" rel="stylesheet" type="text/css">
<link href="/static/style/authority/common_style.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="/static/scripts/authority/commonAll.js"></script>
<script type="text/javascript" src="/static/scripts/fancybox/jquery.fancybox-1.3.4.js"></script>
<script type="text/javascript" src="/static/scripts/fancybox/jquery.fancybox-1.3.4.pack.js"></script>
<link rel="stylesheet" type="text/css" href="/static/style/authority/jquery.fancybox-1.3.4.css" media="screen"></link>
<script type="text/javascript" src="/static/scripts/artDialog/artDialog.js?skin=default"></script>
<div id="container">
<div class="ui_content">
<div class="ui_text_indent">
<div id="box_border">
<div id="box_top">搜索</div>
机房名字 <input type="text" id="mname" name="mname" value="{{ mname }}" class="ui_input_txt03" />
设备名字 <input type="text" id="sname" name="sname" value="{{ sname }}" class="ui_input_txt03" />
ip地址 <input type="text" id="ip" name="ip" value="{{ ip }}" class="ui_input_txt03" />
制造商/型号 <input type="text" id="os" name="ip" value="{{ os }}" class="ui_input_txt03" />
状态
<select id="status" name="status" class="ui_select02">
<option value=100 selected = "selected">所有</option>
<option value=0 {% if status == 0 %} selected = "selected" {% endif %}>在线</option>
<option value=1 {% if status == 1 %} selected = "selected" {% endif %}>已下线</option>
<option value=2 {% if status == 2 %} selected = "selected" {% endif %}>未知</option>
<option value=3 {% if status == 3 %} selected = "selected" {% endif %}>故障</option>
<option value=4 {% if status == 4 %} selected = "selected" {% endif %}>备用</option>
</select>
</div>
<div id="box_bottom">
<div class="pagination">
<span class="current">
{% if networkdevicelist.has_previous %}
<a href="javascript:void(0)" onclick="search_networkdevice({{ networkdevicelist.previous_page_number }});">上一页</a>
{% endif %}
<span class="current"> Page {{ networkdevicelist.number }} of {{ networkdevicelist.paginator.num_pages }}. </span> {% if networkdevicelist.has_next %} <a href="javascript:void(0)" onclick="search_networkdevice({{ networkdevicelist.next_page_number }});">下一页</a> {% endif %} </span>
</div>
<input type="button" value="查询" class="ui_input_btn01" onclick="search_networkdevice(1);"/>
<input type="button" value="新增" class="ui_input_btn01" onclick="add_networkdevice();" />
<input type="button" value="删除" class="ui_input_btn01" onclick="batdel_networkdevice();" />
<input type="button" value="导出EXCEL" class="ui_input_btn01" onclick="excel_networkdevice();" />
</div>
</form>
</div>
</div>
{% endblock %}
{% block content %}
<div class="ui_content">
<div class="ui_tb">
<table class="table" cellspacing="0" cellpadding="0" width="100%" align="center" border="0">
<tr>
<th width="30"><input type="checkbox" id="id" name="id" />
</th>
<th>设备名字</th>
<th>制造商/型号</th>
<th>IP</th>
<th>端口数</th>
<th>备注</th>
<th>状态</th>
<th>机房</th>
<th>操作</th>
</tr>
{% for s in networkdevicelist.object_list %}
<tr>
<td><input type="checkbox" name="idcheck" value={{ s.id }} class="acb" /></td>
<td>{{ s.hostname }}</td>
<td>{{ s.model }}</td>
<td>{{ s.ip }}</td>
<td>{{ s.port_num }}</td>
<td>{{ s.memo }}</td>
<td>{% if s.status == 0 %}
在线
{% elif s.status == 1 %}
已下线
{% elif s.status == 2 %}
未知
{% elif s.status == 3 %}
故障
{% elif s.status == 4 %}
备用
{% endif %}
</td>
<td>{{ s.machinaroom.name }}</td>
<td>
<a href="javascript:void(0)" onclick="edit_networkdevice('{{ s.id }}')" class="edit">编辑</a>
<a href="javascript:void(0)" onclick="del_networkdevice({{ s.id }},{{ networkdevicelist.number }});">删除</a>
</td>
</tr>
{% endfor %}
</table>
</div>
</div>
</div>
<script type="text/javascript">
function search_networkdevice(page){
