整理收集的一些常用java工具类
1.json转换工具
- package com.taotao.utils;
- import java.util.List;
- import com.fasterxml.jackson.core.JsonProcessingException;
- import com.fasterxml.jackson.databind.JavaType;
- import com.fasterxml.jackson.databind.JsonNode;
- import com.fasterxml.jackson.databind.ObjectMapper;
- /**
- * json转换工具类
- */
- public class JsonUtils {
- // 定义jackson对象
- private static final ObjectMapper MAPPER = new ObjectMapper();
- /**
- * 将对象转换成json字符串。
- * <p>Title: pojoToJson</p>
- * <p>Description: </p>
- * @param data
- * @return
- */
- public static String objectToJson(Object data) {
- try {
- String string = MAPPER.writeValueAsString(data);
- return string;
- } catch (JsonProcessingException e) {
- e.printStackTrace();
- }
- return null;
- }
- /**
- * 将json结果集转化为对象
- *
- * @param jsonData json数据
- * @param clazz 对象中的object类型
- * @return
- */
- public static <T> T jsonToPojo(String jsonData, Class<T> beanType) {
- try {
- T t = MAPPER.readValue(jsonData, beanType);
- return t;
- } catch (Exception e) {
- e.printStackTrace();
- }
- return null;
- }
- /**
- * 将json数据转换成pojo对象list
- * <p>Title: jsonToList</p>
- * <p>Description: </p>
- * @param jsonData
- * @param beanType
- * @return
- */
- public static <T>List<T> jsonToList(String jsonData, Class<T> beanType) {
- JavaType javaType = MAPPER.getTypeFactory().constructParametricType(List.class, beanType);
- try {
- List<T> list = MAPPER.readValue(jsonData, javaType);
- return list;
- } catch (Exception e) {
- e.printStackTrace();
- }
- return null;
- }
- }
2.cookie的读写
- package com.taotao.common.utils;
- import java.io.UnsupportedEncodingException;
- import java.net.URLDecoder;
- import java.net.URLEncoder;
- import javax.servlet.http.Cookie;
- import javax.servlet.http.HttpServletRequest;
- import javax.servlet.http.HttpServletResponse;
- /**
- *
- * Cookie 工具类
- *
- */
- public final class CookieUtils {
- /**
- * 得到Cookie的值, 不编码
- *
- * @param request
- * @param cookieName
- * @return
- */
- public static String getCookieValue(HttpServletRequest request, String cookieName) {
- return getCookieValue(request, cookieName, false);
- }
- /**
- * 得到Cookie的值,
- *
- * @param request
- * @param cookieName
- * @return
- */
- public static String getCookieValue(HttpServletRequest request, String cookieName, boolean isDecoder) {
- Cookie[] cookieList = request.getCookies();
- if (cookieList == null || cookieName == null) {
- return null;
- }
- String retValue = null;
- try {
- for (int i = 0; i < cookieList.length; i++) {
- if (cookieList[i].getName().equals(cookieName)) {
- if (isDecoder) {
- retValue = URLDecoder.decode(cookieList[i].getValue(), "UTF-8");
- } else {
- retValue = cookieList[i].getValue();
- }
- break;
- }
- }
- } catch (UnsupportedEncodingException e) {
- e.printStackTrace();
- }
- return retValue;
- }
- /**
- * 得到Cookie的值,
- *
- * @param request
- * @param cookieName
- * @return
- */
- public static String getCookieValue(HttpServletRequest request, String cookieName, String encodeString) {
- Cookie[] cookieList = request.getCookies();
- if (cookieList == null || cookieName == null) {
- return null;
- }
- String retValue = null;
- try {
- for (int i = 0; i < cookieList.length; i++) {
- if (cookieList[i].getName().equals(cookieName)) {
- retValue = URLDecoder.decode(cookieList[i].getValue(), encodeString);
- break;
- }
- }
- } catch (UnsupportedEncodingException e) {
- e.printStackTrace();
- }
- return retValue;
- }
- /**
- * 设置Cookie的值 不设置生效时间默认浏览器关闭即失效,也不编码
- */
- public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName,
- String cookieValue) {
- setCookie(request, response, cookieName, cookieValue, -1);
- }
- /**
- * 设置Cookie的值 在指定时间内生效,但不编码
- */
- public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName,
- String cookieValue, int cookieMaxage) {
- setCookie(request, response, cookieName, cookieValue, cookieMaxage, false);
- }
- /**
- * 设置Cookie的值 不设置生效时间,但编码
- */
- public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName,
- String cookieValue, boolean isEncode) {
