【java】简单实现数据库连接池
一直在想java事务是怎么实现的,在原声jdbc的时候级别下,我们可以通过关掉autocommit 然后再手动commit。但是项目开发中基本上是看不见conection的。所以自己决定简单实现框架的一点皮毛功能。首先就是数据库连接池了
1. 先定义一个接口
import java.sql.Connection; public interface IConnectionPool { /** * 获取一个连接 * @return */ Connection getConnection(); /** * 用完后调用,把连接放回池中,实现复用 */ void freeLocalConnection(); /** * 销毁连接池 */ void destroy(); //测试用 void status(); }
2. 实现数据库连接池的代码, 为了线程安全,简单粗暴地用synchronized关键字
实现事务的关键是,我们执行一个事务的Connection是同一个,我们可以在事务控制的时候用AOP,在事务开始的时候 调用setAutoCommit(false) 然后在事务代码之后调用commit()方法.
所以在数据库连接池中使用了一个ThreadLocal来保证一条线程拿到的是同一个Connection。
import java.io.IOException; import java.io.InputStream; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.util.List; import java.util.Properties; import java.util.Vector; import java.util.concurrent.atomic.AtomicInteger; public class ConnectionPollImpl implements IConnectionPool{ private String username; private String password; private String url; private String driver; private Integer maxSize; private Integer initSize = 5; private long timeOut; //连接总数 private AtomicInteger totalSize = new AtomicInteger(0); //空闲的连接 private List<Connection> freeConnections = new Vector<>(); //已经被使用的连接 private List<Connection> activeConnections = new Vector<>(); //存储当前线程的连接, 事务控制的关键 private ThreadLocal<Connection> localConnection = new ThreadLocal<Connection>(){ /** * 第一次调用get()方法时执行 * @return */ @Override protected Connection initialValue() { try { return connect(); } catch (SQLException e) { e.printStackTrace(); } return null; } @Override public void remove() { Connection connection = get(); activeConnections.remove(connection); freeConnections.add(connection); super.remove(); } }; private static ConnectionPollImpl instance; public ConnectionPollImpl() { loadConfig(); init(); } private void init() { try { for(int i=0;i < initSize;i++){ freeConnections.add(newConnection()); } } catch (SQLException e) { e.printStackTrace(); } } public static ConnectionPollImpl getInstance() { synchronized (ConnectionPollImpl.class) { if (instance == null) { synchronized (ConnectionPollImpl.class) { if(instance == null) { instance = new ConnectionPollImpl(); } } } } return instance; } @Override public synchronized Connection getConnection() { return localConnection.get(); } @Override public void freeLocalConnection() { localConnection.remove(); System.out.println(Thread.currentThread().getName() + "释放了一个连接"); } @Override public synchronized void destroy() { try { for(Connection connection : freeConnections) { freeConnections.remove(connection); connection.close(); } freeConnections = null; for (Connection connection : activeConnections) { activeConnections.remove(connection); connection.close(); } activeConnections = null; } catch (SQLException e) { e.printStackTrace(); } } @Override public synchronized void status() { System.out.println("当前连接池总连接数为: " + totalSize.get() + " , 