var mname = document.getElementById("mname").value;
var sname = document.getElementById("sname").value;
var ip= document.getElementById("ip").value;
var os= document.getElementById("os").value;
var status = document.getElementById("status").value;
var myurl="search_networkdevice.html"+"?"+"mname="+mname+"&&sname="+sname+"&&ip="+ip+"&&os="+os+"&&status="+status+"&&page="+page;
window.location.assign(encodeURI(myurl))
}
function add_networkdevice(){
var width = 400;
var height = 500;
var left = parseInt((screen.availWidth/2) - (width/2));//屏幕居中
var top = parseInt((screen.availHeight/2) - (height/2));
var windowFeatures = "width=" + width + ",height=" + height + ",status,resizable,left=" + left + ",top=" + top + "screenX=" + left + ",screenY=" + top;
newWindow = window.open("add_networkdevice.html", "subWind", windowFeatures);
}
function edit_networkdevice(id){
var width = 400;
var height = 500;
var left = parseInt((screen.availWidth/2) - (width/2));//屏幕居中
var top = parseInt((screen.availHeight/2) - (height/2));
var windowFeatures = "width=" + width + ",height=" + height + ",status,resizable,left=" + left + ",top=" + top + "screenX=" + left + ",screenY=" + top;
newWindow = window.open("edit_networkdevice.html?id="+id, "subWind", windowFeatures);
}
function del_networkdevice(id,page){
if(window.confirm('确定要删除该记录吗?')){
var text1= document.getElementById("mname").value;
var sname=document.getElementById("sname").value;
var ip=document.getElementById("ip").value;
var os=document.getElementById("os").value;
var status=document.getElementById("status").value;
var myurl="del_networkdevice.html"+"?"+"id="+id+"&&mname="+text1+"&&page="+page+"&&ip="+ip+"&&os="+os+"&&status="+status+"&&sname="+sname;
window.location.assign(encodeURI(myurl))
return true;
}else{
//alert("取消");
return false;
}
}
function batdel_networkdevice(){
if(window.confirm('确定要删除记录吗?')){
obj = document.getElementsByName("idcheck");
var text1= document.getElementById("mname").value;
var sname=document.getElementById("sname").value;
var ip=document.getElementById("ip").value;
var os=document.getElementById("os").value;
var status=document.getElementById("status").value;
check_val = [];
for(k in obj){
if(obj[k].checked)
check_val.push(obj[k].value);
}
var myurl="batdel_networkdevice.html"+"?"+"ids="+check_val+"&&mname="+text1+"&&ip="+ip+"&&os="+os+"&&status="+status+"&&sname="+sname;
window.location.assign(encodeURI(myurl))
return true;
}else{
return false;
}
}
function excel_networkdevice(){
var text1 = document.getElementById("mname").value;
var sname=document.getElementById("sname").value;
var ip=document.getElementById("ip").value;
var os=document.getElementById("os").value;
var status=document.getElementById("status").value;
var myurl="excel_networkdevice.html"+"?mname="+text1+"&&ip="+ip+"&&os="+os+"&&status="+status+"&&sname="+sname;
window.location.assign(encodeURI(myurl))
}
</script>
{% endblock %}
{% extends 'base.html' %}
{% block title %}
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.7.1.js"></script>
<link href="/static/style/authority/basic_layout.css" rel="stylesheet" type="text/css">
<link href="/static/style/authority/common_style.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="/static/scripts/authority/commonAll.js"></script>
<script type="text/javascript" src="/static/scripts/fancybox/jquery.fancybox-1.3.4.js"></script>
<script type="text/javascript" src="/static/scripts/fancybox/jquery.fancybox-1.3.4.pack.js"></script>
<link rel="stylesheet" type="text/css" href="/static/style/authority/jquery.fancybox-1.3.4.css" media="screen"></link>
<script type="text/javascript" src="/static/scripts/artDialog/artDialog.js?skin=default"></script>
<div id="container">
<div class="ui_content">