- setCookie(request, response, cookieName, cookieValue, -1, isEncode);
- }
- /**
- * 设置Cookie的值 在指定时间内生效, 编码参数
- */
- public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName,
- String cookieValue, int cookieMaxage, boolean isEncode) {
- doSetCookie(request, response, cookieName, cookieValue, cookieMaxage, isEncode);
- }
- /**
- * 设置Cookie的值 在指定时间内生效, 编码参数(指定编码)
- */
- public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName,
- String cookieValue, int cookieMaxage, String encodeString) {
- doSetCookie(request, response, cookieName, cookieValue, cookieMaxage, encodeString);
- }
- /**
- * 删除Cookie带cookie域名
- */
- public static void deleteCookie(HttpServletRequest request, HttpServletResponse response,
- String cookieName) {
- doSetCookie(request, response, cookieName, "", -1, false);
- }
- /**
- * 设置Cookie的值,并使其在指定时间内生效
- *
- * @param cookieMaxage cookie生效的最大秒数
- */
- private static final void doSetCookie(HttpServletRequest request, HttpServletResponse response,
- String cookieName, String cookieValue, int cookieMaxage, boolean isEncode) {
- try {
- if (cookieValue == null) {
- cookieValue = "";
- } else if (isEncode) {
- cookieValue = URLEncoder.encode(cookieValue, "utf-8");
- }
- Cookie cookie = new Cookie(cookieName, cookieValue);
- if (cookieMaxage > 0)
- cookie.setMaxAge(cookieMaxage);
- if (null != request) {// 设置域名的cookie
- String domainName = getDomainName(request);
- System.out.println(domainName);
- if (!"localhost".equals(domainName)) {
- cookie.setDomain(domainName);
- }
- }
- cookie.setPath("/");
- response.addCookie(cookie);
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
- /**
- * 设置Cookie的值,并使其在指定时间内生效
- *
- * @param cookieMaxage cookie生效的最大秒数
- */
- private static final void doSetCookie(HttpServletRequest request, HttpServletResponse response,
- String cookieName, String cookieValue, int cookieMaxage, String encodeString) {
- try {
- if (cookieValue == null) {
- cookieValue = "";
- } else {
- cookieValue = URLEncoder.encode(cookieValue, encodeString);
- }
- Cookie cookie = new Cookie(cookieName, cookieValue);
- if (cookieMaxage > 0)
- cookie.setMaxAge(cookieMaxage);
- if (null != request) {// 设置域名的cookie
- String domainName = getDomainName(request);
- System.out.println(domainName);
- if (!"localhost".equals(domainName)) {
- cookie.setDomain(domainName);
- }
- }
- cookie.setPath("/");
- response.addCookie(cookie);
- } catch (Exception e) {
- e.printStackTrace();
- }
- }
- /**
- * 得到cookie的域名
- */
- private static final String getDomainName(HttpServletRequest request) {
- String domainName = null;
- String serverName = request.getRequestURL().toString();
- if (serverName == null || serverName.equals("")) {
- domainName = "";
- } else {
- serverName = serverName.toLowerCase();
- serverName = serverName.substring(7);
- final int end = serverName.indexOf("/");
- serverName = serverName.substring(0, end);
- final String[] domains = serverName.split("\\.");
- int len = domains.length;
- if (len > 3) {
- // www.xxx.com.cn
- domainName = "." + domains[len - 3] + "." + domains[len - 2] + "." + domains[len - 1];
- } else if (len <= 3 && len > 1) {
- // xxx.com or xxx.cn
- domainName = "." + domains[len - 2] + "." + domains[len - 1];
- } else {
- domainName = serverName;
- }
- }
- if (domainName != null && domainName.indexOf(":") > 0) {
- String[] ary = domainName.split("\\:");
- domainName = ary[0];
- }
- return domainName;
- }
- }
3.HttpClientUtil 相关包及文档下载
- package com.taotao.utils;
- import java.io.IOException;
- import java.net.URI;
- import java.util.ArrayList;
- import java.util.List;
- import java.util.Map;
- import org.apache.http.NameValuePair;
- import org.apache.http.client.entity.UrlEncodedFormEntity;
- import org.apache.http.client.methods.CloseableHttpResponse;
- import org.apache.http.client.methods.HttpGet;
- import org.apache.http.client.methods.HttpPost;
- import org.apache.http.client.utils.URIBuilder;
- import org.apache.http.entity.ContentType;
- import org.apache.http.entity.StringEntity;
