python-进程池与线程池,协程
一、进程池与线程池
实现并发的手段有两种,多线程和多进程。注:并发是指多个任务看起来是同时运行的。主要是切换+保存状态。
当我们需要执行的并发任务大于cpu的核数时,我们需要知道一个操作系统不能无限的开启进程和线程,通常有几个核就开几个进程,如果进程开启过多,就无法充分利用cpu多核的优势,效率反而会下降。这个时候就引入了进程池线程池的概念。
池的功能就是限制启动的进程数或线程数
concurent.future模块:
concurrent.futures模块提供了高度封装的异步调用接口
ProcessPoolExecutor: 进程池,提供异步调用
p = ProcessPoolExecutor(max_works)对于进程池如果不写max_works:默认的是cpu的数目,默认是4个
ThreadPoolExecutor:线程池,提供异步调用
p = ThreadPoolExecutor(max_works)对于线程池如果不写max_works:默认的是cpu的数目*5
补充:
提交任务的两种方式:
# 同步调用:提交完一个任务之后,就在原地等待,等待任务完完整整地运行完毕拿到结果后,再执行下一行代码,会导致任务是串行执行的
# 异步调用:提交完一个任务之后,不在原地等待,结果???,而是直接执行下一行代码,会导致任务是并发执行的
进程池从无到有创建进程后,然后会固定使用进程池里创建好的进程去执行所有任务,不会开启其他进程
# 基本方法 #submit(fn, *args, **kwargs) 异步提交任务 #map(func, *iterables, timeout=None, chunksize=1) 取代for循环submit的操作 #shutdown(wait=True) 相当于进程池的pool.close()+pool.join()操作 wait=True,等待池内所有任务执行完毕回收完资源后才继续 wait=False,立即返回,并不会等待池内的任务执行完毕 但不管wait参数为何值,整个程序都会等到所有任务执行完毕 submit和map必须在shutdown之前 #result(timeout=None) 取得结果 #add_done_callback(fn) 回调函数
from concurrent.futures import ThreadPoolExecutor,ProcessPoolExecutor import time,random,os import requests def get(url): print('%s GET %s' %(os.getpid(),url)) time.sleep(3) response=requests.get(url) if response.status_code == 200: res=response.text else: res='下载失败' return res def parse(future): time.sleep(1) res=future.result() print('%s 解析结果为%s' %(os.getpid(),len(res))) if __name__ == '__main__': urls=[ 'https://www.baidu.com', 'https://www.sina.com.cn', 'https://www.tmall.com', 'https://www.jd.com', 'https://www.python.org', 'https://www.openstack.org', 'https://www.baidu.com', 'https://www.baidu.com', 'https://www.baidu.com', ] p=ProcessPoolExecutor(9) start=time.time() for url in urls: future=p.submit(get,url) # 异步调用:提交完一个任务之后,不在原地等待,而是直接执行下一行代码,会导致任务是并发执行的,,结果futrue对象会在任务运行完毕后自动传给回调函数 future.add_done_callback(parse) #parse会在任务运行完毕后自动触发,然后接收一个参数future对象 p.shutdown(wait=True) print('主',time.time()-start) print('主',os.getpid())
线程池与进程池相比 他们的同步执行和异步执行是一样的:
from concurrent.futures import ThreadPoolExecutor,ProcessPoolExecutor from threading import current_thread import time,random,os import requests def get(url): print('%s GET %s' %(current_thread().name,url)) time.sleep(3) response=requests.get(url) if response.status_code == 200: res=response.text else: res='下载失败' return res def parse(future): time.sleep(1) res=future.result() print('%s 解析结果为%s' %(current_thread().name,len(res))) if __name__ == '__main__': urls=[ 'https://www.baidu.com', 'https://www.sina.com.cn', 'https://www.tmall.com', 'https://www.jd.com', 'https://www.python.org', 'https://www.openstack.org', 'https://www.baidu.com', 'https://www.baidu.com', 'https://www.baidu.com', ] p=ThreadPoolExecutor(4) for url in urls: future=p.submit(get,url) future.add_done_callback(parse) p.shutdown(wait=True) print('主',current_thread().name)