<div class="ui_text_indent">
<div id="box_border">
<div id="box_top">搜索</div>
机房名字 <input type="text" id="mname" name="mname" value="{{ mname }}" class="ui_input_txt04" />
设备名字 <input type="text" id="sname" name="sname" value="{{ sname }}" class="ui_input_txt04" />
ip地址 <input type="text" id="ip" name="ip" value="{{ ip }}" class="ui_input_txt04" />
制造商/型号 <input type="text" id="model" name="model" value="{{ model }}" class="ui_input_txt04" />
类型 <input type="text" id="type" name="type" value="{{ type }}" class="ui_input_txt04" />
状态
<select id="status" name="status" class="ui_select02">
<option value=100 selected = "selected">所有</option>
<option value=0 {% if status == 0 %} selected = "selected" {% endif %}>在线</option>
<option value=1 {% if status == 1 %} selected = "selected" {% endif %}>已下线</option>
<option value=2 {% if status == 2 %} selected = "selected" {% endif %}>未知</option>
<option value=3 {% if status == 3 %} selected = "selected" {% endif %}>故障</option>
<option value=4 {% if status == 4 %} selected = "selected" {% endif %}>备用</option>
</select>
</div>
<div id="box_bottom">
<div class="pagination">
<span class="current">
{% if securitydevicelist.has_previous %}
<a href="javascript:void(0)" onclick="search_securitydevice({{ securitydevicelist.previous_page_number }});">上一页</a>
{% endif %}
<span class="current"> Page {{ securitydevicelist.number }} of {{ securitydevicelist.paginator.num_pages }}. </span> {% if securitydevicelist.has_next %} <a href="javascript:void(0)" onclick="search_securitydevice({{ securitydevicelist.next_page_number }});">下一页</a> {% endif %} </span>
</div>
<input type="button" value="查询" class="ui_input_btn01" onclick="search_securitydevice(1);"/>
<input type="button" value="新增" class="ui_input_btn01" onclick="add_securitydevice();" />
<input type="button" value="删除" class="ui_input_btn01" onclick="batdel_securitydevice();" />
<input type="button" value="导出EXCEL" class="ui_input_btn01" onclick="excel_securitydevice();" />
</div>
</form>
</div>
</div>
{% endblock %}
{% block content %}
<div class="ui_content">
<div class="ui_tb">
<table class="table" cellspacing="0" cellpadding="0" width="100%" align="center" border="0">
<tr>
<th width="30"><input type="checkbox" id="id" name="id" />
</th>
<th>设备名字</th>
<th>制造商/型号</th>
<th>IP</th>
<th>端口数</th>
<th>类型</th>
<th>备注</th>
<th>状态</th>
<th>机房</th>
<th>操作</th>
</tr>
{% for s in securitydevicelist.object_list %}
<tr>
<td><input type="checkbox" name="idcheck" value={{ s.id }} class="acb" /></td>
<td>{{ s.hostname }}</td>
<td>{{ s.model }}</td>
<td>{{ s.ip }}</td>
<td>{{ s.port_num }}</td>
<td>{{ s.type }}</td>
<td>{{ s.memo }}</td>
<td>{% if s.status == 0 %}
在线
{% elif s.status == 1 %}
已下线
{% elif s.status == 2 %}
未知
{% elif s.status == 3 %}
故障
{% elif s.status == 4 %}
备用
{% endif %}
</td>
<td>{{ s.machinaroom.name }}</td>
<td>
<a href="javascript:void(0)" onclick="edit_securitydevice('{{ s.id }}')" class="edit">编辑</a>
<a href="javascript:void(0)" onclick="del_securitydevice({{ s.id }},{{ securitydevicelist.number }});">删除</a>
</td>
</tr>
{% endfor %}
</table>
</div>
</div>
</div>
<script type="text/javascript">
function search_securitydevice(page){
var mname = document.getElementById("mname").value;
var sname = document.getElementById("sname").value;
var ip= document.getElementById("ip").value;
var model= document.getElementById("model").value;
var type=document.getElementById("type").value;
var status = document.getElementById("status").value;
var myurl="search_securitydevice.html"+"?"+"mname="+mname+"&&sname="+sname+"&&ip="+ip+"&&model="+model+"&&type="+type+"&&status="+status+"&&page="+page;
window.location.assign(encodeURI(myurl))
}