- import org.apache.http.impl.client.CloseableHttpClient;
- import org.apache.http.impl.client.HttpClients;
- import org.apache.http.message.BasicNameValuePair;
- import org.apache.http.util.EntityUtils;
- public class HttpClientUtil {
- public static String doGet(String url, Map<String, String> param) {
- // 创建Httpclient对象
- CloseableHttpClient httpclient = HttpClients.createDefault();
- String resultString = "";
- CloseableHttpResponse response = null;
- try {
- // 创建uri
- URIBuilder builder = new URIBuilder(url);
- if (param != null) {
- for (String key : param.keySet()) {
- builder.addParameter(key, param.get(key));
- }
- }
- URI uri = builder.build();
- // 创建http GET请求
- HttpGet httpGet = new HttpGet(uri);
- // 执行请求
- response = httpclient.execute(httpGet);
- // 判断返回状态是否为200
- if (response.getStatusLine().getStatusCode() == 200) {
- resultString = EntityUtils.toString(response.getEntity(), "UTF-8");
- }
- } catch (Exception e) {
- e.printStackTrace();
- } finally {
- try {
- if (response != null) {
- response.close();
- }
- httpclient.close();
- } catch (IOException e) {
- e.printStackTrace();
- }
- }
- return resultString;
- }
- public static String doGet(String url) {
- return doGet(url, null);
- }
- public static String doPost(String url, Map<String, String> param) {
- // 创建Httpclient对象
- CloseableHttpClient httpClient = HttpClients.createDefault();
- CloseableHttpResponse response = null;
- String resultString = "";
- try {
- // 创建Http Post请求
- HttpPost httpPost = new HttpPost(url);
- // 创建参数列表
- if (param != null) {
- List<NameValuePair> paramList = new ArrayList<>();
- for (String key : param.keySet()) {
- paramList.add(new BasicNameValuePair(key, param.get(key)));
- }
- // 模拟表单
- UrlEncodedFormEntity entity = new UrlEncodedFormEntity(paramList);
- httpPost.setEntity(entity);
- }
- // 执行http请求
- response = httpClient.execute(httpPost);
- resultString = EntityUtils.toString(response.getEntity(), "utf-8");
- } catch (Exception e) {
- e.printStackTrace();
- } finally {
- try {
- response.close();
- } catch (IOException e) {
- // TODO Auto-generated catch block
- e.printStackTrace();
- }
- }
- return resultString;
- }
- public static String doPost(String url) {
- return doPost(url, null);
- }
- public static String doPostJson(String url, String json) {
- // 创建Httpclient对象
- CloseableHttpClient httpClient = HttpClients.createDefault();
- CloseableHttpResponse response = null;
- String resultString = "";
- try {
- // 创建Http Post请求
- HttpPost httpPost = new HttpPost(url);
- // 创建请求内容
- StringEntity entity = new StringEntity(json, ContentType.APPLICATION_JSON);
- httpPost.setEntity(entity);
- // 执行http请求
- response = httpClient.execute(httpPost);
- resultString = EntityUtils.toString(response.getEntity(), "utf-8");
- } catch (Exception e) {
- e.printStackTrace();
- } finally {
- try {
- response.close();
- } catch (IOException e) {
- // TODO Auto-generated catch block
- e.printStackTrace();
- }
- }
- return resultString;
- }
- }
4.FastDFSClient工具类 相关包以及文档下载
- package cn.itcast.fastdfs.cliennt;
- import org.csource.common.NameValuePair;
- import org.csource.fastdfs.ClientGlobal;
- import org.csource.fastdfs.StorageClient1;
- import org.csource.fastdfs.StorageServer;
- import org.csource.fastdfs.TrackerClient;
- import org.csource.fastdfs.TrackerServer;
- public class FastDFSClient {
- private TrackerClient trackerClient = null;
- private TrackerServer trackerServer = null;
- private StorageServer storageServer = null;
- private StorageClient1 storageClient = null;
- public FastDFSClient(String conf) throws Exception {
- if (conf.contains("classpath:")) {
- conf = conf.replace("classpath:", this.getClass().getResource("/").getPath());
- }
- ClientGlobal.init(conf);
- trackerClient = new TrackerClient();
- trackerServer = trackerClient.getConnection();
- storageServer = null;
- storageClient = new StorageClient1(trackerServer, storageServer);
- }
- /**
- * 上传文件方法
- * <p>Title: uploadFile</p>
- * <p>Description: </p>
- * @param fileName 文件全路径
- * @param extName 文件扩展名,不包含(.)
