学习微服务的断路器——hystrix
微服务架构中,微服务之间相互调用,springcloud可以用feign方式和RestTemplate+ribbon方式来实现服务间的相互调用。但如果某一个服务出现问题,所有调用该出问题的服务都将出现阻塞,如果有大量的请求,则Servlet容器的线程资源会被消耗完毕,导致服务瘫痪。服务与服务之间的依赖性,故障会传播,会对整个微服务系统造成灾难性的严重后果,这就是服务故障的“雪崩”效应。
为了解决这个问题,业界提出了断路器模型。
1.ribbon中使用断路器hystrix
build.gradle文件
buildscript {
ext {
springBootVersion = '2.0.4.RELEASE'
}
repositories {
mavenCentral()
}
dependencies {
classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}")
}
}
apply plugin: 'java'
apply plugin: 'eclipse'
apply plugin: 'org.springframework.boot'
apply plugin: 'io.spring.dependency-management'
group = 'com.example'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
ext {
springCloudVersion = 'Finchley.SR1'
}
dependencies {
compile('org.springframework.boot:spring-boot-starter-web')
compile('org.springframework.cloud:spring-cloud-starter-netflix-eureka-client')
compile('org.springframework.cloud:spring-cloud-starter-netflix-hystrix')
compile('org.springframework.cloud:spring-cloud-starter-netflix-ribbon')
testCompile('org.springframework.boot:spring-boot-starter-test')
}
dependencyManagement {
imports {
mavenBom "org.springframework.cloud:spring-cloud-dependencies:${springCloudVersion}"
}
}
application.yml文件
server:
port: 8797
spring:
application:
name: service-ribbon-hystrix
eureka:
client:
service-url:
defaultZone: http://localhost:8791/eureka/
主方法
package com.example.serviceribbonhystrix;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
import org.springframework.cloud.netflix.hystrix.EnableHystrix;
import org.springframework.context.annotation.Bean;
import org.springframework.web.client.RestTemplate;
@EnableHystrix
@EnableEurekaClient
@SpringBootApplication
public class ServiceRibbonHystrixApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceRibbonHystrixApplication.class, args);
}
@Bean
RestTemplate restTemplate() {
return new RestTemplate();
}
}
HelloService.java文件
package com.example.serviceribbonhystrix;
import com.netflix.hystrix.contrib.javanica.annotation.HystrixCommand;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
@Service
public class HelloService {
@Autowired
RestTemplate restTemplate;
@HystrixCommand(fallbackMethod = "errorMsg")
public String hiService() {
return restTemplate.getForObject("http://EUREKA-CLIENT-SAY-HI/hi", String.class);
}
public String errorMsg(){
return "I am ribbon with hystrix,Wrong!";
}
}
HelloController.java文件
package com.example.serviceribbonhystrix;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@Autowired
HelloService helloService;
@RequestMapping("/hi")
public String sayHi() {
return helloService.hiService();
}
}
只是单独启动该服务,不启动eureka-client-say-hi服务,访问http://localhost:8797/hi
返回要返回的信息,此时断路器已经生效了:
2.feign中使用断路器hystrix
因为feign是自带断路器的,所以依赖中就不需要加hystrix
build.gradle
buildscript {
ext {
springBootVersion = '2.0.4.RELEASE'
}
repositories {
mavenCentral()
}
dependencies {
classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}")
}
}
apply plugin: 'java'
apply plugin: 'eclipse'
apply plugin: 'org.springframework.boot'
apply plugin: 'io.spring.dependency-management'
group = 'com.example'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
ext {
springCloudVersion = 'Finchley.SR1'
}
dependencies {
compile('org.springframework.boot:spring-boot-starter-web')
compile('org.springframework.cloud:spring-cloud-starter-netflix-eureka-client')
compile('org.springframework.cloud:spring-cloud-starter-openfeign')
testCompile('org.springframework.boot:spring-boot-starter-test')
}
dependencyManagement {
imports {
mavenBom "org.springframework.cloud:spring-cloud-dependencies:${springCloudVersion}"
}
}
application.yml文件
server:
port: 8796
spring:
application:
name: service-feign-hystrix
eureka:
client:
service-url:
defaultZone: http://localhost:8791/eureka/
feign:
hystrix:
enabled: true #feign自带断路器,但需要打开
主方法:
package com.example.servicefeignhystrix;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
import org.springframework.cloud.openfeign.EnableFeignClients;
@EnableEurekaClient
@EnableFeignClients
@SpringBootApplication
public class ServiceFeignHystrixApplication {
public static void main(String[] args) {
SpringApplication.run(ServiceFeignHystrixApplication.class, args);
}
}
接口SayHiService.java文件
package com.example.servicefeignhystrix;
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@FeignClient(value = "eureka-client-say-hi",fallback = SayHiServiceWithHystrix.class)
public interface SayHiService {
@RequestMapping(value = "/hi", method = RequestMethod.GET)
String sayHiFromClient();
}
SayHiServiceWithHystrix.java文件
package com.example.servicefeignhystrix;
import org.springframework.stereotype.Component;
@Component
public class SayHiServiceWithHystrix implements SayHiService {
@Override
public String sayHiFromClient() {
return "sorry,I am wrong";
}
}
HelloController.java文件
package com.example.servicefeignhystrix;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@Autowired
SayHiService sayHiService;
@RequestMapping("/hi")
public String sayHi(){
return sayHiService.sayHiFromClient();
}
}
此时启动eureka-server,以及eureka-client-say-hi项目的两个实例,以及刚才新建的项目service-feign-hystrix.