空闲连接数为:" + freeConnections.size() + "使用中的连接数为:" + activeConnections.size()); } private synchronized Connection connect() throws SQLException { // 判断有没有闲置的连接 if(freeConnections.size() > 0) { //如果有闲置连接,直接拿第一个 Connection connection = freeConnections.get(0); freeConnections.remove(0); //连接可用,返回;不可用,继续拿 if (isValid(connection)) { activeConnections.add(connection); return connection; } else { return connect(); } } else { //没有闲置连接, 判断当前连接池是否饱和 if(totalSize.get() == maxSize) { //如果饱和,等待, 继续获取 try { wait(timeOut); } catch (InterruptedException e) { e.printStackTrace(); } return connect(); } else { //没有饱和,新建一个连接 Connection connection = newConnection(); if(connection != null) { activeConnections.add(connection); return connection; } else { throw new SQLException(); } } } } private synchronized Connection newConnection() throws SQLException { try { Class.forName(this.driver); } catch (ClassNotFoundException e) { e.printStackTrace(); } Connection connection = DriverManager.getConnection(url, username, password); totalSize.incrementAndGet(); return connection; } private boolean isValid(Connection connection) { try { return connection != null && !connection.isClosed(); } catch (SQLException e) { e.printStackTrace(); } return false; } private void loadConfig(){ //读取配置文件 InputStream in = ConnectionPollImpl.class.getClassLoader().getResourceAsStream("jdbc.properties"); Properties p = new Properties(); try { p.load(in); } catch (IOException e) { e.printStackTrace(); } finally { try { in.close(); } catch (IOException e) { e.printStackTrace(); } } this.username = p.getProperty("jdbc.username"); this.password = p.getProperty("jdbc.password"); this.url = p.getProperty("jdbc.url"); this.driver = p.getProperty("jdbc.driver"); this.maxSize = Integer.valueOf(p.getProperty("noob.maxSize","20")); this.initSize = Integer.valueOf(p.getProperty("noob.initSize","5")); this.timeOut = Long.valueOf(p.getProperty("noob.timeOut","1200")); } }
测试代码
import java.sql.Connection; import java.util.Random; import java.util.concurrent.Executors; import java.util.concurrent.ScheduledExecutorService; import java.util.concurrent.TimeUnit; public class Test { public static void main(String[] args) { IConnectionPool connectionPool = ConnectionPollImpl.getInstance(); //开启一个线程查看连接池的状态 ScheduledExecutorService service = Executors.newScheduledThreadPool(1); service.scheduleWithFixedDelay(connectionPool::status, 0, 5, TimeUnit.SECONDS); //开启20个线程,不断获取连接,比较哈希值看同一个线程取出的连接是不是同一个 for(int i = 0; i < 20; i++) { Random random = new Random(); int count = random.nextInt(30) + 3; Thread t = new Thread(() ->{ try { for (int j = 0; j < count; j++) { Connection connection = connectionPool.getConnection(); System.out.println(Thread.currentThread().getName() + "共" + count + "次循环, 目前第" + (j + 1) + "次" + " hashcode :" + connection.hashCode()); TimeUnit.SECONDS.sleep(1); } } catch (InterruptedException e) { e.printStackTrace(); } connectionPool.freeLocalConnection(); }); t.setName("test" + i); t.start(); } } }