- * @param metas 文件扩展信息
- * @return
- * @throws Exception
- */
- public String uploadFile(String fileName, String extName, NameValuePair[] metas) throws Exception {
- String result = storageClient.upload_file1(fileName, extName, metas);
- return result;
- }
- public String uploadFile(String fileName) throws Exception {
- return uploadFile(fileName, null, null);
- }
- public String uploadFile(String fileName, String extName) throws Exception {
- return uploadFile(fileName, extName, null);
- }
- /**
- * 上传文件方法
- * <p>Title: uploadFile</p>
- * <p>Description: </p>
- * @param fileContent 文件的内容,字节数组
- * @param extName 文件扩展名
- * @param metas 文件扩展信息
- * @return
- * @throws Exception
- */
- public String uploadFile(byte[] fileContent, String extName, NameValuePair[] metas) throws Exception {
- String result = storageClient.upload_file1(fileContent, extName, metas);
- return result;
- }
- public String uploadFile(byte[] fileContent) throws Exception {
- return uploadFile(fileContent, null, null);
- }
- public String uploadFile(byte[] fileContent, String extName) throws Exception {
- return uploadFile(fileContent, extName, null);
- }
- }
- <span style="font-size:14px;font-weight:normal;">public class FastDFSTest {
- @Test
- public void testFileUpload() throws Exception {
- // 1、加载配置文件,配置文件中的内容就是tracker服务的地址。
- ClientGlobal.init("D:/workspaces-itcast/term197/taotao-manager-web/src/main/resources/resource/client.conf");
- // 2、创建一个TrackerClient对象。直接new一个。
- TrackerClient trackerClient = new TrackerClient();
- // 3、使用TrackerClient对象创建连接,获得一个TrackerServer对象。
- TrackerServer trackerServer = trackerClient.getConnection();
- // 4、创建一个StorageServer的引用,值为null
- StorageServer storageServer = null;
- // 5、创建一个StorageClient对象,需要两个参数TrackerServer对象、StorageServer的引用
- StorageClient storageClient = new StorageClient(trackerServer, storageServer);
- // 6、使用StorageClient对象上传图片。
- //扩展名不带“.”
- String[] strings = storageClient.upload_file("D:/Documents/Pictures/images/200811281555127886.jpg", "jpg", null);
- // 7、返回数组。包含组名和图片的路径。
- for (String string : strings) {
- System.out.println(string);
- }
- }
- }</span>
5.获取异常的堆栈信息
- package com.taotao.utils;
- import java.io.PrintWriter;
- import java.io.StringWriter;
- public class ExceptionUtil {
- /**
- * 获取异常的堆栈信息
- *
- * @param t
- * @return
- */
- public static String getStackTrace(Throwable t) {
- StringWriter sw = new StringWriter();
- PrintWriter pw = new PrintWriter(sw);
- try {
- t.printStackTrace(pw);
- return sw.toString();
- } finally {
- pw.close();
- }
- }
- }
6.easyUIDataGrid对象返回值
- package com.taotao.result;
- import java.util.List;
- /**
- * easyUIDataGrid对象返回值
- * <p>Title: EasyUIResult</p>
- * <p>Description: </p>
- * <p>Company: www.itcast.com</p>
- * @author 入云龙
- * @date 2015年7月21日下午4:12:52
- * @version 1.0
- */
- public class EasyUIResult {
- private Integer total;
- private List<?> rows;
- public EasyUIResult(Integer total, List<?> rows) {
- this.total = total;
- this.rows = rows;
- }
- public EasyUIResult(long total, List<?> rows) {
- this.total = (int) total;
- this.rows = rows;
- }
- public Integer getTotal() {
- return total;
- }
- public void setTotal(Integer total) {
- this.total = total;
- }
- public List<?> getRows() {
- return rows;
- }
- public void setRows(List<?> rows) {
- this.rows = rows;
- }
- }
7.ftp上传下载工具类
- package com.taotao.utils;
- import java.io.File;
- import java.io.FileInputStream;
