[雪峰磁针石博客]python 3.7极速入门教程5循环
5循环
语法基础
for语句
Python的for语句针对序列(列表或字符串等)中的子项进行循环,按它们在序列中的顺序来进行迭代。
>>> # Measure some strings: ... words = ['cat', 'window', 'defenestrate'] >>> for w in words: ... print(w, len(w)) ... cat 3 window 6 defenestrate 12
在迭代过程中修改迭代序列不安全,可能导致部分元素重复两次,建议先拷贝:
>>> for w in words[:]: # Loop over a slice copy of the entire list. ... if len(w) > 6: ... words.insert(0, w) ... >>> words ['defenestrate', 'cat', 'window', 'defenestrate']
range()函数
内置函数 range()生成等差数值序列:
>>> range(10) [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
range(10) 生成了一个包含10个值的链表,但是不包含最右边的值。默认从0开始,也可以让range 从其他值开始,或者指定不同的增量值(甚至是负数,有时也称"步长"):
>>> range(5, 10) [5, 6, 7, 8, 9] >>> range(0, 10, 3) [0, 3, 6, 9] >>> range(-10, -100, -30) [-10, -40, -70] >>> range(-10, -100, 30) []
如果迭代时需要索引和值可结合使用range()和len():
>>> a = ['Mary', 'had', 'a', 'little', 'lamb'] >>> for i in range(len(a)): ... print(i, a[i]) ... 0 Mary 1 had 2 a 3 little 4 lamb
不过使用enumerate()更方便,参见后面的介绍。
break和continue语句及循环中的else子句
break语句和C中的类似,用于终止当前的for或while循环。
循环可能有else 子句;它在循环迭代完整个列表(对于 for)后或执行条件为false(对于 while)时执行,但循环break时不会执行。这点和try...else而不是if...else相近。请看查找素数的程序:
>>> for n in range(2, 10): ... for x in range(2, n): ... if n % x == 0: ... print(n, 'equals', x, '*', n//x) ... break ... else: ... # loop fell through without finding a factor ... print(n, 'is a prime number') ... 2 is a prime number 3 is a prime number 4 equals 2 * 2 5 is a prime number 6 equals 2 * 3 7 is a prime number 8 equals 2 * 4 9 equals 3 * 3
continue语句也是从C而来,它表示退出当次循环,继续执行下次迭代。通常可以用if...else替代,请看查找偶数的实例:
>>> for n in range(2, 10): ... for x in range(2, n): ... if n % x == 0: ... print(n, 'equals', x, '*', n//x) ... break ... else: ... # loop fell through without finding a factor ... print(n, 'is a prime number') ... 2 is a prime number 3 is a prime number 4 equals 2 * 2 5 is a prime number 6 equals 2 * 3 7 is a prime number 8 equals 2 * 4 9 equals 3 * 3
pass
pass语句什么也不做。它语法上需要,但是实际什么也不做场合,也常用语以后预留以后扩展。例如:
>>> while True: ... pass # Busy-wait for keyboard interrupt (Ctrl+C) ... >>> class MyEmptyClass: ... pass ... >>> def initlog(*args): ... pass # Remember to implement this! ...
循环技巧
在字典中循环时,关键字和对应的值可以使用 items() 方法同时获取:
>>> knights = {'gallahad': 'the pure', 'robin': 'the brave'} >>> for k, v in knights.items(): ... print(k, v) ... gallahad the pure robin the brave
在序列中循环时 enumerate() 函数同时得到索引位置和对应值:
>>> for i, v in enumerate(['tic', 'tac', 'toe']): ... print(i, v) ... 0 tic 1 tac 2 toe
同时循环两个或更多的序列,可以使用 zip() 打包:
>>> questions = ['name', 'quest', 'favorite color'] >>> answers = ['lancelot', 'the holy grail', 'blue'] >>> for q, a in zip(questions, answers): ... print('What is your {0}? It is {1}.'.format(q, a)) ... What is your name? It is lancelot. What is your quest? It is the holy grail. What is your favorite color? It is blue.