测试结果, 从结果看基本实现了想要的功能
1. 控制连接池的大小
2. 一个线程释放一个连接后会把连接放回池中给别的线程用
3. 一个线程始终取出同一个连接
当前连接池总连接数为: 5 , 空闲连接数为:5使用中的连接数为:0 test6共22次循环, 目前第1次 hashcode :691902360 test2共18次循环, 目前第1次 hashcode :708075980 test3共16次循环, 目前第1次 hashcode :1535444742 test4共25次循环, 目前第1次 hashcode :1149790650 test5共15次循环, 目前第1次 hashcode :1825737020 test1共16次循环, 目前第1次 hashcode :2094482202 test0共21次循环, 目前第1次 hashcode :889774551 test18共16次循环, 目前第1次 hashcode :1626524709 test11共17次循环, 目前第1次 hashcode :912223199 test19共28次循环, 目前第1次 hashcode :422379330 test3共16次循环, 目前第2次 hashcode :1535444742 test2共18次循环, 目前第2次 hashcode :708075980 test6共22次循环, 目前第2次 hashcode :691902360 test5共15次循环, 目前第2次 hashcode :1825737020 test4共25次循环, 目前第2次 hashcode :1149790650 test1共16次循环, 目前第2次 hashcode :2094482202 test0共21次循环, 目前第2次 hashcode :889774551 test18共16次循环, 目前第2次 hashcode :1626524709 test11共17次循环, 目前第2次 hashcode :912223199 test19共28次循环, 目前第2次 hashcode :422379330 test2共18次循环, 目前第3次 hashcode :708075980 test4共25次循环, 目前第3次 hashcode :1149790650 test3共16次循环, 目前第3次 hashcode :1535444742 test6共22次循环, 目前第3次 hashcode :691902360 test5共15次循环, 目前第3次 hashcode :1825737020 test1共16次循环, 目前第3次 hashcode :2094482202 test0共21次循环, 目前第3次 hashcode :889774551 test18共16次循环, 目前第3次 hashcode :1626524709 test11共17次循环, 目前第3次 hashcode :912223199 test19共28次循环, 目前第3次 hashcode :422379330 test5共15次循环, 目前第4次 hashcode :1825737020 test2共18次循环, 目前第4次 hashcode :708075980 test6共22次循环, 目前第4次 hashcode :691902360 test3共16次循环, 目前第4次 hashcode :1535444742 test4共25次循环, 目前第4次 hashcode :1149790650 test1共16次循环, 目前第4次 hashcode :2094482202 test0共21次循环, 目前第4次 hashcode :889774551 test18共16次循环, 目前第4次 hashcode :1626524709 test11共17次循环, 目前第4次 hashcode :912223199 test19共28次循环, 目前第4次 hashcode :422379330 test3共16次循环, 目前第5次 hashcode :1535444742 test5共15次循环, 目前第5次 hashcode :1825737020 test6共22次循环, 目前第5次 hashcode :691902360 test4共25次循环, 目前第5次 hashcode :1149790650 test2共18次循环, 目前第5次 hashcode :708075980 test1共16次循环, 目前第5次 hashcode :2094482202 test0共21次循环, 目前第5次 hashcode :889774551 test18共16次循环, 目前第5次 hashcode :1626524709 test11共17次循环, 目前第5次 hashcode :912223199 test19共28次循环, 目前第5次 hashcode :422379330 test3共16次循环, 目前第6次 hashcode :1535444742 test5共15次循环, 目前第6次 hashcode :1825737020 test4共25次循环, 目前第6次 hashcode :1149790650 test2共18次循环, 目前第6次 hashcode :708075980 test6共22次循环, 目前第6次 hashcode :691902360 当前连接池总连接数为: 10 , 空闲连接数为:0使用中的连接数为:10 test1共16次循环, 目前第6次 hashcode :2094482202 test0共21次循环, 目前第6次 hashcode :889774551 test18共16次循环, 目前第6次 hashcode :1626524709 test11共17次循环, 目前第6次 hashcode :912223199 test19共28次循环, 目前第6次 hashcode :422379330 test2共18次循环, 目前第7次 hashcode :708075980 test4共25次循环, 目前第7次 hashcode :1149790650 test6共22次循环, 目前第7次 hashcode :691902360 test3共16次循环, 目前第7次 hashcode :1535444742 test5共15次循环, 目前第7次 hashcode :1825737020 test1共16次循环, 目前第7次 hashcode :2094482202 test0共21次循环, 目前第7次 hashcode :889774551 test18共16次循环, 