jsp--MVC案例
三层优化
1.加入接口
建议面向接口开发:先接口-再实现类
–service、dao加入接口
–接口与实现类的命名规范
接口:interface, 起名 I实体类Service IStudentService
IStudentDao
实现类:implements 起名 实体类ServiceImpl StudentServiceImpl
StudentDaoImpl
接口: I实体类层所在包名 IStudentService、IStudentDao
接口所在的包: xxx.service xx.dao
实现类: 实体类层所在包名Impl StudentServiceImpl、StudentDaoImpl 实现类所在的包:xxx.service.impl xx.dao.impl 以后使用接口/实现类时,推荐写法: 接口 x = new 实现类(); IStudentDao studentDao = new StudentDaoImpl();
案例:实现用户登录验证
login.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>登录</title> </head> <body> <form action="LoginServlet" method="post"> 用户名:<input type="text" name="uname"><br> 密码:<input type="password" name="upwd"><br> <input type="submit" value="登录"> </form> </body> </html>
welcome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Insert title here</title> </head> <body> 登录成功! </body> </html>
com.xdr.entity–Login.java
package com.xdr.entity; public class Login { private int id; private String uname; private String upwd; public Login() { } public Login(String uname, String upwd) { super(); this.id = id; this.uname = uname; this.upwd = upwd; } public Login(int id, String uname, String upwd) { super(); this.id = id; this.uname = uname; this.upwd = upwd; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getUname() { return uname; } public void setUname(String uname) { this.uname = uname; } public String getUpwd() { return upwd; } public void setUpwd(String upwd) { this.upwd = upwd; } }
com.xdr.dao–loginDao.java
package com.xdr.dao; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import com.xdr.entity.Login; //模型层:用于处理登录(查询数据库) public class LoginDao { public static int login(Login login) {//登录 //boolean flag = false;//登录成功与否标识 int flag = -1; //-1系统异常 ,0用户名或密码有误 1登陆成功 int result = -1; Connection connection = null; PreparedStatement pstmt = null; ResultSet rs = null; try { Class.forName("com.mysql.jdbc.Driver"); connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/jsp01","root","123456"); String sql = "select count(*) from login where uname=? and upwd=?"; pstmt = connection.prepareStatement(sql); pstmt.setString(1, login.getUname()); pstmt.setString(2, login.getUpwd()); rs = pstmt.executeQuery(); if(rs.next()) { result = rs.getInt(1); } if(result>0) { return 1; }else { return 0;//登录失败(用户名或密码有误!) } }catch(ClassNotFoundException e) { e.printStackTrace(); return -1;//登录失败(系统异常!) }catch(SQLException e) { e.printStackTrace(); return -1; }catch(Exception e) { e.printStackTrace(); return -1; }finally { try { if(rs!=null) rs.close(); if(pstmt!=null) pstmt.close(); if(connection!=null) connection.close(); }catch(SQLException e) { e.printStackTrace(); }catch(Exception e) { e.printStackTrace(); } } } }
com.xdr.servlet–LoginServlet.java
package com.xdr.servlet; import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import com.xdr.dao.LoginDao; import com.xdr.entity.Login; //控制层:接受view请求,并分发给model public class LoginServlet extends HttpServlet { protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //处理登录 request.setCharacterEncoding("utf-8"); String name = request.getParameter("uname"); String pwd = request.getParameter("upwd"); Login login = new Login(name,pwd);//用户名,密码 //调用陪你过模型层的 登录功能 int result = LoginDao.login(login); if(result>0) {//成功 response.sendRedirect("welcome.jsp"); }else {//登录失败 response.sendRedirect("login.jsp"); } } protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // TODO Auto-generated method stub doGet(request, response); } }
web.xml配置添加,就能直接从根目录下访问项目了
数据库信息如下:
输入正确的用户信息后:
输入错误的信息会重新跳转到登录面:
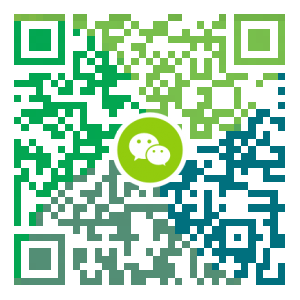
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
垃圾回收机制GC
总结如下: 引用计数 x = 18 #18被引用了一次,计数为1 y = x #18 被引用了两次,计数为2 循环引用 ?循环引用----->存在着内存泄漏的漏洞, 需要清除标记 分代回收 y = 20 #18的引用减1,计数为1 del x #18的引用减1,计数为0 详解部分 引入 解释器在执行到定义变量的语法时,而内存空间是有限的,而内存的容量是有限的,这就涉及到变量值所占用内存空间的回收问题,当一个变量值没有用了(简称垃圾)就应该将其占用的内存给回收掉,那什么样的变量值是没有用的呢? 单从逻辑层面分析,我们定义变量将变量值存起来的目的是为了以后取出来使用,而取得变量值需要通过其绑定的直接引用(如x=10,10被x直接引用)或间接引用(如l=[x,],x=10,10被x直接引用,而被容器类型l间接引用),所以当一个变量值不再绑定任何引用时,我们就无法再访问到该变量值了,该变量值自然就是没有用的,就应该被当成一个垃圾回收。 毫无疑问,内存空间的申请与回收都是非常耗费精力的事情,而且存在很大的危险性,稍有不慎就有可能引发内存溢出问题,好在Cpython解释器提供了自动的垃圾...
- 下一篇
手把手搭建Java共享网盘
在线共享网盘采用jsp+servlet搭建项目结构实现共享网盘,项目分为管理员,普通用户和付费用户三种角色,根据不同角色控制不同权限,实现不同用户对个人文件文件,所有文件,共享文件的增删改查操作。 项目介绍 在线共享网盘采用jsp+servlet搭建项目结构实现共享网盘,项目分为管理员,普通用户和付费用户三种角色,根据不同角色控制不同权限,实现不同用户对个人文件文件,所有文件,共享文件的增删改查操作。 项目适用人群 正在做毕设的学生,或者需要项目实战练习的Java学习者 开发环境: jdk 8 intellij idea tomcat 8.5.40 mysql 5.7 所用技术: jsp+servlet js+ajax layUi jdbc直连 项目访问地址 http://localhost:8090 项目结构 项目截图 注册 我的网盘 我的共享 回收站 会员充值 管理员-所有文件 管理员-共享申请 关键代码: 1.初始化工作 //数据库连接初始化 publicclassDBInfo{ Stringurl=null; Stringusername=null; Stringpasswor...
相关文章
文章评论
共有0条评论来说两句吧...