iOS开发-Masonry简易教程
关于iOS布局自动iPhone6之后就是AutoLayOut,AutoLayOut固然非常好用,不过有时候我们需要在页面手动进行页面布局,VFL算是一种选择,如果对VFL不是很熟悉可以参考iOS开发-VFL(Visual format language)和Autolayout。VFL不复杂,理解起来很容易,实际开发中用的特别熟还好,要是第一次看估计要花点功夫才能搞定。Masonry算是VFL的简化版,用的人比较多,之前项目中用过一次,对手动写页面的开发来说算是福利。
基础知识
首先我们看一个常见的问题将一个子View放在的UIViewController的某个位置,通过设置边距来实现,效果如下:
如果通过VFL我们代码会是这样的:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 | UIView *superview = self.view; UIView *view1 = [[UIView alloc] init]; view1.translatesAutoresizingMaskIntoConstraints = NO; view1.backgroundColor = [UIColor redColor]; [superview addSubview:view1]; UIEdgeInsets padding = UIEdgeInsetsMake(200, 50, 200, 50); [superview addConstraints:@[ //约束 [NSLayoutConstraint constraintWithItem:view1 attribute:NSLayoutAttributeTop relatedBy:NSLayoutRelationEqual toItem:superview attribute:NSLayoutAttributeTop multiplier:1.0 constant:padding.top], [NSLayoutConstraint constraintWithItem:view1 attribute:NSLayoutAttributeLeft relatedBy:NSLayoutRelationEqual toItem:superview attribute:NSLayoutAttributeLeft multiplier:1.0 constant:padding.left], [NSLayoutConstraint constraintWithItem:view1 attribute:NSLayoutAttributeBottom relatedBy:NSLayoutRelationEqual toItem:superview attribute:NSLayoutAttributeBottom multiplier:1.0 constant:-padding.bottom], [NSLayoutConstraint constraintWithItem:view1 attribute:NSLayoutAttributeRight relatedBy:NSLayoutRelationEqual toItem:superview attribute:NSLayoutAttributeRight multiplier:1 constant:-padding.right], ]]; |
只是简单的设置了一个边距,如果视图的关系比较复杂,维护起来会是一个很痛苦的事情,我们看一下Masonry是如何实现的,导入Masonry.h头文件,约束的代码:
1 2 3 4 5 6 7 8 9 | UIView *childView=[UIView new ]; [childView setBackgroundColor:[UIColor redColor]]; //先将子View加入在父视图中 [self.view addSubview:childView]; __weak typeof (self) weakSelf = self; UIEdgeInsets padding = UIEdgeInsetsMake(200, 50, 200, 50); [childView mas_makeConstraints:^(MASConstraintMaker *make) { make.edges.equalTo(weakSelf.view).with.insets(padding); }]; |
通过mas_makeConstraints设置边距有种鸟枪换炮的感觉,我们即将开启一段新的旅程,可以紧接着看下面比较实用的功能~
实用知识
1.View设置大小
1 2 3 4 5 6 7 8 9 10 11 | UIView *childView=[UIView new ]; [childView setBackgroundColor:[UIColor redColor]]; //先将子View加入在父视图中 [self.view addSubview:childView]; __weak typeof (self) weakSelf = self; [childView mas_makeConstraints:^(MASConstraintMaker *make) { //设置大小 make.size.mas_equalTo(CGSizeMake(100, 100)); //居中 make.center.equalTo(weakSelf.view); }]; |
效果如下:
这里友情其实一个小内容,目前我们设置约束都是通过mas_makeConstraints用来创建约束,mas_updateConstraints用来更新约束,mas_remakeConstraints重置约束,清除之前的约束,保留最新的约束,如果想深入解释下,可以阅读下面的英文解释~
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | /** * Creates a MASConstraintMaker with the callee view. * Any constraints defined are added to the view or the appropriate superview once the block has finished executing * * @param block scope within which you can build up the constraints which you wish to apply to the view. * * @return Array of created MASConstraints */ - (NSArray *)mas_makeConstraints:( void (^)(MASConstraintMaker *make))block; /** * Creates a MASConstraintMaker with the callee view. * Any constraints defined are added to the view or the appropriate superview once the block has finished executing. * If an existing constraint exists then it will be updated instead. * * @param block scope within which you can build up the constraints which you wish to apply to the view. * * @return Array of created/updated MASConstraints */ - (NSArray *)mas_updateConstraints:( void (^)(MASConstraintMaker *make))block; /** * Creates a MASConstraintMaker with the callee view. * Any constraints defined are added to the view or the appropriate superview once the block has finished executing. * All constraints previously installed for the view will be removed. * * @param block scope within which you can build up the constraints which you wish to apply to the view. * * @return Array of created/updated MASConstraints */ - (NSArray *)mas_remakeConstraints:( void (^)(MASConstraintMaker *make))block; |
