从Zero到Hero,一文掌握Python关键代码
# 01基础篇
# 变量
1 #int 2 one=1 3 some_number=100 4 5 print("one=",one) #print type1 6 print("some_number=",some_number) #python3 print need add () 7 8 """output 9 one= 1 10 some_number= 100 11 """
1 #booleans 2 true_boolean=True 3 false_boolean=False 4 5 print("true_boolean:",true_boolean) 6 print("False_boolean;",false_boolean) 7 8 """output 9 true_boolean: True 10 False_boolean; False 11 """
1 #string 2 my_name="leizeling" 3 4 print("my_name:{}".format(my_name)) #print type2 5 6 """output 7 true_boolean: True 8 False_boolean; False 9 """
#float book_price=15.80 print("book_price:{}".format(book_price)) """output book_price:15.8 """
# 控制流:条件语句
1 #if 2 if True: 3 print("Hollo Python if") 4 if 2>1: 5 print("2 is greater than 1") 6 7 """output 8 Hollo Python if 9 2 is greater than 1 10 """
1 #if……else 2 if 1>2: 3 print("1 is greater than 2") 4 else: 5 print("1 is not greater than 2") 6 7 """output 8 true_boolean: True 9 False_boolean; False 10 """
#if……elif……else if 1>2: print("1 is greater than 2") elif 1<2: print("1 is not greater than 2") else: print("1 is equal to 2") """output 1 is not greater than 2 """
# 循环/迭代器
#while num=1 while num<=10: print(num) num+=1 """output 1 2 3 4 5 6 7 8 9 10 """
1 #for 2 for i in range(1,11):#range(start,end,step),not including end 3 print(i) 4 5 """output 6 1 7 2 8 3 9 4 10 5 11 6 12 7 13 8 14 9 15 10 16 """
# 02列表:数组数据结构
#create integers list my_integers=[1,2,3,4,5] print(my_integers[0]) print(my_integers[1]) print(my_integers[4]) """output 1 2 5 """
#create str list relatives_name=["Toshiaki","Juliana","Yuji","Bruno","Kaio"] print(relatives_name) """output ['Toshiaki', 'Juliana', 'Yuji', 'Bruno', 'Kaio'] """
1 #add item to list 2 bookshelf=[] 3 bookshelf.append("The Effective Engineer") 4 bookshelf.append("The 4 Hour Work Week") 5 print(bookshelf[0]) 6 print(bookshelf[1]) 7 8 """output 9 The Effective Engineer 10 The 4 Hour Work Week 11 """
# 03字典:键-值数据结构
#dictionary struct dictionary_example={"key1":"value1","key2":"value2","key3":"value3"} dictionary_example """output {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'} """
#create dictionary dictionary_tk={"name":"Leandro","nickname":"Tk","nationality":"Brazilian"} print("My name is %s"%(dictionary_tk["name"])) print("But you can call me %s"%(dictionary_tk["nickname"])) print("And by the waay I am {}".format(dictionary_tk["nationality"])) """output My name is Leandro But you can call me Tk And by the waay I am Brazilian """
1 #dictionary add item 2 dictionary_tk["age"]=24 3 print(dictionary_tk) 4 5 """output 6 {'nationality': 'Brazilian', 'nickname': 'Tk', 'name': 'Leandro', 'age': 24} 7 """
#dictionayr del item dictionary_tk.pop("age") print(dictionary_tk) """output {'nationality': 'Brazilian', 'nickname': 'Tk', 'name': 'Leandro'} """
# 04迭代:数据结构中的循环
#iteration of list bookshelf=["The Effective Engineer","The 4 hours work week","Zero to One","Lean Startup","Hooked"] for book in bookshelf: print(book) """output The Effective Engineer The 4 hours work week Zero to One Lean Startup Hooked """
1 #哈希数据结构(iteration of dictionary) 2 dictionary_tk={"name":"Leandro","nickname":"Tk","nationality":"Brazilian"} 3 for key in dictionary_tk: #the attribute can use any name,not only use "key" 4 print("%s:%s"%(key,dictionary_tk[key])) 5 print("===================") 6 for key,val in dictionary_tk.items(): #attention:this is use dictionary_tk.items().not direct use dictionary_tk 7 print("{}:{}".format(key,val)) 8 9 """output 10 nationality:Brazilian 11 nickname:Tk 12 name:Leandro 13 =================== 14 nationality:Brazilian 15 nickname:Tk 16 name:Leandro 17 """
# 05 类和对象
#define class class Vechicle: pass
#create instance car=Vechicle() print(car) ""output <__main__.Vechicle object at 0x000000E3014EED30> """
#define class class Vechicle(object):#object is a base class def __init__(self,number_of_wheels,type_of_tank,seating_capacity,maximum_velocity): self.number_of_wheels=number_of_wheels self.type_of_tank=type_of_tank self.seating_capacity=seating_capacity self.maximum_velocity=maximum_velocity def get_number_of_wheels(self): return self.number_of_wheels def set_number_of_wheels(self,number): self.number_of_wheels=number #create instance(object) tesla_models_s=Vechicle(4,"electric",5,250) tesla_models_s.set_number_of_wheels(8)#方法的调用显得复杂 tesla_models_s.get_number_of_wheels() """output 8 """