function add_securitydevice(){
var width = 400;
var height = 500;
var left = parseInt((screen.availWidth/2) - (width/2));//屏幕居中
var top = parseInt((screen.availHeight/2) - (height/2));
var windowFeatures = "width=" + width + ",height=" + height + ",status,resizable,left=" + left + ",top=" + top + "screenX=" + left + ",screenY=" + top;
newWindow = window.open("add_securitydevice.html", "subWind", windowFeatures);
}
function edit_securitydevice(id){
var width = 400;
var height = 500;
var left = parseInt((screen.availWidth/2) - (width/2));//屏幕居中
var top = parseInt((screen.availHeight/2) - (height/2));
var windowFeatures = "width=" + width + ",height=" + height + ",status,resizable,left=" + left + ",top=" + top + "screenX=" + left + ",screenY=" + top;
newWindow = window.open("edit_securitydevice.html?id="+id, "subWind", windowFeatures);
}
function del_securitydevice(id,page){
if(window.confirm('确定要删除该记录吗?')){
var text1= document.getElementById("mname").value;
var sname=document.getElementById("sname").value;
var ip=document.getElementById("ip").value;
var model=document.getElementById("model").value;
var type=document.getElementById("type").value;
var status=document.getElementById("status").value;
var myurl="del_securitydevice.html"+"?"+"id="+id+"&&mname="+text1+"&&page="+page+"&&ip="+ip+"&&model="+model+"&&type="+type+"&&status="+status+"&&sname="+sname;
window.location.assign(encodeURI(myurl))
return true;
}else{
//alert("取消");
return false;
}
}
function batdel_securitydevice(){
if(window.confirm('确定要删除记录吗?')){
obj = document.getElementsByName("idcheck");
var text1= document.getElementById("mname").value;
var sname=document.getElementById("sname").value;
var ip=document.getElementById("ip").value;
var model=document.getElementById("model").value;
var type=document.getElementById("type").value;
var status=document.getElementById("status").value;
check_val = [];
for(k in obj){
if(obj[k].checked)
check_val.push(obj[k].value);
}
var myurl="batdel_securitydevice.html"+"?"+"ids="+check_val+"&&mname="+text1+"&&ip="+ip+"&&model="+model+"&&type="+type+"&&status="+status+"&&sname="+sname;
window.location.assign(encodeURI(myurl))
return true;
}else{
return false;
}
}
function excel_securitydevice(){
var text1 = document.getElementById("mname").value;
var sname=document.getElementById("sname").value;
var ip=document.getElementById("ip").value;
var model=document.getElementById("model").value;
var type=document.getElementById("type").value;
var status=document.getElementById("status").value;
var myurl="excel_securitydevice.html"+"?mname="+text1+"&&ip="+ip+"&&model="+model+"&&type="+type+"&&status="+status+"&&sname="+sname;
window.location.assign(encodeURI(myurl))
}
</script>
{% endblock %}
{% extends 'base.html' %}
{% block title %}
<script type="text/javascript" src="/static/scripts/jquery/jquery-1.7.1.js"></script>
<link href="/static/style/authority/basic_layout.css" rel="stylesheet" type="text/css">
<link href="/static/style/authority/common_style.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="/static/scripts/authority/commonAll.js"></script>
<script type="text/javascript" src="/static/scripts/fancybox/jquery.fancybox-1.3.4.js"></script>
<script type="text/javascript" src="/static/scripts/fancybox/jquery.fancybox-1.3.4.pack.js"></script>
<link rel="stylesheet" type="text/css" href="/static/style/authority/jquery.fancybox-1.3.4.css" media="screen"></link>
<script type="text/javascript" src="/static/scripts/artDialog/artDialog.js?skin=default"></script>
<div id="container">
<div class="ui_content">
<div class="ui_text_indent">
<div id="box_border">
<div id="box_top">搜索</div>
机房名字 <input type="text" id="mname" name="mname" value="{{ mname }}" class="ui_input_txt03" />
