spring-boot-plus 1.3.1 发布,XSS-CORS-CodeGenerator 优化
[V1.3.1-RELEASE] 2019.10.15
⭐️ New Features
- Xss跨站脚本工具处理
- CORS跨域配置
⚡️ Optimization
- 代码生成器可自定义配置生成哪些文件
- 请求路径filter配置,配置文件属性名称调整
- Aop切点优化,
Aop
JSON参数输出优化 - 可配置是否生成
Validation
验证代码 - 优化
controller
,entity
模版生成 - 优化代码生成器 CodeGenerator
- 调整
aop
,filter
,interceptor
,controller
,param
,vo
代码目录结构
📝 Added/Modified
- Add
XssFilter
,XssHttpServletRequestWrapper
,XssJacksonDeserializer
,XssJacksonSerializer
- Add
SpringBootPlusCorsProperties
- Update
JacksonConfig
- Update
LogAop
,RequestPathFilter
,ShiroConfig
🐞 Bug Fixes
- fix druid控制面板无法访问问题
📔 Documentation
🔨 Dependency Upgrades
- Upgrade to
spring-boot
2.1.9.RELEASE - Upgrade to
Fastjson
1.2.62 - Upgrade to
hutool
4.6.10 - Add
commons-text
1.8
CORS跨域处理
CORS:Cross-Origin Resource Sharing
- CORS是一种允许当前域(domain)的资源(比如html/js/web service)被其他域(domain)的脚本请求访问的机制,通常由于同域安全策略(the same-origin security policy)浏览器会禁止这种跨域请求。
处理方法
- 后台设置允许的请求源/请求头等信息
后台配置
CorsFilter Bean配置
使用
Spring
提供的CorsFilter
过滤器实现跨域配置
io.geekidea.springbootplus.core.config.SpringBootPlusConfig
/** * CORS跨域设置 * * @return */ @Bean public FilterRegistrationBean corsFilter(SpringBootPlusCorsProperties corsProperties) { UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource(); CorsConfiguration corsConfiguration = new CorsConfiguration(); // 跨域配置 corsConfiguration.setAllowedOrigins(corsProperties.getAllowedOrigins()); corsConfiguration.setAllowedHeaders(corsProperties.getAllowedHeaders()); corsConfiguration.setAllowedMethods(corsProperties.getAllowedMethods()); corsConfiguration.setAllowCredentials(corsProperties.isAllowCredentials()); corsConfiguration.setExposedHeaders(corsProperties.getExposedHeaders()); source.registerCorsConfiguration(corsProperties.getPath(), corsConfiguration); FilterRegistrationBean bean = new FilterRegistrationBean(new CorsFilter(source)); bean.setOrder(Ordered.HIGHEST_PRECEDENCE); bean.setEnabled(corsProperties.isEnable()); return bean; }
配置文件
配置文件类:
io.geekidea.springbootplus.core.properties.SpringBootPlusCorsProperties
- application.yml
spring-boot-plus: ############################ CORS start ############################ # CORS跨域配置,默认允许跨域 cors: # 是否启用跨域,默认启用 enable: true # CORS过滤的路径,默认:/** path: /** # 允许访问的源 allowed-origins: '*' # 允许访问的请求头 allowed-headers: x-requested-with,content-type,token # 是否允许发送cookie allow-credentials: true # 允许访问的请求方式 allowed-methods: OPTION,GET,POST # 允许响应的头 exposed-headers: token # 该响应的有效时间默认为30分钟,在有效时间内,浏览器无须为同一请求再次发起预检请求 max-age: 1800 ############################ CORS end ##############################
参考
XSS跨站脚本攻击处理
XSS:Cross Site Scripting
- 跨站脚本攻击(XSS),是目前最普遍的Web应用安全漏洞。这类漏洞能够使得攻击者嵌入恶意脚本代码到正常用户会访问到的页面中,当正常用户访问该页面时,则可导致嵌入的恶意脚本代码的执行,从而达到恶意攻击用户的目的。
处理方法
将参数中的特殊字符进行转换
- 例如 input参数值,用户输入为:
<script>alert(1);</script>
- 处理后为:
<script>alert(1);</script>
后台处理
pom.xml依赖
使用
commons-text
包中的StringEscapeUtils.escapeHtml4();
方法
<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-text</artifactId> <version>1.8</version> </dependency>
XssHttpServletRequestWrapper
对
HttpServletRequest
对象的请求参数进行处理
public class XssHttpServletRequestWrapper extends HttpServletRequestWrapper { public XssHttpServletRequestWrapper(HttpServletRequest request) { super(request); } @Override public String getQueryString() { String value = super.getQueryString(); return StringEscapeUtils.escapeHtml4(value); } @Override public String getParameter(String name) { String value = super.getParameter(name); return StringEscapeUtils.escapeHtml4(value); } @Override public String[] getParameterValues(String name) { String[] values = super.getParameterValues(name); if (ArrayUtils.isEmpty(values)) { return values; } int length = values.length; String[] escapeValues = new String[length]; for (int i = 0; i < length; i++) { String value = values[i]; escapeValues[i] = StringEscapeUtils.escapeHtml4(value); } return escapeValues; } }