2.设置高度,这里设置左右边距,因此不设置宽度,如果想单独设置width可参考高度的设置方式:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | UIView *childView=[UIView new ]; [childView setBackgroundColor:[UIColor greenColor]]; //先将子View加入在父视图中 [self.view addSubview:childView]; __weak typeof (self) weakSelf = self; [childView mas_makeConstraints:^(MASConstraintMaker *make) { //距离顶部44 make.top.equalTo(weakSelf.view.mas_top).with.offset(44); //距离左边30 make.left.equalTo(weakSelf.view.mas_left).with.offset(30); //距离右边30,注意是负数 make.right.equalTo(weakSelf.view.mas_right).with.offset(-30); //高度150 make.height.mas_equalTo(@150); }]; |
3.子视图之间的位置设置:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | UIView *childView=[UIView new ]; [childView setBackgroundColor:[UIColor greenColor]]; //先将子View加入在父视图中 [self.view addSubview:childView]; __weak typeof (self) weakSelf = self; [childView mas_makeConstraints:^(MASConstraintMaker *make) { //距离顶部44 make.top.equalTo(weakSelf.view.mas_top).with.offset(44); //距离左边30 make.left.equalTo(weakSelf.view.mas_left).with.offset(30); //距离右边30,注意是负数 make.right.equalTo(weakSelf.view.mas_right).with.offset(-30); //高度150 make.height.mas_equalTo(@150); }]; //地址:http://www.cnblogs.com/xiaofeixiang/ UIView *nextView=[UIView new ]; [nextView setBackgroundColor:[UIColor redColor]]; [self.view addSubview:nextView]; [nextView mas_makeConstraints:^(MASConstraintMaker *make) { make.top.equalTo(childView.mas_bottom).with.offset(30); make.right.equalTo(childView.mas_right).with.offset(-30); make.width.mas_equalTo(@100); make.height.mas_equalTo(@100); }]; |
4.链式写法,算是一个便利的写法:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | UIView *childView=[UIView new ]; [childView setBackgroundColor:[UIColor greenColor]]; //先将子View加入在父视图中 [self.view addSubview:childView]; __weak typeof (self) weakSelf = self; [childView mas_makeConstraints:^(MASConstraintMaker *make) { make.top.and.left.mas_equalTo(weakSelf.view).with.offset(100); make.bottom.and.right.mas_equalTo(weakSelf.view).with.offset(-100); //第二种写法更简单,相对于就是父视图 // make.top.and.left.mas_equalTo(100); // make.bottom.and.right.mas_equalTo(-100); }]; UILabel *label=[UILabel new ]; [label setText: @"博客园-FlyElephant" ]; [label setTextColor:[UIColor redColor]]; [label setTextAlignment:NSTextAlignmentCenter]; [self.view addSubview:label]; [label mas_makeConstraints:^(MASConstraintMaker *make) { make.left.mas_equalTo(weakSelf.view).with.offset(10); make.height.mas_equalTo(20); make.right.mas_equalTo(weakSelf.view).with.offset(-10); make.bottom.mas_equalTo(weakSelf.view).with.offset(-50); }]; |
网上关于Masonry的教程很多,给的例子的也很多,这几种情况基本上满足了开发中的需求,不会有太多的出入,算是一个简易版的教程,Masonry的中属性和iOS中的属性是有对应的关系,不过因为很简单,基本上没怎么看,下图是一个对照关系:
本文转自Fly_Elephant博客园博客,原文链接:http://www.cnblogs.com/xiaofeixiang/p/5127825.html,如需转载请自行联系原作者
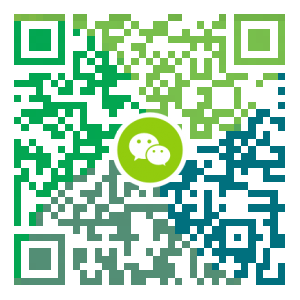
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
各种语言的注释总结--from wiki
Commentscan be classified by: style (inline/block) parse rules (ignored/interpolated/stored in memory) recursivity (nestable/non-nestable) uses (docstrings/throwaway comments/other) Inline comments[edit] Inline comments are generally those that use anewlinecharacter to indicate the end of a comment, and an arbitrarydelimiteror sequence oftokensto indicate the beginning of a comment. Examples: Symbol Languages C Fortran 77and earlier; the 'C' must be in column 1 of a line to indicate a comment. R...
- 下一篇
iOS开发-xCode代码格式化xAlign
xCode默认是可以进行代码格式化的,能满足基础开发需求,如果想要个性一些代码对齐方式宏对齐,等号对齐,属性对齐,xAlign就提供了以上三种功能,参考文中效果~ 基础效果 等号对齐: 属性对齐: 宏对齐: 插件安装 1.控制台命令 1 curl -fsSL http: //qfi.sh/XAlign/build/install.sh | sh 2.手动安装下载插件包:XAlign.xcplugin,将下载的插件放在~/Library/Application Support/Developer/Shared/Xcode/Plug-ins/目录下,Finder路径寻找快捷键command + shift + G; 3.重启xCode,加载xAlign; 4.卸载 1 curl -fsSL http: //qfi.sh/XAlign/build/uninstall.sh | sh 手动安装的直接到第二步~/Library/Application Support/Developer/Shared/Xcode/Plug-ins/删除插件即可~ 5.通过xCode→Edit→XAlign中的选项...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Hadoop3单机部署,实现最简伪集群
- SpringBoot2更换Tomcat为Jetty,小型站点的福音
- Windows10,CentOS7,CentOS8安装Nodejs环境
- CentOS7编译安装Cmake3.16.3,解决mysql等软件编译问题
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- 设置Eclipse缩进为4个空格,增强代码规范
- SpringBoot2全家桶,快速入门学习开发网站教程
- CentOS关闭SELinux安全模块
- CentOS7,8上快速安装Gitea,搭建Git服务器
- CentOS7,CentOS8安装Elasticsearch6.8.6