设备名字 <input type="text" id="sname" name="sname" value="{{ sname }}" class="ui_input_txt03" />
ip地址 <input type="text" id="ip" name="ip" value="{{ ip }}" class="ui_input_txt03" />
制造商/型号 <input type="text" id="model" name="model" value="{{ model }}" class="ui_input_txt03" />
状态
<select id="status" name="status" class="ui_select02">
<option value=100 selected = "selected">所有</option>
<option value=0 {% if status == 0 %} selected = "selected" {% endif %}>在线</option>
<option value=1 {% if status == 1 %} selected = "selected" {% endif %}>已下线</option>
<option value=2 {% if status == 2 %} selected = "selected" {% endif %}>未知</option>
<option value=3 {% if status == 3 %} selected = "selected" {% endif %}>故障</option>
<option value=4 {% if status == 4 %} selected = "selected" {% endif %}>备用</option>
</select>
</div>
<div id="box_bottom">
<div class="pagination">
<span class="current">
{% if storagedevicelist.has_previous %}
<a href="javascript:void(0)" onclick="search_storagedevice({{ storagedevicelist.previous_page_number }});">上一页</a>
{% endif %}
<span class="current"> Page {{ storagedevicelist.number }} of {{ storagedevicelist.paginator.num_pages }}. </span> {% if storagedevicelist.has_next %} <a href="javascript:void(0)" onclick="search_storagedevice({{ storagedevicelist.next_page_number }});">下一页</a> {% endif %} </span>
</div>
<input type="button" value="查询" class="ui_input_btn01" onclick="search_storagedevice(1);"/>
<input type="button" value="新增" class="ui_input_btn01" onclick="add_storagedevice();" />
<input type="button" value="删除" class="ui_input_btn01" onclick="batdel_storagedevice();" />
<input type="button" value="导出EXCEL" class="ui_input_btn01" onclick="excel_storagedevice();" />
</div>
</form>
</div>
</div>
{% endblock %}
{% block content %}
<div class="ui_content">
<div class="ui_tb">
<table class="table" cellspacing="0" cellpadding="0" width="100%" align="center" border="0">
<tr>
<th width="30"><input type="checkbox" id="id" name="id" />
</th>
<th>设备名字</th>
<th>制造商/型号</th>
<th>IP</th>
<th>容量信息</th>
<th>备注</th>
<th>状态</th>
<th>机房</th>
<th>操作</th>
</tr>
{% for s in storagedevicelist.object_list %}
<tr>
<td><input type="checkbox" name="idcheck" value={{ s.id }} class="acb" /></td>
<td>{{ s.hostname }}</td>
<td>{{ s.model }}</td>
<td>{{ s.ip }}</td>
<td>{{ s.disk }}</td>
<td>{{ s.memo }}</td>
<td>{% if s.status == 0 %}
在线
{% elif s.status == 1 %}
已下线
{% elif s.status == 2 %}
未知
{% elif s.status == 3 %}
故障
{% elif s.status == 4 %}
备用
{% endif %}
</td>
<td>{{ s.machinaroom.name }}</td>
<td>
<a href="javascript:void(0)" onclick="edit_storagedevice('{{ s.id }}')" class="edit">编辑</a>
<a href="javascript:void(0)" onclick="del_storagedevice({{ s.id }},{{ storagedevicelist.number }});">删除</a>
</td>
</tr>
{% endfor %}
</table>
</div>
</div>
</div>
<script type="text/javascript">
function search_storagedevice(page){
var mname = document.getElementById("mname").value;
var sname = document.getElementById("sname").value;
var ip= document.getElementById("ip").value;
var model= document.getElementById("model").value;
var status = document.getElementById("status").value;
var myurl="search_storagedevice.html"+"?"+"mname="+mname+"&&sname="+sname+"&&ip="+ip+"&&model="+model+"&&status="+status+"&&page="+page;
window.location.assign(encodeURI(myurl))
}
function add_storagedevice(){
var width = 400;
var height = 500;
var left = parseInt((screen.availWidth/2) - (width/2));//屏幕居中
var top = parseInt((screen.availHeight/2) - (height/2));
var windowFeatures = "width=" + width + ",height=" + height + ",status,resizable,left=" + left + ",top=" + top + "screenX=" + left + ",screenY=" + top;
newWindow = window.open("add_storagedevice.html", "subWind", windowFeatures);