- import java.io.FileNotFoundException;
- import java.io.FileOutputStream;
- import java.io.IOException;
- import java.io.InputStream;
- import java.io.OutputStream;
- import org.apache.commons.net.ftp.FTP;
- import org.apache.commons.net.ftp.FTPClient;
- import org.apache.commons.net.ftp.FTPFile;
- import org.apache.commons.net.ftp.FTPReply;
- /**
- * ftp上传下载工具类
- * <p>Title: FtpUtil</p>
- * <p>Description: </p>
- * <p>Company: www.itcast.com</p>
- * @author 入云龙
- * @date 2015年7月29日下午8:11:51
- * @version 1.0
- */
- public class FtpUtil {
- /**
- * Description: 向FTP服务器上传文件
- * @param host FTP服务器hostname
- * @param port FTP服务器端口
- * @param username FTP登录账号
- * @param password FTP登录密码
- * @param basePath FTP服务器基础目录
- * @param filePath FTP服务器文件存放路径。例如分日期存放:/2015/01/01。文件的路径为basePath+filePath
- * @param filename 上传到FTP服务器上的文件名
- * @param input 输入流
- * @return 成功返回true,否则返回false
- */
- public static boolean uploadFile(String host, int port, String username, String password, String basePath,
- String filePath, String filename, InputStream input) {
- boolean result = false;
- FTPClient ftp = new FTPClient();
- try {
- int reply;
- ftp.connect(host, port);// 连接FTP服务器
- // 如果采用默认端口,可以使用ftp.connect(host)的方式直接连接FTP服务器
- ftp.login(username, password);// 登录
- reply = ftp.getReplyCode();
- if (!FTPReply.isPositiveCompletion(reply)) {
- ftp.disconnect();
- return result;
- }
- //切换到上传目录
- if (!ftp.changeWorkingDirectory(basePath+filePath)) {
- //如果目录不存在创建目录
- String[] dirs = filePath.split("/");
- String tempPath = basePath;
- for (String dir : dirs) {
- if (null == dir || "".equals(dir)) continue;
- tempPath += "/" + dir;
- if (!ftp.changeWorkingDirectory(tempPath)) {
- if (!ftp.makeDirectory(tempPath)) {
- return result;
- } else {
- ftp.changeWorkingDirectory(tempPath);
- }
- }
- }
- }
- //设置上传文件的类型为二进制类型
- ftp.setFileType(FTP.BINARY_FILE_TYPE);
- //上传文件
- if (!ftp.storeFile(filename, input)) {
- return result;
- }
- input.close();
- ftp.logout();
- result = true;
- } catch (IOException e) {
- e.printStackTrace();
- } finally {
- if (ftp.isConnected()) {
- try {
- ftp.disconnect();
- } catch (IOException ioe) {
- }
- }
- }
- return result;
- }
- /**
- * Description: 从FTP服务器下载文件
- * @param host FTP服务器hostname
- * @param port FTP服务器端口
- * @param username FTP登录账号
- * @param password FTP登录密码
- * @param remotePath FTP服务器上的相对路径
- * @param fileName 要下载的文件名
- * @param localPath 下载后保存到本地的路径
- * @return
- */
- public static boolean downloadFile(String host, int port, String username, String password, String remotePath,
- String fileName, String localPath) {
- boolean result = false;
- FTPClient ftp = new FTPClient();
- try {
- int reply;
- ftp.connect(host, port);
- // 如果采用默认端口,可以使用ftp.connect(host)的方式直接连接FTP服务器
- ftp.login(username, password);// 登录
- reply = ftp.getReplyCode();
- if (!FTPReply.isPositiveCompletion(reply)) {
- ftp.disconnect();
- return result;
- }
- ftp.changeWorkingDirectory(remotePath);// 转移到FTP服务器目录
- FTPFile[] fs = ftp.listFiles();
- for (FTPFile ff : fs) {
- if (ff.getName().equals(fileName)) {
- File localFile = new File(localPath + "/" + ff.getName());
- OutputStream is = new FileOutputStream(localFile);
- ftp.retrieveFile(ff.getName(), is);
- is.close();
- }
- }
- ftp.logout();
- result = true;
- } catch (IOException e) {
- e.printStackTrace();
- } finally {
- if (ftp.isConnected()) {
- try {
- ftp.disconnect();
- } catch (IOException ioe) {