map函数:
# 我们的那个p.submit(task,i)和map函数的原理类似。我们就 # 可以用map函数去代替。更减缩了代码 from concurrent.futures import ProcessPoolExecutor, ThreadPoolExecutor import os, time, random def task(n): print('[%s] is running' % os.getpid()) time.sleep(random.randint(1, 3)) # I/O密集型的,,一般用线程,用了进程耗时长 return n ** 2 if __name__ == '__main__': p = ProcessPoolExecutor() obj = p.map(task, range(10)) p.shutdown() # 相当于close和join方法 print('=' * 30) print(obj) # 返回的是一个迭代器 print(list(obj))
回调函数(知乎):https://www.zhihu.com/question/19801131/answer/27459821
二、协程
在单线程的情况下实现并发。
遇到IO就切换就可以降低单线程的IO时间,从而最大限度地提升单线程的效率。
实现并发是让多个任务看起来同时运行(切换+保存状态),cpu在运行一个任务的时候,会在两种情况下去执行其他的任务,一种是遇到了I/O操作,一种是计算时间过长。其中第二种情况使用线程并发并不能提升效率,运算密集型的并发反而会降低效率。
#串行执行 import time def func1(): for i in range(10000000): i+1 def func2(): for i in range(10000000): i+1 start = time.time() func1() func2() stop = time.time() print(stop - start)#1.675490379333496
#基于yield并发执行 import time def func1(): while True: print('func1') 100000+1 yield def func2(): g=func1() for i in range(10000000): print('func2') time.sleep(100) i+1 next(g) start=time.time() func2() stop=time.time() print(stop-start)
yield复习:
函数中只有有yield,这个函数就变成了一个生成器,调用函数不会执行函数体代码,会得到一个返回值,返回值就是生成器对象。
def yield_test(n): for i in range(n): yield call(i) print("i=",i) #做一些其它的事情 print("do something.") print("end.") def call(i): return i*2 #使用for循环 for i in yield_test(5): print(i,",")
协程的本质就是在单线程下,由用户自己控制一个任务遇到IO操作就切换到另一个任务去执行,以此来提升效率。
Gevent:
gevent是第三方库,通过greenlet实现协程,其基本思想是:
当一个greenlet遇到IO操作时,比如访问网络,就自动切换到其他的greenlet,等到IO操作完成,再在适当的时候切换回来继续执行。由于IO操作非常耗时,经常使程序处于等待状态,有了gevent为我们自动切换协程,就保证总有greenlet在运行,而不是等待IO。
由于切换是在IO操作时自动完成,所以gevent需要修改Python自带的一些标准库,这一过程在启动时通过monkey patch完成:
我们用等待的时间模拟IO阻塞 在gevent模块里面要用gevent.sleep(5)表示等待的时间 要是我们想用time.sleep(),就要在最上面导入from gevent import monkey;monkey.patch_all()这句话 如果不导入直接用time.sleep(),就实现不了单线程并发的效果了
注:猴子补丁需要在第一行就运行
from gevent import monkey;monkey.patch_all() from gevent import spawn,joinall #pip3 install gevent import time def play(name): print('%s play 1' %name) time.sleep(5) print('%s play 2' %name) def eat(name): print('%s eat 1' %name) time.sleep(3) print('%s eat 2' %name) start=time.time() g1=spawn(play,'lxx') g2=spawn(eat,'lxx') # g1.join() # g2.join() joinall([g1,g2]) print('主',time.time()-start)