}
function edit_storagedevice(id){
var width = 400;
var height = 500;
var left = parseInt((screen.availWidth/2) - (width/2));//屏幕居中
var top = parseInt((screen.availHeight/2) - (height/2));
var windowFeatures = "width=" + width + ",height=" + height + ",status,resizable,left=" + left + ",top=" + top + "screenX=" + left + ",screenY=" + top;
newWindow = window.open("edit_storagedevice.html?id="+id, "subWind", windowFeatures);
}
function del_storagedevice(id,page){
if(window.confirm('确定要删除该记录吗?')){
var text1= document.getElementById("mname").value;
var sname=document.getElementById("sname").value;
var ip=document.getElementById("ip").value;
var model=document.getElementById("model").value;
var status=document.getElementById("status").value;
var myurl="del_storagedevice.html"+"?"+"id="+id+"&&mname="+text1+"&&page="+page+"&&ip="+ip+"&&model="+model+"&&status="+status+"&&sname="+sname;
window.location.assign(encodeURI(myurl))
return true;
}else{
//alert("取消");
return false;
}
}
function batdel_storagedevice(){
if(window.confirm('确定要删除记录吗?')){
obj = document.getElementsByName("idcheck");
var text1= document.getElementById("mname").value;
var sname=document.getElementById("sname").value;
var ip=document.getElementById("ip").value;
var model=document.getElementById("model").value;
var status=document.getElementById("status").value;
check_val = [];
for(k in obj){
if(obj[k].checked)
check_val.push(obj[k].value);
}
var myurl="batdel_storagedevice.html"+"?"+"ids="+check_val+"&&mname="+text1+"&&ip="+ip+"&&model="+model+"&&status="+status+"&&sname="+sname;
window.location.assign(encodeURI(myurl))
return true;
}else{
return false;
}
}
function excel_storagedevice(){
var text1 = document.getElementById("mname").value;
var sname=document.getElementById("sname").value;
var ip=document.getElementById("ip").value;
var model=document.getElementById("model").value;
var status=document.getElementById("status").value;
var myurl="excel_storagedevice.html"+"?mname="+text1+"&&ip="+ip+"&&model="+model+"&&status="+status+"&&sname="+sname;
window.location.assign(encodeURI(myurl))
}
</script>
{% endblock %}
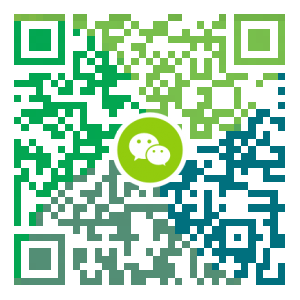
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
用 go 写 WebAssembly入门
Golang WebAssembly 入门 Golang 在1.11版本中引入了 WebAssembly 支持,意味着以后可以用 go编写可以在浏览器中运行的程序,当然这个肯定也是要受浏览器沙盒环境约束的. 1. 浏览器中运行 Go 1.1 code package main func main() { println("Hello, WebAssembly!") } 1.2 编译 必须是 go1.11才行 GOARCH=wasm GOOS=js go build -o test.wasm main.go 1.3 运行 单独的 wasm 文件是无法直接运行的,必须载入浏览器中. mkdir test cp test.wasm test cp $GOROOT/misc/wasm/wasm_exec.{html,js} . 1.3.1 一个测试 http 服务器 chrome 是不支持本地文件中运行 wasm 的,所以必须有一个 http 服务器 //http.go package main import ( "flag" "log" "net/http" "strings" ) var ...
- 下一篇
wordpress个人网站优化心得
第一:文武双全使用了阿里云公共DNS 2014年6月6日,阿里云推出了自己的公共DNS,文武双全就把用了N久的电信DNS换成了阿里云公共DNS。 由于使用了WDCP面板,在wdcp后台修改服务器的DNS也是轻而易举的事情。 第二:文武双全使用了阿里云的云盾保护网站 现在新购买的阿里云主机,默认都开通了云盾的。但是把网站加入云盾的网站安全防御(也就是WAF)内,还需要手动修改域名解析。文武双全的个人网站,早就把所有域名都放到WAF的保护之下。 第三:选择合适的Wordpress主题 文武双全个人网站使用的是威言威语的Weisay Simple主题模板,威言威语自模板推出后的几年时间内一直在坚持不断的优化主题。使得主题的代码越来越规范,使用该主题的网站速度自然也得益不少。 从Weisay Simple主题本身来说,首页模板、文章页模板以及列表页模板都没有太大的图片。主题更多的是文字内容与文字列表,也保证了使用该款主题本身的速度就非常的快。 第四:设置正确的图片缩略图的尺寸 Weisay Simple主题的每篇文章前面有一个图片缩略图,文武双全使用了NextGEN Gallery插件管理网站...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- SpringBoot2初体验,简单认识spring boot2并且搭建基础工程
- CentOS8安装Docker,最新的服务器搭配容器使用
- SpringBoot2整合Thymeleaf,官方推荐html解决方案
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- CentOS7,8上快速安装Gitea,搭建Git服务器
- 设置Eclipse缩进为4个空格,增强代码规范
- SpringBoot2全家桶,快速入门学习开发网站教程
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- Red5直播服务器,属于Java语言的直播服务器
- Docker安装Oracle12C,快速搭建Oracle学习环境