需要逆向循环序列的话,调用 reversed() 函数即可:
>>> for i in reversed(xrange(1, 10, 2)): ... print(i) ... 9 7 5 3 1
使用 sorted() 函数可排序序列,它不改动原序列,而是生成新的已排序的序列:
>>> basket = ['apple', 'orange', 'apple', 'pear', 'orange', 'banana'] >>> for f in sorted(set(basket)): ... print f ... apple banana orange pear
若要在循环时修改迭代的序列,建议先复制。
>>> import math >>> raw_data = [56.2, float('NaN'), 51.7, 55.3, 52.5, float('NaN'), 47.8] >>> filtered_data = [] >>> for value in raw_data: ... if not math.isnan(value): ... filtered_data.append(value) ... >>> filtered_data [56.2, 51.7, 55.3, 52.5, 47.8]
循环
代码:
# -*- coding: utf-8 -*- # Author: xurongzhong#126.com wechat:pythontesting qq:37391319 # 技术支持 钉钉群:21745728(可以加钉钉pythontesting邀请加入) # qq群:144081101 591302926 567351477 # CreateDate: 2018-6-12 # bowtie.py # Draw a bowtie from turtle import * def polygon(n, length): """Draw n-sided polygon with given side length.""" for _ in range(n): forward(length) left(360/n) def main(): """Draw polygons with 3-9 sides.""" for n in range(3, 10): polygon(n, 80) exitonclick() main()
参考资料
- 讨论qq群144081101 591302926 567351477 钉钉免费群21745728
- 本文最新版本地址
- 本文涉及的python测试开发库 谢谢点赞!
- 本文相关海量书籍下载
- 本文源码地址
条件循环while
代码:
# -*- coding: utf-8 -*- # Author: xurongzhong#126.com wechat:pythontesting qq:37391319 # 技术支持 钉钉群:21745728(可以加钉钉pythontesting邀请加入) # qq群:144081101 591302926 567351477 # CreateDate: 2018-6-12 # spiral.py # Draw spiral shapes from turtle import * def spiral(firststep, angle, gap): """Move turtle on a spiral path.""" step = firststep while step > 0: forward(step) left(angle) step -= gap def main(): spiral(100, 71, 2) exitonclick() main()
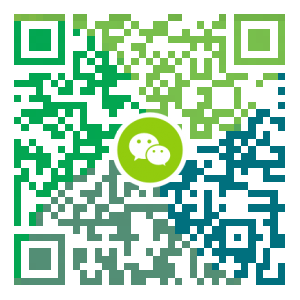
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
[雪峰磁针石博客]使用jython进行dubbo接口及ngrinder性能测试
快速入门(接口测试) 确认mvn和jdk、jython安装ok。 先下载dubbo的demo,编译运行demo。 # git clone https://github.com/alibaba/dubbo.git dubbo # cd dubbo/ # mvn clean install -Dmaven.test.skip # cd dubbo-demo/dubbo-demo-provider/target/ # tar xzvf dubbo-demo-provider-2.5.4-SNAPSHOT-assembly.tar.gz # cd dubbo-demo-provider-2.5.4-SNAPSHOT/bin # ./start.sh # cd /opt/code/dubbo-demo/dubbo-demo-consumer/target/ # tar xzvf dubbo-demo-provider-2.5.4-SNAPSHOT-assembly.tar.gz # cd dubbo-demo-consumer-2.5.4-SNAPSHOT/bin # ./start.sh 注意...
- 下一篇
[雪峰磁针石博客]计算机视觉opcencv工具深度学习快速实战2 opencv快速入门
opencv基本操作 # -*- coding: utf-8 -*- # Author: xurongzhong#126.com wechat:pythontesting qq:37391319 # 技术支持 钉钉群:21745728(可以加钉钉pythontesting邀请加入) # qq群:144081101 591302926 567351477 # CreateDate: 2018-11-17 import imutils import cv2 # 读取图片信息 image = cv2.imread("jp.png") (h, w, d) = image.shape print("width={}, height={}, depth={}".format(w, h, d)) # 显示图片 cv2.imshow("Image", image) cv2.waitKey(0) # 访问像素 (B, G, R) = image[100, 50] print("R={}, G={}, B={}".format(R, G, B)) # 选取图片区间 ROI (Region of Inter...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Hadoop3单机部署,实现最简伪集群
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- SpringBoot2整合Redis,开启缓存,提高访问速度
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- Eclipse初始化配置,告别卡顿、闪退、编译时间过长
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- CentOS7,8上快速安装Gitea,搭建Git服务器
- CentOS8安装MyCat,轻松搞定数据库的读写分离、垂直分库、水平分库