- }
- }
- }
- return result;
- }
- public static void main(String[] args) {
- try {
- FileInputStream in=new FileInputStream(new File("D:\\temp\\image\\gaigeming.jpg"));
- boolean flag = uploadFile("192.168.25.133", 21, "ftpuser", "ftpuser", "/home/ftpuser/www/images","/2015/01/21", "gaigeming.jpg", in);
- System.out.println(flag);
- } catch (FileNotFoundException e) {
- e.printStackTrace();
- }
- }
- }
8.各种id生成策略
- package com.taotao.utils;
- import java.util.Random;
- /**
- * 各种id生成策略
- * <p>Title: IDUtils</p>
- * <p>Description: </p>
- * @date 2015年7月22日下午2:32:10
- * @version 1.0
- */
- public class IDUtils {
- /**
- * 图片名生成
- */
- public static String genImageName() {
- //取当前时间的长整形值包含毫秒
- long millis = System.currentTimeMillis();
- //long millis = System.nanoTime();
- //加上三位随机数
- Random random = new Random();
- int end3 = random.nextInt(999);
- //如果不足三位前面补0
- String str = millis + String.format("%03d", end3);
- return str;
- }
- /**
- * 商品id生成
- */
- public static long genItemId() {
- //取当前时间的长整形值包含毫秒
- long millis = System.currentTimeMillis();
- //long millis = System.nanoTime();
- //加上两位随机数
- Random random = new Random();
- int end2 = random.nextInt(99);
- //如果不足两位前面补0
- String str = millis + String.format("%02d", end2);
- long id = new Long(str);
- return id;
- }
- public static void main(String[] args) {
- for(int i=0;i< 100;i++)
- System.out.println(genItemId());
- }
- }
9.上传图片返回值
- package com.result;
- /**
- * 上传图片返回值
- * <p>Title: PictureResult</p>
- * <p>Description: </p>
- * <p>Company: www.itcast.com</p>
- * @author 入云龙
- * @date 2015年7月22日下午2:09:02
- * @version 1.0
- */
- public class PictureResult {
- /**
- * 上传图片返回值,成功:0 失败:1
- */
- private Integer error;
- /**
- * 回显图片使用的url
- */
- private String url;
- /**
- * 错误时的错误消息
- */
- private String message;
- public PictureResult(Integer state, String url) {
- this.url = url;
- this.error = state;
- }
- public PictureResult(Integer state, String url, String errorMessage) {
- this.url = url;
- this.error = state;
- this.message = errorMessage;
- }
- public Integer getError() {
- return error;
- }
- public void setError(Integer error) {
- this.error = error;
- }
- public String getUrl() {
- return url;
- }
- public void setUrl(String url) {
- this.url = url;
- }
- public String getMessage() {
- return message;
- }
- public void setMessage(String message) {
- this.message = message;
- }
- }
10.自定义响应结构
- package com.result;
- import java.util.List;
- import com.fasterxml.jackson.databind.JsonNode;
- import com.fasterxml.jackson.databind.ObjectMapper;
- /**
- * 自定义响应结构
- */
- public class TaotaoResult {
- // 定义jackson对象
- private static final ObjectMapper MAPPER = new ObjectMapper();
- // 响应业务状态
- private Integer status;
- // 响应消息
- private String msg;
- // 响应中的数据
- private Object data;
- public static TaotaoResult build(Integer status, String msg, Object data) {
- return new TaotaoResult(status, msg, data);
- }
- public static TaotaoResult ok(Object data) {
- return new TaotaoResult(data);
- }
- public static TaotaoResult ok() {
- return new TaotaoResult(null);
- }
- public TaotaoResult() {
- }
- public static TaotaoResult build(Integer status, String msg) {
- return new TaotaoResult(status, msg, null);
- }
- public TaotaoResult(Integer status, String msg, Object data) {
- this.status = status;
- this.msg = msg;
- this.data = data;
- }
- public TaotaoResult(Object data) {
- this.status = 200;