目前第7次 hashcode :1626524709 test11共17次循环, 目前第7次 hashcode :912223199 test19共28次循环, 目前第7次 hashcode :422379330 test3共16次循环, 目前第8次 hashcode :1535444742 test5共15次循环, 目前第8次 hashcode :1825737020 test2共18次循环, 目前第8次 hashcode :708075980 test6共22次循环, 目前第8次 hashcode :691902360 test4共25次循环, 目前第8次 hashcode :1149790650 test1共16次循环, 目前第8次 hashcode :2094482202 test0共21次循环, 目前第8次 hashcode :889774551 test18共16次循环, 目前第8次 hashcode :1626524709 test11共17次循环, 目前第8次 hashcode :912223199 test19共28次循环, 目前第8次 hashcode :422379330 test5共15次循环, 目前第9次 hashcode :1825737020 test4共25次循环, 目前第9次 hashcode :1149790650 test3共16次循环, 目前第9次 hashcode :1535444742 test2共18次循环, 目前第9次 hashcode :708075980 test6共22次循环, 目前第9次 hashcode :691902360 test1共16次循环, 目前第9次 hashcode :2094482202 test0共21次循环, 目前第9次 hashcode :889774551 test18共16次循环, 目前第9次 hashcode :1626524709 test11共17次循环, 目前第9次 hashcode :912223199 test19共28次循环, 目前第9次 hashcode :422379330 test5共15次循环, 目前第10次 hashcode :1825737020 test6共22次循环, 目前第10次 hashcode :691902360 test3共16次循环, 目前第10次 hashcode :1535444742 test2共18次循环, 目前第10次 hashcode :708075980 test4共25次循环, 目前第10次 hashcode :1149790650 test1共16次循环, 目前第10次 hashcode :2094482202 test0共21次循环, 目前第10次 hashcode :889774551 test18共16次循环, 目前第10次 hashcode :1626524709 test11共17次循环, 目前第10次 hashcode :912223199 test19共28次循环, 目前第10次 hashcode :422379330 当前连接池总连接数为: 10 , 空闲连接数为:0使用中的连接数为:10 test5共15次循环, 目前第11次 hashcode :1825737020 test3共16次循环, 目前第11次 hashcode :1535444742 test6共22次循环, 目前第11次 hashcode :691902360 test4共25次循环, 目前第11次 hashcode :1149790650 test2共18次循环, 目前第11次 hashcode :708075980 test1共16次循环, 目前第11次 hashcode :2094482202 test0共21次循环, 目前第11次 hashcode :889774551 test18共16次循环, 目前第11次 hashcode :1626524709 test11共17次循环, 目前第11次 hashcode :912223199 test19共28次循环, 目前第11次 hashcode :422379330 test2共18次循环, 目前第12次 hashcode :708075980 test5共15次循环, 目前第12次 hashcode :1825737020 test3共16次循环, 目前第12次 hashcode :1535444742 test6共22次循环, 目前第12次 hashcode :691902360 test4共25次循环, 目前第12次 hashcode :1149790650 test1共16次循环, 目前第12次 hashcode :2094482202 test0共21次循环, 目前第12次 hashcode :889774551 test18共16次循环, 目前第12次 hashcode :1626524709 test11共17次循环, 目前第12次 hashcode :912223199 test19共28次循环, 目前第12次 hashcode :422379330 test6共22次循环, 目前第13次 hashcode :691902360 test2共18次循环, 目前第13次 hashcode :708075980 test3共16次循环, 目前第13次 hashcode :1535444742 test5共15次循环, 目前第13次 hashcode :1825737020 test4共25次循环, 目前第13次 hashcode :1149790650 test1共16次循环, 目前第13次 hashcode :2094482202 test0共21次循环, 目前第13次 hashcode :889774551 test18共16次循环, 目前第13次 hashcode :1626524709 test11共17次循环, 目前第13次 hashcode :912223199 test19共28次循环, 目前第13次 hashcode :422379330 test3共16次循环, 目前第14次 hashcode :1535444742 test5共15次循环, 目前第14次 hashcode :1825737020 test6共22次循环, 目前第14次 hashcode :691902360 test4共25次循环, 目前第14次 hashcode :1149790650 test2共18次循环, 目前第14次 hashcode :708075980 test1共16次循环, 目前第14次 hashcode :2094482202 test0共21次循环, 目前第14次 hashcode :889774551 test18共16次循环, 目前第14次 hashcode :1626524709 