访问http://localhost:8796/hi
出现以下两种场景:
和
如果关掉8792端口的服务,则会返回hi,I am port 8793
如果关掉eureka-client-say-hi服务的8792和8793两个端口实例,则会返回
此时标明,feign的断路器已经生效了。
现在加Java高级架构群即可获取Java工程化、高性能及分布式、高性能、高架构。性能调优、Spring,MyBatis,Netty源码分析和大数据等多个知识点高级进阶干货的直播免费学习权限及领取相关资料群号是:83563&8062
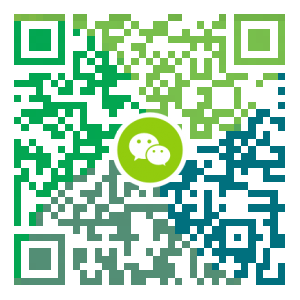
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
什么是物联网的边缘计算?
越来越多的“连接”设备产生了过多的数据,随着物联网(IoT)技术和用例在未来几年的发展,这种情况将继续存在。根据研究公司Gartner的数据,到2020年,将有多达200亿台连接设备为每位用户生成数十亿字节的数据。这些设备不仅仅是智能手机或笔记本电脑,还包括联网汽车,自动售货机,智能可穿戴设备,手术医疗机器人等等。 由无数类型的此类设备生成的大量数据需要推送到集中式云以进行保留(数据管理),分析和决策。然后,再将分析的数据结果传回设备。这种数据的往返消耗了大量网络基础设施和云基础设施资源,进一步增加了延迟和带宽消耗问题,从而影响关键任务物联网使用。例如,在自动驾驶的连接车中,每小时产生了大量数据;,数据必须上传到云端,进行分析,并将指令发送回汽车。低延迟或资源拥塞可能会延迟对汽车的响应,严重时可能导致交通事故。 物联网边缘计算 这就是边缘计算的用武之地。边缘计算体系结构可用于优化云计算系统,以便在网络边缘执行数据处理和分析,更接近数据源。通过这种方法,可以在设备本身附近收集和处理数据,而不是将数据发送到云或数据中心。 边缘计算的好处: ●边缘计算可以降低传感器和中央云之间所需的网络带宽...
- 下一篇
【我们一起写框架】MVVM的WPF框架(一)—序篇
前言我想,有一部分程序员应该是在二三线城市的,虽然不知道占比,但想来应该不在少数。 我是这部分人群中的一份子。 我们这群人,面对的客户,大多是国内中小企业,或者政府的小部门。这类客户的特点是,资金有限,人力有限。 什么意思呢?就是你如果敢给他安一台Linux服务器,客户的信息员和测试员会把你堵在墙角问候你全家安好,他们Window都用不明白呢,你给安Linux,要疯啊。 所以,Core对我们而言,没有意义,因为大家都是Windows。 关于业务在二三线城市的我们,立身之本不是写算法,也不是各种高级的、新出的技术,而是,写业务模块。 不要小看写业务模块,在二三线城市,一个不会写业务模块的程序员,即便知识面再广,也是个烂程序员。为什么?因为他不能干活呀。 其实把业务模块写好,并不是件容易的事。因为它涉及到对业务的理解,对社会的认知。 以我多年的经验,能写好业务模块的优秀开发人员,通常都需要三四年经验。普通一点,大约就需要五到十年。当然还有十年以上经验,还很没掌握写业务的。 这里面有个特例,那就是硕士和博士。因为他们的年龄较大,阅历较多,所以,通常两年就能把业务写的很好。此外就没有特例了,什...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS8编译安装MySQL8.0.19
- MySQL8.0.19开启GTID主从同步CentOS8
- SpringBoot2全家桶,快速入门学习开发网站教程
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- CentOS7,CentOS8安装Elasticsearch6.8.6
- Red5直播服务器,属于Java语言的直播服务器
- CentOS8安装MyCat,轻松搞定数据库的读写分离、垂直分库、水平分库
- SpringBoot2整合MyBatis,连接MySql数据库做增删改查操作
- CentOS6,CentOS7官方镜像安装Oracle11G
- Jdk安装(Linux,MacOS,Windows),包含三大操作系统的最全安装