- this.msg = "OK";
- this.data = data;
- }
- // public Boolean isOK() {
- // return this.status == 200;
- // }
- public Integer getStatus() {
- return status;
- }
- public void setStatus(Integer status) {
- this.status = status;
- }
- public String getMsg() {
- return msg;
- }
- public void setMsg(String msg) {
- this.msg = msg;
- }
- public Object getData() {
- return data;
- }
- public void setData(Object data) {
- this.data = data;
- }
- /**
- * 将json结果集转化为TaotaoResult对象
- *
- * @param jsonData json数据
- * @param clazz TaotaoResult中的object类型
- * @return
- */
- public static TaotaoResult formatToPojo(String jsonData, Class<?> clazz) {
- try {
- if (clazz == null) {
- return MAPPER.readValue(jsonData, TaotaoResult.class);
- }
- JsonNode jsonNode = MAPPER.readTree(jsonData);
- JsonNode data = jsonNode.get("data");
- Object obj = null;
- if (clazz != null) {
- if (data.isObject()) {
- obj = MAPPER.readValue(data.traverse(), clazz);
- } else if (data.isTextual()) {
- obj = MAPPER.readValue(data.asText(), clazz);
- }
- }
- return build(jsonNode.get("status").intValue(), jsonNode.get("msg").asText(), obj);
- } catch (Exception e) {
- return null;
- }
- }
- /**
- * 没有object对象的转化
- *
- * @param json
- * @return
- */
- public static TaotaoResult format(String json) {
- try {
- return MAPPER.readValue(json, TaotaoResult.class);
- } catch (Exception e) {
- e.printStackTrace();
- }
- return null;
- }
- /**
- * Object是集合转化
- *
- * @param jsonData json数据
- * @param clazz 集合中的类型
- * @return
- */
- public static TaotaoResult formatToList(String jsonData, Class<?> clazz) {
- try {
- JsonNode jsonNode = MAPPER.readTree(jsonData);
- JsonNode data = jsonNode.get("data");
- Object obj = null;
- if (data.isArray() && data.size() > 0) {
- obj = MAPPER.readValue(data.traverse(),
- MAPPER.getTypeFactory().constructCollectionType(List.class, clazz));
- }
- return build(jsonNode.get("status").intValue(), jsonNode.get("msg").asText(), obj);
- } catch (Exception e) {
- return null;
- }
- }
- }
11.jedis操作
- package com.taotao.jedis;
- public interface JedisClient {
- String set(String key, String value);
- String get(String key);
- Boolean exists(String key);
- Long expire(String key, int seconds);
- Long ttl(String key);
- Long incr(String key);
- Long hset(String key, String field, String value);
- String hget(String key, String field);
- Long hdel(String key, String... field);
- }
- package com.taotao.jedis;
- import org.springframework.beans.factory.annotation.Autowired;
- import redis.clients.jedis.JedisCluster;
- public class JedisClientCluster implements JedisClient {
- @Autowired
- private JedisCluster jedisCluster;
- @Override
- public String set(String key, String value) {
- return jedisCluster.set(key, value);
- }
- @Override
- public String get(String key) {
- return jedisCluster.get(key);
- }
- @Override
- public Boolean exists(String key) {
- return jedisCluster.exists(key);
- }
- @Override
- public Long expire(String key, int seconds) {
- return jedisCluster.expire(key, seconds);
- }
- @Override
- public Long ttl(String key) {
- return jedisCluster.ttl(key);
- }
- @Override
- public Long incr(String key) {
- return jedisCluster.incr(key);
- }
- @Override
- public Long hset(String key, String field, String value) {
- return jedisCluster.hset(key, field, value);
- }
- @Override
- public String hget(String key, String field) {
- return jedisCluster.hget(key, field);
