高德地图web端笔记;发送http请求的工具类
1.查询所有电子围栏
package com.skjd.util; import java.io.BufferedReader; import java.io.InputStream; import java.io.InputStreamReader; import java.io.OutputStream; import java.net.HttpURLConnection; import java.net.URL; import java.util.ArrayList; import java.util.List; import java.util.Map; import javax.servlet.http.HttpServletRequest; import org.apache.http.HttpEntity; import org.apache.http.NameValuePair; import org.apache.http.client.entity.UrlEncodedFormEntity; import org.apache.http.client.methods.CloseableHttpResponse; import org.apache.http.client.methods.HttpGet; import org.apache.http.client.methods.HttpPost; import org.apache.http.impl.client.CloseableHttpClient; import org.apache.http.impl.client.HttpClients; import org.apache.http.message.BasicNameValuePair; public class HttpUtils { // 代理服务器的HTTP请求协议,客户端真实IP字段名称 public static final String X_REAL_IP = "x-forwarded-for"; public static String get(final String url) throws Exception { String result = null; CloseableHttpClient httpclient = HttpClients.createDefault(); try { HttpGet httpGet = new HttpGet(url); CloseableHttpResponse response = httpclient.execute(httpGet); try { System.out.println(response.getStatusLine()); HttpEntity entity = response.getEntity(); // do something useful with the response body // and ensure it is fully consumed result = org.apache.http.util.EntityUtils.toString(entity); org.apache.http.util.EntityUtils.consume(entity); } finally { response.close(); } } finally { httpclient.close(); } return result; } public static String post(final String url, final Map<String, String> param) throws Exception { String result = null; CloseableHttpClient httpclient = HttpClients.createDefault(); try { HttpPost httpPost = new HttpPost("http://httpbin.org/post"); List<NameValuePair> nvps = new ArrayList<NameValuePair>(); for (String key : param.keySet()) { nvps.add(new BasicNameValuePair(key, param.get(key))); } httpPost.setEntity(new UrlEncodedFormEntity(nvps)); CloseableHttpResponse response = httpclient.execute(httpPost); try { System.out.println(response.getStatusLine()); HttpEntity entity = response.getEntity(); // do something useful with the response body // and ensure it is fully consumed result = org.apache.http.util.EntityUtils.toString(entity); org.apache.http.util.EntityUtils.consume(entity); } finally { response.close(); } } finally { httpclient.close(); } return result; } /** * 发送POST请求的方法 */ public static String sendPostRequest(String url,String param){ HttpURLConnection httpURLConnection = null; OutputStream out = null; //写 InputStream in = null; //读 int responseCode = 0; //远程主机响应的HTTP状态码 String result=""; try{ URL sendUrl = new URL(url); httpURLConnection = (HttpURLConnection)sendUrl.openConnection(); //post方式请求 httpURLConnection.setRequestMethod("POST"); //设置头部信息 httpURLConnection.setRequestProperty("headerdata", "ceshiyongde"); //一定要设置 Content-Type 要不然服务端接收不到参数 httpURLConnection.setRequestProperty("Content-Type", "application/Json; charset=UTF-8"); //指示应用程序要将数据写入URL连接,其值默认为false(是否传参) httpURLConnection.setDoOutput(true); //httpURLConnection.setDoInput(true); httpURLConnection.setUseCaches(false); httpURLConnection.setConnectTimeout(30000); //30秒连接超时 httpURLConnection.setReadTimeout(30000); //30秒读取超时 //获取输出流 out = httpURLConnection.getOutputStream(); //输出流里写入POST参数 out.write(param.getBytes()); out.flush(); out.close(); responseCode = httpURLConnection.getResponseCode(); BufferedReader br = new BufferedReader(new InputStreamReader(httpURLConnection.getInputStream(),"UTF-8")); result =br.readLine(); }catch(Exception e) { e.printStackTrace(); } return result; } /** * 发送GET请求的方法 */ public static String sendPostRequestGet(String url){ HttpURLConnection httpURLConnection = null; OutputStream out = null; //写 InputStream in = null; //读 int