gevent.spawn()”方法会创建一个新的greenlet协程对象,并运行它。”gevent.joinall()”方法会等待所有传入的greenlet协程运行结束后再退出,这个方法可以接受一个”timeout”参数来设置超时时间,单位是秒。
在单线程内实现socket并发:
from gevent import monkey;monkey.patch_all() from socket import * from gevent import spawn def comunicate(conn): while True: # 通信循环 try: data = conn.recv(1024) if len(data) == 0: break conn.send(data.upper()) except ConnectionResetError: break conn.close() def server(ip, port, backlog=5): server = socket(AF_INET, SOCK_STREAM) server.bind((ip, port)) server.listen(backlog) while True: # 链接循环 conn, client_addr = server.accept() print(client_addr) # 通信 spawn(comunicate,conn) if __name__ == '__main__': g1=spawn(server,'127.0.0.1',8080) g1.join()
from threading import Thread,current_thread from socket import * def client(): client=socket(AF_INET,SOCK_STREAM) client.connect(('127.0.0.1',8080)) n=0 while True: msg='%s say hello %s' %(current_thread().name,n) n+=1 client.send(msg.encode('utf-8')) data=client.recv(1024) print(data.decode('utf-8')) if __name__ == '__main__': for i in range(500): t=Thread(target=client) t.start()
焚膏油以继晷,恒兀兀以穷年。
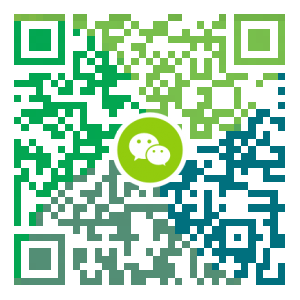
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
第七章 异常, 断言和日志
处理错误 出现错误时程序应该(1)返回安全状态并允许用户执行一些其他命令; (2)或者允许用户保存所有操作的结果,并以妥善方式退出程序; 错误产生的原因:(1)用户输入错误;(2)设备错误;(3)物理限制;(4)代码错误: 有些情况下代码的调用方并不能根据程序的错误返回码进行处理, 这时就需要抛出异常给异常处理器去处理; 应用程序不应该抛出Error级别的错误, 出现这种错误一般时JVM或者资源耗尽, 除了通知用户并中止程序外,无法再做更多; Exception 的子类 RuntimeException 表示异常时由程序错误导致, 如果出现RuntimeException异常, 程序员就一定有责任去处理杜绝它. 派生于Error 和 RuntimeException的异常被称为unchecked异常, 其他为checked异常. (checked翻译为受查) 下面四种情况下应该抛出异常: 1. 调用了抛出异常的方法; 2. 程序throw出一个异常; 3. 程序出错; 4. JVM或运行时库报错 对于给外部调用的方法, 有义务在方法上声明throws异常(checked)类型, 但不包...
- 下一篇
Python全栈 Web(JavaScript 运算符、流程控制)
运算符: 赋值运算符:= 算术运算符:+ - * / % 自增自减:++ -- 自增自减在单独使用时放前放后没区别 如果参与其他运算时分前缀和后缀 ++ 在后 先使用后自增 ++ 在前 先自增后使用 关系运算符 >、 >=、 <、 <=、 ==、 != ===全等/恒等 !==不全等/不恒等 关系运算符的结果都为boolean类型 数字与字符串进行比较: 实现自动转换数据类型在比较 如果转换成功 按照数字比较 失败会变成NaN与数字比较 永远都是false 字符串之间的比较: 每个字符分别按照Unicode编码比较大小 如果当前字符相同进行后移 不相同直接出结果 == != 先进行数据转换后进行比较 只进行值得比较 === !== 直接进行数据比较 进行数据转换 同时判断数据类型和值是否完全相等 逻辑运算符: !非 对现有条件进行取反 非真
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS7,8上快速安装Gitea,搭建Git服务器
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- Jdk安装(Linux,MacOS,Windows),包含三大操作系统的最全安装
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- Windows10,CentOS7,CentOS8安装MongoDB4.0.16
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- Eclipse初始化配置,告别卡顿、闪退、编译时间过长
- 设置Eclipse缩进为4个空格,增强代码规范
- SpringBoot2整合Redis,开启缓存,提高访问速度
- Windows10,CentOS7,CentOS8安装Nodejs环境