#use @property让方法像属性一样使用 #define class class Vechicle_1(object):#object is a base class def __init__(self,number_of_wheels,type_of_tank,seating_capacity,maximum_velocity):#first attr must is self,do not input the attr self._number_of_wheels=number_of_wheels ###属性名和方法名不要重复 self.type_of_tank=type_of_tank self.seating_capacity=seating_capacity self.maximum_velocity=maximum_velocity @property #读属性 def number_of_wheels(self): return self._number_of_wheels @number_of_wheels.setter #写属性 def number_of_wheels(self,number): self._number_of_wheels=number def make_noise(self): print("VRUUUUUUUM") tesla_models_s_1=Vechicle_1(4,"electric",5,250) print(tesla_models_s_1.number_of_wheels) tesla_models_s_1.number_of_wheels=2 print(tesla_models_s_1.number_of_wheels) print(tesla_models_s_1.make_noise()) """output 4 2 VRUUUUUUUM None """
# 06封装:隐藏信息
1 #公开实例变量 2 class Person: 3 def __init__(self,first_name): 4 self.first_name=first_name 5 tk=Person("TK") 6 print(tk.first_name) 7 tk.first_name="Kaio" 8 print(tk.first_name) 9 10 """output 11 TK 12 Kaio 13 """
#私有实例变量 class Person: def __init__(self,first_name,email): self.first_name=first_name self._email=email def get_email(self): return self._email def update_email(self,email): self._email=email tk=Person("TK","tk@mail.com") tk.update_email("new_tk@mail.com") print(tk.get_email()) """output new_tk@mail.com """
#公有方法 class Person: def __init__(self,first_name,age): self.first_name=first_name self._age=age def show_age(self): return self._age tk=Person("TK",24) print(tk.show_age()) """output 24 """
1 #私有方法 2 class Person: 3 def __init__(self,first_name,age): 4 self.first_name=first_name 5 self._age=age 6 def _show_age(self): 7 return self._age 8 def show_age(self): 9 return self._show_age() #此处的self不要少了 10 tk=Person("TK",24) 11 print(tk.show_age()) 12 13 """output 14 24 15 """
1 #继承 2 class Car:#父类 3 def __init__(self,number_of_wheels,seating_capacity,maximum_velocity): 4 self.number_of_wheels=number_of_wheels 5 self.seating_capacity=seating_capacity 6 self.maximum_velocity=maximum_velocity 7 class ElectricCar(Car):#派生类 8 def __init(self,number_of_wheels_1,seating_capacity_1,maximum_velocity_1): 9 Car.__init__(self,number_of_wheels_1,seating_capacity_1,maximum_velocity_1) #利用父类进行初始化 10 11 my_electric_car=ElectricCar(4,5,250) 12 print(my_electric_car.number_of_wheels) 13 print(my_electric_car.seating_capacity) 14 print(my_electric_car.maximum_velocity) 15 16 """output 17 4 18 5 19 250 20 """
注:从机器之心转载
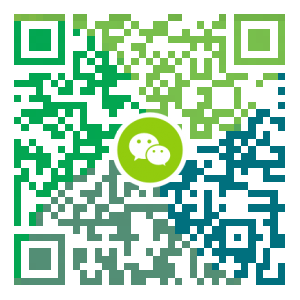
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
专访唐亘:计算机编程和数学建模缺一不可
点击图片购书 参与文末话题讨论,每日赠送异步图书 ——异步小编 异步社区:可以介绍一下自己吗?目前正在做哪些事情? 唐亘:大家好,我叫唐亘,是《精通数据科学:从线性回归到深度学习》一书的作者,现就职于一家叫finogeeks的创业公司。在这家创业公司里面,我主要负责两个项目,一是利用市场上的各种信息,在金融领域里搭建知识图谱;二是构建量化指标体系用于刻画客户的投资行为,并以此为基础搭建个人专属的智能投顾机器人。 异步社区:是什么初衷开始创作《精通数据科学:从线性回归到深度学习》一书?这本书写给哪些人看? 唐亘:现在回想起来,有3个主要的原因促成我开始写这本书吧:目前大热的数据科学(data science)是一门新兴学科,它涉及计算机、计量经济学、机器学习等多方面的内容。但比较遗憾的是,将这3门学科融汇在一起的图书比较少见(在我有限的认知里),因此想通过自己的写作将这点遗憾弥补掉。 网上有关数据科学的资料很多,但这些资料都比较碎片化,不成体系。所以我希望能成体系地写一本书勾勒出有关数据科学的全景图。 从学习经历上来讲,我是一个比较喜欢自学和分享的人,因此很想将自己的学习心得和对学科的...
- 下一篇
ES6 javascript 实用开发技巧
ES6 实用开发技巧 本文只罗列出在 ES6 开发过程中相对实用的内容,而非一个高大全的文档,如果希望查阅详细的内容,可购买阮一峰老师所出版的 ES6 相关图书。 定义变量/常量 ES6 中新增加了 let 和 const 两个命令,let 用于定义变量,const 用于定义常量 两个命令与原有的 var 命令所不同的地方在于,let, const 都是块级作用域,其有效范围仅在代码块中,实例如下: //es5 if(1==1){ var b = 'foo'; } console.log(b);//foo //es6 if(1==1){ let b = 'foo'; } console.log(b);//undefined (b is not defined) 定义常量对象 const a = {a:1,b:2}; a.b = 3; console.log(a);//{a:1,b:3} 上例中,常量 a 中的内容在定义后,再进行修改依然有效,原因是对于对象类型的使用是指针式引用,常量只是指向了对象的指针,对象本身的内容却依然可以被修改,注意,数组(Array) 也是对象; 那么如果在定...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS7设置SWAP分区,小内存服务器的救世主
- Linux系统CentOS6、CentOS7手动修改IP地址
- Docker安装Oracle12C,快速搭建Oracle学习环境
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- Windows10,CentOS7,CentOS8安装MongoDB4.0.16
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- CentOS7,CentOS8安装Elasticsearch6.8.6
- Hadoop3单机部署,实现最简伪集群
- MySQL8.0.19开启GTID主从同步CentOS8