第11章—使用对象关系映射持久化数据—SpringBoot+SpringData+Jpa进行查询修改数据库
SpringBoot+SpringData+Jpa进行查询修改数据库
JPA由EJB 3.0软件专家组开发,作为JSR-220实现的一部分。但它又不限于EJB 3.0,你可以在Web应用、甚至桌面应用中使用。JPA的宗旨是为POJO提供持久化标准规范,由此可见,经过这几年的实践探索,能够脱离容器独立运行,方便开发和测试的理念已经深入人心了。Hibernate3.2+、TopLink 10.1.3以及OpenJPA都提供了JPA的实现。
JPA的总体思想和现有Hibernate、TopLink、JDO等ORM框架大体一致。总的来说,JPA包括以下3方面的技术:
ORM映射元数据
JPA支持XML和JDK5.0注解两种元数据的形式,元数据描述对象和表之间的映射关系,框架据此将实体对象持久化到数据库表中;
API
用来操作实体对象,执行CRUD操作,框架在后台替代我们完成所有的事情,开发者从繁琐的JDBC和SQL代码中解脱出来。
查询语言
这是持久化操作中很重要的一个方面,通过面向对象而非面向数据库的查询语言查询数据,避免程序的SQL语句紧密耦合。
例子:SpringBoot+SpringData+Jpa
这里我的Demo的结构图如下:
相关的代码如下:
pom.xml:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.beacon</groupId> <artifactId>springdata-jpa</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <description>Demo project for Spring Boot</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.0.3.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-configuration-processor</artifactId> <optional>true</optional> </dependency> <!--数据库组件--> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <!--数据库组件--> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid</artifactId> <version>1.1.10</version> </dependency> <!--//添加AOP--> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-aop</artifactId> </dependency> <!-- Spring boot 引用Thymeleaf模板依赖包(Thymeleaf模板如果不适用,这里也可以不添加这段配置,Thymeleaf模板使用在下面会讲到) --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
StudentController:
package com.example.springdatajpa.controller; import com.example.springdatajpa.entity.Student; import com.example.springdatajpa.service.StudentService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RestController; import java.util.List; @RestController public class StudentController { @Autowired private StudentService studentService; @GetMapping("/getAllStu") public List<Student> getAllStu(){ return studentService.findAll(); } @GetMapping("/findById/{id}") public Student findById(@PathVariable("id")Integer id){ return studentService.findById(id); } //根据money查询 再根据age排序 @GetMapping("/findByMoney/{money}") public List<Student> findByMoney(@PathVariable("money")Integer money,Integer age){ return studentService.findByMoneyOrAgeOrderByAgeDesc(money,age); } @GetMapping("/findByName/{name}") public Student findByNameIgnoresCase(@PathVariable("name")String name){ return studentService.findByName(name); } }
StudentDao:
package com.example.springdatajpa.dao; import com.example.springdatajpa.entity.Student; import org.springframework.data.jpa.repository.JpaRepository; import java.util.List; public interface StudentDao extends JpaRepository<Student,Object> { Student findById(Integer id); public List<Student> findByMoneyOrAgeOrderByAgeDesc(Integer money, Integer age) ; Student findByName(String name); }
Student:
package com.example.springdatajpa.entity; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name="student") public class Student { private Integer id; private String name; private Integer age; private String password; private Integer money; //注意:这里一定要在主键的get方法上加上这两个注解 // @Id // @GeneratedValue // 不然会报错找不到 @Id @GeneratedValue public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public Integer getMoney() { return money; } public void setMoney(Integer money) { this.money = money; } @Override public String toString() { return "Student{" + "id=" + id + ", name='" + name + '\'' + ", age=" + age + ", password='" + password + '\'' + ", money=" + money + '}'; } }
StudentService
package com.example.springdatajpa.service; import com.example.springdatajpa.entity.Student; import java.util.List; public interface StudentService { List<Student> findAll(); Student findById(Integer id); List<Student> findByMoneyOrAgeOrderByAgeDesc(Integer money, Integer age); Student findByName(String name); }
StudentServiceImpl
package com.example.springdatajpa.service.impl; import com.example.springdatajpa.dao.StudentDao; import com.example.springdatajpa.entity.Student; import com.example.springdatajpa.service.StudentService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import org.springframework.web.bind.annotation.PathVariable; import java.util.List; @Service public class StudentServiceImpl implements StudentService { @Autowired private StudentDao studentDao; @Override public List<Student> findAll() { return studentDao.findAll(); } @Override public Student findById(Integer id) { return studentDao.findById(id); } @Override public List<Student> findByMoneyOrAgeOrderByAgeDesc(Integer money, Integer age) { return studentDao.findByMoneyOrAgeOrderByAgeDesc(money,age); } @Override public Student findByName(String name) { return studentDao.findByName(name); } }
application.yml:
server: port: 8089 spring: datasource: url: jdbc:mysql://localhost:3306/test?useSSL=false username: root password: root driver-class-name: com.mysql.jdbc.Driver type: com.alibaba.druid.pool.DruidDataSource initialSize: 20 minIdle: 50 maxActive: 80 maxWait: 10000 timeBetweenEvictionRunsMillis: 60000 minEvictableIdleTimeMills: 300000 jpa: hibernate: ddl-auto: update generate-ddl: true show-sql: true # thymeleaf thymeleaf: prefix: classpath:templates/ suffix: .html mode: HTML5 encoding: utf-8 servlet: content-type: text/html cache: false
这里我们可以使用的是JPA的定义查询,相应的方法名的规则如下:
相应的演示如下:
访问:http://localhost:8089/getAllStu
访问:http://localhost:8089/findByMoney/9(这个地方可以把相应的数据查出来并按照相应的字段进行排序)
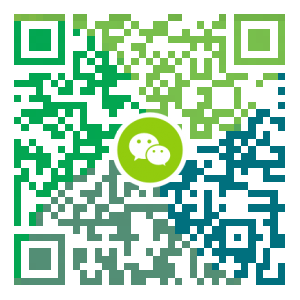
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
Java多线程进一步的理解之------------缓存机制
public class CacheDemo { private Map<String,Object> cache = new HashMap<String,Object>(50); public static void main(String[] args) { } /* * 多个线程的并发执行,保证数据正确 * */ private ReadWriteLock rwl = new ReentrantReadWriteLock(); public Object getData(String key) { rwl.readLock().lock();// 加读锁,都可以读, Object value = null; try{ value = cache.get(key); if (value == null) { rwl.readLock().unlock(); // 释放读锁,都不可以读,让第一个线程去数据库中查数据,查好后输出, rwl.writeLock().lock(); // 加写锁,只有第一个线程可以加写锁添加成功, try { if (value ...
- 下一篇
Java 最常用的10大算法
String/Array/Matrix 在Java中,String是一个包含char数组和其它字段、方法的类。 toCharArray() //get char array of a String Arrays.sort() //sort an array Arrays.toString(char[] a) //convert to string charAt(int x) //get a char at the specific index length() //string length length //array size substring(int beginIndex) substring(int beginIndex, int endIndex) Integer.valueOf()//string to integer String.valueOf()/integer to string 逆波兰表示法最长回文字符串单词分割字梯两个排序数组的中值正则表达式匹配合并间隔
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- CentOS8编译安装MySQL8.0.19
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- SpringBoot2整合MyBatis,连接MySql数据库做增删改查操作
- SpringBoot2整合Redis,开启缓存,提高访问速度
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- Hadoop3单机部署,实现最简伪集群
- CentOS7,CentOS8安装Elasticsearch6.8.6
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- SpringBoot2编写第一个Controller,响应你的http请求并返回结果