Springmvc常见问题
问题一:org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'userController': Failed to introspect bean class [com.blog.controller.UserController] for lookup method metadata: could not find class that it depends on; nested exception is java.lang.NoClassDefFoundError: javax/mail/MessagingException
出现这种问题的原因是因为,以我个人博客为例,我在注册中用到了这个邮箱工具类,邮箱工具类默认抛出Exception和MessagingException异常,然后我去除了该工具类,但未将异常去掉,然后在项目启动中直接报错。如果是用jsp作为表现层直接到首页或者其他界面直接会报500异常。所以说,我们在Controller中的方法,有时throws异常要慎用。
普遍的解决办法:就是MVC模式以jsp作为表现层,控制器-模型-视图,通过这种视图跳转的方式,邮箱注册发送邮件是没有任何问题的。如果用纯HTML或纯jsp,而不是通过视图跳转到jsp。
正常情况下,视图应该是这样的。
@RequestMapping("test") public String test(){ return "test"; }
通过上述这种方式跳转jsp,而不是直接浏览器输入,例如这样的,localhost:8080/test/test.jsp。当然这样返回也可以,但是不太符合MVC这种方式。
问题二:
描述:为什么返回重复的url呢?明明写了正确的路径。出现这种情况比较多的是由SpringMVC返回JSON数据与前台进行异步交互。‘
问题原因:出现这个的问题的原因是因为我的url正常应该给前台返回json数据,通常在springmvc中url要给前台返回json,必须要定义一个ResponseBody,而我没有定义这个,所以出现了这个异常。
解决办法:
加上ResponseBody时,就能正常访问到JSON数据
如图所示:
不过通常针对控制层而言,如果某个类基本返回的是JSON,建议使用RestController
这样一来不必每一个方法上都添加@ResponseBody
重复性添加该注解是一件很麻烦的事情,总而言之能偷懒就偷点懒。
下面贴一下RestController的源代码:
/* * Copyright 2002-2017 the original author or authors. * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. */ package org.springframework.web.bind.annotation; import java.lang.annotation.Documented; import java.lang.annotation.ElementType; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.annotation.Target; import org.springframework.stereotype.Controller; /** * A convenience annotation that is itself annotated with * {@link Controller @Controller} and {@link ResponseBody @ResponseBody}. * <p> * Types that carry this annotation are treated as controllers where * {@link RequestMapping @RequestMapping} methods assume * {@link ResponseBody @ResponseBody} semantics by default. * * <p><b>NOTE:</b> {@code @RestController} is processed if an appropriate * {@code HandlerMapping}-{@code HandlerAdapter} pair is configured such as the * {@code RequestMappingHandlerMapping}-{@code RequestMappingHandlerAdapter} * pair which are the default in the MVC Java config and the MVC namespace. * In particular {@code @RestController} is not supported with the * {@code DefaultAnnotationHandlerMapping}-{@code AnnotationMethodHandlerAdapter} * pair both of which are also deprecated. * * @author Rossen Stoyanchev * @author Sam Brannen * @since 4.0 */ @Target(ElementType.TYPE) @Retention(RetentionPolicy.RUNTIME) @Documented @Controller @ResponseBody public @interface RestController { /** * The value may indicate a suggestion for a logical component name, * to be turned into a Spring bean in case of an autodetected component. * @return the suggested component name, if any (or empty String otherwise) * @since 4.0.1 */ String value() default ""; }
通常情况只要在类上面加上诸如RequstMapping或者其他注解,一般都是全局的。
就如RestController源码一样,看它的注解就可以明白它同时具有Controller和ResponseBody两个特性。
关于常用注解这里贴个图,便于回顾:
@Documented的作用:将此注解包含在 javadoc 中 ,它代表着此注解会被javadoc工具提取成文档
这里在顺便贴下Controller的源码:
/* * Copyright 2002-2017 the original author or authors. * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. */ package org.springframework.stereotype; import java.lang.annotation.Documented; import java.lang.annotation.ElementType; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.annotation.Target; /** * Indicates that an annotated class is a "Controller" (e.g. a web controller). * * <p>This annotation serves as a specialization of {@link Component @Component}, * allowing for implementation classes to be autodetected through classpath scanning. * It is typically used in combination with annotated handler methods based on the * {@link org.springframework.web.bind.annotation.RequestMapping} annotation. * * @author Arjen Poutsma * @author Juergen Hoeller * @since 2.5 * @see Component * @see org.springframework.web.bind.annotation.RequestMapping * @see org.springframework.context.annotation.ClassPathBeanDefinitionScanner */ @Target({ElementType.TYPE}) @Retention(RetentionPolicy.RUNTIME) @Documented @Component public @interface Controller { /** * The value may indicate a suggestion for a logical component name, * to be turned into a Spring bean in case of an autodetected component. * @return the suggested component name, if any (or empty String otherwise) */ String value() default ""; }
关于这段源码,简单的说其实关键就是上面的注解。
为什么SpringMVC能够通过@Controller+@RequestMapping得到正确的URL
首先不说@RequestMapping,针对如上源码,之所以可以扫描到这个Conroller,关键就在@Component这个注解上。
这个注解可以理解为Struts2的action,对于struts2而言,每个action是一个实例,因此struts2是多例的。多例意味着,每一个对应的action,必须要在配置文件中配置,因此我们可以看到随着项目的扩大,struts2对应的action相关的xml文件会极速增加的。
而SpringMVC是单例,全局共享一个实例,因此通过@Component直接实例为Spring容器。
简单的说,在我们初学spring时,要想将对象交给Spring进行管理,通常要配置如下的bean:
如下bean的作用主要是扫描com.eluzhu.rms.mapper的文件下的数据访问层的代码,通过该代码就不用一个一个写很多bean
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer"> <property name="basePackage" value="com.eluzhu.rms.mapper"/> </bean>
而@Component在此隐性创建bean,不需要你管理对象,而直接交给spring管理。
这样对于效率的提高是非常有帮助的。
我之所以喜欢SpringMVC的最大原因,是因为简单明了轻量化,struts2的话,当初学习的时候,感觉有不少难度,当然了,学习完后,用的多,习惯就好,但是每次比较烦躁的就是要增加一个类似于Controller作用的类,我都必须要配置一个struts2相关的xml文件。时间久了,CV大法即可搞定,当后来接触SpringMVC时,就发现我再也不想用struts2了。SpringMVC可以说是从Struts2演变而来并借助Spring的名气。
当然了,在校学习Java的同学们,建议还是把Struts2学好,毕竟Struts2的更面向对象。而且涉及不少设计模式。学好Struts2,对理解SpringMVC非常有帮助。
问题三:SpringMVC的配置文件关于注解配置,没有配置好导致本因返回JSON数据,最后却变成了XML。
如图所示:
解决办法:在spring-mvc.xml配置文件,添加如下内容即可解决:
<!-- FastJson注入 --> <mvc:annotation-driven> <mvc:message-converters register-defaults="true"> <!-- 避免IE执行AJAX时,返回JSON出现下载文件 --> <!-- FastJson --> <bean id="fastJsonHttpMessageConverter" class="com.alibaba.fastjson.support.spring.FastJsonHttpMessageConverter"> <property name="supportedMediaTypes"> <list> <!-- 这里顺序不能反,一定先写text/html,不然ie下出现下载提示 --> <value>text/html;charset=UTF-8</value> <value>application/json;charset=UTF-8</value> </list> </property> </bean> </mvc:message-converters> </mvc:annotation-driven>
’
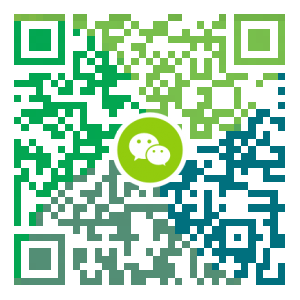
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
想做JavaScript开发者,但不知道如何成长,来看看这个吧
对初学者来说,我们都知道要成为一个优秀的开发者,会有很多东西要学。但是就是因为东西太多了,各种各样的技术,搞得一头雾水,不知道从哪里开始学起。所以我整理了一张脑图,选择了一些最重要或者是比较有价值的技术知识点,供大家作为学习时的参考。 JavaScript开发者知识路线
- 下一篇
云栖社区专家系列课——Java必修课第一讲
本节课为Java必修课第一讲。在本节课中,最课程创始人、微软MVP陆敏枝为了大家介绍了当前Java行业的IT人才供需情况、Java基本语法、JDK的下载与安装、Java环境变量的配置以及第一个Java程序的编写、架构与运行,内容既涵盖理论分析又包括动手实践,对零基础入门Java的初学者而言非常适用。 课程基本信息 开课时间:每周四下午:14:00-15:30 主讲人:云栖社区专家,陆敏枝 直播视频链接: https://yq.aliyun.com/video/play/1492 欢迎下载或在线观看 本节课程重点内容 一、IT人才供需情况以及Java语言在IT行业的不同排名状况 每年IT人才市场缺口约在100万人;同时,根据IEEE Spectrum网站的2017年公布的排名,Java在按雇主需求的语言排名第一,在按趋势发展的排行榜排名第四。Jav
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS8编译安装MySQL8.0.19
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- SpringBoot2整合MyBatis,连接MySql数据库做增删改查操作
- SpringBoot2整合Redis,开启缓存,提高访问速度
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- Hadoop3单机部署,实现最简伪集群
- CentOS7,CentOS8安装Elasticsearch6.8.6
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- SpringBoot2编写第一个Controller,响应你的http请求并返回结果