test11共17次循环, 目前第14次 hashcode :912223199 test19共28次循环, 目前第14次 hashcode :422379330 test2共18次循环, 目前第15次 hashcode :708075980 test5共15次循环, 目前第15次 hashcode :1825737020 test4共25次循环, 目前第15次 hashcode :1149790650 test6共22次循环, 目前第15次 hashcode :691902360 test3共16次循环, 目前第15次 hashcode :1535444742 test1共16次循环, 目前第15次 hashcode :2094482202 test0共21次循环, 目前第15次 hashcode :889774551 test18共16次循环, 目前第15次 hashcode :1626524709 test11共17次循环, 目前第15次 hashcode :912223199 test19共28次循环, 目前第15次 hashcode :422379330 当前连接池总连接数为: 10 , 空闲连接数为:0使用中的连接数为:10 test6共22次循环, 目前第16次 hashcode :691902360 test4共25次循环, 目前第16次 hashcode :1149790650 test5释放了一个连接 test3共16次循环, 目前第16次 hashcode :1535444742 test2共18次循环, 目前第16次 hashcode :708075980 test1共16次循环, 目前第16次 hashcode :2094482202 test0共21次循环, 目前第16次 hashcode :889774551 test18共16次循环, 目前第16次 hashcode :1626524709 test11共17次循环, 目前第16次 hashcode :912223199 test19共28次循环, 目前第16次 hashcode :422379330 test7共6次循环, 目前第1次 hashcode :1825737020 test2共18次循环, 目前第17次 hashcode :708075980 test3释放了一个连接 test6共22次循环, 目前第17次 hashcode :691902360 test4共25次循环, 目前第17次 hashcode :1149790650 test1释放了一个连接 test0共21次循环, 目前第17次 hashcode :889774551 test18释放了一个连接 test11共17次循环, 目前第17次 hashcode :912223199 test19共28次循环, 目前第17次 hashcode :422379330 test7共6次循环, 目前第2次 hashcode :1825737020 test12共12次循环, 目前第1次 hashcode :2094482202 test15共12次循环, 目前第1次 hashcode :1626524709 test8共31次循环, 目前第1次 hashcode :1535444742 test4共25次循环, 目前第18次 hashcode :1149790650 test2共18次循环, 目前第18次 hashcode :708075980 test6共22次循环, 目前第18次 hashcode :691902360 test0共21次循环, 目前第18次 hashcode :889774551 test11释放了一个连接 test19共28次循环, 目前第18次 hashcode :422379330 test7共6次循环, 目前第3次 hashcode :1825737020 test8共31次循环, 目前第2次 hashcode :1535444742 test12共12次循环, 目前第2次 hashcode :2094482202 test15共12次循环, 目前第2次 hashcode :1626524709 test4共25次循环, 目前第19次 hashcode :1149790650 test6共22次循环, 目前第19次 hashcode :691902360 test2释放了一个连接 test0共21次循环, 目前第19次 hashcode :889774551 test13共30次循环, 目前第1次 hashcode :912223199 test19共28次循环, 目前第19次 hashcode :422379330 test14共27次循环, 目前第1次 hashcode :708075980 test7共6次循环, 目前第4次 hashcode :1825737020 test12共12次循环, 目前第3次 hashcode :2094482202 test15共12次循环, 目前第3次 hashcode :1626524709 test8共31次循环, 目前第3次 hashcode :1535444742 test6共22次循环, 目前第20次 hashcode :691902360 test4共25次循环, 目前第20次 hashcode :1149790650 test0共21次循环, 目前第20次 hashcode :889774551 test13共30次循环, 目前第2次 hashcode :912223199 test19共28次循环, 目前第20次 hashcode :422379330 test14共27次循环, 目前第2次 hashcode :708075980 test7共6次循环, 目前第5次 hashcode :1825737020 test8共31次循环, 目前第4次 hashcode :1535444742 test15共12次循环, 目前第4次 hashcode :1626524709 test12共12次循环, 目前第4次 hashcode :2094482202 当前连接池总连接数为: 10 , 空闲连接数为:0使用中的连接数为:10 test6共22次循环, 目前第21次 hashcode :691902360 test4共25次循环, 目前第21次 hashcode :1149790650 test0共21次循环, 目前第21次 hashcode :889774551 test13共30次循环, 目前第3次 hashcode :912223199 test19共28次循环, 目前第21次 hashcode :422379330 test14共27次循环, 目前第3次 hashcode :708075980 test7共6次循环, 目前第6次 hashcode :1825737020 test12共12次循环, 目前第5次 hashcode :2094482202 test8共31次循环, 目前第5次 hashcode :1535444742 test15共12次循环, 目前第5次 hashcode :1626524709 test4共25次循环, 目前第22次 hashcode :1149790650 test6共22次循环, 目前第22次 hashcode :691902360 test0释放了一个连接 test19共28次循环, 目前第22次 hashcode :422379330 test13共30次循环, 目前第4次 hashcode :912223199 test14共27次循环, 目前第4次 hashcode :708075980 test16共19次循环, 目前第1次 hashcode :889774551 test7释放了一个连接 test8共31次循环, 目前第6次 hashcode :1535444742 test12共12次循环, 目前第6次 hashcode :2094482202 test15共12次循环, 目前第6次 hashcode :1626524709 test4共25次循环, 目前第23次 hashcode :1149790650 test6释放了一个连接 test19共28次循环, 目前第23次 hashcode :422379330 test14共27次循环, 目前第5次 hashcode :708075980 test13共30次循环, 目前第5次 hashcode :912223199 test16共19次循环, 目前第2次 hashcode :889774551 test12共12次循环, 目前第7次 hashcode :2094482202 test9共15次循环, 目前第1次 hashcode :691902360 test17共15次循环, 目前第1次 hashcode :1825737020 test8共31次循环, 目前第7次 hashcode :1535444742 test15共12次循环, 目前第7次 hashcode :1626524709 test4共25次循环, 目前第24次 hashcode :1149790650 test19共28次循环, 目前第24次 hashcode :422379330 test14共27次循环, 目前第6次 hashcode :708075980 test13共30次循环, 目前第6次 hashcode :912223199 test16共19次循环, 目前第3次 hashcode :889774551 test8共31次循环, 目前第8次 hashcode :1535444742 test12共12次循环, 目前第8次 hashcode :2094482202 test17共15次循环, 目前第2次 hashcode :1825737020 test9共15次循环, 目前第2次 hashcode :691902360 test15共12次循环, 目前第8次 hashcode :1626524709 test4共25次循环, 目前第25次 hashcode :1149790650 test19共28次循环, 目前第25次 hashcode :422379330 test13共30次循环, 目前第7次 hashcode :912223199 test14共27次循环, 目前第7次 hashcode :708075980 test16共19次循环, 目前第4次 hashcode :889774551 test12共12次循环, 目前第9次 hashcode :2094482202 test9共15次循环, 目前第3次 hashcode :691902360 test17共15次循环, 目前第3次 hashcode :1825737020 test8共31次循环, 目前第9次 hashcode :1535444742 test15共12次循环, 目前第9次 hashcode :1626524709 当前连接池总连接数为: 10 , 空闲连接数为:0使用中的连接数为:10 test4释放了一个连接 test13共30次循环, 目前第8次 hashcode :912223199 test19共28次循环, 目前第26次 hashcode :422379330 test14共27次循环, 目前第8次 hashcode :708075980 test10共17次循环, 目前第1次 hashcode :1149790650 test16共19次循环, 目前第5次 hashcode :889774551 test9共15次循环, 目前第4次 hashcode :691902360 test12共12次循环, 目前第10次 hashcode :2094482202 test8共31次循环, 目前第10次 hashcode :1535444742 test17共15次循环, 目前第4次 hashcode :1825737020 test15共12次循环, 目前第10次 hashcode :1626524709 test19共28次循环, 目前第27次 hashcode :422379330 test13共30次循环, 目前第9次 hashcode :912223199 test14共27次循环, 目前第9次 hashcode :708075980 test10共17次循环, 目前第2次 hashcode :1149790650 test16共19次循环, 目前第6次 hashcode :889774551 test8共31次循环, 目前第11次 hashcode :1535444742 test17共15次循环, 目前第5次 hashcode :1825737020 test12共12次循环, 目前第11次 hashcode :2094482202 test9共15次循环, 目前第5次 hashcode :691902360 test15共12次循环, 目前第11次 hashcode :1626524709 test14共27次循环, 目前第10次 hashcode :708075980 test13共30次循环, 目前第10次 hashcode :912223199 test19共28次循环, 目前第28次 hashcode :422379330 test10共17次循环, 目前第3次 hashcode :1149790650 test16共19次循环, 目前第7次 hashcode :889774551 test9共15次循环, 目前第6次 hashcode :691902360 test12共12次循环, 目前第12次 hashcode :2094482202 test8共31次循环, 目前第12次 hashcode :1535444742 test17共15次循环, 目前第6次 hashcode :1825737020 test15共12次循环, 目前第12次 hashcode :1626524709 test14共27次循环, 目前第11次 hashcode :708075980 test19释放了一个连接 test13共30次循环, 目前第11次 hashcode :912223199 test10共17次循环, 目前第4次 hashcode :1149790650 test16共19次循环, 目前第8次 hashcode :889774551 test9共15次循环, 目前第7次 hashcode :691902360 test12释放了一个连接 test17共15次循环, 目前第7次 hashcode :1825737020 test8共31次循环, 目前第13次 hashcode :1535444742 test15释放了一个连接 test13共30次循环, 目前第12次 hashcode :912223199 test14共27次循环, 目前第12次 hashcode :708075980 test10共17次循环, 目前第5次 hashcode :1149790650 test16共19次循环, 目前第9次 hashcode :889774551 test9共15次循环, 目前第8次 hashcode :691902360 test8共31次循环, 目前第14次 hashcode :1535444742 test17共15次循环, 目前第8次 hashcode :1825737020 当前连接池总连接数为: 10 , 空闲连接数为:3使用中的连接数为:7 test14共27次循环, 目前第13次 hashcode :708075980 test13共30次循环, 目前第13次 hashcode :912223199 test10共17次循环, 目前第6次 hashcode :1149790650 test16共19次循环, 目前第10次 hashcode :889774551 test8共31次循环, 目前第15次 hashcode :1535444742 test17共15次循环, 目前第9次 hashcode :1825737020 test9共15次循环, 目前第9次 hashcode :691902360 test14共27次循环, 目前第14次 hashcode :708075980 test13共30次循环, 目前第14次 hashcode :912223199 test10共17次循环, 目前第7次 hashcode :1149790650 test16共19次循环, 目前第11次 hashcode :889774551 test17共15次循环, 目前第10次 hashcode :1825737020 test8共31次循环, 目前第16次 hashcode :1535444742 test9共15次循环, 目前第10次 hashcode :691902360 test13共30次循环, 目前第15次 hashcode :912223199 test14共27次循环, 目前第15次 hashcode :708075980 test10共17次循环, 目前第8次 hashcode :1149790650 test16共19次循环, 目前第12次 hashcode :889774551 test8共31次循环, 目前第17次 hashcode :1535444742 test9共15次循环, 目前第11次 hashcode :691902360 test17共15次循环, 目前第11次 hashcode :1825737020 test13共30次循环, 目前第16次 hashcode :912223199 test14共27次循环, 目前第16次 hashcode :708075980 test10共17次循环, 目前第9次 hashcode :1149790650 test16共19次循环, 目前第13次 hashcode :889774551 test17共15次循环, 目前第12次 hashcode :1825737020 test8共31次循环, 目前第18次 hashcode :1535444742 test9共15次循环, 目前第12次 hashcode :691902360 test13共30次循环, 目前第17次 hashcode :912223199 test14共27次循环, 目前第17次 hashcode :708075980 test10共17次循环, 目前第10次 hashcode :1149790650 test16共19次循环, 目前第14次 hashcode :889774551 test9共15次循环, 目前第13次 hashcode :691902360 test8共31次循环, 目前第19次 hashcode :1535444742 test17共15次循环, 目前第13次 hashcode :1825737020 当前连接池总连接数为: 10 , 空闲连接数为:3使用中的连接数为:7 test13共30次循环, 目前第18次 hashcode :912223199 test14共27次循环, 目前第18次 hashcode :708075980 test10共17次循环, 目前第11次 hashcode :1149790650 test16共19次循环, 目前第15次 hashcode :889774551 test8共31次循环, 目前第20次 hashcode :1535444742 test9共15次循环, 目前第14次 hashcode :691902360 test17共15次循环, 目前第14次 hashcode :1825737020 test14共27次循环, 目前第19次 hashcode :708075980 test13共30次循环, 目前第19次 hashcode :912223199 test10共17次循环, 目前第12次 hashcode :1149790650 test16共19次循环, 目前第16次 hashcode :889774551 test8共31次循环, 目前第21次 hashcode :1535444742 test9共15次循环, 目前第15次 hashcode :691902360 test17共15次循环, 目前第15次 hashcode :1825737020 test13共30次循环, 目前第20次 hashcode :912223199 test14共27次循环, 目前第20次 hashcode :708075980 test10共17次循环, 目前第13次 hashcode :1149790650 test16共19次循环, 目前第17次 hashcode :889774551 test8共31次循环, 目前第22次 hashcode :1535444742 test17释放了一个连接 test9释放了一个连接 test13共30次循环, 目前第21次 hashcode :912223199 test14共27次循环, 目前第21次 hashcode :708075980 test10共17次循环, 目前第14次 hashcode :1149790650 test16共19次循环, 目前第18次 hashcode :889774551 test8共31次循环, 目前第23次 hashcode :1535444742 test13共30次循环, 目前第22次 hashcode :912223199 test14共27次循环, 目前第22次 hashcode :708075980 test10共17次循环, 目前第15次 hashcode :1149790650 test16共19次循环, 目前第19次 hashcode :889774551 test8共31次循环, 目前第24次 hashcode :1535444742 当前连接池总连接数为: 10 , 空闲连接数为:5使用中的连接数为:5 test14共27次循环, 目前第23次 hashcode :708075980 test13共30次循环, 目前第23次 hashcode :912223199 test10共17次循环, 目前第16次 hashcode :1149790650 test16释放了一个连接 test8共31次循环, 目前第25次 hashcode :1535444742 test13共30次循环, 目前第24次 hashcode :912223199 test14共27次循环, 目前第24次 hashcode :708075980 test10共17次循环, 目前第17次 hashcode :1149790650 test8共31次循环, 目前第26次 hashcode :1535444742 test14共27次循环, 目前第25次 hashcode :708075980 test13共30次循环, 目前第25次 hashcode :912223199 test10释放了一个连接 test8共31次循环, 目前第27次 hashcode :1535444742 test14共27次循环, 目前第26次 hashcode :708075980 test13共30次循环, 目前第26次 hashcode :912223199 test8共31次循环, 目前第28次 hashcode :1535444742 test13共30次循环, 目前第27次 hashcode :912223199 test14共27次循环, 目前第27次 hashcode :708075980 test8共31次循环, 目前第29次 hashcode :1535444742 当前连接池总连接数为: 10 , 空闲连接数为:7使用中的连接数为:3 test13共30次循环, 目前第28次 hashcode :912223199 test14释放了一个连接 test8共31次循环, 目前第30次 hashcode :1535444742 test13共30次循环, 目前第29次 hashcode :912223199 test8共31次循环, 目前第31次 hashcode :1535444742 test13共30次循环, 目前第30次 hashcode :912223199 test8释放了一个连接 test13释放了一个连接 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0 当前连接池总连接数为: 10 , 空闲连接数为:10使用中的连接数为:0
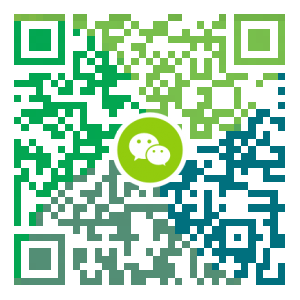
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
java源码-ThreadPoolExecutor(3)
开篇 部门以前有一个很跳的程序员写了一个神奇的代码,通过不停创建ThreadPoolExecutor而不调用shutdown导致线程过多溢出,然后想想毕竟我还是一个不跳的程序员,所以还是研究研究比较合适。这篇文章就是说明线程池的退出过程。java源码-ThreadPoolExecutor(1)java源码-ThreadPoolExecutor(2)java源码-ThreadPoolExecutor(3) shutdown源码解析 1、上锁,mainLock是线程池的主锁,是可重入锁,当要操作workers set这个保持线程的HashSet时,需要先获取mainLock,还有当要处理largestPoolSize、completedTaskCount这类统计数据时需要先获取mainLock 2、判断调用者是否有权限shutdown线程池 3、使用CAS操作将线程池状态设置为shutdown,shutdown之后将不再接收新任务 4、中断所有空闲线程 interruptIdleWorkers() 5、onShutdown(),ScheduledThreadPoolExecutor中实现...
- 下一篇
【java】对jdbc操作结果简单的映射封装
1. 对jdbc做一个简单的封装,select可以返回一个javabean对象,而不是resultset。主要用了反射。这是我之前写的代码,做了简单的修改。 实现功能:a.对数据库的基本操作 增删改查 b.对查询的单条记录返回一个指定类型的javabean对象,利用java反射,jdbc ResultSet类和ResultSetMetaData类 c. 对查到的结果集返回一个List, 泛型 数据源:用到的 数据库连接池是我自己简单实现的一个连接池:【java】简单实现数据库连接池,主要为了后续实现事务的简单实现 用到的java知识 : 反射,泛型,jdbc import com.yeyeck.noob.ConnectionPollImpl; import com.yeyeck.noob.IConnectionPool; import java.lang.reflect.Field; import java.lang.reflect.InvocationTargetException; import java.lang.reflect.Method; import java.sq...
相关文章
文章评论
共有0条评论来说两句吧...