SpringMVC集成UEditor
UEditor是由百度开发的富文本web编辑器。其后端jsp代码实现的文件保存/读取路径受限于传统文件系统且只能在应用的webapp目录下, 故作出修改。但是暂没有使用官方后端代码,且只实现了图片上传下载功能。
1. 下载
- 下载地址:http://ueditor.baidu.com/website/download.html, 下载其中的
jsp版本
-
文件解压后目录结构如下所示
目录结构.png
2. 搭建项目
2.1. 新建一个maven项目
2.2. pom依赖
<properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <maven.compiler.source>1.7</maven.compiler.source> <maven.compiler.target>1.7</maven.compiler.target> <junit.version>4.11</junit.version> <spring.version>4.3.9.RELEASE</spring.version> <fileupload.version>1.3.2</fileupload.version> <commons.io.version>2.3</commons.io.version> <slf4j.version>1.6.4</slf4j.version> <jackson.version>2.8.7</jackson.version> <fastjson.version>1.2.4</fastjson.version> <servlet.api.version>3.0.1</servlet.api.version> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>${junit.version}</version> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>${jackson.version}</version> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>${commons.io.version}</version> </dependency> <dependency> <groupId>commons-fileupload</groupId> <artifactId>commons-fileupload</artifactId> <version>${fileupload.version}</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-log4j12</artifactId> <version>${slf4j.version}</version> </dependency> <dependency> <groupId>com.alibaba</groupId> <artifactId>fastjson</artifactId> <version>${fastjson.version}</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>${servlet.api.version}</version> </dependency> </dependencies>
- 2.3. spring-mvc.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <mvc:annotation-driven/> <context:component-scan base-package="com.github.brandonbai.springmvcueditordemo.controller" /> <bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"> <property name="defaultEncoding" value="utf-8" /> <property name="maxUploadSize" value="10485760000" /> <property name="maxInMemorySize" value="40960" /> </bean> <mvc:default-servlet-handler /> </beans>
- 2.4. applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:p="http://www.springframework.org/schema/p" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-4.0.xsd"> <context:component-scan base-package="com.github.brandonbai.springmvcueditordemo" /> </beans>
-
2.5. 将
ueditor
下载解压后目录下图目录结构.png
第1部分的代码拷贝到项目的webapp
下,如下图所示:项目结构.jpg 2.6. 将
ueditor
下载解压后目录下图目录结构.png
第2部分config.json的代码拷贝到项目的src/main/resources
下,如下图所示:
3. 前端配置
修改2.4图中的ueditor.config.js
的服务器请求路径
32 // 服务器统一请求接口路径 33 , serverUrl: URL + "./ueConvert"
4. 后端实现
- UEditorController.java
package com.github.brandonbai.springmvcueditordemo.controller; import java.io.BufferedReader; import java.io.File; import java.io.FileInputStream; import java.io.InputStreamReader; import java.io.PrintWriter; import java.text.SimpleDateFormat; import java.util.Date; import java.util.HashMap; import java.util.Map; import javax.servlet.ServletOutputStream; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.multipart.MultipartFile; import com.alibaba.fastjson.JSON; /** * * @author brandonbai * */ @Controller public class UEditorController { private static final String DIR_NAME = "~/Desktop"; private static final String PREFIX = "/editor/image"; private static final String FILE_SEPARATOR = File.separator; private static final String PATH_SEPARATOR = "/"; private static final String PATH_FORMAT = "yyyyMMddHHmmss"; private static final String CONFIG_FILE_NAME = "config.json"; private static final String ACTION_NAME_CONFIG = "config"; private static final String ACTION_NAME_UPLOAD_IMAGE = "uploadimage"; private static final Logger logger = LoggerFactory.getLogger(UEditorController.class); /** * 配置、图片处理 */ @RequestMapping("/ueConvert") public void ueditorConvert(HttpServletRequest request, HttpServletResponse response, String action, MultipartFile upfile) { try { request.setCharacterEncoding("utf-8"); response.setHeader("Content-Type", "text/html"); PrintWriter pw = response.getWriter(); if (ACTION_NAME_CONFIG.equals(action)) { String content = readFile(this.getClass().getResource(PATH_SEPARATOR).getPath() + CONFIG_FILE_NAME); pw.write(content); } else if (ACTION_NAME_UPLOAD_IMAGE.equals(action)) { Map<String, Object> map = new HashMap<String, Object>(16); String time = new SimpleDateFormat(PATH_FORMAT).format(new Date()); try { String originalFilename = upfile.getOriginalFilename(); String type = originalFilename.substring(originalFilename.lastIndexOf(".")); String dirName = DIR_NAME + PREFIX + FILE_SEPARATOR + time; File dir = new File(dirName); if(!dir.exists() || !dir.isDirectory()) { dir.mkdirs(); } String fileName = dirName + FILE_SEPARATOR + originalFilename; upfile.transferTo(new File(fileName)); map.put("state", "SUCCESS"); map.put("original", originalFilename); map.put("size", upfile.getSize()); map.put("title", fileName); map.put("type", type); map.put("url", "." + PREFIX + PATH_SEPARATOR + time + PATH_SEPARATOR + originalFilename); } catch (Exception e) { e.printStackTrace(); logger.error("upload file error", e); map.put("state", "error"); } response.setHeader("Content-Type", "application/json"); pw.write(JSON.toJSONString(map)); pw.close(); } } catch (Exception e) { e.printStackTrace(); } } /** * 图片读取 */ @RequestMapping(PREFIX + "/{time}/{path}.{type}") public void ueditorConvert(@PathVariable("time") String time, @PathVariable("path") String path, @PathVariable("type") String type, HttpServletRequest request, HttpServletResponse response) { try (FileInputStream fis = new FileInputStream(DIR_NAME + PREFIX + PATH_SEPARATOR + time + PATH_SEPARATOR + path + "." + type)) { int len = fis.available(); byte[] data = new byte[len]; fis.read(data); fis.close(); ServletOutputStream out = response.getOutputStream(); out.write(data); out.close(); } catch (Exception e) { logger.error("read file error", e); } } private String readFile(String path) { StringBuilder builder = new StringBuilder(); try(BufferedReader bfReader = new BufferedReader(new InputStreamReader(new FileInputStream(path), "UTF-8"))) { String tmpContent = null; while ((tmpContent = bfReader.readLine()) != null) { builder.append(tmpContent); } bfReader.close(); } catch (Exception e) { e.printStackTrace(); } return builder.toString().replaceAll("/\\*[\\s\\S]*?\\*/", ""); } }
5.演示
示例代码
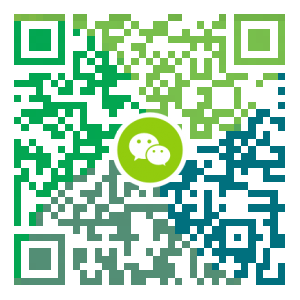
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
JavaScript高级程序设计学习(四)之引用类型
在javascript中也是有引用类型的,java同样如此。 javascript常见也比较常用的引用类型就熟Object和Array。 一个对象和一个数组,这个在前后端分离开发中也用的最多。比如ajax传参,有时我不仅仅只是需要一个参数,例如添加方法,传的是一个对象,对象存在属性。在java中对象也可以说类。因为类存在属性,例如人类,他的属性有身高,体重,姓名,年龄,性别等。而js对象,也可以这样,比如车,它可以有品牌,颜色,造型等等。 js对象可以做什么呢?同java对象有什么区别呢? 第一,如果要问题js对象可以做什么,最简单最直白最实用,我可以将一些公共属性放到这个对象中,然而我只需对象.属性,即可拥有。别说这个不常用,比如项目路径,全局变量等,都可以这样做。这样的话,代码会得到一定的规范。代码规范是很重要的,这个对于初学者而言,要牢记。不规范导致的只是无止休的重复劳动。 第二,js对象方便异步ajax传参,无论是react或者vue.js,我相信异步传参基本还是要用对象的; 作为IT从业者而言,时间对于我们就是金钱。时间是非常宝贵的,因为时间让我们增长自己的知识才能,在这个培...
- 下一篇
第二篇详细Python正则表达式操作指南(re使用)
接下来昨天的内容 执行匹配 一旦你有了已经编译了的正则表达式的对象,你要用它做什么呢?`RegexObject` 实例有一些方法和属性。这里只显示了最重要的几个,如果要看完整的列表请查阅 Python Library Reference 如果没有匹配到的话,match() 和 search() 将返回 None。如果成功的话,就会返回一个 `MatchObject` 实例,其中有这次匹配的信息:它是从哪里开始和结束,它所匹配的子串等等。 你可以用采用人机对话并用 re 模块实验的方式来学习它。如果你有 Tkinter 的话,你也许可以考虑参考一下 Tools/scripts/redemo.py,一个包含在 Python 发行版里的示范程序。 首先,运行 Python 解释器,导入 re 模块并编译一个 RE: 现在,你可以试着用 RE
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- CentOS8安装Docker,最新的服务器搭配容器使用
- Docker使用Oracle官方镜像安装(12C,18C,19C)
- CentOS8编译安装MySQL8.0.19
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- SpringBoot2整合MyBatis,连接MySql数据库做增删改查操作
- SpringBoot2整合Redis,开启缓存,提高访问速度
- SpringBoot2配置默认Tomcat设置,开启更多高级功能
- Hadoop3单机部署,实现最简伪集群
- CentOS7,CentOS8安装Elasticsearch6.8.6
- CentOS6,7,8上安装Nginx,支持https2.0的开启