responseCode = 0; //远程主机响应的HTTP状态码 String result=""; try{ URL sendUrl = new URL(url); httpURLConnection = (HttpURLConnection)sendUrl.openConnection(); //post方式请求 httpURLConnection.setRequestMethod("GET"); //设置头部信息 httpURLConnection.setRequestProperty("headerdata", "ceshiyongde"); //一定要设置 Content-Type 要不然服务端接收不到参数 httpURLConnection.setRequestProperty("Content-Type", "application/Json; charset=UTF-8"); //指示应用程序要将数据写入URL连接,其值默认为false(是否传参) httpURLConnection.setDoOutput(true); //httpURLConnection.setDoInput(true); httpURLConnection.setUseCaches(false); httpURLConnection.setConnectTimeout(30000); //30秒连接超时 httpURLConnection.setReadTimeout(30000); //30秒读取超时 responseCode = httpURLConnection.getResponseCode(); BufferedReader br = new BufferedReader(new InputStreamReader(httpURLConnection.getInputStream(),"UTF-8")); result =br.readLine(); }catch(Exception e) { e.printStackTrace(); } return result; } /** * for nigx返向代理构造 获取客户端IP地址 * @param request * @return */ public static String getRemoteHost(HttpServletRequest request){ String ip = request.getHeader(X_REAL_IP); if(ip == null || ip.length() == 0 || ip.equalsIgnoreCase("unknown")) { ip = request.getRemoteAddr(); } return ip.split(",")[0]; } public static void main(String[] args) { try { System.out.println(get("http://www.baidu.com")); } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
/** * 根据key查询所有的围栏 * 返回所有围栏的信息 * @param pd * @return * @throws Exception */ public List<Map<String,Object>> select(Map<String,Object> pd) throws Exception{ Gson gson=new Gson(); String key=pd.get("key").toString(); String url="http://restapi.amap.com/v4/geofence/meta?key=" +key; //请求返回的参数 String data=HttpUtils.sendPostRequestGet(url); //转为对象便于获取 Map<String,Object> dataJson = gson.fromJson(data, HashMap.class); Map<String, Object> map=(LinkedTreeMap)dataJson.get("data"); List<Map<String,Object>> list = (List)map.get("rs_list"); return list; }
2.创建围栏
/** * 传入对应格式的坐标区域,创建一个围栏;返回status(新增成功0,已存在返回1;失败返回2);若新增成功则返回gid;message信息 */ public static PageData xyUtil(PageData pd){ PageData pd2=new PageData(); pd.put("key", "be8e6c802d51a4be29c99be895d8c2c7"); //数据库读取的坐标参数 /*114.288221,30.593268#114.321866,30.580559#114.311223,30.560015#114.291278,30.602487#*/ //第三方接口要求的格式:lon1,lat1;lon2,lat2;lon3,lat3(3<=点个数<=5000) //将#号换为; String points = pd.getString("points").replace("#", ";"); String key=pd.getString("key"); String name=pd.getString("name1"); PageData pd3=new PageData(); pd3.put("points", points); pd3.put("name", name); /*pd3.put("valid_time ", DateUtil.getDay());设置过期时间、默认90天*/ pd3.put("repeat", "Mon,Tues,Wed,Thur,Fri,Sat,Sun");//可以指定每天的监控时间段和一周内监控的日期 Gson gson=new Gson(); //传入的参数 String param=gson.toJson(pd3); String url="http://restapi.amap.com/v4/geofence/meta?key=" + key + "&type=ajaxRequest"; String data=HttpUtil.sendPostRequest(url,param); //请求回来的数据 System.out.println(data); PageData data2 = gson.fromJson(data, pd.getClass()); PageData data3 = gson.fromJson(data2.get("data").toString(), pd.getClass()); //状态为0说明新增成功! if(data3.get("status").toString().equals("0.0")){ pd2.put("status", "0"); pd2.put("gid", data3.get("gid").toString()); //返回状态106.0说明已存在,则查询此区域的gid }else if(data3.get("status").toString().equals("106.0")){ pd2.put("status", "1"); }else{ pd2.put("status", "2"); } pd2.put("message", data3.get("message").toString()); return pd2; }
3.更新围栏
/** * 更新围栏;传入围栏名称name;gid;传入围栏坐标点points * @param pd * @return 返回status */ public static PageData xyupdata(PageData pd){ PageData pd2=new PageData(); pd.put("key", "be8e6c802d51a4be29c99be895d8c2c7"); String key=pd.getString("key"); /* String gid=pd.getString("gid");*/ String gid=pd.getString("gid"); pd2.put("repeat", "Mon,Tues,Wed,Thur,Fri,Sat,Sun"); pd2.put("points",pd.getString("points").replace("#", ";")); pd2.put("name", pd.getString("name1")); Gson gson=new Gson(); //传入的参数 String param=gson.toJson(pd2); String url="http://restapi.amap.com/v4/geofence/meta?key=" + key+"&gid=" +gid + "&type=ajaxRequest&method=patch"; String data=HttpUtil.sendPostRequest(url,param); System.out.println(data); PageData data2 = gson.fromJson(data, pd.getClass()); PageData data3 = gson.fromJson(data2.get("data").toString(), pd.getClass()); return data3; }