XssFilter
使用
WebFilter
注解,拦截所有请求,过滤请求参数
@Slf4j @WebFilter(filterName = "xssFilter", urlPatterns = "/*", asyncSupported = true) public class XssFilter implements Filter { @Override public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException { HttpServletRequest request = (HttpServletRequest) servletRequest; XssHttpServletRequestWrapper xssHttpServletRequestWrapper = new XssHttpServletRequestWrapper(request); filterChain.doFilter(xssHttpServletRequestWrapper, servletResponse); } }
启动类添加@ServletComponentScan注解
扫描使用servlet注解的类,启用 XssFilter
@ServletComponentScan
JSON字符串请求参数处理
实现Jackson反序列化方法,将参数值转义处理
public class XssJacksonDeserializer extends JsonDeserializer<String> { @Override public String deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException, JsonProcessingException { return StringEscapeUtils.escapeHtml4(jsonParser.getText()); } }
JSON字符串响应结果处理
实现Jackson序列化方法,将参数值转义处理
@Slf4j public class XssJacksonSerializer extends JsonSerializer<String> { @Override public void serialize(String s, JsonGenerator jsonGenerator, SerializerProvider serializerProvider) throws IOException { jsonGenerator.writeString(StringEscapeUtils.escapeHtml4(s)); } }
重点,Jackson配置Xss
@Configuration public class JacksonConfig implements WebMvcConfigurer { @Override public void extendMessageConverters(List<HttpMessageConverter<?>> converters) { // code... // XSS序列化 simpleModule.addSerializer(String.class, new XssJacksonSerializer()); simpleModule.addDeserializer(String.class, new XssJacksonDeserializer()); // code... } }
总结
实现字符串转义的核心方法:
org.apache.commons.text.StringEscapeUtils
StringEscapeUtils.escapeHtml4();
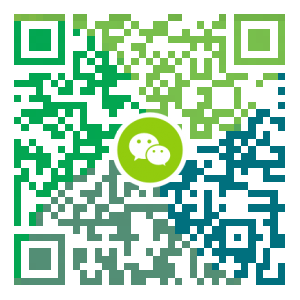
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
SOP 2.4.0 发布,开放平台解决方案项目
SOP 2.4.0 发布,本次发布内容如下: 支持自定义限流持续时间(每n秒允许m个请求,需要执行`sop-2.4.0.sql`升级脚本) 注意:本次升级需要执行sop-2.4.0.sql脚本 在使用窗口限流的情况下,可指定窗口时间,即在n秒内允许m个请求,如下图所示: 关于 SOP SOP(Simple Open Platform) 一个开放平台解决方案项目,基于Spring Cloud实现,目标是能够让用户快速得搭建起自己的开放平台。 SOP提供了两种接口调用方式,分别是:支付宝开放平台的调用方式和淘宝开放平台的调用方式。 通过简单的配置后,你的项目就具备了和支付宝开放平台的一样的接口提供能力。 SOP封装了开放平台大部分功能包括:签名验证、统一异常处理、统一返回内容 、业务参数验证(JSR-303)、秘钥管理等,未来还会实现更多功能。 项目特点 接入方式简单,与老项目不冲突,老项目注册到注册中心,然后在方法上加上注解即可。 架构松耦合,业务代码实现在各自微服务上,SOP不参与业务实现,这也是Spring Cloud微服务体系带来的好处。 扩展简单,开放平台对应的功能各自独立,可...
- 下一篇
SpringBoot 增强库 yue-library 更新,提供模板项目
yue-library yue-library 是一个基于 SpringBoot 封装的基础库 内置丰富的 JDK 工具 自动装配了一系列的基础 Bean 与环境配置项 快速构建 SpringCloud 项目,让微服务变得更简单 收藏一波以表支持吧(≧▽≦)/!本次更新主要完善注释与文档,提供 SpringBoot 模板项目,优化与修复 BUG 为主。接下来计划添加 oss、sms 等模块。 点击查看完整的版本更新日志 Greenwich.SR2.1【2019-10-15】 新特性 完善包注释与类注释,提供更舒爽的 javadoc,完善项目文档,提供更多的代码示例与使用说明。同时优化项目部分代码结构与紧急修复 redis User 类 合并统一异常处理类继承结构,更改redis常量配置属性为可配置属性 【base】增强字段校验器Validator类型自动识别与错误参数提示,更加强大方便好用 【base】Result新增方法public <D> List<D> dataToList(Class<D> clazz) { 【base】Result新增方法p...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS关闭SELinux安全模块
- SpringBoot2更换Tomcat为Jetty,小型站点的福音
- CentOS7安装Docker,走上虚拟化容器引擎之路
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- CentOS7编译安装Cmake3.16.3,解决mysql等软件编译问题
- SpringBoot2整合Thymeleaf,官方推荐html解决方案
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- CentOS8编译安装MySQL8.0.19
- CentOS7,8上快速安装Gitea,搭建Git服务器
- Hadoop3单机部署,实现最简伪集群