- }
- @Override
- public Long hdel(String key, String... field) {
- return jedisCluster.hdel(key, field);
- }
- }
- package com.taotao.jedis;
- import org.springframework.beans.factory.annotation.Autowired;
- import redis.clients.jedis.Jedis;
- import redis.clients.jedis.JedisPool;
- public class JedisClientPool implements JedisClient {
- @Autowired
- private JedisPool jedisPool;
- @Override
- public String set(String key, String value) {
- Jedis jedis = jedisPool.getResource();
- String result = jedis.set(key, value);
- jedis.close();
- return result;
- }
- @Override
- public String get(String key) {
- Jedis jedis = jedisPool.getResource();
- String result = jedis.get(key);
- jedis.close();
- return result;
- }
- @Override
- public Boolean exists(String key) {
- Jedis jedis = jedisPool.getResource();
- Boolean result = jedis.exists(key);
- jedis.close();
- return result;
- }
- @Override
- public Long expire(String key, int seconds) {
- Jedis jedis = jedisPool.getResource();
- Long result = jedis.expire(key, seconds);
- jedis.close();
- return result;
- }
- @Override
- public Long ttl(String key) {
- Jedis jedis = jedisPool.getResource();
- Long result = jedis.ttl(key);
- jedis.close();
- return result;
- }
- @Override
- public Long incr(String key) {
- Jedis jedis = jedisPool.getResource();
- Long result = jedis.incr(key);
- jedis.close();
- return result;
- }
- @Override
- public Long hset(String key, String field, String value) {
- Jedis jedis = jedisPool.getResource();
- Long result = jedis.hset(key, field, value);
- jedis.close();
- return result;
- }
- @Override
- public String hget(String key, String field) {
- Jedis jedis = jedisPool.getResource();
- String result = jedis.hget(key, field);
- jedis.close();
- return result;
- }
- @Override
- public Long hdel(String key, String... field) {
- Jedis jedis = jedisPool.getResource();
- Long result = jedis.hdel(key, field);
- jedis.close();
- return result;
- }
- }
相关话题:JAVA初级、中级、高级,你是什么级别,你觉得不同级别应该要对哪些技能呢? 与阿里巴巴JAVA大神一起进行JAVA互动交流吧!
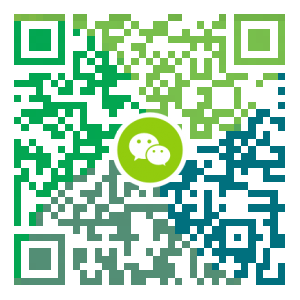
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
从R-CNN到YOLO,一文带你了解目标检测模型(附论文下载)
R-CNN R-CNN是2014年出现的。它是将CNN用于对象检测的起源,能够基于丰富的特征层次结构进行目标精确检测和语义分割来源。 如何确定这些边界框的大小和位置呢?R-CNN网络是这样做的:在图像中提出了多个边框,并判断其中的任何一个是否对应着一个具体对象。 要想进一步了解,可以查看以下PPT和笔记: http://www.image-net.org/challenges/LSVRC/2013/slides/r-cnn-ilsvrc2013-workshop.pdf http://www.cs.berkeley.edu/~rbg/slides/rcnn-cvpr14-slides.pdf http://zhangliliang.com/2014/07/23/paper-note-rcnn/ Fast R-CNN 2015年,R-CNN的作者R
- 下一篇
Java基础知识总结
一:java概述(快速浏览): 1991 年Sun公司的James Gosling等人开始开发名称为 Oak 的语言,希望用于控制嵌入在有线电视交换盒、PDA等的微处理器; 1994年将Oak语言更名为Java; Java的三种技术架构: JAVAEE:Java PlatformEnterpriseEdition,开发企业环境下的应用程序,主要针对web程序开发; JAVASE:Java Platform Standard Edition,完成桌面应用程序的开发,是其它两者的基础; JAVAME:Java PlatformMicro Edition,开发电子消费产品和嵌入式设备,如手机中的程序; 1,JDK:Java Development Kit,java的开发和运行环境,java的开发工具和jre。 2,JRE:Java Runtime Environment,java程序的运行环境,java运行的所需的类库+JVM(java虚拟机)。 3,配置环境变量:让java jdk\bin目录下的工具,可以在任意目录下运行,原因是,将该工具所在目录告诉了系统,当使用该工具时,由系统帮我...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- SpringBoot2整合Redis,开启缓存,提高访问速度
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- CentOS7,CentOS8安装Elasticsearch6.8.6
- SpringBoot2整合MyBatis,连接MySql数据库做增删改查操作
- CentOS6,7,8上安装Nginx,支持https2.0的开启
- 2048小游戏-低调大师作品
- CentOS7设置SWAP分区,小内存服务器的救世主
- CentOS8编译安装MySQL8.0.19
- CentOS6,CentOS7官方镜像安装Oracle11G