4.删除围栏
/** * 删除围栏;传入围栏名称gid; * @param pd * @return 返回status */ public static PageData xydel(PageData pd){ PageData pd2=new PageData(); pd.put("key", "be8e6c802d51a4be29c99be895d8c2c7"); String key=pd.getString("key"); /* String gid=pd.getString("gid");*/ String gid=pd.getString("gid"); Gson gson=new Gson(); //传入的参数 String param=gson.toJson(pd2); String url="http://restapi.amap.com/v4/geofence/meta?key=" + key+"&gid=" +gid + "&type=ajaxRequest&method=delete"; String data=HttpUtil.sendPostRequest(url,param); System.out.println(data); PageData data2 = gson.fromJson(data, pd.getClass()); PageData data3 = gson.fromJson(data2.get("data").toString(), pd.getClass()); return data3; }
5.查询一个坐标是否在围栏内;如果需要查询是否在指定的围栏内,需要做进一步处理
/**需要传入参数key;diu(唯一设备号);时间戳;坐标点 *返回 状态status(0表示在范围内;1表示不在范围内) *如果在范围内,则返回围栏列表,带有gid和name * @throws Exception */ public Map<String,Object> xyTest(Map<String,Object> pd) throws Exception{ Map<String,Object> pd2=new HashMap(); //坐标点 String locations =pd.get("locations").toString(); //key String key=pd.get("key").toString(); //设备唯一标识 String diu=pd.get("diu").toString(); //时间戳 String time="1484816232"; Gson gson=new Gson(); String url="http://restapi.amap.com/v4/geofence/status?" + "key="+key + "&diu="+diu + "&locations="+locations+"," + time; //请求返回的参数 String data=HttpUtils.sendPostRequestGet(url); //转为对象便于获取 Map<String,Object> dataJson = gson.fromJson(data, HashMap.class); Map<String, Object> map=(LinkedTreeMap)dataJson.get("data"); //获取围栏事件列表;如果为空说明不在围栏内 if(map.get("fencing_event_list")==null||((List)map.get("fencing_event_list")).size()<1){ pd2.put("status", "1"); return pd2; } //如果不为空,则获取里面的返回值 List<Map<String,Object>> list = (List) map.get("fencing_event_list"); List<Map<String,Object>> list2 = new ArrayList<>(); for(int i=0;i<list.size();i++){ Map<String,Object> pd3=new HashMap(); Map<String,Object> map2 = list.get(i); //如果高德地图api返回的状态为in说明在范围内 if(map2.get("client_status").toString().equals("in")){ //围栏信息 Map<String,Object> map3 =(Map)map2.get("fence_info"); //添加全局围栏gid值; pd3.put("fence_gid",map3.get("fence_gid").toString()); //添加围栏名称 pd3.put("fence_name", map3.get("fence_name").toString()); list2.add(pd3); } } //添加状态值 pd2.put("status", "0"); pd2.put("list", list2); return pd2; }
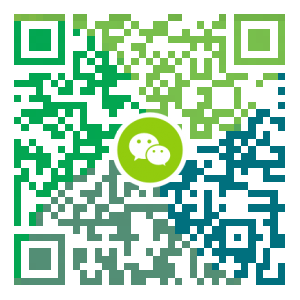
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
spring boot 源码解析2-SpringApplication初始化
前⾔我们⽣成⼀个spring boot 项⽬时,会⾃带⼀个启动类. 代码如下: @SpringBootApplication public class SpringBootAnalysisApplication { public static void main(String[] args) { SpringApplication.run(SpringBootAnalysisApplication.class, args); } } 就是这么简单的代码,构成了spring boot的世界. 那么代码中只有⼀个@SpringBootApplication 注解 和 调⽤了SpringApplication#run⽅法.那么我们先来解析SpringApplication的run⽅法. 解析⾸先调⽤了org.springframework.boot.SpringApplication#run(Object, String...) ⽅法.代码如下: public static ConfigurableApplicationContext run(Object source, String......
- 下一篇
Python语言程序设计学习 之 了解Python
Python简介 Python是一种面向对象的解释型计算机程序设计语言,由荷兰人Guido van Rossum于1989年发明,第一个公开发行版发行于1991年。 Python是纯粹的自由软件,源代码和解释器CPython遵循GPL(GNUGeneral Public License)协议。 Python语法简洁清晰,特色之一是强制用空白符(white space)作为语句缩进。 Python具有丰富和强大的库。它常被昵称为胶水语言,能够把用其他语言制作的各种模块(尤其是C/C++)很轻松地联结在一起。常见的一种应用情形是,使用Python快速生成程序的原型 (有时甚至是程序的最终界面),然后对其中有特别要求的部分,用更合适的语言改写,比如3D游戏中的图形渲染模块,性能要求特别高,就可以用C/C++重写,而后封装为Python可以调用的扩 展类库。需要注意的是在您使用扩展类库时可能需要考虑平台问题,某些可能不提供跨平台的实现。 7月20日,IEEE发布2017年编程语言排行榜:Python高居首位。 2018年3月,该语言作者在邮件列表上宣布 Python 2.7将于2020年1月1...
相关文章
文章评论
共有0条评论来说两句吧...